本博文源于java课堂上的作业,理论和实践。有需要的读者可以收藏
理论
1、
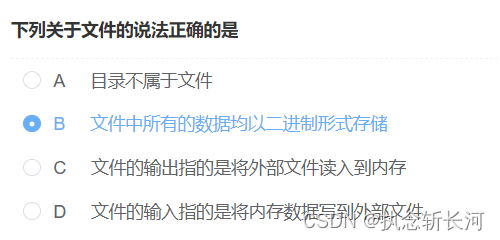
2、
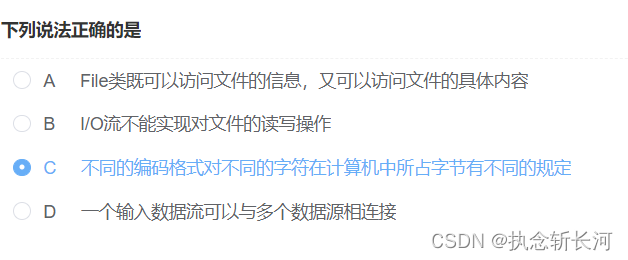
3、
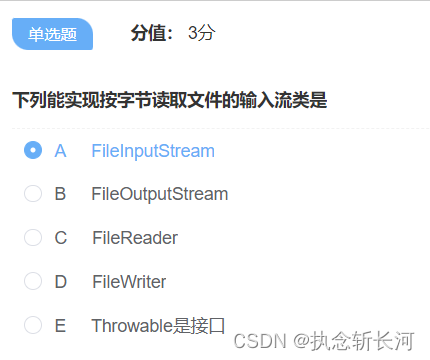
4、
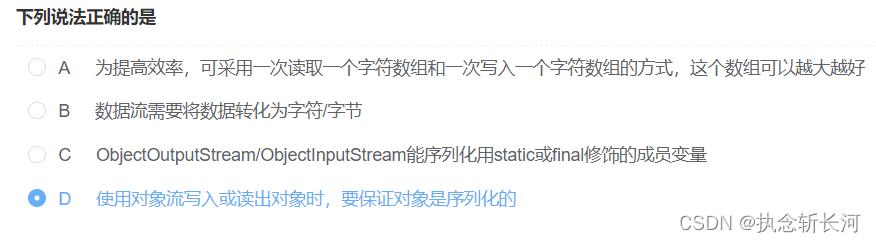
5、
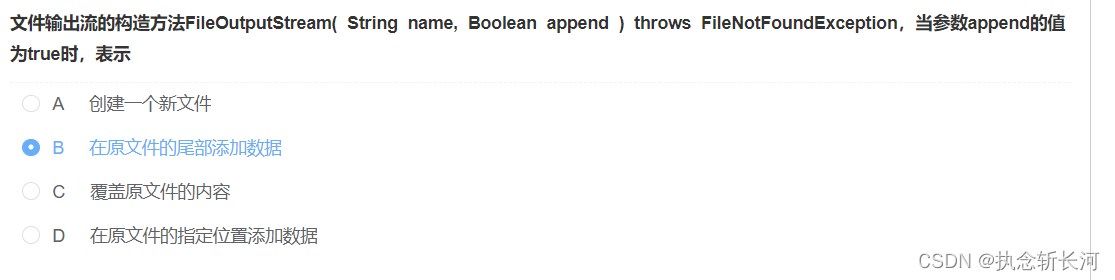
6、
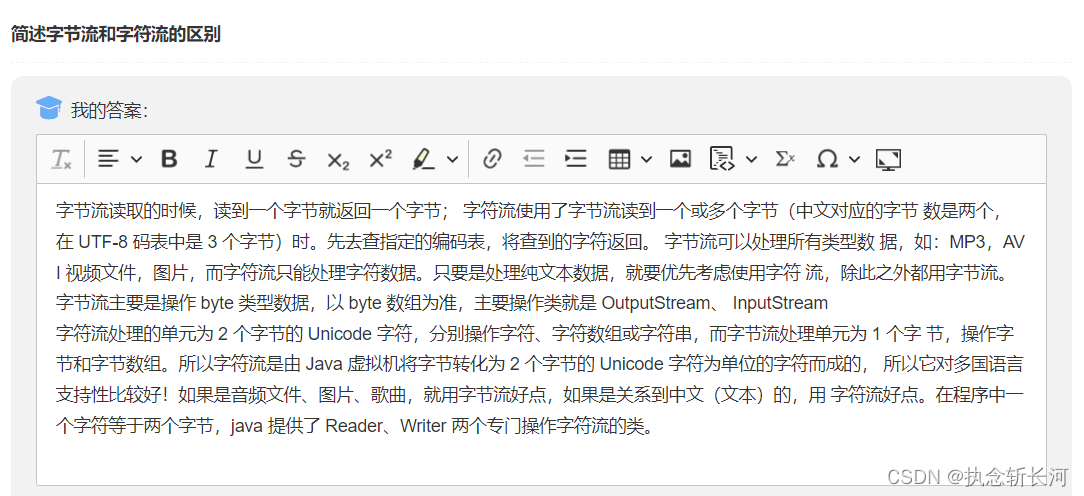
字节流读取的时候,读到一个字节就返回一个字节; 字符流使用了字节流读到一个或多个字节(中文对应的字节 数是两个,在 UTF-8 码表中是 3 个字节)时。先去查指定的编码表,将查到的字符返回。 字节流可以处理所有类型数 据,如:MP3,AVI 视频文件,图片,而字符流只能处理字符数据。只要是处理纯文本数据,就要优先考虑使用字符 流,除此之外都用字节流。字节流主要是操作 byte 类型数据,以 byte 数组为准,主要操作类就是 OutputStream、 InputStream 字符流处理的单元为 2 个字节的 Unicode 字符,分别操作字符、字符数组或字符串,而字节流处理单元为 1 个字 节,操作字节和字节数组。所以字符流是由 Java 虚拟机将字节转化为 2 个字节的 Unicode 字符为单位的字符而成的, 所以它对多国语言支持性比较好!如果是音频文件、图片、歌曲,就用字节流好点,如果是关系到中文(文本)的,用 字符流好点。在程序中一个字符等于两个字节,java 提供了 Reader、Writer 两个专门操作字符流的类。
8、阅读代码,指出其中的错误并改正
import java.io.*;
2. public class Test {
3. public static void main(String[] args) {
4. File f = new File("1.txt");
5. try {
6. FileOutputStream out = new FileOutputStream(f);
7. byte[] a = "hello world".getBytes();
8. out.write(a);
9. System.out.println(" >>>成功输出!");
10. out.flush();
11. } catch (Exception e) {
12. System.out.println(e.getMessage());
13. }
14. try {
15. FileReader in=new FileReader(f);
16. byte[] b = new byte[1024];
17. int len = in.read(b);
18. System.out.println(" >>>成功读取!");
19. int i=0;
20. while(b[i]!=0){
21. System.out.print((char)b[i++]);
22. }
23. } catch (Exception e) {
24. System.out.println(e.getMessage());
25. }
26. }
27. }
17行read函数参数类型错误
8、阅读代码,指出其中的错误并改正
1. import java.io.*;
2. public class Test {
3. public static void main(String[] args) {
4. File f = new File("1.txt");
5. try {
6. FileOutputStream out = new FileOutputStream(f);
7. byte[] a = "hello world".getBytes();
8. out.write(a);
9. System.out.println(" >>>成功输出!");
10. out.close();
11.
12. } catch (Exception e) {
13. System.out.println(e.getMessage());
14. }
15. try {
16. FileInputStream in=new FileInputStream (f);
17.
