创建项目
首先就是创建,创建SpringBoot工程方法很多。工具自动,人工手动。
工具经典方法:
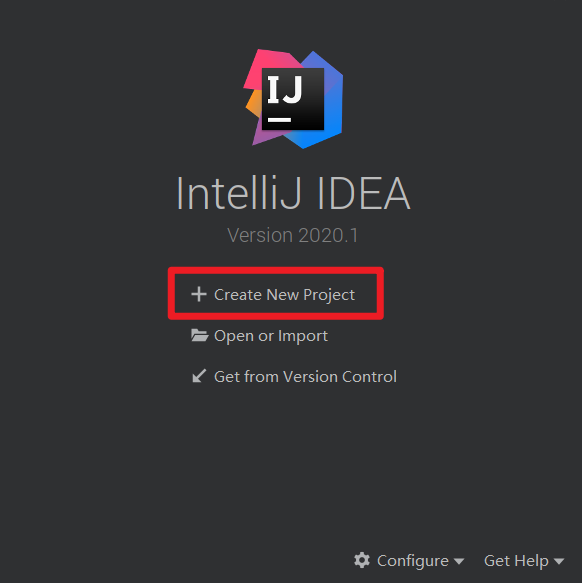
选择JDK时,建议1.8
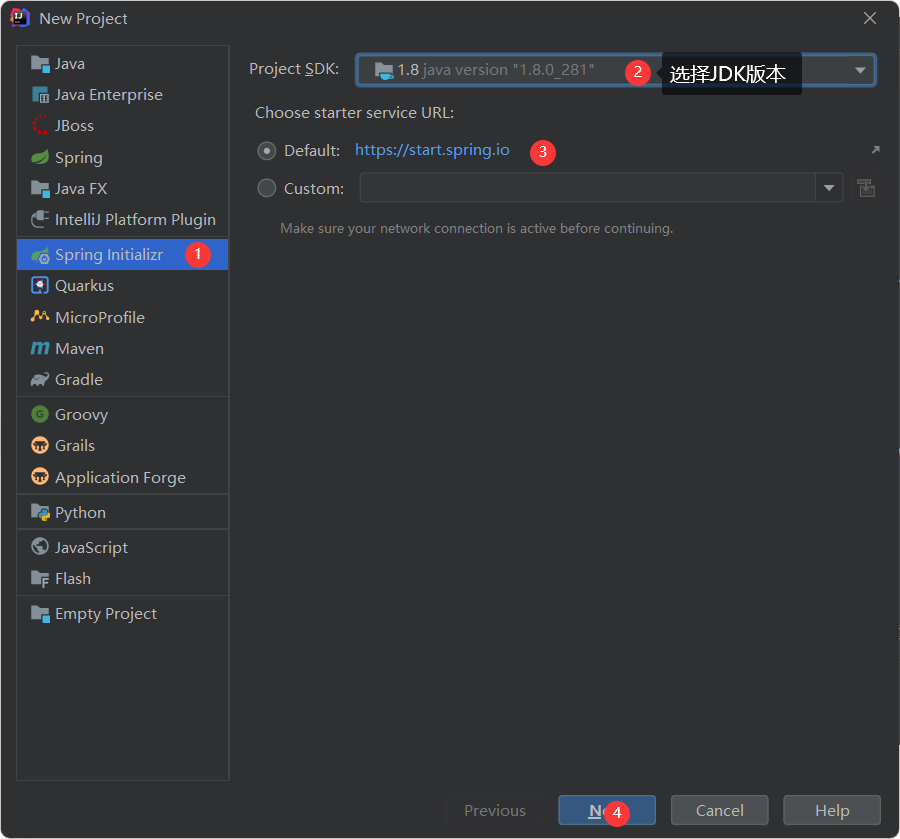
之后就是耐心等待,网好一次过,网卡请努力
