1.概述 1.1为什么学? ? ●能够看懂公司里的代码 ? ●大数量下处理集合效率高?(并行流) ? ●代码可读性高 ? ●消灭嵌套地狱 ?
? 例如:
? java8之前代码:
//查询未成年作家的评分在70以上的书籍由于洋流影响所以作家和书籍可能出现重复,需要进行去重
List<Book> bookList = new ArrayList<>();
Set<Book> uniqueBookValues = new Hashset<>();
Set<Author> uniqueAuthorValues = new HashSet<>();
for(Author author :authors)
{
if (uniqueAuthorValues.add(author)) {
if (author.getAge() < 18) {
List<Book> books = author.getBooks();
for (Book book : books) {
if (book.getScore() > 70) {
if (uniqueBookValues.add(book)) {
bookList.add(book);
}
}
}
}
}
}
System.out.println(bookList);
使用函数式编程:
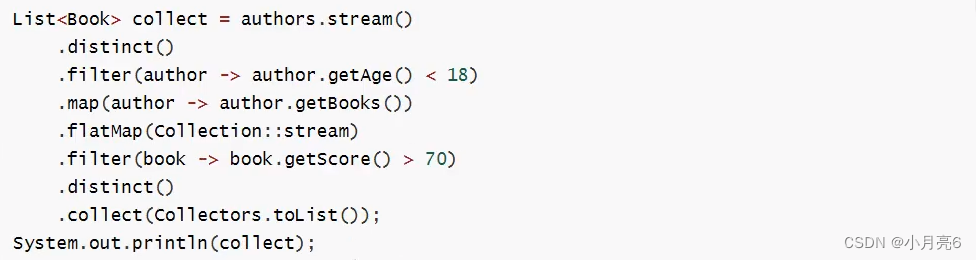
1.2函数式编程思想 ? 1.2.1概念 ? ? ? ? ? ?面向对象思想需要关注用什么对象完成什么事情。而函数式编程思想就类似于我们数学中的函数。它主要关注的是对数据进行了什么操作。 ? 1.2.2优点 ? ●代码简洁,开发快速 ? ●接近自然语言,易于理解 ? ●易于"并发编程"
2. Lambda表达式 2.1概述 ? ? ? Lambda是DK8中一个语法糖。他可以对某些匿名内部类的写法进行简化。它是函数式编程思想的一个重要体现。让我们不用关注是什么对象。而是更关注我们对数据进行了什么操作。 回
2.2核心原则 ? ? 可推导可省略 2.3基本格式 ? ?(参数列表)->{代码}
例一 我们在创建线程并启动时可以使用匿名内部类的写法:
public static void main(String[] args) {
new Thread(new Runnable() {
@Override
public void run() {
System.out.println("hello world");
}
}).start();
}
Lambda表示为:
new Thread(() -> System.out.println("hello world")).start();
? 这个匿名内部类必须是一个接口且必须只有一个抽象方法?
@FunctionalInterface
public interface Runnable {
/**
* When an object implementing interface <code>Runnable</code> is used
* to create a thread, starting the thread causes the object's
* <code>run</code> method to be called in that separately executing
* thread.
* <p>
* The general contract of the method <code>run</code> is that it may
* take any action whatsoever.
*
* @see java.lang.Thread#run()
*/
public abstract void run();
}
? Lambda表达式不关注类名(接口),也不关注方法名,只关注方法参数和方法体
? 刚开始写Lambda表达式可以直接省略到类名和方法名 ,加一个? -> 就可以了
? 例如Runnable
public static void main(String[] args) {
new Thread(
() -> {
System.out.println("hello world");
}).start();
}
根据Lamdba省略规则,进一步省略大括号,小括号等
Lambda表达式省略的都是JDK能识别出来的内容,省略的都是一些没有意义的代码
在Java8支持Lambda表达式以后,为了满足Lambda表达式的一些典型使用场景,JDK为我们提供了大量常用的函数式接口。它们主要在 java.util.function 包中
四大常用函数式接口:
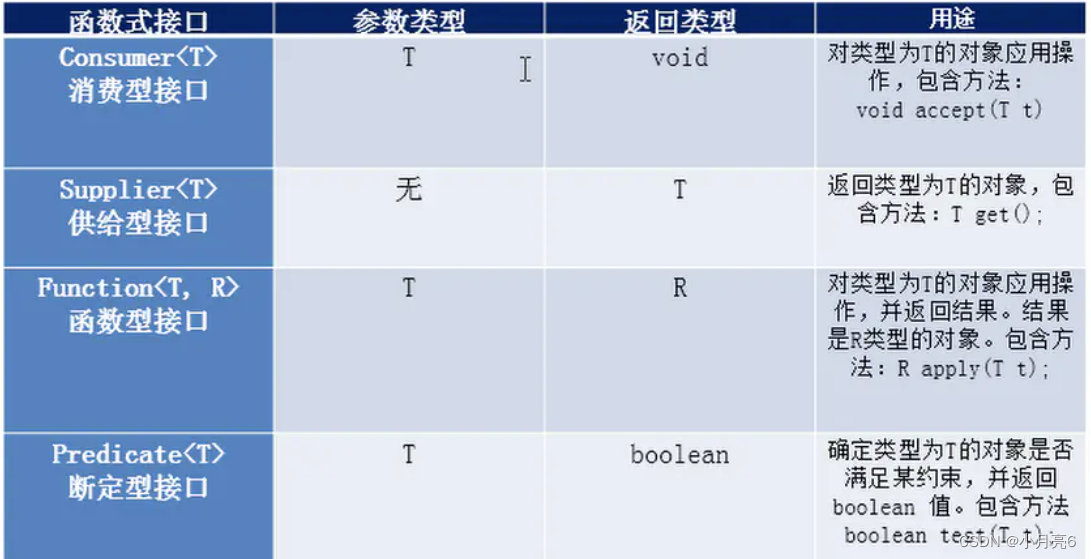
例2: idea自动生成Lambda表达式快捷键 ctrl + 回车 也可以从lambda表达式变成普通写法
public static void main(String[] args) {
int i = calculateNum((left, right) -> left + right);
System.out.println(i);
}
public static int calculateNum(IntBinaryOperator operator){
int a = 10;
int b = 20;
return operator.applyAsInt(a,b);
}
例 3:
public static void main(String[] args) {
printNum(new IntPredicate() {
@Override
public boolean test(int value) {
return value%2==0;
}
});
printNum((int value) ->
value%2==0
);
}
public static void printNum(IntPredicate predicate){
int[] arr = {1,2,3,4,5,6,7,8,9,10};
for (int i : arr) {
if (predicate.test(i)){
System.out.println(i);
}
}
}
例4:
public static void main(String[] args) {
Integer integer = typeConver(s -> Integer.valueOf(s));
System.out.println(integer+1);
String s = typeConver((String s)-> {
return "l" + s;
});
System.out.println(s);
}
public static <R> R typeConver(Function<String,R> function){
String str = "12345";
R result = function.apply(str);
return result;
}
例5 :
public static void main(String[] args) {
foreachArr(value -> System.out.println(value));
}
public static void foreachArr(IntConsumer consumer){
int[] arr = {1,2,3,4,5,6,7,8,9,10};
for (int i : arr) {
consumer.accept(i);
}
}
2.4省略规则 ●参数类型可以省略 ●方法体只有-句代码时大括号return和唯一 一句代码的分号可以省略 ●方法只有一个参数时小括号可以省略 ●以上这些规则都记不住也可以省略不记
3. Stream流 3.1概述 Java8的Stream使用的是函数式编程模式,如同它的名字一样,它可以被用来对集合或数组进行链状流式的操作。可以更方便的让我们对集合或数组操作。
3.2案例数据准备 ?
