1. AnnotatedElement接口
AnnotatedElement接口表示目前正在此 JVM 中运行的程序的一个已注释元素,该接口允许反射性地读取注释。
该接口主要有如下几个实现类:
- Class:类定义
- Constructor:构造器定义
- Field:类的成员变量定义
- Method:类的方法定义
- Package:类的包定义
调用AnnotatedElement对象的如下方法可以访问Annotation信息:
getAnnotation(Class<T>annotationClass) :返回该程序元素上存在的指定类型的注释,如果该类型的注释不存在,则返回null。Annotation[] getAnnotations() :返回此元素上存在的所有注释。boolean isAnnotationPresent(Class<?extendsAnnotation>annotationClass) :判断该程序元素上是否存在指定类型的注释,如果存在则返回true,否则返回false。Annotation[] getDeclaredAnnotations() :返回直接存在于此元素上的所有注释。与此接口中的其他方法不同,该方法将忽略继承的注释。
2. Method类实现了AnnotatedElement接口
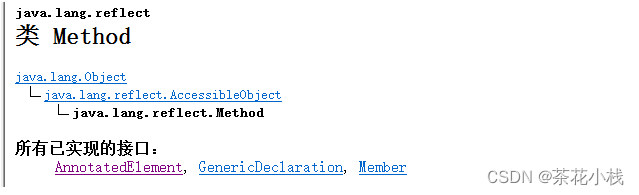
3. 获取方法上的注解
① 自定义注解
@Retention(value = RetentionPolicy.RUNTIME)
@Target(value = {ElementType.METHOD, ElementType.PARAMETER, ElementType.FIELD,ElementType.TYPE})
public @interface MyAnnotation {
public int age();
public String name() default "张三";
}
② 给Test类的show1方法上加3个注解: @ResponseBody、 @PostMapping、@MyAnnotation(name = “李四”,age=12)
@ControllerAdvice
@Controller
public class Test {
@ResponseBody
@PostMapping
@MyAnnotation(name = "李四",age=12)
public void show1(){
System.out.println("111");
}
@ResponseBody
@PostMapping
@MyAnnotation(name = "李四",age=12)
public void show2(){
System.out.println("111");
}
}
③ 获取show1() 方法上的指定类型的注解
public class Main {
public static void main(String[] args) throws NoSuchMethodException, ClassNotFoundException {
Class<?> clazz = Class.forName("com.example.redislock.annotation.Test");
Method method = clazz.getMethod("show1");
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
int age = annotation.age();
String name = annotation.name();
System.out.println("name="+name+", age="+age);
}
}
 ④ 判断方法上是否存在指定类型的注解,并获取指定类型的注解
public class Main {
public static void main(String[] args) throws NoSuchMethodException, ClassNotFoundException {
Class<?> clazz = Class.forName("com.example.redislock.annotation.Test");
Method[] methods = clazz.getMethods();
for (Method method : methods) {
if(method.isAnnotationPresent(MyAnnotation.class)){
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
int age = annotation.age();
String name = annotation.name();
System.out.println("name="+name+", age="+age);
}
}
}
}
 ⑤ 获取所有方法的所有注解
public class Main {
public static void main(String[] args) throws NoSuchMethodException, ClassNotFoundException {
Class<?> clazz = Class.forName("com.example.redislock.annotation.Test");
Method[] methods = clazz.getMethods();
for (Method method : methods) {
Annotation[] annotations = method.getAnnotations();
for (Annotation annotation : annotations) {
if(annotation instanceof MyAnnotation){
MyAnnotation myAnnotation = (MyAnnotation) annotation;
int age = myAnnotation.age();
String name = myAnnotation.name();
System.out.println("name="+name+", age="+age);
}
}
}
}
}

|