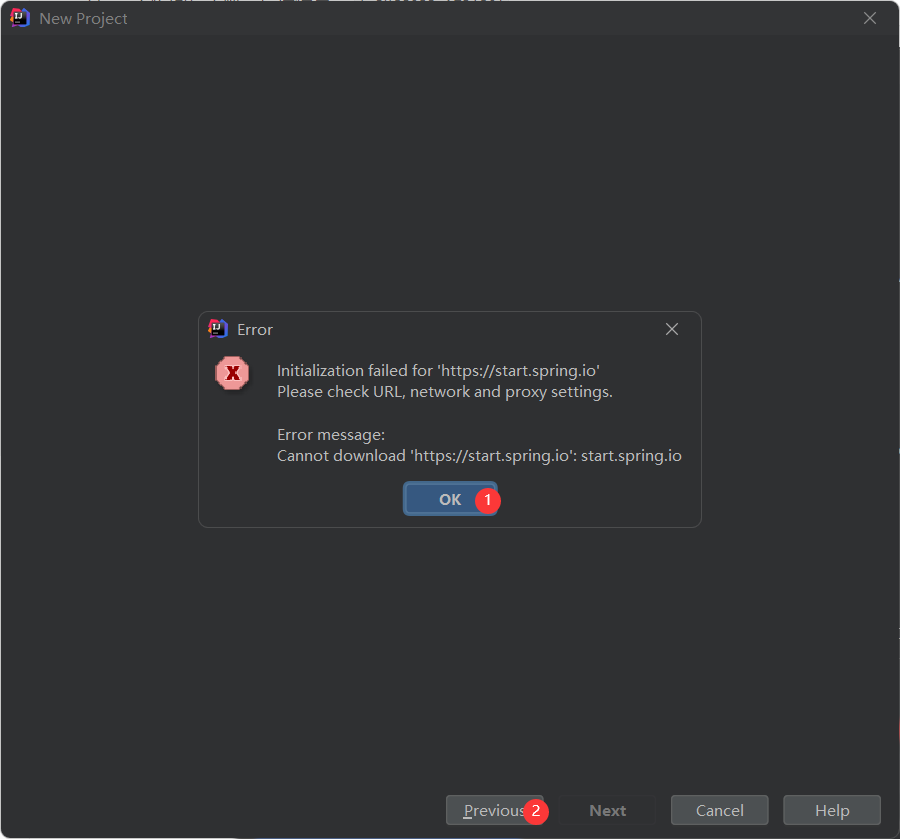
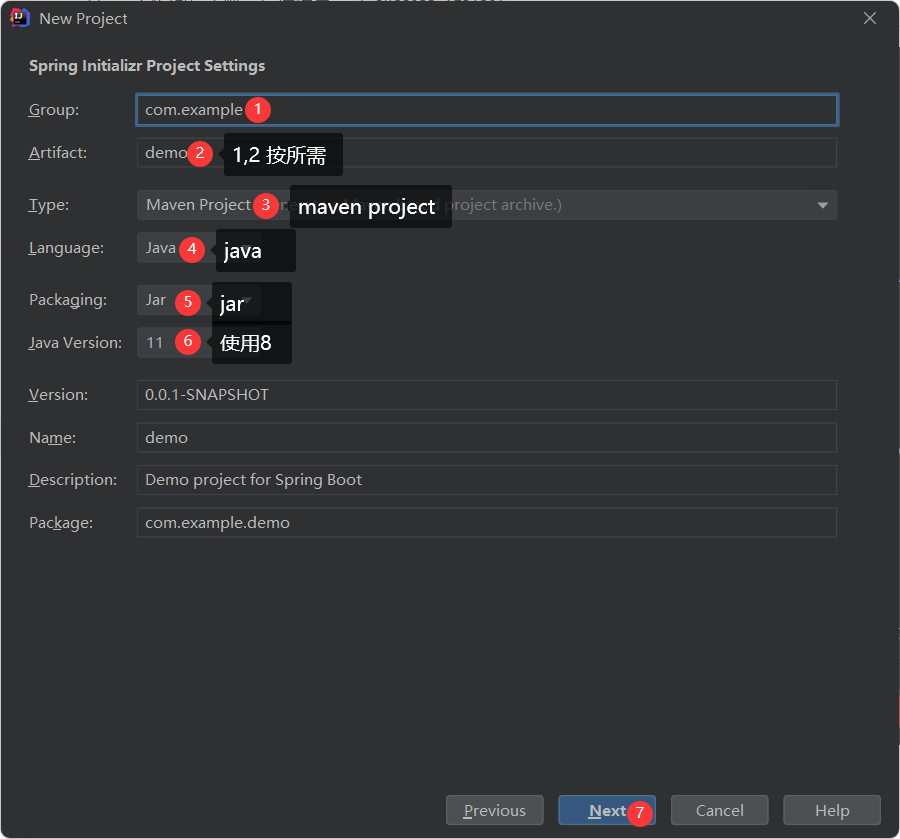
web工程
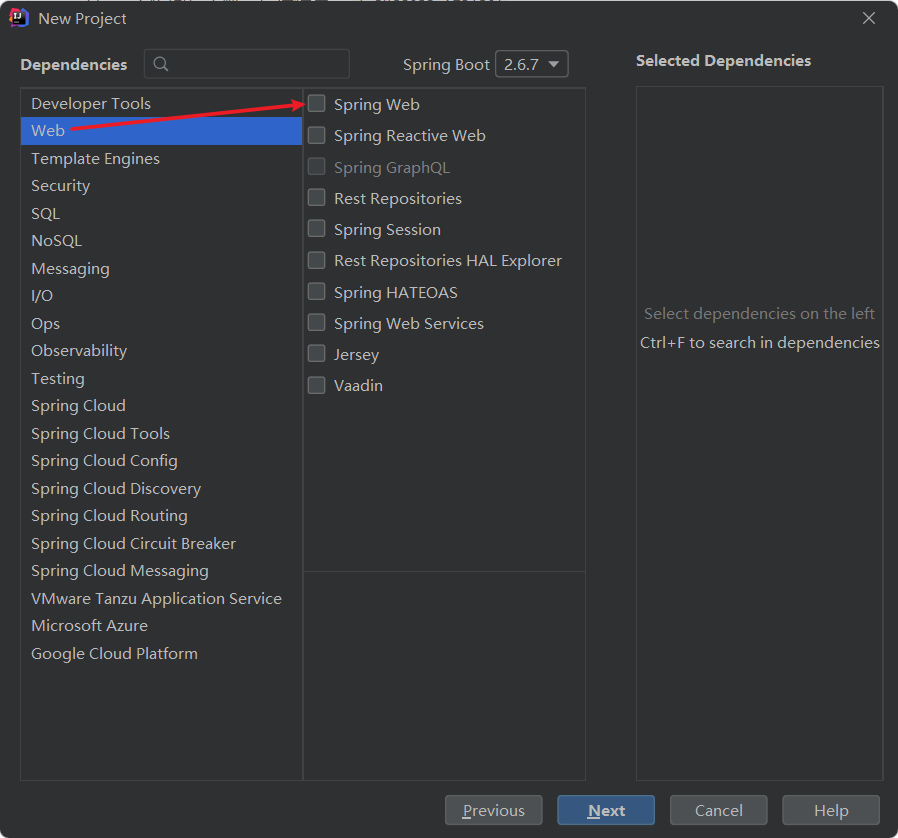
mybatis,如使用mybatis-plus手动pom
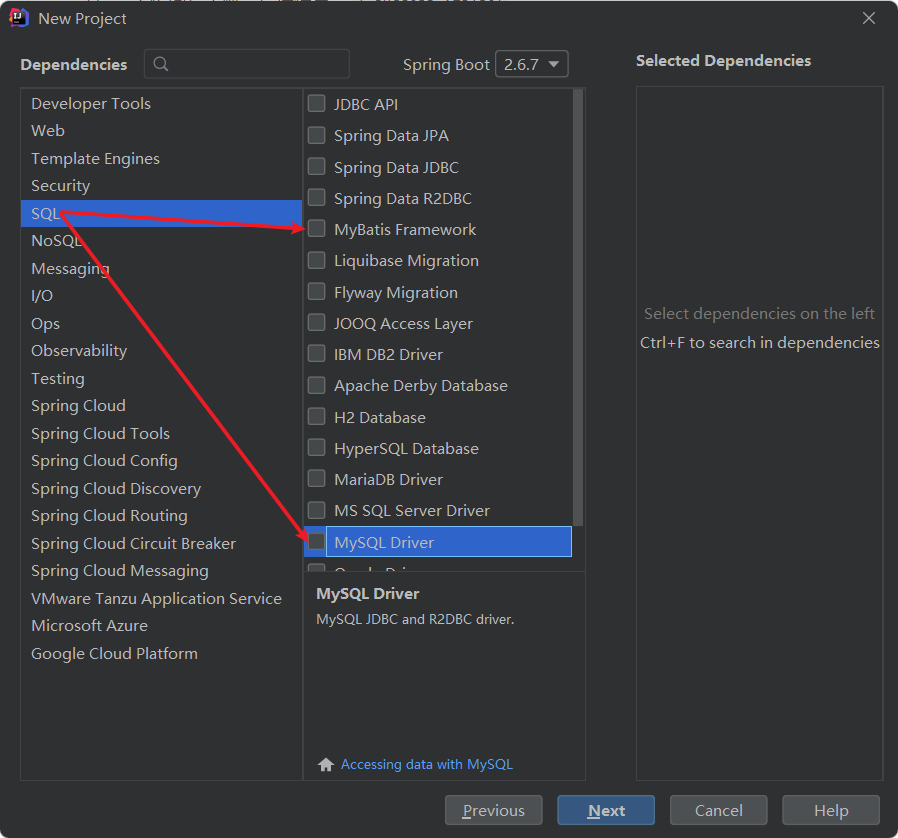