package Stream;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
@EqualsAndHashCode//用于后期的去重使用
public class Author {
//id
private Long id;
//姓名
private String name;
//年龄
private Integer age;
//简介
private String intro;
//作品
private List<Book> books;
}
@Data
@AllArgsConstructor
@NoArgsConstructor
@EqualsAndHashCode //用于后期的去重使用
public class Book {
// id
private Long id;
// 书名
private String name;
// 分类
private String category;
// 评分
private Integer score;
// 简介
private String intro;
}
//数据初始化
Author author = new Author(1L,"蒙多",33,"一个从菜刀中明悟哲理的祖安人",null);
Author author2 = new Author(2L,"亚拉索",15,"狂风也追逐不上他的思考速度",null);
Author author3 = new Author(3L,"易",14,"是这个世界在限制他的思维",null);
Author author4= new Author(3L,"易",14,"是这个世界在限制他的思维",null);
//书籍列表
List<Book> book1 = new ArrayList<>();
List<Book> book2 = new ArrayList<>();
List<Book> book3 = new ArrayList<>();
book1.add(new Book(1L,"刀的两侧是光明与黑暗","哲学,爱情",88,"用一把刀划分了爱恨"));
book1.add(new Book(2L,"一个人不能死在同-一把刀下","个人成长,爱情",99,"讲述如何从失败中明悟真理"));
book2.add(new Book(3L,"那风吹不到的地方","哲学" ,85,"带你用思维去领略世界的尽头"));
book2.add(new Book(3L,"那风吹不到的地方","哲学" ,85,"带你用思维去领略世界的尽头"));
book2.add(new Book(4L,"吹或不吹","爱情,个人传记" ,56,"一个哲学家的恋爱观注定很难把他所在的时代理解"));
book3.add(new Book(5L,"你的剑就是我的剑","爱情",56,"无法想象一个武者能对他的伴侣这么的宽容"));
book3.add(new Book(6L,"风与剑","个人传记",100,"两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?"));
book3.add(new Book(6L,"风与剑","个人传记",100,"两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?"));
author.setBooks(book1);
author2.setBooks(book2);
author3.setBooks(book3);
author4.setBooks(book3);
List<Author> authorList = new ArrayList<>(Arrays.asList(author, author2, author3, author4));
return authorList;
3.3快速入门 3.3.1需求 我们可以调用getAuthors方法获取到作家的集合。现在需要打印所有年龄小于1 8的作家的名字,并且要注意去重。
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.filter( author ->
author.getAge() < 18
)
.forEach(author -> System.out.println(author.getName()));
}
3.4常用操作 3.4.1创建流 单列集合:1集合对 象.stream()
List<Author> authors = getAuthors();
Stream<Author> stream = authors.stream();
数组:? Arrays .stream(数组)或者使用Stream. of来创建
Integer[] arr = {1,2,3,4,5};
Stream<Integer> stream = Arrays.stream(arr);
stream.forEach(integer -> System.out.println(integer));
//或
//Stream<Integer> stream = Arrays.stream(arr);
Stream<Integer> arr1 = Stream.of(arr);
arr1.forEach(integer -> System.out.println(integer));
双列集合:转换成单列集合后再创建
private static void test03() {
Map<String,Integer> map = new HashMap<>();
map.put("蜡笔小新",19);
map.put("黑子",17);
map.put("日向",16);
Set<Map.Entry<String, Integer>> entries = map.entrySet();
Stream<Map.Entry<String, Integer>> stream = entries.stream();
stream.filter(stringIntegerEntry -> stringIntegerEntry.getValue() > 16)
.forEach(stringIntegerEntry -> System.out.println(stringIntegerEntry.getKey() + stringIntegerEntry.getValue()));
}
idea debug查看?

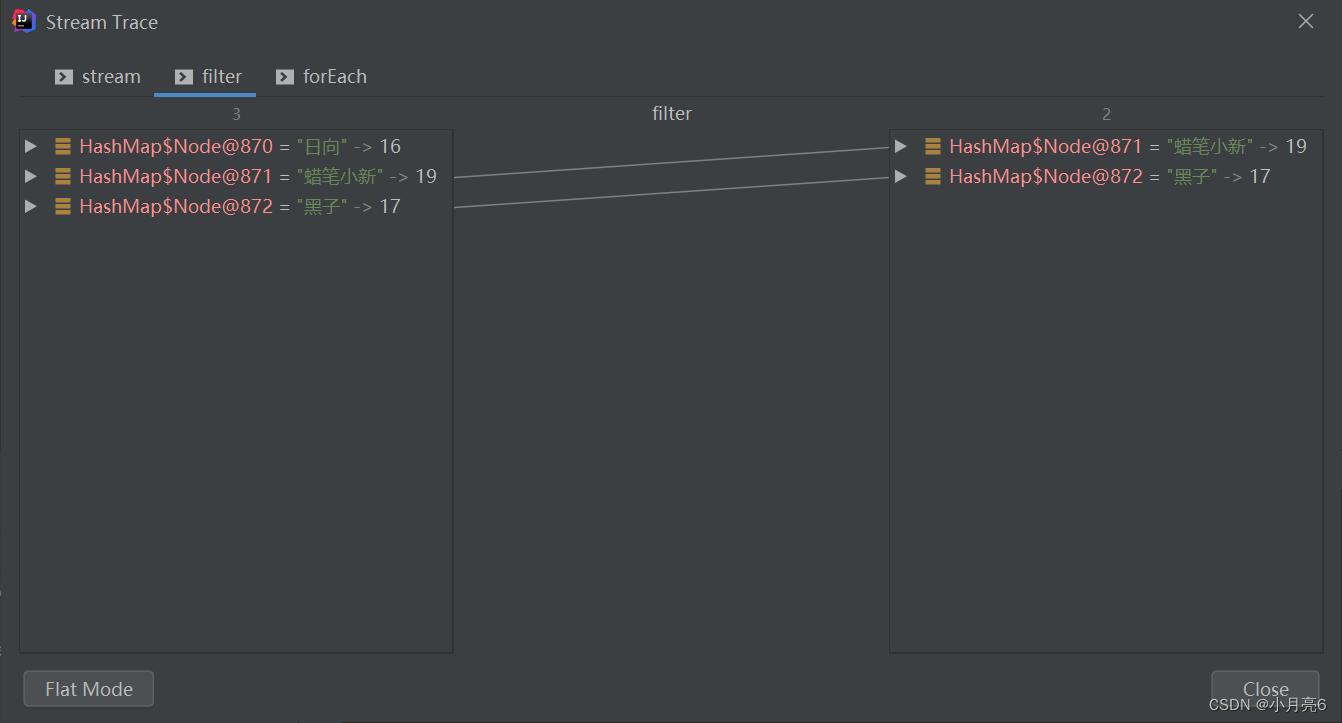
?3.4.2中间操作 ? filter ? 可以对流中的元素进行条件过滤,符合过滤条件的才能继续留在流中。
private static void test04() {
List<Author> authors = getAuthors();
//打印所有姓名长度大于1的作家的姓名
authors.stream().filter(author -> author.getName().length() > 1)
.forEach( author -> System.out.println(author));
}
map 转化类型或者计算
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getAge()) //获取所有作家的年龄 类型转换
.map(integer -> integer + 10) //对整数类型的年龄 加+10
.forEach(o -> System.out.println(o)); //输出
distinct
可以去除流中的重复的元素
注意: distinct方法是依赖Object的equals方法来判断是否是相同对象的。所以需要注意重写equals方法。 ?
