目录
一、目录结构
二、项目介绍
三、代码实现
1、ApplicationContext类
2、BeanDefinition类
3、BeanNameAware接口
4、BeanPostProcessor接口
5、MyBeanPostProcessor类
6、InitializingBean接口
7、AppConfig类
8、OrderService类
9、UserInterface类
10、UserService类
11、Autowired注解
12、Component注解
13、ComponentScan注解
14、Scope注解
一、目录结构
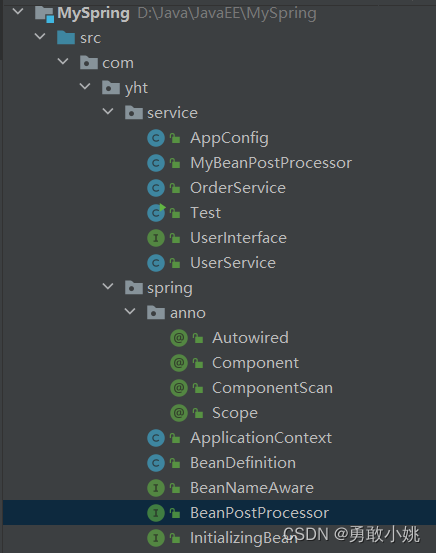
二、项目介绍
????????该项目简单模拟了Spring的实现,包括:Spring扫描包、BeanDefinition的实现、getBean方法的实现、Bean的创建流程、依赖注入、Aware回调机制、Spring中bean的初始化机制、BeanPostProcessor机制以及AOP机制。最终完成了Spring功能的简单模拟
三、代码实现
1、ApplicationContext类
package com.yht.spring;
import com.yht.spring.anno.Autowired;
import com.yht.spring.anno.Component;
import com.yht.spring.anno.ComponentScan;
import com.yht.spring.anno.Scope;
import java.beans.Introspector;
import java.io.File;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ConcurrentHashMap;
/**
* 通过应用程序上下文环境创建、获取、管理bean
*/
public class ApplicationContext {
//配置类
private Class configClass;
//存储BeanDefinition
private ConcurrentHashMap< String, BeanDefinition> beanDefinitionMap = new ConcurrentHashMap<>();
//单例池
private ConcurrentHashMap< String, Object> singletonObjectMap = new ConcurrentHashMap<>();
//存储后置执行器
private List<BeanPostProcessor> beanPostProcessorList = new ArrayList<>();
public ApplicationContext(Class configClass) {
//设置配置类
this.configClass = configClass;
//判断配置类有没有配置ComponentScan注解
if(configClass.isAnnotationPresent(ComponentScan.class)){
//获取ComponentScan的注解信息
ComponentScan componentScanAnnotation = (ComponentScan) configClass.getAnnotation(ComponentScan.class);
//获取value属性的值,即:扫描路径
String path = componentScanAnnotation.value();
//把路径由com.yht.service转为com/yht/service
path = path.replace(".", "/");
//获取当前类的类加载器,并得到绝对路径
ClassLoader classLoader = ApplicationContext.class.getClassLoader();
URL resource = classLoader.getResource(path);
File file = new File(resource.getFile());
//如果是文件夹
if(file.isDirectory()){
//获取当前文件夹下的所有文件
File[] files = file.listFiles();
for(File f : files){
//获取文件的绝对路径
String fileName = f.getAbsolutePath();
//寻找class文件
if(fileName.endsWith(".class")){
//解析className
String className = fileName.substring(fileName.indexOf("com"), fileName.indexOf(".class"));
className = className.replace("\\", ".");
//判断一个类有没有Component注解
try {
Class<?> aClass = classLoader.loadClass(className);
//如果有Component注解
if(aClass.isAnnotationPresent(Component.class)){
Component component = aClass.getAnnotation(Component.class);
//当前类是不是由BeanPostProcessor派生的
if(BeanPostProcessor.class.isAssignableFrom(aClass)){
//创建对象,存入beanPostProcessorList
BeanPostProcessor instance = (BeanPostProcessor) aClass.newInstance();
beanPostProcessorList.add(instance);
}
//获取beanName
String beanName = component.value();
if(beanName.equals("")){
beanName = Introspector.decapitalize(aClass.getSimpleName());
}
//定义BeanDefinition
BeanDefinition beanDefinition = new BeanDefinition();
beanDefinition.setType(aClass);
//设置bean的scope属性
if(aClass.isAnnotationPresent(Scope.class)){
Scope scopeAnnotation = aClass.getAnnotation(Scope.class);
beanDefinition.setScope(scopeAnnotation.value());
}else{