选择好所需,下一步
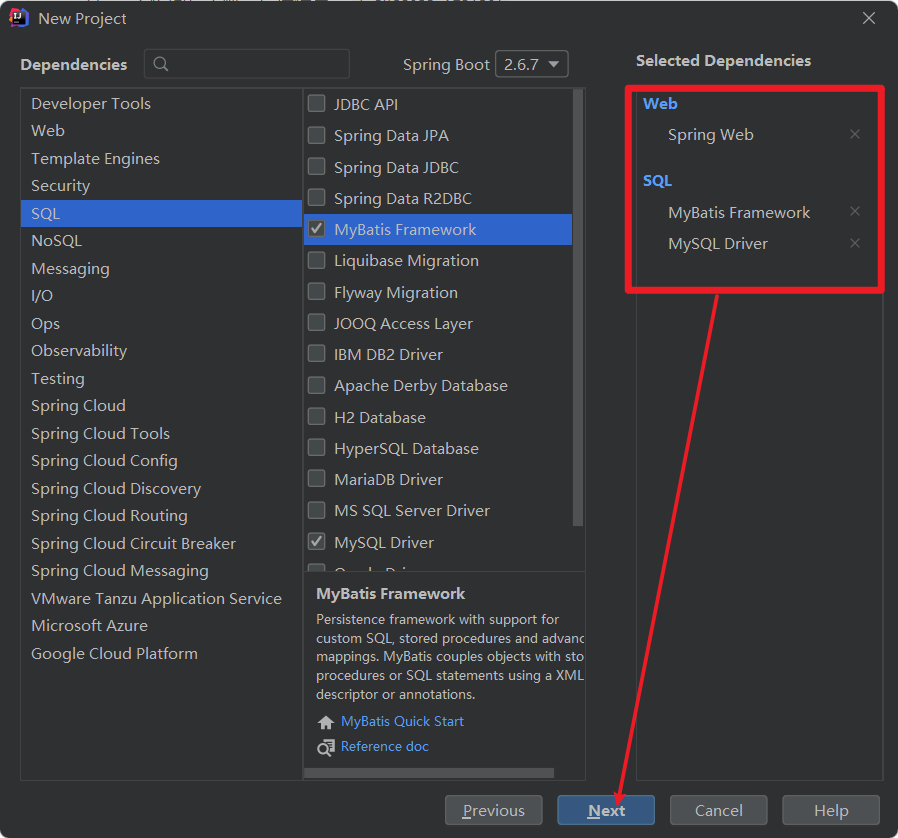
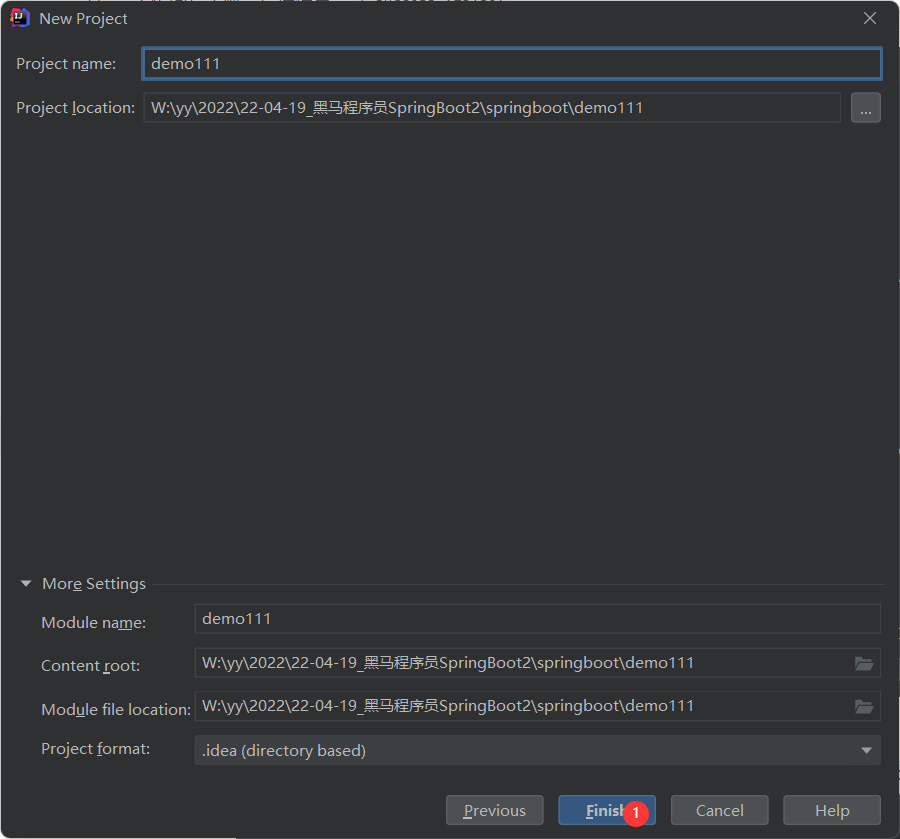
创建完成,刷新maven即可使用。
或者使用阿里,速度更快,但配置和项目结构有些不同。
