1. 线程同步方法
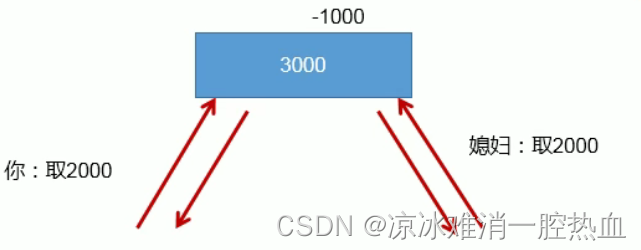
-
关于同步方法的踪迹:
- 同步方法仍然涉及到同步监视器,只是不需要我们显式的声明
- 非静态的同步方法,同步监视器是:this
- 静态的同步方法,同步监视器是:当前类本身
package ThreadSynchronizationTest;
import static java.lang.Thread.sleep;
class windows implements Runnable {
private int ticket = 10;
Object obj = new Object();
@Override
public void run() {
synchronized(this) {
while (true) {
try {
sleep(100);
} catch (Exception e) {
e.printStackTrace();
}
if (ticket > 0) {
System.out.println(Thread.currentThread().getName() + "售出票号为: " + ticket + "的票");
ticket--;
} else {
break;
}
}
}
}
}
class windows2 extends Thread {
private static int ticket = 10;
private static Object obj = new Object();
@Override
public void run() {
synchronized(windows2.class) {
while (true) {
try {
sleep(100);
} catch (Exception e) {
e.printStackTrace();
}
if (ticket > 0) {
System.out.println(getName() + "售出票号为: " + ticket + "的票");
ticket--;
} else {
break;
}
}
}
}
}
public class ThreadWindowsDemo {
public static void main(String[] args) {
ThreadWindowsDemo demo = new ThreadWindowsDemo();
demo.method();
System.out.println("--------------------------");
}
public void method() {
windows w = new windows();
Thread t1 = new Thread(w, "window_1");
Thread t2 = new Thread(w, "window_2");
Thread t3 = new Thread(w, "window_3");
t1.start();
t2.start();
t3.start();
}
public void method2() {
windows2 w2_1 = new windows2();
windows2 w2_2 = new windows2();
windows2 w2_3 = new windows2();
w2_1.setName("window2_1");
w2_2.setName("window2_2");
w2_3.setName("window2_3");
w2_1.start();
w2_2.start();
w2_3.start();
}
}
package ThreadSynchronizationTest;
import static java.lang.Thread.sleep;
class windows3 implements Runnable {
private int ticket = 100;
@Override
public void run() {
while (ticket > 0) {
show();
}
}
private synchronized void show() {
try {
sleep(10);
} catch (Exception e) {
e.printStackTrace();
}
if (ticket > 0) {
System.out.println(Thread.currentThread().getName() + "售出票号为: " + ticket + "的票");
ticket--;
}
}
}
class windows4 extends Thread {
private static int ticket = 100;
@Override
public void run() {
while (ticket > 0) {
show();
}
}
private static synchronized void show() {
try {
sleep(10);
} catch (Exception e) {
e.printStackTrace();
}
if (ticket > 0) {
System.out.println(Thread.currentThread().getName() + "售出票号为: " + ticket + "的票");
ticket--;
}
}
}
public class ThreadWindowsDemo2 {
public static void main(String[] args) {
ThreadWindowsDemo2 demo = new ThreadWindowsDemo2();
System.out.println("--------------------------");
demo.method4();
}
public void method3() {
windows3 w = new windows3();
Thread t1 = new Thread(w, "window3_1");
Thread t2 = new Thread(w, "window3_2");
Thread t3 = new Thread(w, "window3_3");
t1.start();
t2.start();
t3.start();
}
public void method4() {
windows4 w4_1 = new windows4();
windows4 w4_2 = new windows4();
windows4 w4_3 = new windows4();
w4_1.setName("window4_1");
w4_2.setName("window4_2");
w4_3.setName("window4_3");
w4_1.start();
w4_2.start();
w4_3.start();
}
}
2. 线程安全的单例模式之懒汉式
- 通过同步方法/同步代码块 将单例模式设置为线程安全的
- 可以通过外加判断来提高 线程安全懒汉式单例模式的效率
package SingletonPatternTest;
public class SingletonBankTest {
public static void main(String[] args) {
}
}
class Bank {
private Bank() {}
private static Bank instance = null;
public static Bank getInstance() {
if (instance == null) {
synchronized (Bank.class) {
if (instance == null) {
instance = new Bank();
}
return instance;
}
}
}
}
|