18. byte[] b = new byte[1024];
19. int len = in.read(b);
20. System.out.println(" >>>成功读取!");
21. int i=0;
22. while(b[i]!=0){
23. System.out.print((char)b[i++]);
24. }
25. } catch (Exception e) {
26. System.out.println(e.getMessage());
27. }
28. }
29. }
没有错误
9、阅读代码片段,将该代码片段运行3次,写出每次运行的输出结果1. import java.io.*;
import java.io.*;
2. public class test1 {
3. public static void main(String[] args){
4. try {
5. String file_name = "test.txt";
6. File file = new File(file_name);
7. if(file.exists()) {
8. System.out.println(file + " is existed!");
9. file.delete();
10. }
11. else {
12. file.createNewFile();
13. System.out.println(file + " has been created successfully!");
14. }
15. }
16. catch (IOException e){
17. e.printStackTrace();
18. }
19. }
20. }
}
test.txt has been created successfully!
test.txt is existed!
test.txt has been created successfully!
10、阅读代码片段,写出运行的输出结果
. import java.io.*;
2. public class test2 {
3. public static void main(String[] args) {
4. File file = new File("d:/TextRw.txt");
5. try {
6. if (!file.exists()) {
7. file.createNewFile();
8. }
9. FileOutputStream fl = new FileOutputStream(file);
10. String str = "Name : Jack\n";
11. String str2 = "Id : 10086\n";
12. fl.write(str.getBytes());
13. fl.write(str2.getBytes());
14. fl.close();
15. System.out.println("写入完成!");
16. FileInputStream fis = new FileInputStream(file);
17. System.out.println("Read from: " + file);
18. int d = fis.read();
19. while (d != -1) {
20. System.out.print((char) d);
21. d = fis.read();
22. }
23. System.out.println("输出完成!");
24. fis.close();
25. } catch (Exception e) {
26. e.printStackTrace();
27. }
28. }
29. }
11、请在当前目录下新建一个”test.txt”文件,在第一行写入”Hello World!”,在第二行写入“JAVA程序设计”。写入完成后请将文件中的内容读取,并输出到屏幕上,可以尝试多种方法实现文件的基本读取与写入。
package com.company;
import jdk.jfr.events.FileWriteEvent;
import jdk.nashorn.internal.ir.visitor.NodeOperatorVisitor;
import java.util.*;
import java.io.*;
class Main{
public static void main(String[] args) throws IOException {
BufferedReader buffR = null;
BufferedWriter buffW = null;
File fi = new File("test.txt");
try{
if(!fi.exists()){
fi.createNewFile();
}
buffW = new BufferedWriter(new FileWriter(fi));
buffW.write("Hello world\n");
buffW.write("java程序设计");
buffW.flush();
buffR = new BufferedReader(new FileReader(fi));
String temp = null;
while ((temp = buffR.readLine()) != null) {
System.out.println(temp);
}
} catch (Exception exception) {
exception.printStackTrace();
}finally {
buffR.close();
buffW.close();
}
}
}
实验
保存至main.java
1
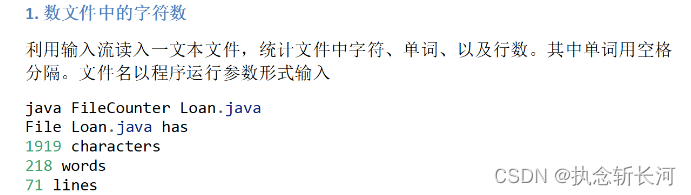
package com.company;
import jdk.jfr.events.FileWriteEvent;
import jdk.nashorn.internal.ir.visitor.NodeOperatorVisitor;
import java.util.*;
