1.根据xml 方式整合
pom.xml
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.5.4</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.35</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.16</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.5</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.5</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>5.2.0</version>
</dependency>
<!-- 数据库连接池使用druid-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.1</version>
</dependency>
</dependencies>
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd">
<bean id="datasource" class="com.alibaba.druid.pool.DruidDataSource" init-method="init"
destroy-method="close">
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/user_test?useUnicode=true&useSSL=false&characterEncoding=utf-8&serverTimezone=Asia/Shanghai"></property>
<property name="username" value="root"></property>
<property name="password" value="root"></property>
</bean>
<bean id="dataSourceTransactionManager"
class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="datasource"></property>
</bean>
<!--使用注解配置事务-->
<tx:annotation-driven transaction-manager="dataSourceTransactionManager"></tx:annotation-driven>
<context:annotation-config></context:annotation-config>
<context:component-scan base-package="com.entor"></context:component-scan>
<!-- 配置mybatis会话工厂 mybatisConfiguration.xml -->
<bean id="sessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="datasource"></property>
<!-- 加载orm映射文件mappers -> mapper -->
<property name="mapperLocations" value="classpath:mappers/*.xml"></property>
<property name="typeAliasesPackage" value="com.entor.entity"></property>
<property name="configuration">
<bean class="org.apache.ibatis.session.Configuration">
<property name="mapUnderscoreToCamelCase" value="true"></property>
<property name="logImpl" value="org.apache.ibatis.logging.stdout.StdOutImpl"></property>
<property name="cacheEnabled" value="true"></property>
</bean>
</property>
<property name="plugins">
<array>
<bean class="com.github.pagehelper.PageInterceptor"></bean>
</array>
</property>
</bean>
<!-- 自动扫描指定包下的接口,为接口创建实现类对象 session.getMapper(xxx.class)-->
<bean id="scannerConfigurer" class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<!-- 指定接口包名-->
<property name="basePackage" value="com.entor.mappers"></property>
<!-- 配置会话工厂,系统中存在单个工厂不用配置-->
<property name="sqlSessionFactoryBeanName" value="sessionFactory"></property>
</bean>
<!-- mybatis配置类-->
</beans>
mybatis的映射类 xxxMapper.class
package com.entor.mappers;
import com.entor.entity.User;
import org.apache.ibatis.annotations.*;
import java.util.List;
@CacheNamespace
public interface UserMapper {
public abstract int add(User user);
public abstract int addMore(List<User> list);
public abstract int update(User user);
@Delete("delete from emp where id = #{id}")
public abstract int deleteById(int id);
@Delete("delete from emp where id in (${ids})")
public abstract int deleteByIds(String ids);
@Select("select id,name,sex,phone,email,entry_date,create_time,password from emp where id = #{id}")
public abstract User queryById(int id);
@Select("select id,name,sex,phone,email,entry_date," +
"create_time,password from emp limit #{pageNum},#{pageSize}")
public abstract List<User> queryByPage
(@Param("pageNum") int pageNo, @Param("pageSize") int pageSize);
