前言
本系列记录Java从入门开始的知识点,面向对象编程:初始面向对象,方法回顾和加深,对象的创建分析,面向对象三大特性,抽象类和接口。
一、初始面向对象
- 面向对象编码的本质就是:
以类的方式组织代码,以对象的方式封装数据 。
二、方法回顾和加深
1、方法定义
public static void main(String[] args) {
}
public String sayHello(){
return "hello world";
}
public int max(int a,int b){
return a > b ? a : b ;
}
}
2、方法的调用
1.静态方法和非静态方法
public class Student {
public static void say() {
System.out.println("学生说话了");
}
public void run(){
System.out.println("学生跑了");
}
public static void a(){
}
public void b(){}
}
public class demo02 {
public static void main(String[] args) {
Student.say();
Student student = new Student();
student.run();
}
}
- 引用传递
public class Demo03 {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.name);
System.out.println("===========================");
change(person);
System.out.println(person.name);
}
public static void change(Person person) {
person.name = "fanafan";
}
}
class Person{
String name;
}
三、对象的创建分析
1、对象的创建
public class Application {
public static void main(String[] args) {
Student xiaoming = new Student();
Student xiaohong = new Student();
xiaoming.name = "小明";
xiaoming.age = 33;
xiaohong.name = "小红";
xiaohong.age = 56;
System.out.println(xiaoming.name);
}
}
public class Student {
String name;
int age;
public void study(){
System.out.println(this.name+"在学习");
}
}
2、构造器
- 构造器和类名相同;
- 没有返回值
- new本质就是在调用构造方法;
- 构造方法可以初始化对象的值;
定义有参构造之后,如果想使用无参构造,就要显示的定义一个无参构造。
public static void main(String[] args) {
Person person = new Person();
Person fanafan = new Person("fanafan");
System.out.println(person.name);
System.out.println(fanafan.name);
}
public class Person {
String name;
public Person(){
this.name = "fanafan";
}
public Person(String name){
this.name = name;
}
}
四、面向对象三大特性
1、封装
高内聚,低耦合 ;- 提高程序的安全性,保护数据;
- 隐藏代码的实现细节;
- 统一接口;
- 系统的可维护性增加了。
- 封装快捷键:alt + insert
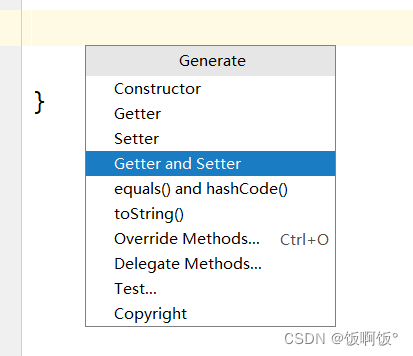
public class Application {
public static void main(String[] args) {
Student s1 = new Student();
s1.setName("fanafan");
System.out.println(s1.getName());
}
}
public class Student {
private String name;
private int id;
private char sex;
public String getName(){
return this.name;
}
public void setName(String name){
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
}
2、继承
- extands的意思是“扩展”,子类是父类的扩展;
- ctrl + h :会展示当前的继承树;
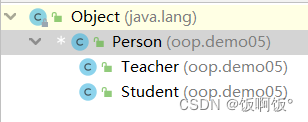 - Java中只有单继承,没有多继承,一个儿子只有一个父亲,一个父亲可以有多个孩子。
public class Person {
private int money = 10_0000_0000;
public void say(){
System.out.println("说了一句话");
}
public int getMoney() {
return money;
}
public void setMoney(int money) {
this.money = money;
}
}
public class Student extends Person{
}
public static void main(String[] args) {
Student student = new Student();
student.say();
System.out.println(student.getMoney());
}
- 详解super
(1)super调用父类的构造方法,必须在构造方法的第一个; (2)super只能出现在子类的方法或者构造方法中; (3)super和this不能同时调用构造方法; (4)VS this : ①代表的对象不同,this代表本身调用者;super代表父类; ②前提不同,this没有继承也能使用;super只能在继承条件下才可以使用; ③构造方法不同,this()本类的构造;super()父类的构造。
public class Application {
public static void main(String[] args) {
Student student = new Student();
student.test("weihan");
}
}
public class Person {
private int money = 10_0000_0000;
protected String name = "fanafan";
public void say(){
System.out.println("说了一句话");
}
public int getMoney() {
return money;
}
public void setMoney(int money) {
this.money = money;
}
public Person() {
System.out.println("Person无参执行了//");
}
}
public class Student extends Person{
private String name = "Vivian";
public void test(String name){
System.out.println(name);
System.out.println(this.name);
System.out.println(super.name);
}
public Student() {
System.out.println("Student无参执行了");
}
}
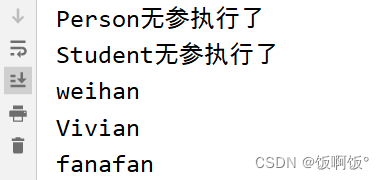
- 重写:需要有继承关系,子类重写父类的方法!