import java.io.*;
class Main{
public static void main(String[] args) throws Exception {
int lineCnt = 0;
int wrdCount = 0;
int charCnt = 0;
FileReader fR = new FileReader("test.txt");
BufferedReader bufR = new BufferedReader(fR);
String tmp = null;
while((tmp = bufR.readLine())!=null){
System.out.println(tmp);
lineCnt++;
String[] split = tmp.split(" ");
wrdCount += split.length;
String replace = tmp.replace(" ","");
charCnt += replace.length();
}
bufR.close();
System.out.println(charCnt + "characters");
System.out.println(wrdCount + "words");
System.out.println(lineCnt + "lines");
}
}
2、
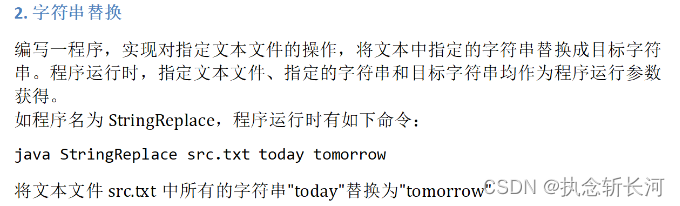
package com.company;
import jdk.jfr.events.FileWriteEvent;
import jdk.nashorn.internal.ir.visitor.NodeOperatorVisitor;
import javax.naming.StringRefAddr;
import java.util.*;
import java.io.*;
class Main{
public static void main(String[] args) throws IOException {
Scanner sc = new Scanner(System.in);
String filname = sc.next();
String target = sc.next();
String replacement = sc.next();
StringReplace(filname,target,replacement);
}
public static void StringReplace(String filename,String target,String replaced) throws IOException {
FileReader fR = new FileReader(filename);
BufferedReader bR = new BufferedReader(fR);
String tmp = null;
String replaceStr = "";
while((tmp = bR.readLine()) != null){
String replace = tmp.replace(target,replaced);
replaceStr += replace+'\n';
}
bR.close();
FileWriter fW = new FileWriter(filename);
BufferedWriter bW = new BufferedWriter(fW);
bW.write(replaceStr);
bW.close();
System.out.println("success");
}
}
3、
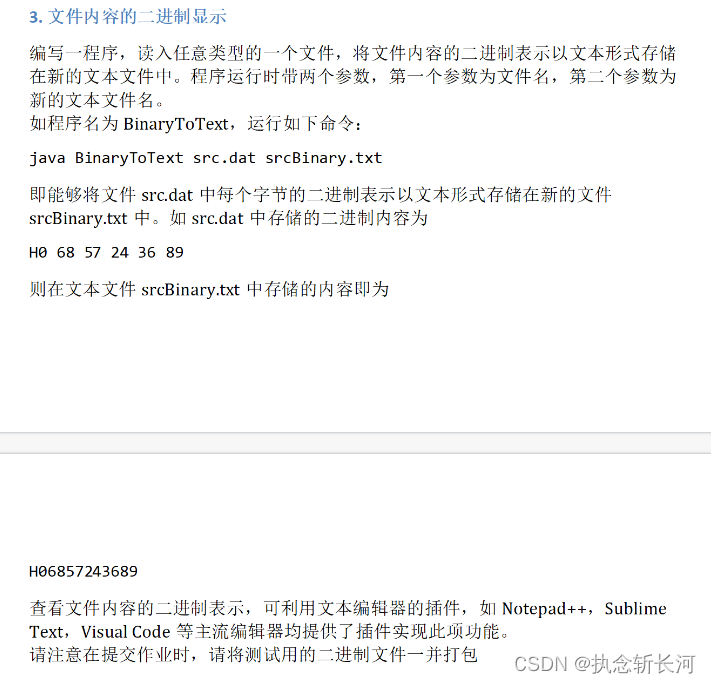
package com.company;
import jdk.jfr.events.FileWriteEvent;
import jdk.nashorn.internal.ir.visitor.NodeOperatorVisitor;
import javax.naming.StringRefAddr;
import java.util.*;
import java.io.*;
class Main{
public static void main(String[] args){
Scanner scanner = new Scanner(System.in);
String filename = scanner.next();
String newFilename = scanner.next();
try {
BinaryToTxt(filename, newFilename);
} catch (IOException e) {
e.printStackTrace();
}
}
private static void BinaryToTxt(String filename, String newFilename) throws IOException {
FileInputStream fileInputStream = new FileInputStream(filename);
int byteCount = 0;
String container = "";
while ((byteCount = fileInputStream.read()) != -1){
String string = Integer.toHexString(byteCount);
container += string;
}
System.out.println(container);
fileInputStream.close();
FileWriter fileWriter = new FileWriter(newFilename);
BufferedWriter bufferedWriter = new BufferedWriter(fileWriter);
bufferedWriter.write(container);
bufferedWriter.flush();
bufferedWriter.close();
}
}
|