前言
本文依托于
在SpringCloud Alibaba的使用过程中,我总结为如下步骤:
- 下载并启动服务端
- 客户端引入spring-cloud-starter-alibaba的jar包
- 客户端properties或yml加入相关配置
- 客户端加上相应的注解开启功能
- 服务端增加相应配置
- 数据持久化,服务端集群部署
sentinel服务端
1.下载sentinel
https://github.com/alibaba/Sentinel/releases/
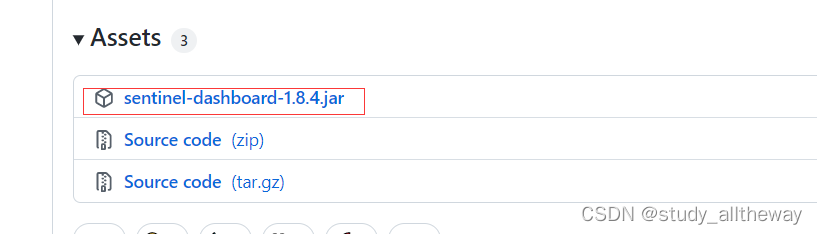
2.启动sentinel服务
java -jar sentinel-dashboard-1.8.4.jar
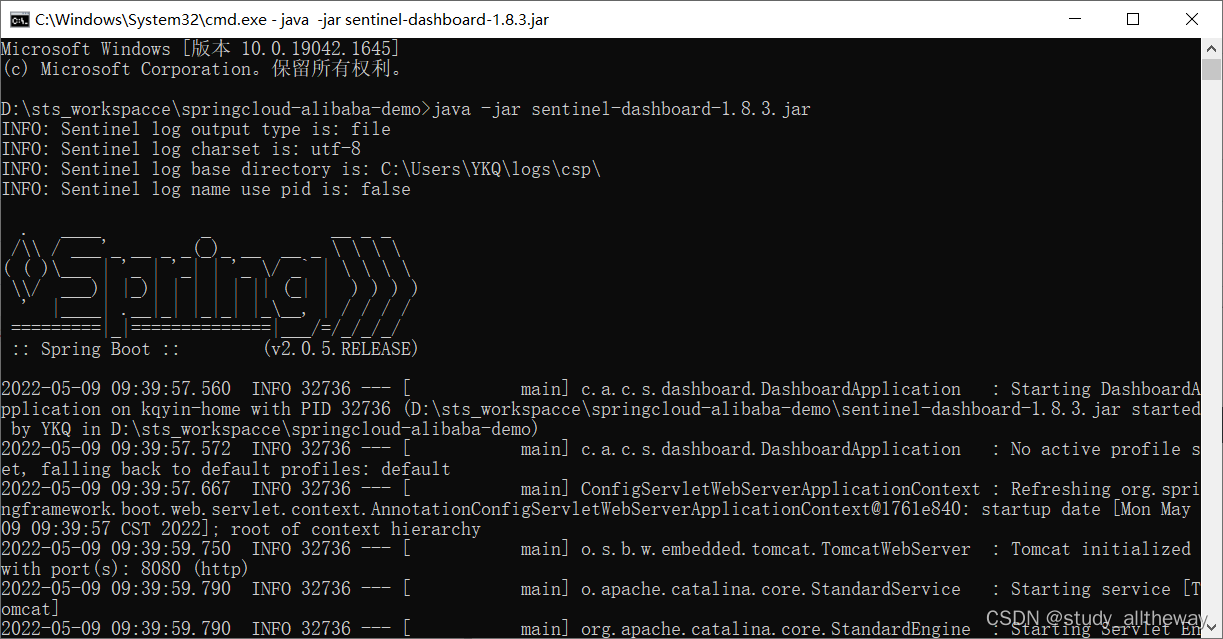
3.访问sentinel服务
sentinel默认端口为8080,用户名:sentinel,密码:sentinel,上下文为空,路径不需要加sentinel
http://127.0.0.1:8080/
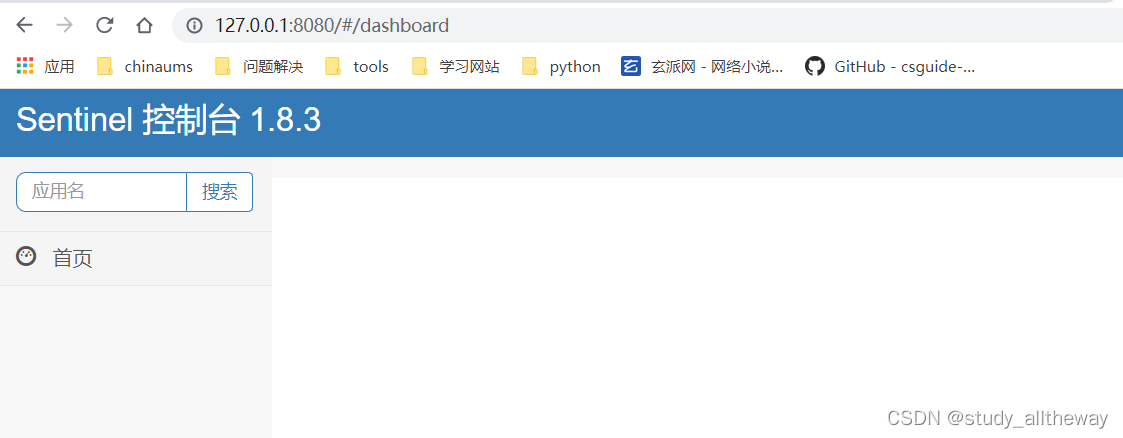
sentinel客户端
1.引入jar
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
2.properties加入配置
# 应用名称
spring.application.name=springcloud-alibaba-sentinel
server.port=9007
# Sentinel 控制台地址
spring.cloud.sentinel.transport.dashboard=192.168.43.11:8080
# 取消Sentinel控制台懒加载
# 默认情况下 Sentinel 会在客户端首次调用的时候进行初始化,开始向控制台发送心跳包
# 配置 sentinel.eager=true 时,取消Sentinel控制台懒加载功能
spring.cloud.sentinel.eager=true
# 如果有多套网络,又无法正确获取本机IP,则需要使用下面的参数设置当前机器可被外部访问的IP地址,供admin控制台使用
# spring.cloud.sentinel.transport.client-ip=
# feign中激活sentinel,有些类似hystrix
feign.sentinel.enabled=true
3.加入注解@SentinelResource
package com.ykq.springcloudalibabasentinel.controller;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SentinelController {
