依赖
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.6.2</version>
</dependency>
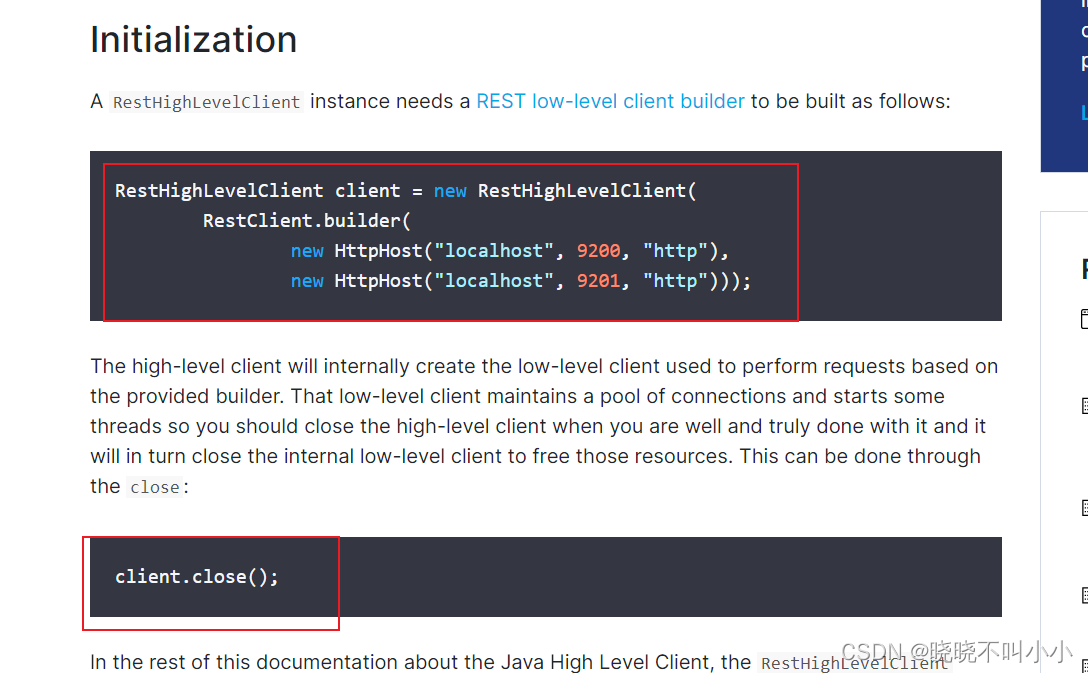
配置基本项目:
?配置类:
package com.li.esapi.config;
import org.apache.http.HttpHost;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestHighLevelClient;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ElasticSearchClientConfig {
/*
spring 两步骤
1、找对象
2、放到spring中待用
*/
@Bean
public RestHighLevelClient restHighLevelClient() {
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http")
// 配置集群
/*,new HttpHost("localhost", 9201, "http")*/));
return client;
}
}
1.创建索引
2.判断索引是否存在
3.删除索引
4.创建文档
5.CRUD文档
package com.li.esapi;
import com.alibaba.fastjson.JSON;
import com.li.esapi.pojo.Users;
import org.elasticsearch.action.admin.indices.delete.DeleteIndexRequest;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.delete.DeleteRequest;
import org.elasticsearch.action.delete.DeleteResponse;
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.action.support.master.AcknowledgedResponse;
import org.elasticsearch.action.update.UpdateRequest;
import org.elasticsearch.action.update.UpdateResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.client.indices.CreateIndexRequest;
import org.elasticsearch.client.indices.CreateIndexResponse;
import org.elasticsearch.client.indices.GetIndexRequest;
import org.elasticsearch.common.unit.TimeValue;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.query.MatchAllQueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.index.query.TermQueryBuilder;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import org.elasticsearch.search.fetch.subphase.FetchSourceContext;
import org.elasticsearch.search.fetch.subphase.highlight.HighlightBuilder;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.test.context.SpringBootTest;
import java.io.IOException;
import java.util.ArrayList;
import java.util.concurrent.TimeUnit;
@SpringBootTest
class EsApiApplicationTests {
@Autowired
@Qualifier("restHighLevelClient")
private RestHighLevelClient client;
// 测试索引的创建 request
@Test
void testCreateIndex() throws IOException {
// 1.创建索引请求
CreateIndexRequest request = new CreateIndexRequest("li_index");
// 2.客户端执行请求 indicesClient , 请求后获得响应
CreateIndexResponse createIndexResponse =
client.indices().create(request, RequestOptions.DEFAULT);
System.out.println(createIndexResponse);
client.close();
}
// 测试获取索引 只能判断是否存在
@Test
void testExistIndex() throws IOException {
GetIndexRequest request = new GetIndexRequest("li_index");
boolean exists = client.indices().exists(request, RequestOptions.DEFAULT);
System.out.println(exists);
client.close();
}
// 测试删除索引
@Test
void testDeleteIndex() throws IOException {
DeleteIndexRequest request = new DeleteIndexRequest("li_index");
//删除
AcknowledgedResponse delete = client.indices().delete(request, RequestOptions.DEFAULT);
// 判断是否删除成功
System.out.println(delete.isAcknowledged());
System.out.println(delete);
client.close();
}
// 测试添加文档
@Test
void testAddDocument() throws IOException {
// 创建对象
Users users = new Users("阿离", 18);
// 创建请求
IndexRequest request = new IndexRequest("li_index");
// 规则 put /li_index/_doc/1
request.id("id");
// request.timeout("1s"); 于下方?