//打印所有作家的姓名,并且要求其中不能有重复元素。
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.forEach(author -> System.out.println(author.getName()));
sorted 可以对流中的元索进行排序。
例如: 对流中的元素按照年龄进行降序排序,组要求不能有重复的元素。
空参:
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted()
.forEach(author -> System.out.println(author.getAge()));
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import java.util.Comparator;
import java.util.List;
import java.util.Objects;
@Data
@AllArgsConstructor
@NoArgsConstructor
@EqualsAndHashCode
public class Author implements Comparable<Author> {
//id
private Long id;
//姓名
private String name;
//年龄
private Integer age;
//简介
private String intro;
//作品
private List<Book> books;
@Override
public int compareTo(Author o) {
return this.getAge() - o.getAge();
}
/* @Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Author author = (Author) o;
return Objects.equals(id, author.id) &&
Objects.equals(name, author.name) &&
Objects.equals(age, author.age) &&
Objects.equals(intro, author.intro) &&
Objects.equals(books, author.books);
}
@Override
public int hashCode() {
return Objects.hash(id, name, age, intro, books);
}*/
}
?有参:
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o2.getAge() - o1.getAge())
.forEach(author -> System.out.println(author.getAge()));
注意:如果调用空参的sorted()方法,要流中的元素是实现了Comparable。
limit 可以设置流的最大长度,超出的部分将被抛弃。
例如: 对流中的元素按照年龄进行降序排序,组要求不能有重复的元素然后打印其中年龄最大的两个作家的姓名。 ?
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o1.getAge() - o2.getAge())
.limit(2)
.forEach(author -> System.out.println(author.getAge()));
skip 跳过流中的前n个元素,返回剩下的元素
例如: 打印除了年龄最大的作家外的其他作家,要求不能有重复元素,并且按照年龄降序排序。 ?
List<Author> authors = getAuthors();
authors.stream()
.sorted((o1,o2) -> o2.getAge() - o1.getAge())
.distinct()
.skip(1)
.forEach(author -> System.out.println(author.getAge()));
flatMap map只能把一个对象转换成另一 个对象来作为流中的元素。而flatMap可以把一个对象转换成多个对象作为流中的元素。 (简单来说,就是author 对应多个 Book,需要把book拼接成一个流,然后去重、输出)
例一: 打印所有书籍的名字。要求对重复的元索进行去重。 ?
List<Author> authors = getAuthors();
authors.stream().flatMap((Function<Author, Stream<Book>>) author -> author.getBooks().stream())
.distinct()
.forEach(book -> System.out.println(book.getName()));
例二:
打印现有数据的所有分类。要求对分类进行去重。不能出现这种格式:哲学,爱情 ?
List<Author> authors = getAuthors();
authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.flatMap((Function<Book, Stream<String>>) book -> Arrays.stream(book.getCategory().split(",")))
.filter(b -> !b.contains("爱情")&&!b.contains("小说"))
.distinct()
.forEach(c -> System.out.println(c));
3.4.3终结操作 forEach ? 对流中的元素进行遍历操作,我们通过传入的参数去指定对遍历到的元素进行什么具体操作。
例子: ? ? ? ?输出所有作家的名字
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.forEach(author -> System.out.println(author.getName()));
count 可以用来获取当前流中元素的个数。 例子: 打印这些作家的所出书籍的数目,注意删除重复元素。 ?
List<Author> authors = getAuthors();
long count = authors.stream().flatMap((Function<Author, Stream<Book>>) author -> author.getBooks().stream())
.distinct()
.count();
System.out.println(count);
max&min? 可以用来或者流中的最值。 例子: 分别获取这些作家的所出书籍的最高分和最低分并打印。
? max最高分:
List<Author> authors = getAuthors();
Optional<Book> max = authors.stream()
.flatMap(author -> author.getBooks().stream())
.max((score1, score2) -> score1.getScore() - score2.getScore());
System.out.println(max.get().getScore());
min最低分:
Optional<Book> min = authors.stream()
.flatMap(author -> author.getBooks().stream())
.min((score1, score2) -> score1.getScore() - score2.getScore());
System.out.println(min.get().getScore());
collect 把当前流转换成一个集合。
例子: ? ? ? ?获取一个存放所有作者名字的List集合。
List<Author> authors = getAuthors();
List<String> collect = authors.stream()
.map(author -> author.getName())
.collect(Collectors.toList());
System.out.println(collect);
? ? ?获取一个所有作者名的Set集合。 ?
List<Author> authors = getAuthors();
Set<String> collect = authors.stream()
.map(author -> author.getName())
.collect(Collectors.toSet());
Iterator<String> iterator = collect.iterator();
while (iterator.hasNext())
{
System.out.println(iterator.next());
}
获取一-个Map集合,map的key为作者名,value,为List <Book>
List<Author> authors = getAuthors();
Map<String, List<Book>> collect = authors.stream().distinct().collect(Collectors.toMap(author -> author.getName(), author -> author.getBooks()));
查找与匹配 ? ?anyMatch ? ?可以用来判断是否有任意符合匹配条件的元素,结果为boolean类型。 例子: 判断是否有年龄在29以上的作家 (只要有一个就返回true)
List<Author> authors = getAuthors();
boolean b = authors.stream()
.anyMatch(author -> author.getAge() > 29);
System.out.println(b);
allMatch 可以用来判断是否都符合匹配条件,结果为boolean类型。 如果都符合结果为true,否则结果为false. 例子: 判断是否所有的作家都是成年人(必须所有都满足才返回 true) ?
