问:请描述下Spring Bean的生命周期
答:简单的说就3步:
1、创建BeanDefination阶段 2、创建Bean阶段 3、销毁Bean阶段
创建BeanDefination阶段
1.BeanFactory通过xml、注解配置类创建BeanDefination 2.通过BeanFactoryPostProcessor.postProcessBeanFactory()对BeanFination进行修改
创建Bean阶段
1.反射调用构造函数,创建初始化的Bean对象 2.注入@Autowired 3.注入Aware对象 4.BeanPostProcessor的postProcessBeforeInitialization() 5.@PostConstruct 6.InitializingBean.afterPropertiesSet() 7.<bean init-method> 8.BeanPostProcessor.postProcessAfterInitialization()
销毁Bean阶段
1.@PreDestroy 2.DisposableBean.destroy() 3.<bean destroy-method>
测试代码
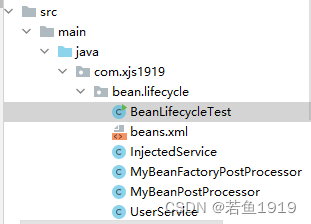
public class BeanLifecycleTest {
public static void main(String[] args) {
AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext();
ctx.scan("com.xjs1919.bean.lifecycle");
ctx.refresh();
ctx.close();
}
}
@Service
public class MyBeanFactoryPostProcessor implements BeanFactoryPostProcessor {
@Override
public void postProcessBeanFactory(ConfigurableListableBeanFactory configurableListableBeanFactory) throws BeansException {
BeanDefinition beanDefinition = configurableListableBeanFactory.getBeanDefinition("userService");
if(beanDefinition != null){
System.out.println("BeanFactoryPostProcessor.postProcessBeanFactory()");
}
}
}
@Service
public class MyBeanPostProcessor implements BeanPostProcessor {
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
if(bean.getClass() == UserService.class){
System.out.println("BeanPostProcessor.postProcessBeforeInitialization()");
}
return bean;
}
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
if(bean.getClass() == UserService.class){
System.out.println("BeanPostProcessor.postProcessAfterInitialization()");
}
return bean;
}
}
@Service
@ImportResource("classpath:com/xjs1919/bean/lifecycle/beans.xml")
public class UserService implements InitializingBean,DisposableBean,ApplicationContextAware {
@Autowired
private InjectedService injectedService;
public UserService(){
System.out.println("Constructor, injectedService="+injectedService);
}
public void setApplicationContext(ApplicationContext context) throws BeansException{
System.out.println("Aware, injectedService="+injectedService);
}
@Override
public void afterPropertiesSet() throws Exception {
System.out.println("InitializingBean.afterPropertiesSet()");
}
@Override
public void destroy() throws Exception {
System.out.println("DisposableBean.destroy()");
}
@PostConstruct
public void postConstruct(){
System.out.println("@PostConstruct");
}
@PreDestroy
public void preDestroy(){
System.out.println("@PreDestroy");
}
public void initMethod() {
System.out.println("initMethod");
}
public void destroyMethod(){
System.out.println("destroyMethod");
}
}
@Service
public class InjectedService {
}
beans.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="userService"
class="com.xjs1919.bean.lifecycle.UserService" init-method="initMethod" destroy-method="destroyMethod"/>
</beans>
输出结果:
BeanFactoryPostProcessor.postProcessBeanFactory()
Constructor, injectedService=null
Aware, injectedService=com.xjs1919.bean.lifecycle.InjectedService@5e4c8041
BeanPostProcessor.postProcessBeforeInitialization()
@PostConstruct
InitializingBean.afterPropertiesSet()
initMethod
BeanPostProcessor.postProcessAfterInitialization()
@PreDestroy
DisposableBean.destroy()
destroyMethod
|