request.timeout(TimeValue.timeValueSeconds(1));
// 将我们的数据放入请求 json
IndexRequest source = request.source(JSON.toJSON(users), XContentType.JSON);
// 客户端发送请求 , 获取响应的结果
IndexResponse indexResponse = client.index(request, RequestOptions.DEFAULT);
System.out.println(indexResponse.toString());
// 查看状态
System.out.println(indexResponse.status());
client.close();
}
// 获取文档, 判断是否存在 get /index/doc/1
@Test
void testIsExists() throws IOException {
GetRequest request = new GetRequest("li_index", "1");
// 不获取返回 的_source 的上下文了
request.fetchSourceContext(new FetchSourceContext(false));
request.storedFields("_none_");
boolean exists = client.exists(request, RequestOptions.DEFAULT);
System.out.println(exists);
client.close();
}
// 获取文档信息
@Test
void testGetDocument() throws IOException {
GetRequest request = new GetRequest("li_index", "1");
GetResponse response = client.get(request, RequestOptions.DEFAULT);
// 打印文档的内容
System.out.println(response.getSourceAsString());
// 返回全部内容和命令一样
System.out.println(response);
client.close();
}
// 更新文档信息
@Test
void testUpdateDocument() throws IOException {
UpdateRequest updateRequest = new UpdateRequest("li_index", "1");
updateRequest.timeout("1s");
Users user = new Users("还是阿离", 18);
updateRequest.doc(JSON.toJSONString(user), XContentType.JSON);
UpdateResponse response = client.update(updateRequest, RequestOptions.DEFAULT);
System.out.println(response.status()); // OK
client.close();
}
// 删除文档记录
@Test
void testDeleteDocument() throws IOException {
DeleteRequest request = new DeleteRequest("li_index", "1");
request.timeout("1s");
DeleteResponse deleteResponse = client.delete(request, RequestOptions.DEFAULT);
System.out.println(deleteResponse.status());
}
// 批量插入数据
@Test
void testBulkRequest() throws IOException {
BulkRequest bulkRequest = new BulkRequest();
bulkRequest.timeout("10s");
ArrayList<Users> users = new ArrayList<>();
users.add(new Users("li1", 18));
users.add(new Users("li2", 18));
users.add(new Users("li3", 18));
users.add(new Users("ling1", 18));
users.add(new Users("ling2", 18));
users.add(new Users("ling3", 18));
// 批处理请求
for (int i = 0; i < users.size(); i++) {
bulkRequest.add(new IndexRequest("li_index")
.id("" + (i + 1)) // 不写id 会生成一个随机id
.source(JSON.toJSONString(users.get(i)), XContentType.JSON));
}
BulkResponse bulkResponse = client.bulk(bulkRequest, RequestOptions.DEFAULT);
// 是否失败 false 代表成功
System.out.println(bulkResponse.hasFailures());
}
/**
*查询
* SearchRequest 搜索请求
* SearchSourceBuilder 条件构造
* HighlightBuilder 构建高亮
* TermQueryBuilder 精准查询
* MatchAllQueryBuilder 匹配所有
* XXXQueryBuilder 对应执行的命令
* @throws IOException
*/
@Test
void testSearch() throws IOException {
SearchRequest searchRequest = new SearchRequest("li_index");
// 构建搜索条件
SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();
// 查询条件,我们可以使用 QueryBuilders 工具类实现
// QueryBuilders.termQuery 精确查询
// QueryBuilders.matchAllQuery() 匹配所有
TermQueryBuilder termQueryBuilder = QueryBuilders.termQuery("name", "li1");
// MatchAllQueryBuilder matchAllQueryBuilder = QueryBuilders.matchAllQuery();
sourceBuilder.query(termQueryBuilder);
/*sourceBuilder.from(); 开始页
sourceBuilder.size(); 每页条数*/
sourceBuilder.timeout(new TimeValue(60, TimeUnit.SECONDS));
searchRequest.source(sourceBuilder);
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
System.out.println(JSON.toJSONString(searchResponse.getHits()));
System.out.println("======================================");
for (SearchHit hit : searchResponse.getHits().getHits()) {
System.out.println(hit.getSourceAsMap());
}
}
}
?依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
推荐视频:?【狂神说Java】ElasticSearch7.6.x最新完整教程通俗易懂_哔哩哔哩_bilibili
|