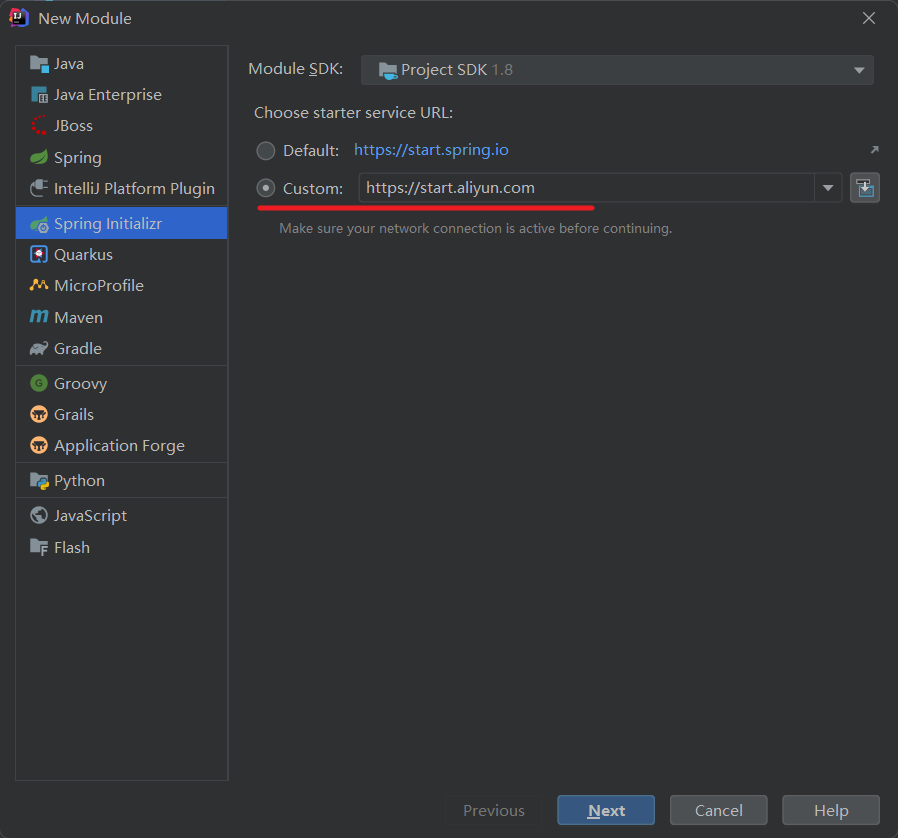
手动方式无非复制之前创建好的项目,修改名称,**注意pom中名称的修改,**然后刷新pom。
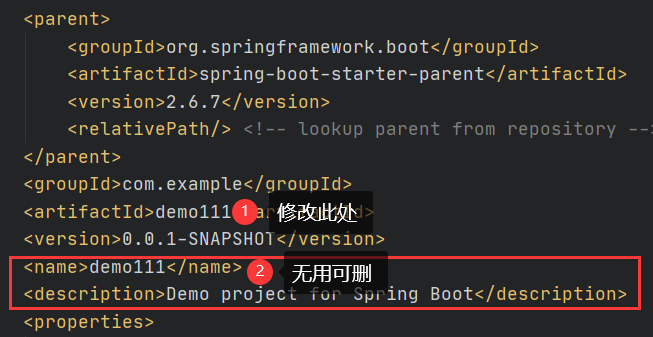
启动类出错
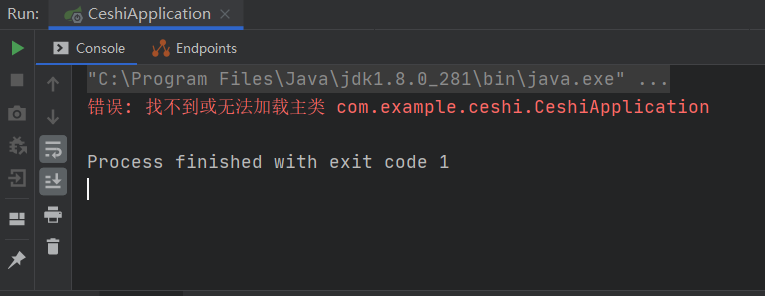
IDEA没有加载启动类?