//默认为singleton
beanDefinition.setScope("singleton");
}
//放入beanDefinitionMap
beanDefinitionMap.put(beanName, beanDefinition);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
}
}
//创建单例Bean
for(String beanName : beanDefinitionMap.keySet()){
//获取BeanDefinition
BeanDefinition beanDefinition = beanDefinitionMap.get(beanName);
//如果是单例bean
if(beanDefinition.getScope().equals("singleton")){
//创建单例bean,并放入单例池中
Object o = createBean(beanName, beanDefinition);
singletonObjectMap.put(beanName, o);
}
}
}
//获取bean
public Object getBean(String beanName){
//根据beanName获取beanDefintion
BeanDefinition beanDefinition = beanDefinitionMap.get(beanName);
if(beanDefinition == null){
throw new NullPointerException();
}else{
//获取Bean的scope属性
String scope = beanDefinition.getScope();
//如果是单例bean
if("singleton".equals(scope)){
//从单例池中获取bean对象
Object bean = singletonObjectMap.get(beanName);
//如果单例池中没有
if(bean == null){
//创建bean对象,然后放入单例池
Object o = createBean(beanName, beanDefinition);
singletonObjectMap.put(beanName, o);
}
return bean;
}else{ //如果是多例bean,则直接创建bean
return createBean(beanName, beanDefinition);
}
}
}
/**
* 创建bean对象
* @param beanName
* @param beanDefinition
* @return
*/
public Object createBean(String beanName, BeanDefinition beanDefinition) {
//获取类的类型
Class aClass = beanDefinition.getType();
Object instance = null;
try {
//创建类
instance = aClass.getConstructor().newInstance();
//依赖注入,获取类的字段
for (Field f : aClass.getDeclaredFields()) {
//如果有autowired注解
if(f.isAnnotationPresent(Autowired.class)){
//修改字段可见性
f.setAccessible(true);
//设置值
f.set(instance, getBean(f.getName()));
}
}
//判断当前类有没有实现BeanNameAware接口,如果实现了就进行Aware回调,实现BeanName的回调
if(instance instanceof BeanNameAware){
((BeanNameAware) instance).setBeanName(beanName);
}
//初始化之前执行
for (BeanPostProcessor beanPostProcessor : beanPostProcessorList) {
instance = beanPostProcessor.postProcessBeforeInitialization(beanName, instance);
}
//初始化
if(instance instanceof InitializingBean){
((InitializingBean) instance).afterPropertiesSet();
}
//初始化后执行
for (BeanPostProcessor beanPostProcessor : beanPostProcessorList) {
//代理对象
instance = beanPostProcessor.postProcessAfterInitialization(beanName, instance);
}
return instance;
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (NoSuchMethodException e) {
e.printStackTrace();
}
return null;
}
}
2、BeanDefinition类
package com.yht.spring;
/**
* bean信息的定义,描述一个bean的全部信息,如:类的class类型、Bean的作用域、是否懒加载等
*/
public class BeanDefinition {
//class类型
private Class type;
//bean的作用域
private String scope;
public Class getType() {
return type;
}
public void setType(Class type) {
this.type = type;
}
public String getScope() {
return scope;
}
public void setScope(String scope) {
this.scope = scope;
}
}
3、BeanNameAware接口
package com.yht.spring;
/**
* Bean如果想知道自身的名字,就需要实现该接口。
*/
public interface BeanNameAware {
void setBeanName(String beanName);
}
4、BeanPostProcessor接口
package com.yht.spring;
/**
* 后置处理器
* bean对象在实例化和依赖注入完毕之后,在显式调用初始化方法的前后添加自定义的逻辑
*/
public interface BeanPostProcessor {
/**
* 在调用初始化之前完成的一些操作
* @param beanName