public abstract User login(String name,String password);
@Select("select count(id) from emp")
public abstract int getCount();
@Select("select * from emp")
public abstract List<User> queryAll();
public abstract List<User> queryByParam(User user);
}
mybatis 映射文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.entor.mappers.UserMapper">
<sql id="common">
id,name,sex,phone,email,entry_date,create_time,password
</sql>
<sql id="common2">
name,sex,phone,email,entry_date,create_time,password
</sql>
<insert id="add">
insert into emp (
<if test="name!=null and name !=''">
name,
</if>
<if test="sex!=null and sex !=''">
sex,
</if>
<if test="phone!=null and phone !=''">
phone,
</if>
<if test="email!=null and email !=''">
email,
</if>
<if test="entry_date!=null ">
entry_date,
</if>
<if test="create_time!=null">
create_time,
</if>
<if test="password!=null and password !=''">
password
</if>
)
values (
<if test="name!=null and name !=''">
#{name},
</if>
<if test="sex!=null and sex !=''">
#{sex},
</if>
<if test="phone!=null and phone !=''">
#{phone},
</if>
<if test="email!=null and email !=''">
#{email},
</if>
<if test="entry_date!=null">
#{entry_date},
</if>
<if test="create_time!=null ">
#{create_time},
</if>
<if test="password!=null and password !=''">
#{password}
</if>
)
</insert>
<insert id="addMore" useGeneratedKeys="true" keyColumn="id" keyProperty="id">
insert into emp(<include refid="common2"/>)values
<foreach collection="list" separator="," item="user">
(#{user.name},
#{user.sex},
#{user.phone},
#{user.email},
#{user.entry_date},
#{user.create_time},
#{user.password})
</foreach>
</insert>
<update id="update">
update emp
<set>
<if test="name!=null and name !=''">
name=#{name},
</if>
<if test="sex!=null and sex !=''">
sex=#{sex},
</if>
<if test="phone!=null and phone !=''">
phone=#{phone},
</if>
<if test="email!=null and email !=''">
email=#{email},
</if>
<if test="entry_date!=null">
entry_date=#{entry_date},
</if>
<if test="create_time!=null ">
create_time=#{create_time},
</if>
<if test="password!=null and password !=''">
password=#{password}
</if>
</set>
where id = #{id}
</update>
<select id="queryByParam" resultType="User">
select <include refid="common"/> from emp
<where>
<if test="name!=null and name!=''">
name like concat('%' ,#{name},'%')
</if>
<if test="sex!=null">
and sex = #{sex}
</if>
<if test="phone!=null and phone!=''">
and phone like concat('%' ,#{phone},'%')
</if>
</where>
</select>
</mapper>
测试test
ClassPathXmlApplicationContext cal = new ClassPathXmlApplicationContext("/applicationContext.xml");
UserMapper bean = cal.getBean(UserMapper.class);
注解的方式mybatis集成spring主要有2种方式
2. 基于注解的方式
①mapper xml文件放在resource目录,和Mapper接口不在一个目录的情况
DROP DATABASE IF EXISTS `javacode2018`;
CREATE DATABASE `javacode2018`;
USE `javacode2018`;
DROP TABLE IF EXISTS `t_user`;
CREATE TABLE t_user (
id BIGINT AUTO_INCREMENT PRIMARY KEY COMMENT '主键,用户id,自动增长',
`name` VARCHAR(32) NOT NULL DEFAULT '' COMMENT '姓名'
) COMMENT '用户表';
SELECT * FROM t_user;
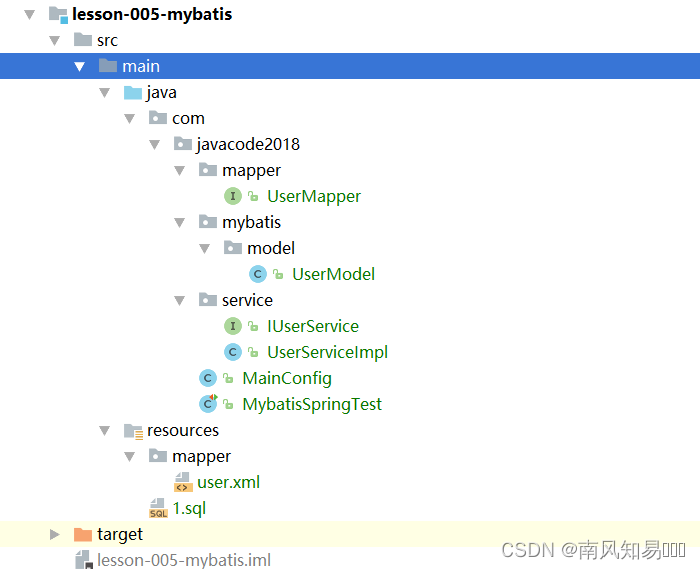
package com.javacode2018.mybatis.model;
import lombok.*;
@Getter
@Setter
@Builder
@NoArgsConstructor
@AllArgsConstructor
@ToString
public class UserModel {
private Long id;
private String name;
}
UserMapper
package com.javacode2018.mapper;