List<Author> authors = getAuthors();
boolean b = authors.stream()
.allMatch(author -> author.getAge() > 29);
System.out.println(b);
noneMatch 可以判断流中的元素是否都不符合匹配条件。如果都不符合结果为true,否则结果为false 例子: 判断作家是否都没有超过100岁的。
findAny 获取流中的任意- -个元素。该方法没有办法保证获取的一定是流中的第一个元素。 例子: 获取任意一个年龄大于18的作家,如果存在就输出他的名字 ?
List<Author> authors = getAuthors();
Optional<Author> any = authors.stream().filter(author -> author.getAge() > 18).findAny();
any.ifPresent(author -> System.out.println(author.getName()));
findFirst 获取流中的第一个元素。 例子: ? ? ? ?获取一个年龄最小的作家,并输出他的姓名。 ?
List<Author> authors = getAuthors();
Optional<Author> first = authors.stream()
.sorted((o1, o2) -> o1.getAge() - o2.getAge())
.findFirst();
first.ifPresent(author -> System.out.println(author.getName()));
reduce归并 对流中的数据按照你指定的计算方式计算出一个结果。(缩减操作) reduce的作用是把stream中的元素给组合起来,我们可以传入一个初始值,它会按照我们的计算方式依次拿流中的元素和在初始化值 的基础上进行计算,计算结果再和后面的元素计算。 他内部的计算方式如下: ?
? 获取所有作家的年龄和
//获取所有作家的年龄和
List<Author> authors = getAuthors();
Integer reduce = authors.stream()
.map(author -> author.getAge())
.distinct()
.reduce(0, (integer, integer2) -> integer + integer2);
System.out.println(reduce);
使用 reduce 求所有作者中年龄的最大值
//使用 reduce 求所有作者中年龄的最大值
Integer reduce = getAuthors().stream()
.map(author -> author.getAge())
.reduce(Integer.MIN_VALUE, (result, integer2) -> {
if (result < integer2) {
result = integer2;
}
return result;
});
System.out.println(reduce);
?reduce一个参数的重载形式内部的计算
?源码注解为,其实就是把数组第一个元素,赋值为result
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
//使用reduce 一个参数 求所有作者中年龄的最小值
Optional<Integer> reduce = getAuthors().stream().map(author -> author.getAge())
.reduce((result, integer2) -> {
if (result > integer2) {
result = integer2;
}
return result;
});
reduce.ifPresent(integer -> System.out.println(integer));
3.5注意事项 ●惰性求值(如果没有终结操作,中间操作是不会得到执行的) ●流是一次性的(一旦一个流对象经过一个终结操作后。这个流就不能再被使用) ●不会影响原数据(我们在流中可以多数据做很多处理。但是正常情况下是不会影响原来集合中的元素的。这往往也是我们期望的)
4. Optional 4.1概述 我们在编写代码的时候出现最多的就是空指针异常。所以在很多情况下我们需要做各种非空的判断。 例如:
private static void test26() {
Author author = getAuthor();
if (author != null){
System.out.println(author.getAge());
}
}
private static Author getAuthor() {
Author author = new Author(1L, "蒙多", 33, "一个从菜刀中明悟哲理的祖安人", null);
return null;
}
尤其是对象中的属性还是一个对象的情况下。这种判断会更多。 过多的判断语句会让我们的代码显得臃肿不堪。 所以在JDK8中引入了Optional,养成使用Optional的习惯后你可以写出更优雅的代码来避免空指针异常。 并且在很多函数式编程相关的API中也都用到了Optional,如果不会使用Optional也会对函数式编程的学习造成影响。
4.2使用 4.2.1创建对象? ? Optional就好像是包装类,可以把我们的具体数据封装Optional对象内部。 然后我们去使用Optional中封装好的方法操作封装进去的数据就可以非常优雅的避免空指针异常。(这个只是创建时不抛异常,使用时不一定,需要和ifPresent连用 才彻底不会抛异常)
我们一般使用Optional的静态方 法ofNullable来把数据封装成一个Optional对象。 无论传入的参数是否为null都不会出现问题。 ?
Author author = getAuthor();
Optional<Author> authorOptional = Optional.ofNullable(author);
? ?ofNullable 源码
public static <T> Optional<T> ofNullable(T value) {
return value == null ? empty() : of(value);
}
直接封装到方法体里会很方便
private static Optional<Author> getAuthorOptional() {
Author author = new Author(1L, "蒙多", 33, "一个从菜刀中明悟哲理的祖安人", null);
Optional<Author> author1 = Optional.ofNullable(author);
return author1;
}
而且在实际开发中我们的数据很多是从数据库获取的。Mybatis从3.5版本可以也已经支持Optiona了。我们可以直接把dao方法的返回值类型定义成Optional类型,MyBastis会自己把数据封装成Optional对象返回。封装的过程也不需要我们自己操作。
不建议使用下面一种创建方式: 如果你确定一个对象不是空的则可以使用Optional的静态方法of来把数据封装成Optional对象。
Author author = new Author();
Optional<Author> author1 = Optional.of(author);
如果为null 时会抛java.lang.NullPointerException异常
4.2.2安全消费值 我们获取到一个Optional对象后肯定需要对其中的数据进行使用。这时候我们可以使用其ifPresent方法对来消费其中的值。 这个方法会判断其内封装的数据是否为空,不为空时才会执行具体的消费代码。这样使用起来就更加安全了。 例如,以下写法就优雅的避免了空指针异常。
author1.ifPresent(author2 -> System.out.println(author2));
4.2.3获取值 如果我们想获取值自己进行处理可以使用get方法获取,但是不推荐。因为当Optional内部的数据为空的时候会出现异常。 ?
private static Optional<Author> getAuthorOptional() {
//Author author = new Author(1L, "蒙多", 33, "一个从菜刀中明悟哲理的祖安人", null);
Author author = null;
Optional<Author> author1 = Optional.ofNullable(author);
return author1;
}
? ?这种会抛异常 java.util.NoSuchElementException: No value present
Optional<Author> authorOptional = getAuthorOptional();
System.out.println(authorOptional.get());
4.2.4安全获取值 如果我们期望安全的获取值。我们不推荐使用get方法,而是使用Optional提供的以下方法。 ?●orElseGet ? ? ?获取数据并且设置数据为空时的默认值。如果数据不为空就能获取到该数据。如果为空则根据你传入的参数来创建对象作为默认值返回。
Optional<Author> authorOptional = getAuthorOptional();
Author author = authorOptional.orElseGet(() -> new Author(1L, "蒙多2", 33, "一个从菜刀中明悟哲理的祖安人", null));
System.out.println(author);
●orElseThrow 获取数据,如果数据不为空就能获取到该数据。如果为空则根据你传入的参数来创建异常抛出。
Optional<Author> authorOptional = getAuthorOptional();
try {
Author author = authorOptional.orElseThrow(() -> new RuntimeException("数据为null"));
System.out.println(author);
} catch (Throwable throwable) {
throwable.printStackTrace();
}
4.2.5过滤 我们可以使用filter方法对数据进行过滤。如果原本是有数据的,但是不符合判断,也会变成一个无数据的Optional对象。 ?