打开启动类,等待spring管理
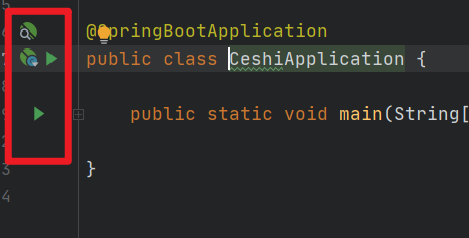
然后运行就好了
打包速度
关闭test
目前还是很慢,但有提升。
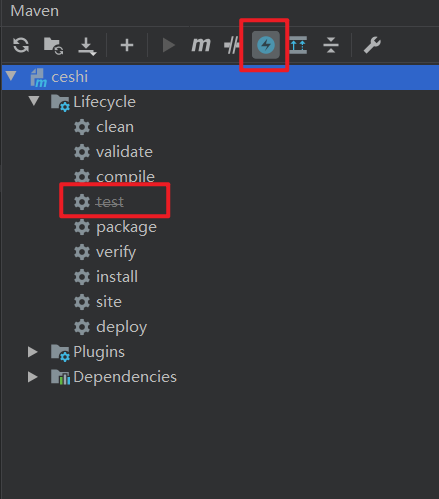
数据库配置
链接失败密码问题
测试多种组合
数字和特殊字符识别错误(进制和格式问题)
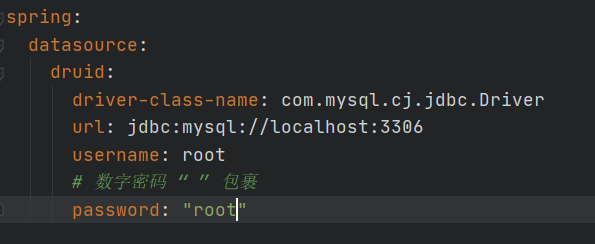
例子:
dataSource:
password: 9.
测试类
@SpringBootTest
class Springboot13ConfigurationApplicationTests {
@Value("${dataSource.password}")
private String password;
@Test
void contextLoads() {
System.out.println(password);
}
}

使用""包裹密码,解决问题
mybatis-plus
表的匹配和id自增
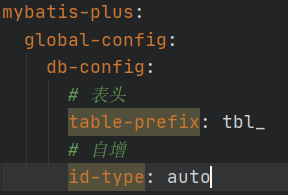
拦截器
配置分页拦截器
@Configuration
public class MPConfig {
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor(){
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
interceptor.addInnerInterceptor(new PaginationInnerInterceptor());
return interceptor;
}
}
日志记录
红框必备
level:后添加mapper包即可
使用mybatis修改第一行,删除-plus
file-name-pattern使用全路径
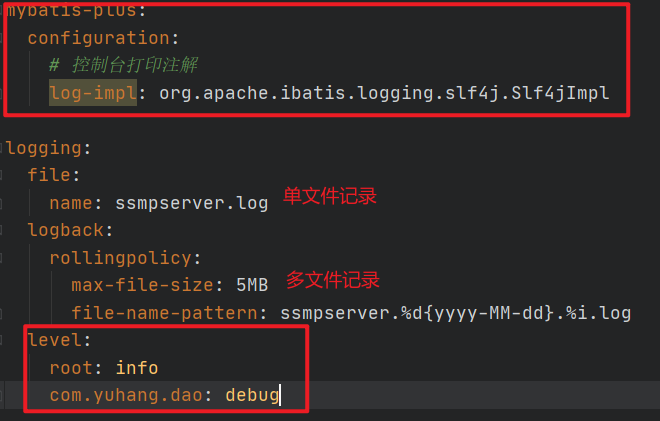
校验
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
</dependency>
实体类
@Validated
public class ServletConfig {
private String ipAddress;
@Max(value = 100,message = "最大值不该超过100")
private int port;
private long timeout;
}
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
</dependency>
设置最大值100的限制,实际101
servlet:
ipAddress: 192.168.0.1
port: 101
timeout: -1
超过提示
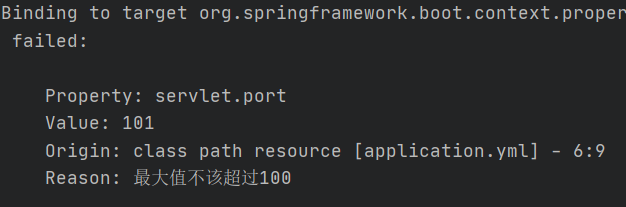
计量单位
实体类
@ConfigurationProperties(prefix = "servlet")
@Component
@Data
public class ServletConfig {
private String ipAddress;
private int port;
@DurationUnit(ChronoUnit.HOURS)
private Duration timeout;
}
servlet:
ipAddress: 192.168.0.1
port: 50
timeout: 10
启动类
ServletConfig bean = run.getBean(ServletConfig.class);
System.out.println(bean);

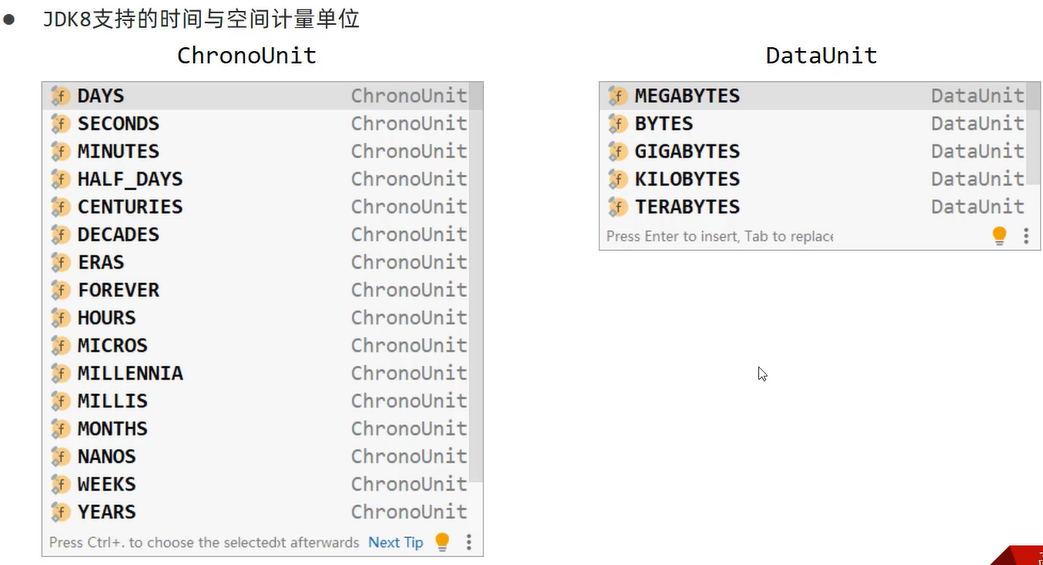
临时专用属性
测试时yml中无配置,如有配置注解优先
方法一:
@SpringBootTest(properties = {"test.prop = testValue1"})
public class PropertiesAndArgsTest {
@Value("${test.prop}")
private String prop;
@Test
void testProperties(){
System.out.println(prop);
}
}

方法二:
@SpringBootTest(args = {"--test.prop=testValue2"})
public class PropertiesAndArgsTest {
@Value("${test.prop}")
private String prop;
@Test
void testProperties(){
System.out.println(prop);
}
}

args优先级大于properties
临时专用配置
不使用配置类注解
public class MsgConfig {
@Bean
public String msg(){
return "textMsgConfig";
}
}
使用Import注解可以加载临时配置
@SpringBootTest
@Import(MsgConfig.class)
public class TestMsgConfig {
@Autowired
private String msg;
@Test
void testMsg(){
System.out.println(msg);
}
}
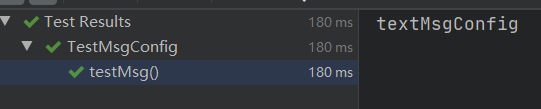
