1.单文件上传到服务器
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="/upload" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="提交">
</form>
</body>
</html>
fileController
@RestController
public class fileuploadController {
/**
* 按日期分类
*/
SimpleDateFormat sdf=new SimpleDateFormat("/yyyy/MM/dd/");
@RequestMapping("/upload")
public String upliad(MultipartFile file, HttpServletRequest req){
//获取临时目录
String realpath=req.getServletContext().getRealPath("/");
//最终路径
String format = sdf.format(new Date());
String path=realpath+ format;
File folder=new File(path);
if (!folder.exists()){
folder.mkdirs();
}
String oldName = file.getOriginalFilename();
String newName= UUID.randomUUID().toString()+oldName.substring(oldName.lastIndexOf("."));
try {
file.transferTo(new File(path+newName));
String newName1 =req.getScheme()+"://"+req.getServerName()+":"+req.getServerPort()+req.getContextPath()+format+ newName;
return newName1;
// return path;
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
}
返回结果
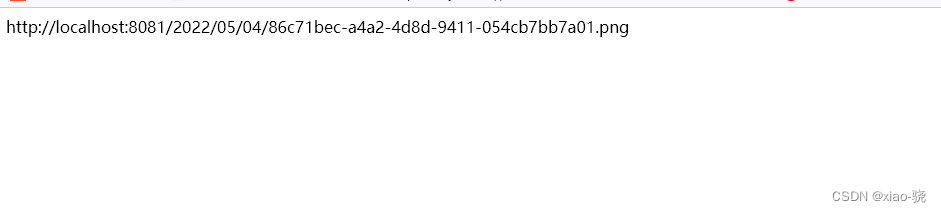
打开链接就可以看见上传的文件
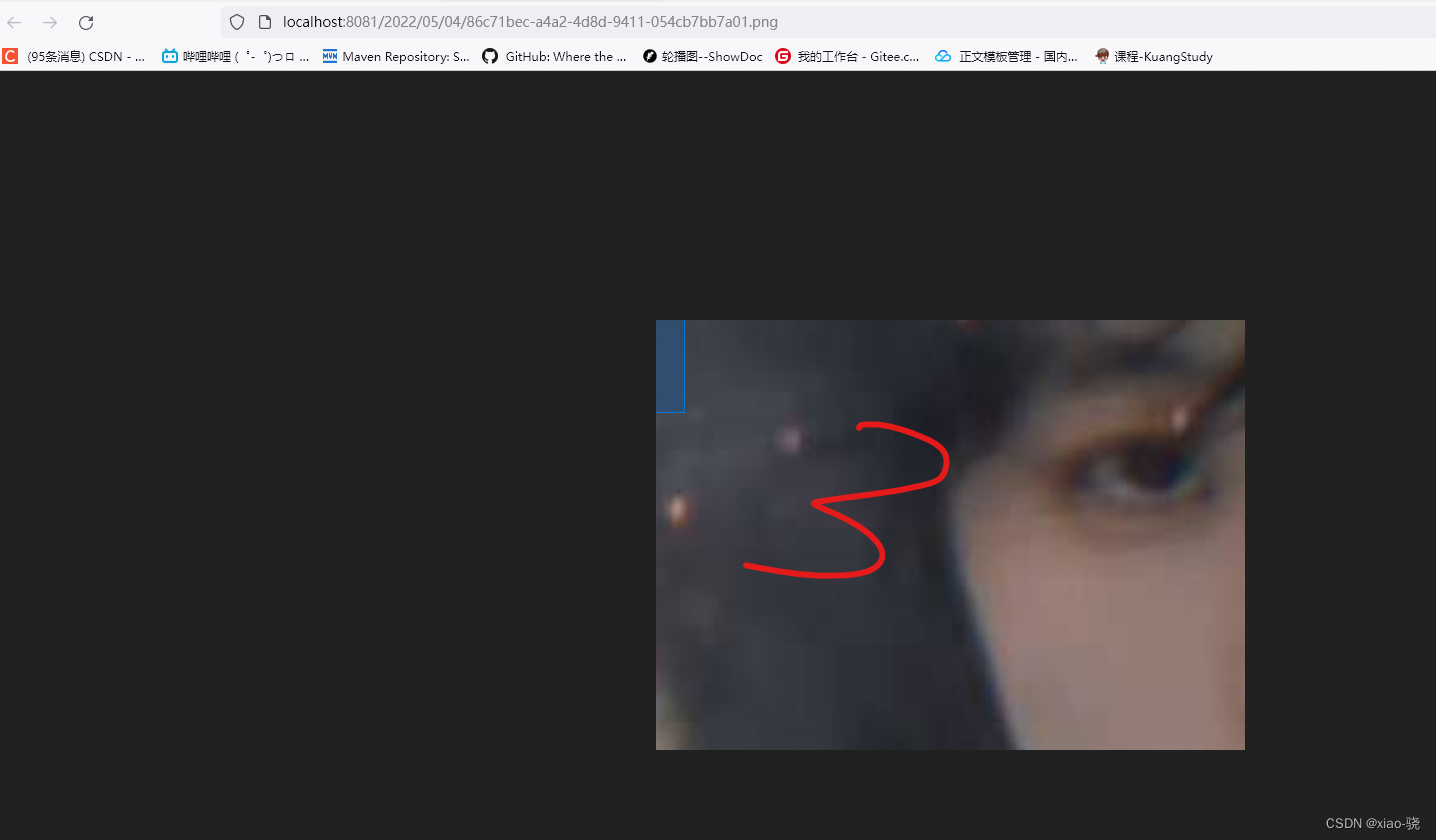
2.单文件上传到本地
@RequestMapping("/upload")
public String upload2(@RequestBody MultipartFile file, HttpServletRequest req) throws IOException {//MultipartFile 接收前端传过来的文件
String format = sdf.format(new Date());
String path="D:/img"+format;
File folder=new File(path);
if (!folder.exists()){
folder.mkdirs();
}
String oldName = file.getOriginalFilename();
String newName= UUID.randomUUID().toString()+oldName.substring(oldName.lastIndexOf("."));
file.transferTo(new File(path+newName));
return path+ newName;
}
多文件上传
1.一次性选择多个文件
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="/uploads2" method="post" enctype="multipart/form-data">
<input type="file" name="files" multiple>
<input type="submit" value="提交">
</form>
</body>
</html>
?updaloadController
SimpleDateFormat sdf=new SimpleDateFormat("/yyyy/MM/dd/");
@RequestMapping("/uploads2")
public String upliad(MultipartFile[] files, HttpServletRequest req){
//获取临时目录
String realpath=req.getServletContext().getRealPath("/");
//最终路径
String format = sdf.format(new Date());
String path=realpath+ format;
File folder=new File(path);
if (!folder.exists()){
folder.mkdirs();
}
try {
for (MultipartFile file:files){
String oldName = file.getOriginalFilename();
String newName= UUID.randomUUID().toString()+oldName.substring(oldName.lastIndexOf("."));
file.transferTo(new File(path+newName));
String newName1 =req.getScheme()+"://"+req.getServerName()+":"+req.getServerPort()+req.getContextPath()+format+ newName;
System.out.println(newName1);
}
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
?2.选择多次
index.html
SimpleDateFormat sdf=new SimpleDateFormat("/yyyy/MM/dd/");
