新建项目cros01,web依赖
package com.example.cors01;
import org.springframework.web.bind.annotation.*;
@RestController
//@CrossOrigin(origins = "http://localhost:8081")
//maxAge: 设置以秒为单位的缓存时间
//origins: 设置允许跨域的域名,可以设置多个
//allowCredentials: 设置是否允许发送Cookie
//allowedHeaders: 设置允许跨域的Header
//exposedHeaders: 设置跨域时允许返回的Header
//methods: 设置允许跨域的请求方法
public class HelloController {
@GetMapping("/hello")
public String hello(){
return "hello cros";
}
@PostMapping("/hello")
public String helloPost(){
return "hello cros";
}
}
新建项目cros02
01.html
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://code.jquery.com/jquery-3.6.0.js" integrity="sha256-H+K7U5CnXl1h5ywQfKtSj8PCmoN9aaq30gDh27Xc0jk=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<input type="button" onclick="getData()" value="get">
<input type="button" onclick="putData()" value="put">
<script>
function getData(){
$.get("http://localhost:8080/hello",function (msg) {
alert(msg);
})
}
function putData(){
$.ajax({
url:"http://localhost:8080/hello",
type:"post",
success:function (msg) {
alert(msg);
}
})
}
</script>
</body>
</html>
将cros2的运行端口变成8081
运行之后:点击get按钮,出现错误,跨域问题
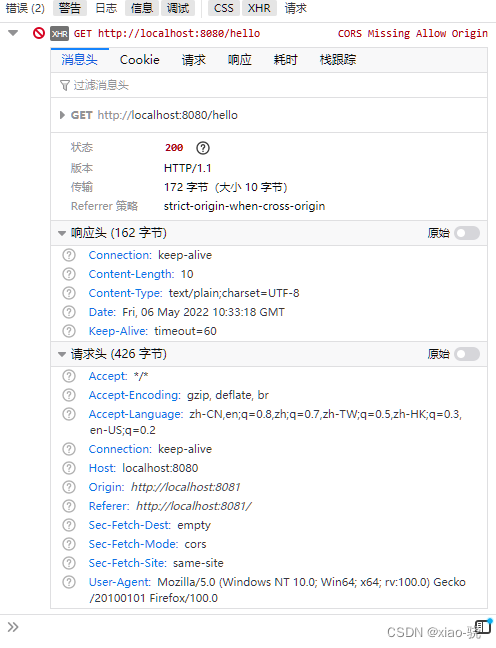
?解决跨域问题有3种方法
1.在方法或者类上面加注解@CrossOrigin
package com.example.cors01;
import org.springframework.web.bind.annotation.*;
@RestController
@CrossOrigin(origins = "http://localhost:8081")
//maxAge: 设置以秒为单位的缓存时间
//origins: 设置允许跨域的域名,可以设置多个
//allowCredentials: 设置是否允许发送Cookie
//allowedHeaders: 设置允许跨域的Header
//exposedHeaders: 设置跨域时允许返回的Header
//methods: 设置允许跨域的请求方法
public class HelloController {
@GetMapping("/hello")
public String hello(){
return "hello cros";
}
@PostMapping("/hello")
public String helloPost(){
return "hello cros";
}
}
2.实现WebMvcConfigurer
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedHeaders("*")
.allowedMethods("*")
.allowedOrigins("http://localhost:8081")
.maxAge(1800);
}
}
3.注入一个CorsFilter到容器
@Configuration
public class WebMvcConfig {
@Bean
CorsFilter corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration buildConfig = new CorsConfiguration();
buildConfig.addAllowedOrigin("http://localhost:8081");
buildConfig.addAllowedMethod("*");
source.registerCorsConfiguration("/**", buildConfig);
return new CorsFilter(source);
}
}
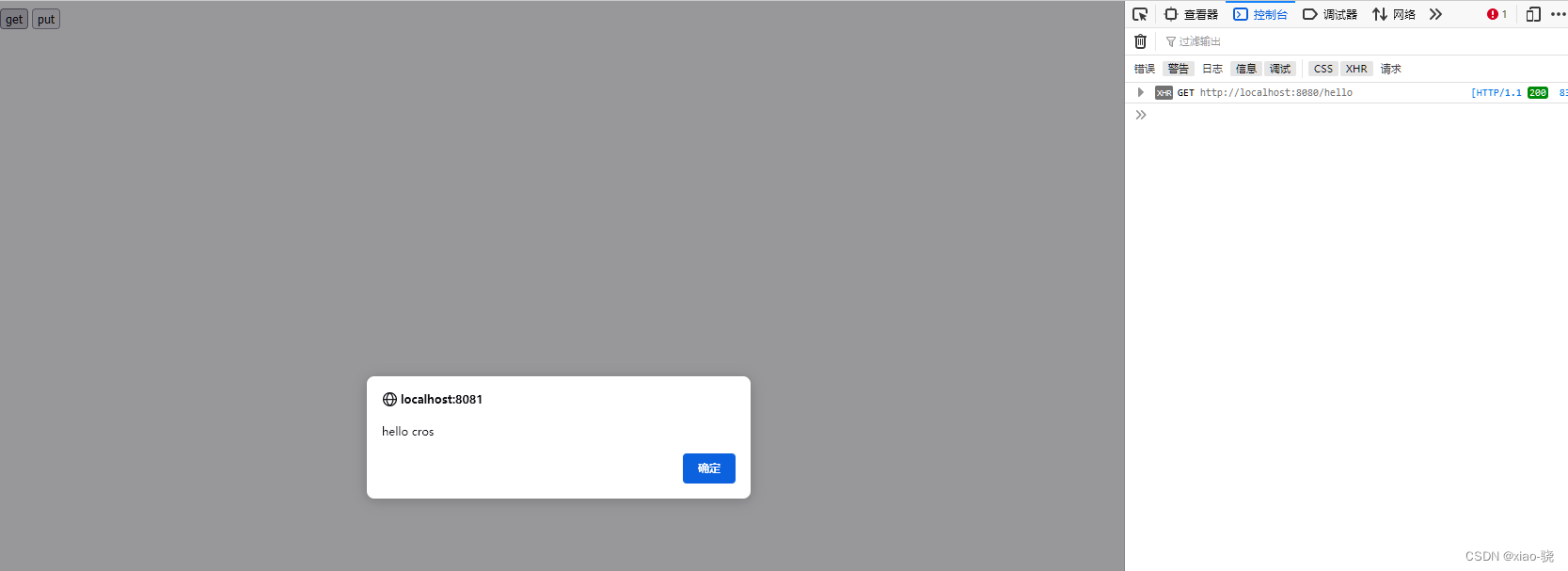
?
第一个是针对某一个方法或者类,而下面两个是针对全局
|