?方法引用::其实就是lambda表达式的化简!!! 必须借助接口! ?按照我所引用的方法来实现这个接口中的唯一方法,谁调用它我不管,我只需要实现就行
? 一、引用类中的静态方法
?格式: 类名::静态方法。方法引用中的静态方法所接收的参数就是一个接口中唯一方法中的参数。 ?只需要保证这个静态方法和所对应的接口方法参数和返回值一致就可以使用! ?形如:
printTest( (s)-> System.out.println(s));
printTest(System.out::println);
?示例1: 传入一个字符串输出
?所需接口:
public interface PrintInterface{
void print(String s);
}
public class Test1 {
public static void main(String[] args) {
printTest( (s)-> System.out.println(s));
printTest( System.out::println );
}
public static void printTest(PrintInterface p){
p.print("Hello World");
}
}
?示例2: 传入两个字符串输出 ?所用到的接口:
public interface Printable {
void myPrint(String s ,String s1);
}
?测试代码:
public class Test5 {
public static void main(String[] args) {
userPrint( (s1,s2)-> newPrint(s1,s2) );
userPrint( Test::newPrint );
}
public static void newPrint(String s , String s1){
System.out.println(s+s1);
}
public static void userPrint(Printable p){
p.myPrint("蚌埠住了!","方法引用!");
}
}
?二、引用类中的构造方法
?格式: 类名::new 。 ?形如:
Student s = testNewStu( (id,name)->new Student(id,name) );
Student s1 = testNewStu(Student::new);
?直接看例子 ?示例: 实例化Student类 ?所用到的接口:
public interface Stuable {
Student newStu(int id ,String name);
}
?测试函数
public class Test3 {
public static void main(String[] args) {
Student s = testNewStu( (id,name)->new Student(id,name) );
Student s1 = testNewStu(Student::new);
System.out.println(s);
}
public static Student testNewStu(Stuable s){
Student stu1 = s.newStu(1,"TTT");
return stu1;
}
}
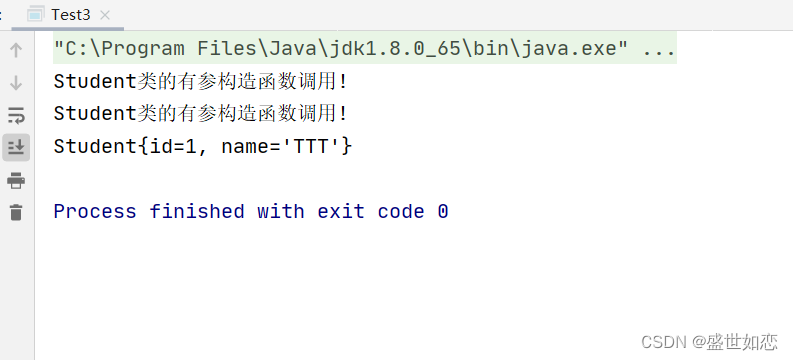
?三、引用类的成员方法
?跟上述的大同小异,唯一区别的是,如果引用类的成员方法,则需要传入调用这个成员方法的对象。也就是说接口方法第一个参数是类对象,其他参数是该成员方法的参数! ?形如:
StringTest( (s,index)->s.charAt(index) );
StringTest( String::charAt );
?示例: 调用String方法中的charAt()方法,来获取某字符
public interface Stringable {
char getChar(String s , int index);
}
?主函数:
public class Test4 {
public static void main(String[] args) {
StringTest( (s,index)->s.charAt(index) );
StringTest( String::charAt );
}
public static void StringTest(Stringable s){
char t = s.getChar("HelloWorld!",7);
System.out.println(t);
}
}
?四、引用类对象的成员方法
?格式: 类对象名::成员方法 。跟一差不多,就是变成了对象中的成员方法来引用。 ?这个在Stream流中非常常见。 当引用的方法是没有参数传递时,第一个参数就是该对象调用了该对象的无参数传入的方法。 ?形如:
Test t = new Test();
userPrint( (s1,s2)-> t.new1Print(s1,s2) );
userPrint( t::new1Print);
?示例: 修改以下一的示例代码 ?接口
public interface Printable {
void myPrint(String s ,String s1);
}
?主函数:
public class Test6 {
public static void main(String[] args) {
Test t = new Test();
userPrint( (s1,s2)-> t.new1Print(s1,s2) );
userPrint( t::new1Print);
}
public static void userPrint(Printable p){
p.myPrint("蚌埠住了!","方法引用!");
}
public void new1Print(String s , String s1){
System.out.println(s+s1);
}
}
|