@SentinelResource(value = "sentinelTestA", blockHandler = "handleTestA")
@RequestMapping(value = "/sentinelTestA")
public String sentinelTestA() {
return "sentinelTestA";
}
public String handleTestA(BlockException blockException) {
return "sentinelTestA is limited";
}
@SentinelResource(value = "sentinelTestB", fallback = "testBFallBack", blockHandler = "handleTestB")
@RequestMapping(value = "/sentinelTestB")
public String sentinelTestB() {
throw new RuntimeException("sentinelTestB throw RuntimeException");
}
public String handleTestB(BlockException blockException) {
return "sentinelTestB is limited";
}
public String testBFallBack() {
return "sentinelTestB is stop";
}
}
4.在sentinel配置相关信息
(1)配置限流规则
qps每秒多少次请求。下图表示在单机情况下,超过每秒3次请求就快速失败。 对应资源为代码中@SentinelResource值为sentinelTestA的代码。 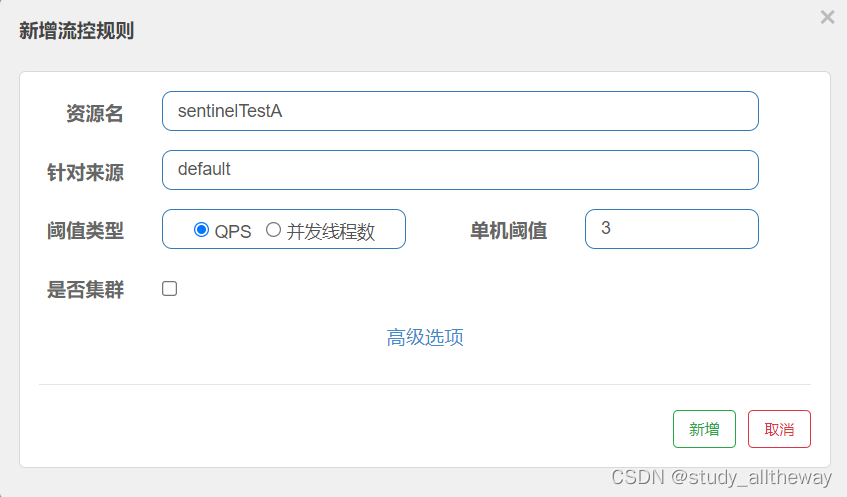
(2)配置熔断规则
在1000ms内,请求2次及以上,失败概率为100%的,熔断5秒 对应资源为代码中@SentinelResource值为sentinelTestB的代码。 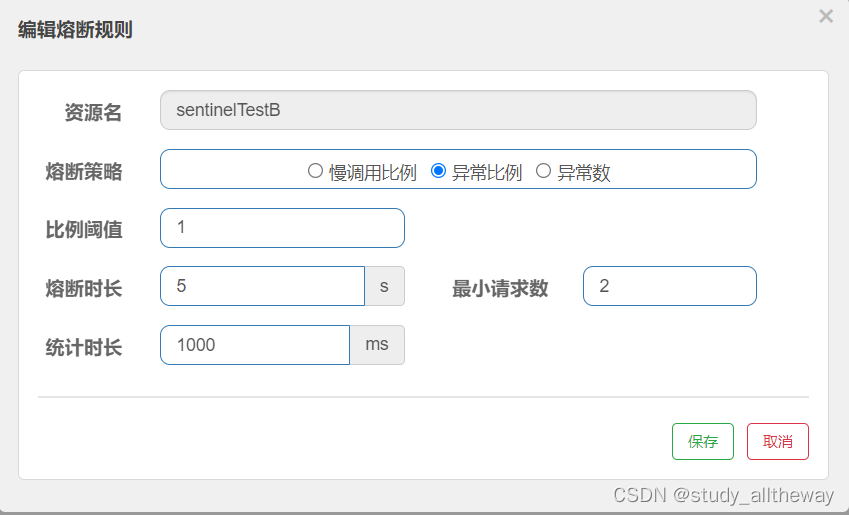
5.blockHandler不生效
@SentinelResource中的value和@RequestParam中的value不要一致。我测试时,两者一致,导致出现规则生效,但blockHandler大概率失效的情况。这是因为sentinel将方法已经定义为资源,value为@RequestParam的值 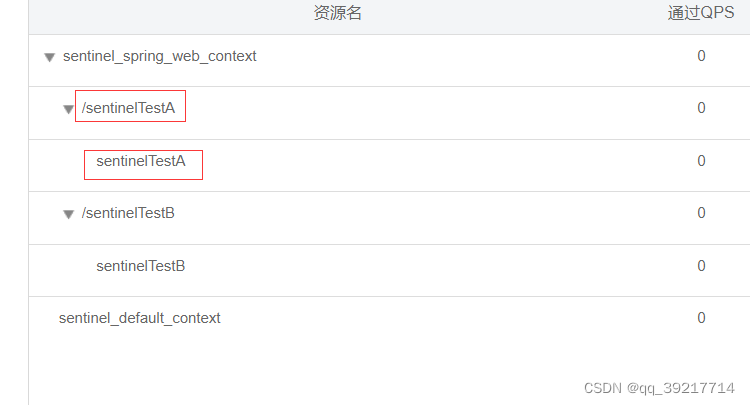
sentinel数据持久化
我们可以注意到,在sentinel中配置的规则,在客户端重启后,就失效了。这是因为规则默认保存在客户端的内存中,生产上是不允许这样使用的。需要将规则持久化到nacos配置中心。