Optional<Author> authorOptional = getAuthorOptional();
Optional<Author> authorOptional1 = authorOptional
.filter(author -> author.getAge() > 88);
4.2.6判断 我们可以使用isPresent方法进行是否存在数据的判断。如果为空返回值为false,如果不为空,返回值为true。 ?
Optional<Author> authorOptional = getAuthorOptional();
boolean present = authorOptional.isPresent();
System.out.println(present);
4.2.7数据转换 Optional还提供了map可以让我们的对数据进行转换,并且转换得到的数据也还是被Optional包装好的,保证了我们的使用安全。 ? 例如 : 我们想获取作家的书籍集合。 ? 从Author ->List<Book>类型的转换
Optional<Author> authorOptional = getAuthorOptional();
Optional<List<Book>> books = authorOptional.map(author -> author.getBooks());
System.out.println(books);
5.函数式接口 5.1概述 只有一个抽象方法的接口我们称之为函数接口。 JDK的函数式接口都加上了@FunctionalInterface注解进行标识。但是无论是否加上该注解只要接口中只有一个抽象方法,都是函数式接口。
5.2常见函数式接口 ●Consumer消费接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中对传入的参数进行消费。
●Function计算转换接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中对传入的参数计算或转换,把结果返回
●Predicate判断接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中对传入的参数条件判断,返回判断结果
●Supplier产型接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中创建对象,把创建好的对象返回 ?
之前使用lambda表达式的式子都函数式接口,这里前面也有说过,下面都是,只要加@FunctionalInterface,必须只能有一个抽象方法的接
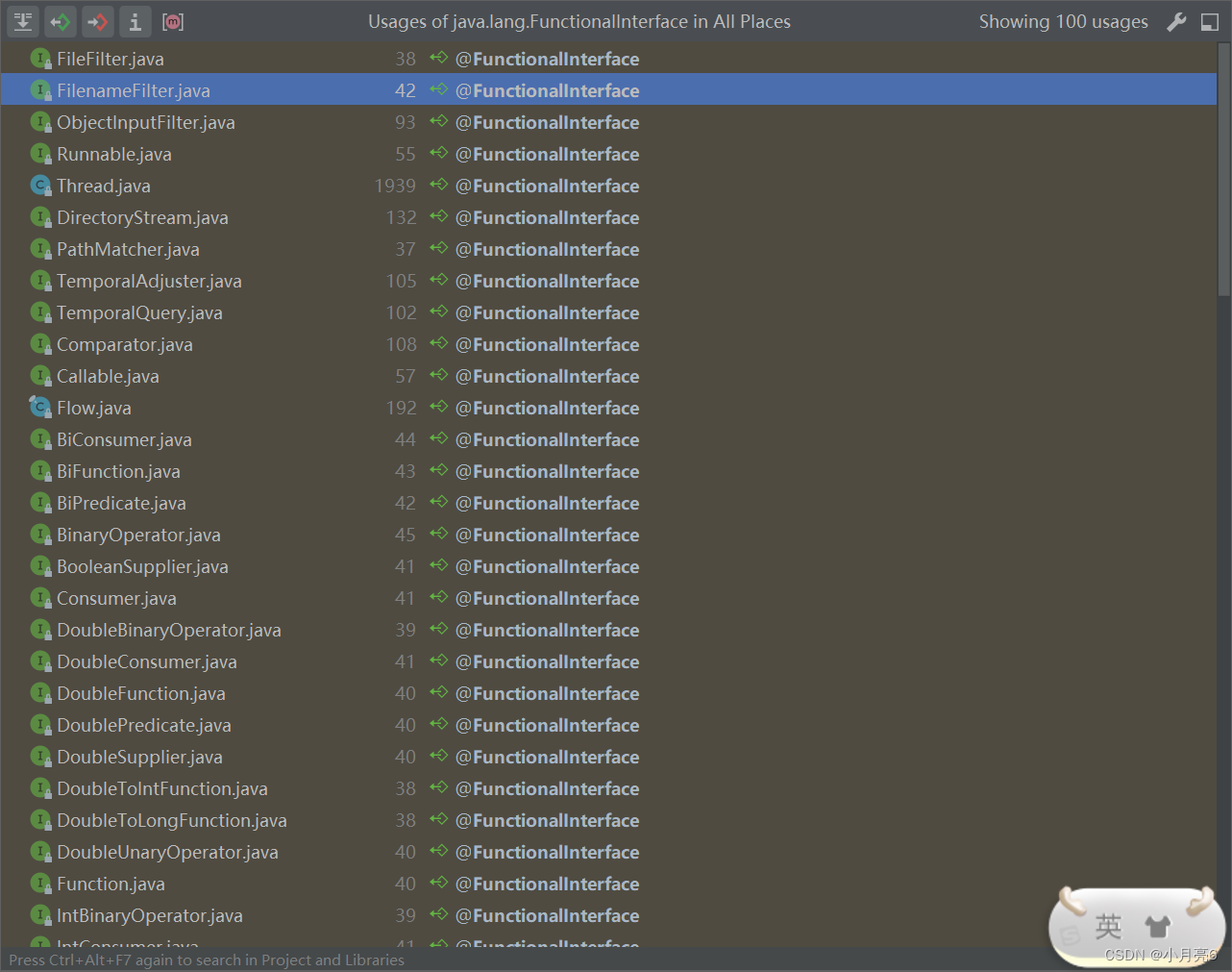
?5.3常用时默认方法
and 我们在使用Predicate接口时候可能需要进行判断条件的拼接。而and方法相当于是使用&&来拼接两个判断条件 例如: 打印作家中年龄大于17并且姓名的长度大于1的作家。 ?