测试类
随机端口
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class TestWeb {
@Test
void test(){
}
}

发送虚拟请求
controller
@RestController
@RequestMapping("/books")
public class BookController {
@GetMapping
public String getById(){
System.out.println("getById...");
return "books";
}
}
test
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@AutoConfigureMockMvc
public class TestWeb {
@Autowired
private MockMvc mockMvc;
@Test
void test() throws Exception {
MockHttpServletRequestBuilder mockHttpServletRequestBuilder = MockMvcRequestBuilders.get("/books");
mockMvc.perform(mockHttpServletRequestBuilder);
}
}

设置预期值
状态预期
MockHttpServletRequestBuilder mockHttpServletRequestBuilder = MockMvcRequestBuilders.get("/books");
ResultActions perform = mockMvc.perform(mockHttpServletRequestBuilder);
StatusResultMatchers status = MockMvcResultMatchers.status();
ResultMatcher ok = status.isOk();
perform.andExpect(ok);
成功无输出
失败有提示
MockHttpServletRequest:
HTTP Method = GET
Request URI = /books1
Parameters = {}
Headers = []
Body = null
Session Attrs = {}
Handler:
Type = org.springframework.web.servlet.resource.ResourceHttpRequestHandler
Async:
Async started = false
Async result = null
Resolved Exception:
Type = null
ModelAndView:
View name = null
View = null
Model = null
FlashMap:
Attributes = null
MockHttpServletResponse:
Status = 404
Error message = null
Headers = [Vary:"Origin", "Access-Control-Request-Method", "Access-Control-Request-Headers"]
Content type = null
Body =
Forwarded URL = null
Redirected URL = null
Cookies = []
java.lang.AssertionError: Status expected:<200> but was:<404>
Expected :200
Actual :404
<Click to see difference>
内容请求体预期
和controller中return比较
@Test
void test2() throws Exception {
MockHttpServletRequestBuilder mockHttpServletRequestBuilder = MockMvcRequestBuilders.get("/books");
ResultActions perform = mockMvc.perform(mockHttpServletRequestBuilder);
ContentResultMatchers content = MockMvcResultMatchers.content();
ResultMatcher ok = content.string("book");
perform.andExpect(ok);
}
成功无输出
失败有提示
MockHttpServletRequest:
HTTP Method = GET
Request URI = /books
Parameters = {}
Headers = []
Body = null
Session Attrs = {}
Handler:
Type = com.yuhang.controller.BookController
Method = com.yuhang.controller.BookController#getById()
Async:
Async started = false
Async result = null
Resolved Exception:
Type = null
ModelAndView:
View name = null
View = null
Model = null
FlashMap:
