- 新建springboot项目,版本选择2.6.7
- pom文件添加swagger,版本为2.9.2
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.7</version>
<relativePath/>
</parent>
<groupId>com.yf</groupId>
<artifactId>security-oauth2</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>security-oauth2</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.6.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.2.RELEASE</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.18</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
- 添加swagger配置类
package com.yf.securityoauth2.config;
import io.swagger.annotations.Api;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class Swagger2Configuration {
@Bean
public Docket createRestApi(){
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("com.yf.securityoauth2.controller"))
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo(){
return new ApiInfoBuilder()
.title("spring security测试的接口文档")
.description("用于描述spring security接口的详细文档")
.termsOfServiceUrl("http://www.baidu.com")
.version("1.0")
.build();
}
}
- 添加配置文件
server:
port: 8012
spring:
mvc:
pathmatch:
matching-strategy: ant_path_matcher
# 防止访问控制台时报参数类型转换异常
logging:
level:
io:
swagger:
models:
parameters:
AbstractSerializableParameter: error
- 注解用法
5.1@Api 用于标识类,表示该类是Swagger的资源 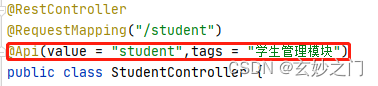 5.2@ApiIgnore 用于表示类,值忽略对该类的扫描 5.3@ApiOperation 用于方法,描述 Controller类中的 method接口 5.4@ApiParam: 用于参数,单个参数描述,与 @ApiImplicitParam不同的是,他是写在参数左侧的。如( @ApiParam(name=“username”,value=“用户名”)Stringusername) 5.5@ApiModel: 用于类,表示对类进行说明,用于参数用实体类接收 5.6@ApiProperty:用于方法,字段,表示对model属性的说明或者数据操作更改 5.7@ApiImplicitParam: 用于方法,表示单独的请求参数 5.8@ApiImplicitParams: 用于方法,包含多个 @ApiImplicitParam 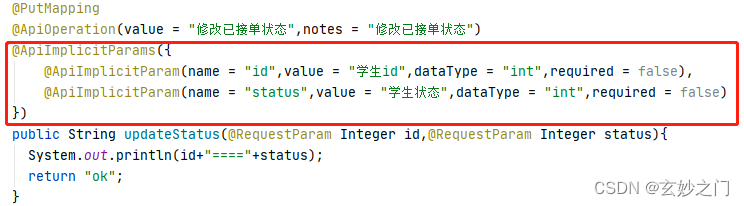
5.9@ApiResponse: 用于方法,描述单个出参信息 5.10@ApiResponses: 用于方法,包含多个@ApiResponse 5.10@ApiError: 用于方法,接口错误所返回的信息
|