List<Author> authors = getAuthors();
getAuthors().stream()
.filter(((Predicate<Author>) author -> author.getAge() > 17)
.and(author -> author.getName().length() > 1)).forEach(author -> System.out.println(author.getName()));
在自定方法中使用,效果更好
public static void printNum2(IntPredicate predicate,IntPredicate predicate2){
int[] arr = {1,2,3,4,5,6,7,8,9,10};
for (int i : arr) {
if (predicate.and(predicate2).test(i)){
System.out.println(i);
}
}
}
test:
printNum2(value -> value > 1, value -> value%2 ==0);
or 我们在使用Predicate接C时候可能需要进行判断条件的拼接。而or方法相当于是使用| |来拼接两个 判断条件。 例如: . 打印作家中年龄大于17或者姓名的长度小于2的作家。
getAuthors().stream().filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getName().equals("蒙多");
}
}.or(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge() == 14;
}
})).forEach(author -> System.out.println(author));
●negate Predicate接口中的方法。negate方法相当于 是在判断添加前面加了个!表示取反 例如: 打印作家中年龄不大于17的作家。 ?
//打印作家中年龄不大于17的作家。
getAuthors().stream().filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge() > 17;
}
}.negate()).forEach(author -> System.out.println(author.getAge()));
//Lambda
getAuthors().stream().filter(((Predicate<Author>) author -> author.getAge() > 17).negate()).forEach(author -> System.out.println(author.getAge()));
6.方法引用 我们在使用lambda时,如果方法体中只有- -个方法的调用的话(包括构造方法) ,我们可以用方法用进一步简化代码。
6.1推荐用法 我们在使用lambda时不需要考虑什么时候用方法引用,用哪种方法引用,方法引用的格式是什么。我们只需要在写完lambda方法发现 方法体只有一行代码, 并且是方法的调用时使用快捷键尝试是否能够转换成方法引用即可。当我们方法弓|用使用的多了慢慢的也可以直接写出方法引用。
6.2基本格式 类名或者对象名:方法名 ?
6.3语法详解(了解) 6.3.1引用静态方法 其实就是引|用类的静态方法 格式 ? ? ?类名::方法名
●使用前提 如果我们在重写方法的时候,方法体中只有一行代码,并粗这行代码是调用了某个类的静态方法,组我们把要重写的抽象方法中所有的参数都按照顺序传入了这个静态方法中,这个时候我们就可以用类的静态方法。 例如: 如一下代码就可以用方法引|用进行简化
List<Author> authors = getAuthors();
authors.stream().map(author -> author.getAge()).map(new Function<Integer, String>() {
@Override
public String apply(Integer age) {
return String.valueOf(age);
}
}).forEach(s -> System.out.println(s));
方法引用后:
List<Author> authors = getAuthors();
authors.stream().map(author -> author.getAge())
.map(String::valueOf)
.forEach(s -> System.out.println(s));
6.3.2引用对象的实例方法I 格式 ? ? ? 对象名::方法名 使用前提 如果我们在重写方法的时候,方法体中只有一行代码,且这行代码是调用了某个对象的成员方法,并且我们把要重写的抽象方法中所有的参数都按照顺序传入了这个成员方法中,这个时候我们就可以引|用对象的实例方法
例如: ?
StringBuilder sb = new StringBuilder();
getAuthors().stream().map(author -> author.getName())
.forEach(new Consumer<String>() {
@Override
public void accept(String s) {
sb.append(s);
}
});
方法引用后;
StringBuilder sb = new StringBuilder();
getAuthors().stream().map(author -> author.getName())
.forEach(sb::append);
6.3.4引朋类的实例方法 格式 ? ? 类名::方法名 使用前提 如果我们在重写方法的时候,方法体中只有一行代码,并且这行代码是调用了第一个参数的成员方法,并且我们把要重写的抽象方法中剩余的所有的参数都按照顺序传入了这个成员方法中,这个时候我们就可以引用类的实例方法。 例如: ?
private static void test37() {
String str = subAuthorName("李小龙", new UseString() {
@Override
public String use(String str, int start, int length) {
return str.substring(start, length);
}
});
System.out.println(str);
}
interface UseString {
String use(String str, int start, int length);
}
public static String subAuthorName(String str, UseString useString) {
int start = 0;
int length = 1;
return useString.use(str, start, length);
}
方法引用后:
String str = subAuthorName("李小龙", String::substring);
6.3.5构造器引用 如果方法体中的一-行代码是构造器的话就可以使用构造器弓|用。 格式 ? ? ? ? 类名: :new 使用前提 如果我们在重写方法的时候,方法体中只有一行代码,且这行代码是调用了某个类的构造方法,并且我们把要重写的抽象方法中的所有的参数都按照顺序传入了这个构造方法中,这个时候我们就可以构造器引用。 例如:
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getName())
.map(new Function<String, StringBuilder>() {
@Override
public StringBuilder apply(String s) {
return new StringBuilder(s);
}
}).forEach(stringBuilder -> System.out.println(stringBuilder));
方法引用后:
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getName())
.map(StringBuilder::new).forEach(stringBuilder -> System.out.println(stringBuilder));
7.高级用法 基本数据类型优化 ? ? ? 我们之前用到的很多Stream的方法由于都使用了泛型。所以涉及到的参数和返回值都是引用数据类型。 ? ? ? 即使我们操作的是整数小数,但是实际用的都是他们的包装类。JDK5中弓|入的自动装箱和自动拆箱让我们在使用对应的包装类时就好像使用基本数据类型一样方便。但是你一定要知道装箱和拆箱肯定是要消耗时间的。虽然这个时间消耗很下。但是在大量的数据不断的重复装箱拆箱的时候,你就不能无视这个时间损耗了。 所以为了让我们能够对这部分的时间消耗进行优化。Stream还提供了很多专门针对基本数据类型的方法。
?mapTolnt,mapToLong,mapToDouble,flatMapTolnt,flatMapToDouble等。
?例如:
getAuthors().stream().map(author -> author.getAge())
.map(age -> age + 10)
.filter(age -> age > 1)
.forEach(age -> System.out.println(age));
执行需 map 变成 mapToInt
getAuthors().stream().mapToInt(author -> author.getAge())
.map(age -> age + 10)
.filter(age -> age > 1)
.forEach(age -> System.out.println(age));
并行流
当流中有大元素时,我们可以使用并行流去提高操作的效率。实并行流就是把任务分配给多个线程完全。如果我们自己去用代码实现的话其实会非常的复杂,并且要求你对并发编程有足够的理解和认识。而如果我们使用Stream的话,我们只需要修改一个方法的调用就可以使用并行流来帮我们实现,从而提高效率。
parallel() 可以使执行程序从单线程变成多线程
不加时打印线程名称只有main
Integer[] arr = {1,2,3,4,5,6,7,8,9,10};
Stream<Integer> stream = Arrays.stream(arr);
Optional<Integer> reduce = stream.peek(integer -> System.out.println(Thread.currentThread().getName() + " num= "+ integer)).filter(integer -> integer > 5)
.reduce((result, ele) -> result + ele);
使用后后台打印线程名称
Integer[] arr = {1,2,3,4,5,6,7,8,9,10};
Stream<Integer> stream = Arrays.stream(arr);
Optional<Integer> reduce = stream.parallel().peek(integer -> System.out.println(Thread.currentThread().getName() + " num= "+ integer)).filter(integer -> integer > 5)
.reduce((result, ele) -> result + ele);
import lombok.val;
import java.util.*;
import java.util.function.*;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class T1 {
public static void main(String[] args) {
//List<Author> authors = getAuthors();
//Stream<Author> stream = authors.stream();
//test(authors);
//test2();
//test03();
//test04();
//test05();
//test06();
//test07();
//test08();
//test09();
//test10();
//test11();
//test12();
//test13();
//test14();
//test15();
//test16();
//test17();
//test18();
//test19();
//test20();
//test21();
//test22();
//test23();
//test24();
//test25();
//test26();
//test27();
//test28();
//test29();
//test30();
//test31();
//test32();
//test33();
//test34();
//testAnd();
//printNum2(value -> value > 1, value -> value%2 ==0);
//testOr();
//testNagate();
//test35();
//test36();
//test37();
//test38();
//test39();