* @param bean
* @return
*/
Object postProcessBeforeInitialization(String beanName, Object bean);
/**
* 在初始化之后执行
* @param beanName
* @param bean
* @return
*/
Object postProcessAfterInitialization(String beanName, Object bean);
}
5、MyBeanPostProcessor类
package com.yht.service;
import com.yht.spring.BeanPostProcessor;
import com.yht.spring.anno.Component;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
/**
* eanPostProcessor的实现类
*/
@Component
public class MyBeanPostProcessor implements BeanPostProcessor {
/**
* 初始化前执行的方法
* @param beanName
* @param bean
* @return
*/
@Override
public Object postProcessBeforeInitialization(String beanName, Object bean) {
if(beanName.equals("userService")){
System.out.println("初始化前");
}
return bean;
}
/**
* 初始化后执行的方法
* @param beanName
* @param bean
* @return
*/
@Override
public Object postProcessAfterInitialization(String beanName, Object bean) {
if(beanName.equals("userService")){
//生成代理对象
Object proxy = Proxy.newProxyInstance(MyBeanPostProcessor.class.getClassLoader(), bean.getClass().getInterfaces(), new InvocationHandler() {
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("切面逻辑");
return method.invoke(bean, args);
}
});
return proxy;
}
return bean;
}
}
6、InitializingBean接口
package com.yht.spring;
/**
* 初始化接口
*/
public interface InitializingBean {
/**
* 初始化方法
*/
void afterPropertiesSet();
}
7、AppConfig类
package com.yht.service;
import com.yht.spring.anno.ComponentScan;
/**
* 配置类
*/
@ComponentScan("com.yht.service")
public class AppConfig {
}
8、OrderService类
package com.yht.service;
import com.yht.spring.anno.Component;
@Component
public class OrderService {
}
9、UserInterface类
package com.yht.service;
public interface UserInterface {
void test();
}
10、UserService类
package com.yht.service;
import com.yht.spring.BeanNameAware;
import com.yht.spring.InitializingBean;
import com.yht.spring.anno.Autowired;
import com.yht.spring.anno.Component;
import com.yht.spring.anno.Scope;
@Component("userService")
public class UserService implements BeanNameAware, InitializingBean, UserInterface {
@Autowired
private OrderService orderService;
private String beanName;
private String num;
public void test(){
System.out.println(orderService);
}
@Override
public void setBeanName(String beanName) {
}
@Override
public void afterPropertiesSet() {
}
}
11、Autowired注解
package com.yht.spring.anno;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Autowired {
}
12、Component注解
package com.yht.spring.anno;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
//只能写在类上
@Target(ElementType.TYPE)
public @interface Component {
String value() default "";
}
13、ComponentScan注解
package com.yht.spring.anno;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
//只能写在类上
@Target(ElementType.TYPE)
public @interface ComponentScan {
String value() default "";
}
14、Scope注解
package com.yht.spring.anno;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
//只能写在类上
@Target(ElementType.TYPE)
public @interface Scope {
String value() default "";
}
执行结果:
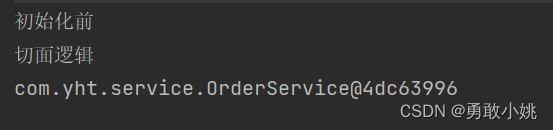
?
|