1.引入jar
<dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>sentinel-datasource-nacos</artifactId>
<version>1.8.4</version>
</dependency>
2.properties加入配置
# 资源sentinelTestA持久化到nacos配置
spring.cloud.sentinel.datasource.sentinelTestA.nacos.username=nacos
spring.cloud.sentinel.datasource.sentinelTestA.nacos.password=nacos
spring.cloud.sentinel.datasource.sentinelTestA.nacos.server-addr=192.168.43.11:8000
spring.cloud.sentinel.datasource.sentinelTestA.nacos.dataId=${spring.application.name}-sentinelTestA
spring.cloud.sentinel.datasource.sentinelTestA.nacos.groupId=SENTINEL_GROUP
# 规则:flow流控规则,degrade降级规则,authority授权规则,system系统规则, param-flow热点key规则
spring.cloud.sentinel.datasource.sentinelTestA.nacos.rule-type=flow
spring.cloud.sentinel.datasource.sentinelTestA.nacos.data-type=json
# 资源sentinelTestB持久化到nacos配置
spring.cloud.sentinel.datasource.sentinelTestB.nacos.username=nacos
spring.cloud.sentinel.datasource.sentinelTestB.nacos.password=nacos
spring.cloud.sentinel.datasource.sentinelTestB.nacos.server-addr=192.168.43.11:8000
spring.cloud.sentinel.datasource.sentinelTestB.nacos.dataId=${spring.application.name}-sentinelTestB
spring.cloud.sentinel.datasource.sentinelTestB.nacos.groupId=SENTINEL_GROUP