Attributes = null
MockHttpServletResponse:
Status = 200
Error message = null
Headers = [Content-Type:"text/plain;charset=UTF-8", Content-Length:"5"]
Content type = text/plain;charset=UTF-8
Body = books
Forwarded URL = null
Redirected URL = null
Cookies = []
java.lang.AssertionError: Response content expected:<book> but was:<books>
Expected :book
Actual :books
<Click to see difference>
JSON预期
实体类
@Data
public class Book {
private Integer id;
private String name;
private Integer money;
}
controller
@RestController
@RequestMapping("/books")
public class BookController {
@GetMapping
public Book getById(){
System.out.println("getById...");
Book book = new Book();
book.setId(1);
book.setName("xiyou");
book.setMoney(100);
return book;
}
}
test
@Test
void testJson() throws Exception {
MockHttpServletRequestBuilder mockHttpServletRequestBuilder = MockMvcRequestBuilders.get("/books");
ResultActions perform = mockMvc.perform(mockHttpServletRequestBuilder);
ContentResultMatchers content = MockMvcResultMatchers.content();
ResultMatcher ok = content.json("{\"id\":1,\"name\":\"xiyo\",\"money\":10}");
perform.andExpect(ok);
}
成功无输出
失败有提示
MockHttpServletRequest:
HTTP Method = GET
Request URI = /books
Parameters = {}
Headers = []
Body = null
Session Attrs = {}
Handler:
Type = com.yuhang.controller.BookController
Method = com.yuhang.controller.BookController#getById()
Async:
Async started = false
Async result = null
Resolved Exception:
Type = null
ModelAndView:
View name = null
View = null
Model = null
FlashMap:
Attributes = null
MockHttpServletResponse:
Status = 200
Error message = null
Headers = [Content-Type:"application/json"]
Content type = application/json
Body = {"id":1,"name":"xiyou","money":100}
Forwarded URL = null
Redirected URL = null
Cookies = []
java.lang.AssertionError: money
Expected: 10
got: 100
; name
Expected: xiyo
got: xiyou
<Click to see difference>
以上可以写一起一起匹配
事务回滚
需要提交事务加@Rollback(false)
@SpringBootTest
@Transactional
@Rollback(false)
public class TestMP {
@Autowired
private BookService bookService;
@Test
void save(){
Book book = new Book();
book.setName("xiyou");
book.setMoney(100);
bookService.save(book);
}
}
配置文件随机值
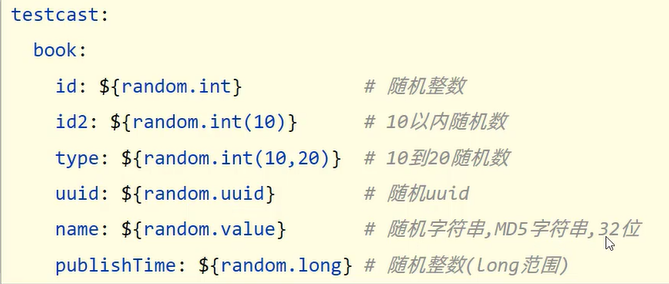
数据层技术选型
数据源
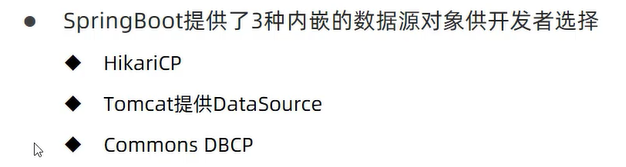
不配置DataSource默认HikariCP
spring:
h2:
console:
enabled: true
path: /h2
datasource:
url: jdbc:h2:~/test
username: sa
password: 123456
持久化
内置持久化解决方案——JdbcTemplate