test40();
}
private static void test40() {
Integer[] arr = {1,2,3,4,5,6,7,8,9,10};
Stream<Integer> stream = Arrays.stream(arr);
Optional<Integer> reduce = stream.parallel().peek(integer -> System.out.println(Thread.currentThread().getName() + " num= "+ integer)).filter(integer -> integer > 5)
.reduce((result, ele) -> result + ele);
System.out.println(reduce.get());
}
private static void test39() {
getAuthors().stream().mapToInt(author -> author.getAge())
.map(age -> age + 10)
.filter(age -> age > 1)
.forEach(age -> System.out.println(age));
}
private static void test38() {
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getName())
.map(StringBuilder::new).forEach(stringBuilder -> System.out.println(stringBuilder));
}
private static void test37() {
String str = subAuthorName("李小龙", String::substring);
System.out.println(str);
}
interface UseString {
String use(String str, int start, int length);
}
public static String subAuthorName(String str, UseString useString) {
int start = 0;
int length = 1;
return useString.use(str, start, length);
}
private static void test36() {
StringBuilder sb = new StringBuilder();
getAuthors().stream().map(author -> author.getName())
.forEach(sb::append);
}
private static void test35() {
List<Author> authors = getAuthors();
authors.stream().map(author -> author.getAge())
.map(String::valueOf)
.forEach(s -> System.out.println(s));
}
private static void testNagate() {
//打印作家中年龄不大于17的作家。
getAuthors().stream().filter(((Predicate<Author>) author -> author.getAge() > 17).negate()).forEach(author -> System.out.println(author.getAge()));
}
private static void testOr() {
getAuthors().stream().filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getName().equals("蒙多");
}
}.or(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge() == 14;
}
})).forEach(author -> System.out.println(author));
}
public static void printNum2(IntPredicate predicate, IntPredicate predicate2) {
int[] arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (int i : arr) {
if (predicate.and(predicate2).test(i)) {
System.out.println(i);
}
}
}
private static void testAnd() {
List<Author> authors = getAuthors();
getAuthors().stream()
.filter(((Predicate<Author>) author -> author.getAge() > 17)
.and(author -> author.getName().length() > 1)).forEach(author -> System.out.println(author.getName()));
}
private static void test34() {
Optional<Author> authorOptional = getAuthorOptional();
Optional<List<Book>> books = authorOptional.map(author -> author.getBooks());
System.out.println(books);
}
private static void test33() {
Optional<Author> authorOptional = getAuthorOptional();
boolean present = authorOptional.isPresent();
System.out.println(present);
}
private static void test32() {
Optional<Author> authorOptional = getAuthorOptional();
Optional<Author> authorOptional1 = authorOptional
.filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge() > 88;
}
});
System.out.println(authorOptional1);
}
private static void test31() {
Optional<Author> authorOptional = getAuthorOptional();
try {
Author author = authorOptional.orElseThrow(() -> new RuntimeException("数据为null"));
System.out.println(author);
} catch (Throwable throwable) {
throwable.printStackTrace();
}
}
private static void test30() {
Optional<Author> authorOptional = getAuthorOptional();
Author author = authorOptional.orElseGet(() -> new Author(1L, "蒙多2", 33, "一个从菜刀中明悟哲理的祖安人", null));
System.out.println(author);
}
private static void test29() {
Optional<Author> authorOptional = getAuthorOptional();
System.out.println(authorOptional.get());
}
private static void test28() {
Optional<Author> oof = Oof();
System.out.println(oof);
}
private static Optional<Author> Oof() {
Author author = new Author();
Optional<Author> author1 = Optional.of(null);
return author1;
}
private static Optional<Author> getAuthorOptional() {
Author author = new Author(1L, "蒙多", 33, "一个从菜刀中明悟哲理的祖安人", null);
//Author author = null;
List<Book> book1 = new ArrayList<>();
book1.add(new Book(1L, "刀的两侧是光明与黑暗", "哲学,爱情", 88, "用一把刀划分了爱恨"));
book1.add(new Book(2L, "一个人不能死在同-一把刀下", "个人成长,爱情", 99, "讲述如何从失败中明悟真理"));
author.setBooks(book1);
Optional<Author> author1 = Optional.ofNullable(author);
return author1;
}
private static void test27() {
Author author = getAuthor();
Optional<Author> authorOptional = Optional.ofNullable(author);
}
private static void test26() {
Author author = getAuthor();
if (author != null) {
System.out.println(author.getAge());
}
}
private static Author getAuthor() {
Author author = new Author(1L, "蒙多", 33, "一个从菜刀中明悟哲理的祖安人", null);
return null;
}
private static void test25() {
//使用reduce 求所有作者中年龄的最小值
Optional<Integer> reduce = getAuthors().stream().map(author -> author.getAge())
.reduce((result, integer2) -> {
if (result > integer2) {
result = integer2;
}
return result;
});
reduce.ifPresent(integer -> System.out.println(integer));
}
private static void test24() {
//使用 reduce 求所有作者中年龄的最大值
Integer reduce = getAuthors().stream()
.map(author -> author.getAge())
.reduce(Integer.MIN_VALUE, (result, integer2) -> {
if (result < integer2) {
result = integer2;
}
return result;
});
System.out.println(reduce);
}
private static void test23() {
//获取所有作家的年龄和
List<Author> authors = getAuthors();
Integer reduce = authors.stream()
.map(author -> author.getAge())
.distinct()
.reduce(0, (integer, integer2) -> integer + integer2);
System.out.println(reduce);
}
private static void test22() {
List<Author> authors = getAuthors();
Optional<Author> first = authors.stream()
.sorted((o1, o2) -> o1.getAge() - o2.getAge())
.findFirst();
first.ifPresent(author -> System.out.println(author.getName()));
}
private static void test21() {
List<Author> authors = getAuthors();
Optional<Author> any = authors.stream().filter(author -> author.getAge() > 18).findAny();
any.ifPresent(author -> System.out.println(author.getName()));
}
private static void test20() {
List<Author> authors = getAuthors();
boolean b = authors.stream()