import com.javacode2018.mybatis.model.UserModel;
import org.apache.ibatis.annotations.Mapper;
import java.util.List;
@Mapper
public interface UserMapper {
void insert(UserModel userModel);
List<UserModel> getList();
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.javacode2018.mapper.UserMapper">
<insert id="insert" parameterType="com.javacode2018.mybatis.model.UserModel" keyProperty="id" useGeneratedKeys="true">
<CDATA[ INSERT INTO `t_user` (name) VALUES (#{name})]]>
</insert>
<select id="getList" resultType="com.javacode2018.mybatis.model.UserModel">
<CDATA[
SELECT id,name FROM t_user
]]>
</select>
</mapper>
IUserService
package com.javacode2018.service;
import com.javacode2018.mybatis.model.UserModel;
import java.util.List;
public interface IUserService {
UserModel insert(UserModel userModel);
List<UserModel> getList();
}
UserServiceImpl
package com.javacode2018.service;
import com.javacode2018.mapper.UserMapper;
import com.javacode2018.mybatis.model.UserModel;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
@Service
public class UserServiceImpl implements IUserService {
@Autowired
private UserMapper userMapper;
@Transactional(rollbackFor = Exception.class)
@Override
public UserModel insert(UserModel userModel) {
userMapper.insert(userModel);
return userModel;
}
@Transactional(propagation = Propagation.SUPPORTS, readOnly = true)
@Override
public List<UserModel> getList() {
return userMapper.getList();
}
}
spring配置类MainConfig
@EnableTransactionManagement
@ComponentScan
@Configuration
@MapperScan(basePackageClasses = {UserMapper.class}, annotationClass = Mapper.class)
public class MainConfig {
@Bean
public DataSource dataSource() {
org.apache.tomcat.jdbc.pool.DataSource dataSource = new org.apache.tomcat.jdbc.pool.DataSource();
dataSource.setDriverClassName("com.mysql.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/javacode2018?characterEncoding=UTF-8");
dataSource.setUsername("root");
dataSource.setPassword("root123");
dataSource.setInitialSize(5);
return dataSource;
}
@Bean
public TransactionManager transactionManager(DataSource dataSource) {
return new DataSourceTransactionManager(dataSource);
}
@Bean
public SqlSessionFactoryBean sqlSessionFactoryBean(DataSource dataSource) throws IOException {
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(dataSource);
Resource[] resources = new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/**/*.xml");
sqlSessionFactoryBean.setMapperLocations(resources);
return sqlSessionFactoryBean;
}
}
②方式2:mapper xml文件和Mapper接口在同一个目录 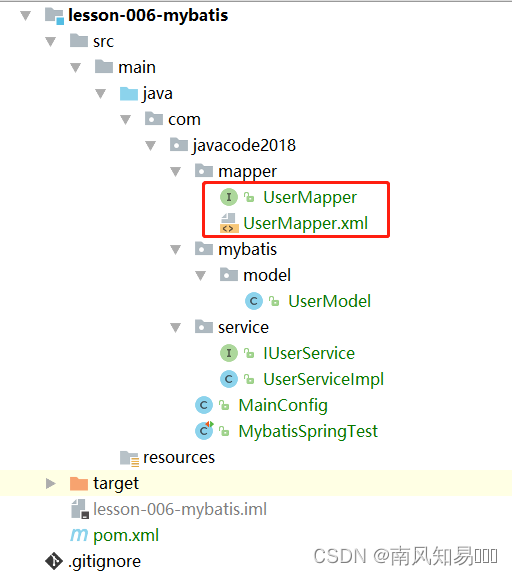 修改SqlSessionFactoryBean的定义:
需要修改MainConfig中SqlSessionFactoryBean的定义,如下,更简洁了,不需要在指定mapper xml的位置了,这里需要注意一点,方式2中将mapper xml文件和mapper接口放在一个目录的时候,这2个文件的名字必须一样,这样在定义SqlSessionFactoryBean的时候才不需要指定mapper xml的位置。
@Bean
public SqlSessionFactoryBean sqlSessionFactoryBean(DataSource dataSource) throws IOException {
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(dataSource);
return sqlSessionFactoryBean;
}
调整一下pom.xml配置
<build>
<resources>
<resource>
<directory>${project.basedir}/src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</resource>
<resource>
<directory>${project.basedir}/src/main/resources</directory>
<includes>
<include>**/*</include>
</includes>
</resource>
</resources>
</build>
|