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
@SpringBootTest
public class TestJdbc {
@Test void testGetAll(@Autowired JdbcTemplate jdbcTemplate){
String sql = "select * from tbl_book";
RowMapper<Book> rm = new RowMapper<Book>() {
@Override
public Book mapRow(ResultSet rs, int rowNum) throws SQLException {
Book book = new Book();
book.setId(rs.getInt("id"));
book.setName(rs.getString("name"));
book.setMoney(rs.getInt("money"));
return book;
}
};
List<Book> bookList = jdbcTemplate.query(sql, rm);
System.out.println(bookList);
}
@Test void testJdbc(@Autowired JdbcTemplate jdbcTemplate){
String sql = "insert into tbl_book values (3,'jdbch3',10)";
jdbcTemplate.update(sql);
}
数据库
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
spring:
h2:
console:
enabled: true
path: /h2
datasource:
url: jdbc:h2:~/test
username: sa
password: 123456
网页输入http://localhost:8080/h2登录
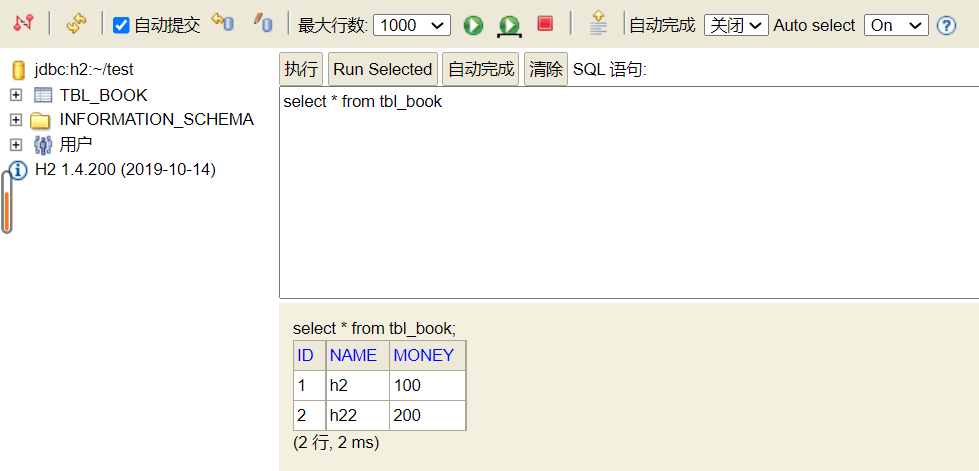
Redis
保证Redis启动
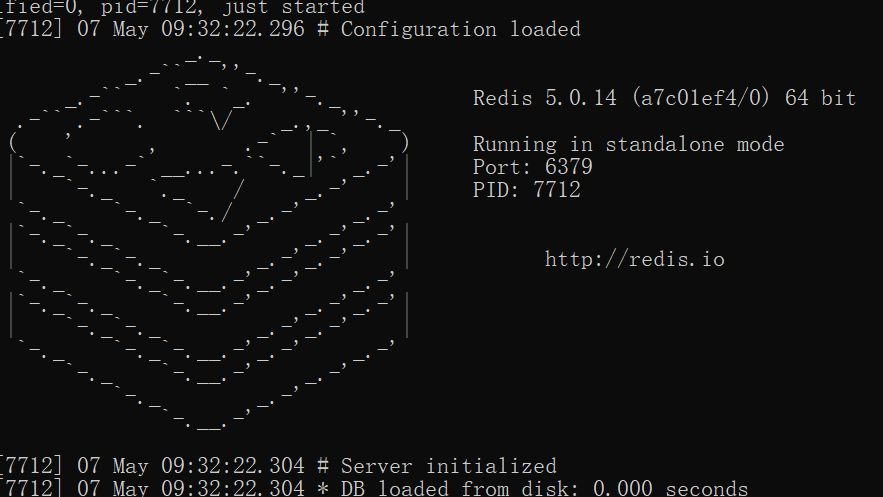
创建springboot工程
勾选如下,下一步
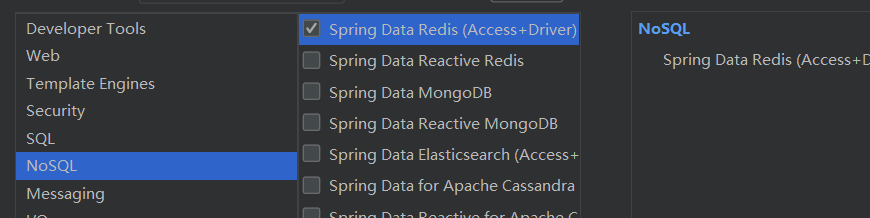
yml
spring:
redis:
host: localhost
port: 6379
选类型操作
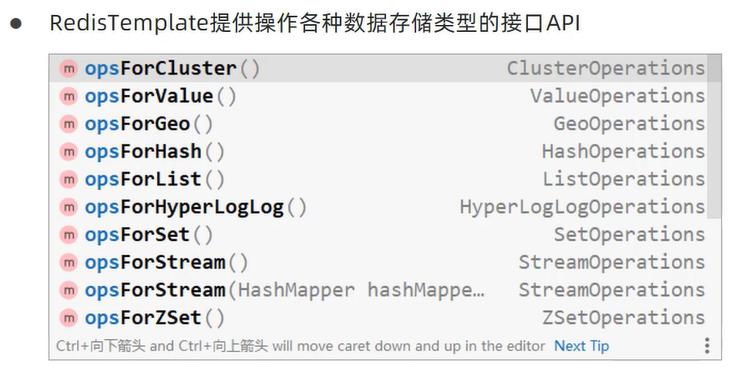
test
@SpringBootTest
class Springboot16RedisApplicationTests {
@Autowired
private RedisTemplate redisTemplate;
@Test
void set() {
ValueOperations valueOperations = redisTemplate.opsForValue();
valueOperations.set("name","yuhang");
}
@Test
void get() {
ValueOperations valueOperations = redisTemplate.opsForValue();
Object name = valueOperations.get("name");
System.out.println(name);
}
@Test
void hset() {
HashOperations hashOperations = redisTemplate.opsForHash();
hashOperations.put("hash","hh","aa");
}
@Test
void hget() {
HashOperations hashOperations = redisTemplate.opsForHash();
Object o = hashOperations.get("hash", "hh");
System.out.println(o);
}
}
如需使用cmd中添加的
使用StringRedisTemplate
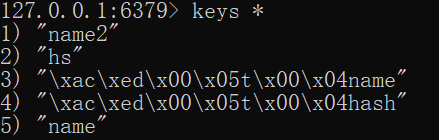

@Test
void get2() {
ValueOperations valueOperations = redisTemplate.opsForValue();
Object name = valueOperations.get("name2");
System.out.println(name);
}
@Test void stringTest(@Autowired StringRedisTemplate stringRedisTemplate){
ValueOperations<String, String> valueOperations = stringRedisTemplate.opsForValue();
String s = valueOperations.get("name2");
System.out.println(s);
}
使用jedis,默认lettuce
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
spring:
redis:
host: localhost
port: 6379
client-type: jedis

MongoDB
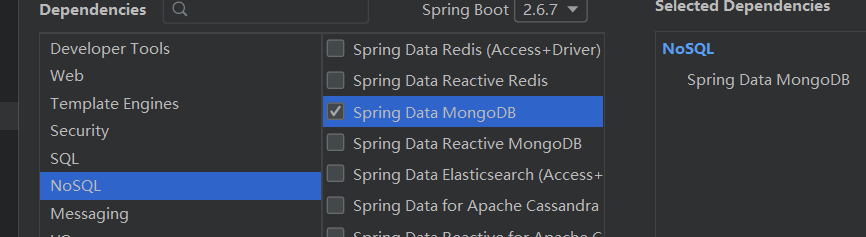
|