.noneMatch(author -> author.getAge() > 29);
System.out.println(b);
}
private static void test19() {
List<Author> authors = getAuthors();
boolean b = authors.stream()
.allMatch(author -> author.getAge() > 29);
System.out.println(b);
}
private static void test18() {
List<Author> authors = getAuthors();
boolean b = authors.stream()
.anyMatch(author -> author.getAge() > 29);
System.out.println(b);
}
private static void test17() {
List<Author> authors = getAuthors();
Map<String, List<Book>> collect = authors.stream().distinct().collect(Collectors.toMap(author -> author.getName(), author -> author.getBooks()));
}
private static void test16() {
List<Author> authors = getAuthors();
Set<String> collect = authors.stream()
.map(author -> author.getName())
.collect(Collectors.toSet());
Iterator<String> iterator = collect.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
private static void test15() {
List<Author> authors = getAuthors();
List<String> collect = authors.stream()
.map(author -> author.getName())
.collect(Collectors.toList());
System.out.println(collect);
}
private static void test14() {
List<Author> authors = getAuthors();
Optional<Book> max = authors.stream()
.flatMap(author -> author.getBooks().stream())
.max((score1, score2) -> score1.getScore() - score2.getScore());
System.out.println(max.get().getScore());
Optional<Book> min = authors.stream()
.flatMap(author -> author.getBooks().stream())
.min((score1, score2) -> score1.getScore() - score2.getScore());
System.out.println(min.get().getScore());
}
private static void test13() {
List<Author> authors = getAuthors();
authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.flatMap((Function<Book, Stream<String>>) book -> Arrays.stream(book.getCategory().split(",")))
.filter(b -> !b.contains("爱情") && !b.contains("小说"))
.distinct()
.forEach(c -> System.out.println(c));
}
private static void test12() {
List<Author> authors = getAuthors();
long count = authors.stream().flatMap((Function<Author, Stream<Book>>) author -> author.getBooks().stream())
.distinct()
.count();
System.out.println(count);
}
private static void test11() {
List<Author> authors = getAuthors();
authors.stream()
.sorted((o1, o2) -> o2.getAge() - o1.getAge())
.distinct()
.skip(1)
.forEach(author -> System.out.println(author.getAge()));
}
private static void test10() {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o1.getAge() - o2.getAge())
.limit(2)
.forEach(author -> System.out.println(author.getAge()));
}
private static void test09() {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o2.getAge() - o1.getAge())
.forEach(author -> System.out.println(author.getAge()));
}
private static void test08() {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted()
.forEach(author -> System.out.println(author.getAge()));
}
private static void test07() {
//打印所有作家的姓名,并且要求其中不能有重复元素。
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.forEach(author -> System.out.println(author.getName()));
}
private static void test06() {
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getAge())
.map(integer -> integer + 10)
.forEach(o -> System.out.println(o));
}
private static void test05() {
List<Author> authors = getAuthors();
authors.stream().map(author -> author.getName())
.forEach(s -> System.out.println(s));
}
private static void test04() {
List<Author> authors = getAuthors();
//打印所有姓名长度大于1的作家的姓名
authors.stream().filter(author -> author.getName().length() > 1)
.forEach(author -> System.out.println(author));
}
private static void test03() {
Map<String, Integer> map = new HashMap<>();
map.put("蜡笔小新", 19);
map.put("黑子", 17);
map.put("日向", 16);
Set<Map.Entry<String, Integer>> entries = map.entrySet();
Stream<Map.Entry<String, Integer>> stream = entries.stream();
stream.filter(stringIntegerEntry -> stringIntegerEntry.getValue() > 16)
.forEach(stringIntegerEntry -> System.out.println(stringIntegerEntry.getKey() + stringIntegerEntry.getValue()));
}
private static void test2() {
Integer[] arr = {1, 2, 3, 4, 5};
//Stream<Integer> stream = Arrays.stream(arr);
Stream<Integer> arr1 = Stream.of(arr);
arr1.forEach(integer -> System.out.println(integer));
}
private static void test(List<Author> authors) {
authors.stream()
.distinct()
.filter(author ->
author.getAge() < 18
)
.forEach(author -> System.out.println(author.getName()));
}
private static List<Author> getAuthors() {
//数据初始化
Author author = new Author(1L, "蒙多", 33, "一个从菜刀中明悟哲理的祖安人", null);
Author author2 = new Author(2L, "亚拉索", 15, "狂风也追逐不上他的思考速度", null);
Author author3 = new Author(3L, "易", 14, "是这个世界在限制他的思维", null);
Author author4 = new Author(3L, "易", 14, "是这个世界在限制他的思维", null);
/* author2.setAge(14);
author2.setId(3L);
author2.setName("易");
author2.setIntro("是这个世界在限制他的思维");
author2.setBooks(null);
System.out.println(author2.hashCode());
System.out.println(author3.hashCode());
System.out.println(author4.hashCode());
System.out.println(author4);*/
//书籍列表
List<Book> book1 = new ArrayList<>();
List<Book> book2 = new ArrayList<>();
List<Book> book3 = new ArrayList<>();
book1.add(new Book(1L, "刀的两侧是光明与黑暗", "哲学,爱情", 88, "用一把刀划分了爱恨"));
book1.add(new Book(2L, "一个人不能死在同-一把刀下", "个人成长,爱情", 99, "讲述如何从失败中明悟真理"));
book2.add(new Book(3L, "那风吹不到的地方", "哲学", 85, "带你用思维去领略世界的尽头"));
book2.add(new Book(3L, "那风吹不到的地方", "哲学", 85, "带你用思维去领略世界的尽头"));
book2.add(new Book(4L, "吹或不吹", "爱情,个人传记", 56, "一个哲学家的恋爱观注定很难把他所在的时代理解"));
book3.add(new Book(5L, "你的剑就是我的剑", "爱情", 56, "无法想象一个武者能对他的伴侣这么的宽容"));
book3.add(new Book(6L, "风与剑", "个人传记", 100, "两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?"));
book3.add(new Book(6L, "风与剑", "个人传记", 100, "两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?"));
author.setBooks(book1);
author2.setBooks(book2);
author3.setBooks(book3);
author4.setBooks(book3);
List<Author> authorList = new ArrayList<>(Arrays.asList(author, author2, author3, author4));
return authorList;
}
}
视频连接:不会Lambda表达式、函数式编程?你确定能看懂公司代码?-java8函数式编程(Lambda表达式,Optional,Stream流)从入门到精通-最通俗易懂_哔哩哔哩_bilibili
|