①方法名、参数列表必须相同; ②修饰符范围可以扩大,但不能缩小; ③抛出的异常范围可以缩小但不能扩大。
3、多态
- 多态是方法的多态,属性没有多态;
- 父类和子类,要有联系,否则会类型转换异常,ClassCastException;
- 存在条件:继承关系,方法需要重写,父类引用指向子类对象。
public static void main(String[] args) {
Student s1 = new Student();
Person s2 = new Student();
Object s3 = new Student();
s2.run();
s1.run();
((Student) s2).eat();
}
public class Student extends Person{
@Override
public void run() {
System.out.println("son");
}
public void eat(){
System.out.println("eat");
}
}
public class Person {
public void run(){
System.out.println("run");
}
}
- instanceof
判断两个类是不是父子关系;
public class Application {
public static void main(String[] args) {
Object object = new Student();
System.out.println(object instanceof Student);
System.out.println(object instanceof Person);
System.out.println(object instanceof Object);
System.out.println(object instanceof Teacher);
System.out.println(object instanceof String);
System.out.println("============================================");
Person person = new Student();
System.out.println(person instanceof Student);
System.out.println(person instanceof Person);
System.out.println(person instanceof Object);
System.out.println(person instanceof Teacher);
System.out.println("============================================");
Student student = new Student();
System.out.println(student instanceof Student);
System.out.println(student instanceof Person);
System.out.println(student instanceof Object);
}
}
- 高类型没办法调用低类型中的方法
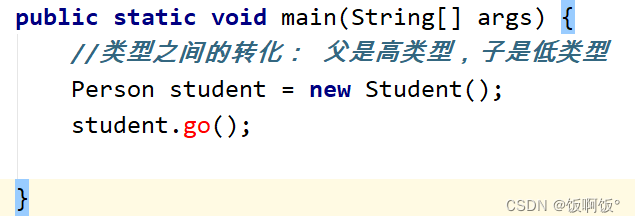 - 子类转换为父类,可能会丢失一些方法,

五、static关键字详解
- 非静态属性不能通过类直接调用
 - 非静态方法可以直接调用静态方法,但静态方法不能调用非静态方法。
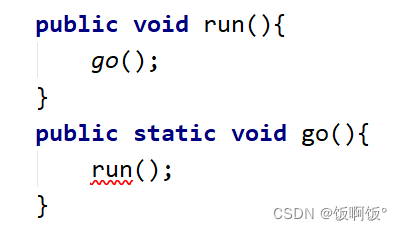
六、抽象类和接口
1、抽象类
- abstract 抽象方法,只有方法的名字,没有方法的实现。
public abstract class Action {
public abstract void doSomething();
}
- 抽象类不能new,只能靠子类去实现它;
- 抽象类中可以写普通方法;
- 抽象方法必须在抽象类中。
2、接口
- 接口不是类,而是对类的一组需求描述,这些类要遵从接口描述的统一格式进行定义;
- 定义的关键字是interface;
- 接口中的所有定义其实都是抽象的 public abstract,接口都需要有实现类
- 接口不能被实例化,接口中没有构造方法;
- implements可以实现多个接口,必须要重写接口中的方法。
public interface UserService {
void add(String name);
void delete(String name);
void update(String name);
void query(String name);
}
public interface TimeService {
void timer();
}
public class UserServiceImpl implements UserService,TimeService {
@Override
public void add(String name) {
}
}
|