@RequestMapping("/uploads3")
public String upliad(MultipartFile files1,MultipartFile files2, HttpServletRequest req){
//获取临时目录
String realpath=req.getServletContext().getRealPath("/");
//最终路径
String format = sdf.format(new Date());
String path=realpath+ format;
File folder=new File(path);
if (!folder.exists()){
folder.mkdirs();
}
try {
String oldName = files1.getOriginalFilename();
String newName= UUID.randomUUID().toString()+oldName.substring(oldName.lastIndexOf("."));
files1.transferTo(new File(path+newName));
String s1 =req.getScheme()+"://"+req.getServerName()+":"+req.getServerPort()+req.getContextPath()+format+ newName;
System.out.println(s1);
String oldName2 = files2.getOriginalFilename();
String newName2= UUID.randomUUID().toString()+oldName2.substring(oldName2.lastIndexOf("."));
files2.transferTo(new File(path+newName2));
String s2 =req.getScheme()+"://"+req.getServerName()+":"+req.getServerPort()+req.getContextPath()+format+ newName2;
System.out.println(s2);
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
?updaloadController
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="/uploads3" method="post" enctype="multipart/form-data">
<input type="file" name="files1">
<input type="file" name="files2">
<input type="submit" value="提交">
</form>
</body>
</html>
ajax上传
ajax.html
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://code.jquery.com/jquery-3.6.0.js" integrity="sha256-H+K7U5CnXl1h5ywQfKtSj8PCmoN9aaq30gDh27Xc0jk=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="result"></div>
<input type="file" id="file">
<input type="button" value="提交" onclick="uploadFile()">
<script>
function uploadFile(){
var file =$("#file")[0].files[0];
var formData = new FormData();
formData.append("file", file);
$.ajax({
type:"post",
url:"/upload",
processData:false,
contentType:false,
data:formData,
success:function (msg){
$("#result").html(msg);
}
});
}
</script>
</body>
</html>
uploadController
SimpleDateFormat sdf=new SimpleDateFormat("/yyyy/MM/dd/");
@RequestMapping("/upload")
public String upliad(MultipartFile file, HttpServletRequest req){
//获取临时目录
String realpath=req.getServletContext().getRealPath("/");
//最终路径
String format = sdf.format(new Date());
String path=realpath+ format;
File folder=new File(path);
if (!folder.exists()){
folder.mkdirs();
}
String oldName = file.getOriginalFilename();
String newName= UUID.randomUUID().toString()+oldName.substring(oldName.lastIndexOf("."));
try {
file.transferTo(new File(path+newName));
String newName1 =req.getScheme()+"://"+req.getServerName()+":"+req.getServerPort()+req.getContextPath()+format+ newName;
return newName1;
// return path;
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
|