# 规则:flow流控规则,degrade降级规则,authority授权规则,system系统规则, param-flow热点key规则
spring.cloud.sentinel.datasource.sentinelTestB.nacos.rule-type=degrade
spring.cloud.sentinel.datasource.sentinelTestB.nacos.data-type=json
3.nacos配置规则
可以注意到,当nacos中配置的规则修改后,可以同步到sentinel的服务,但是sentinel修改后,不能修改nacos中的配置,只能保存到内存中。
(1)配置参数来源
规则配置的参数名,可以在AbstractRule的几个实现类中去查看,分别是
- FlowRule(流控规则)
- DegradeRule(熔断规则)
- ParamFlowRule(热点规则)
- SystemRule(系统规则)
- AuthorityRule(授权规则)
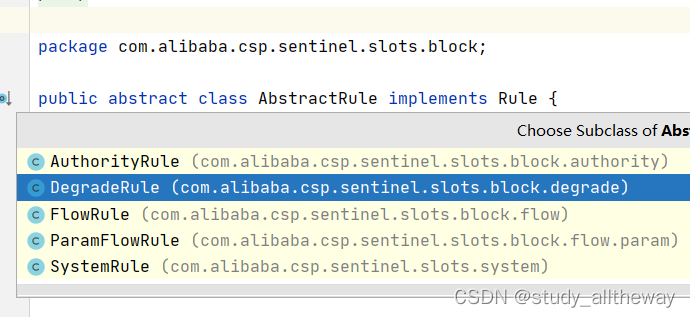
(2)流控规则
FlowRule(流控规则)参数
private int grade = RuleConstant.FLOW_GRADE_QPS;
private double count;
private int strategy = RuleConstant.STRATEGY_DIRECT;
private String refResource;
private int controlBehavior = RuleConstant.CONTROL_BEHAVIOR_DEFAULT;
private int warmUpPeriodSec = 10;
private int maxQueueingTimeMs = 500;
private boolean clusterMode;
private ClusterFlowConfig clusterConfig;
private TrafficShapingController controller;
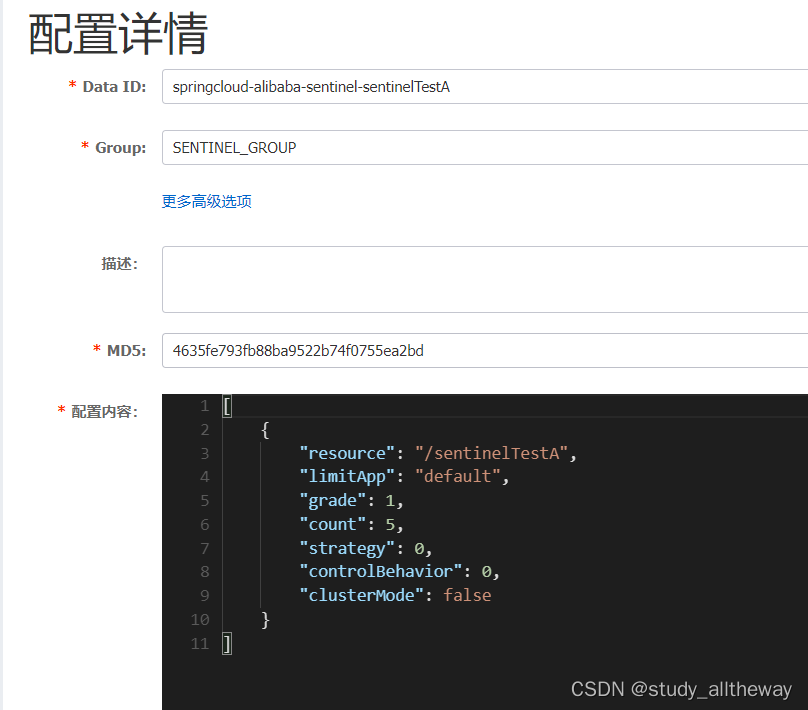
(3)熔断规则
DegradeRule(熔断规则)参数
private int grade = RuleConstant.DEGRADE_GRADE_RT;
private double count;
private int timeWindow;
private int minRequestAmount = RuleConstant.DEGRADE_DEFAULT_MIN_REQUEST_AMOUNT;
private double slowRatioThreshold = 1.0d;
private int statIntervalMs = 1000;
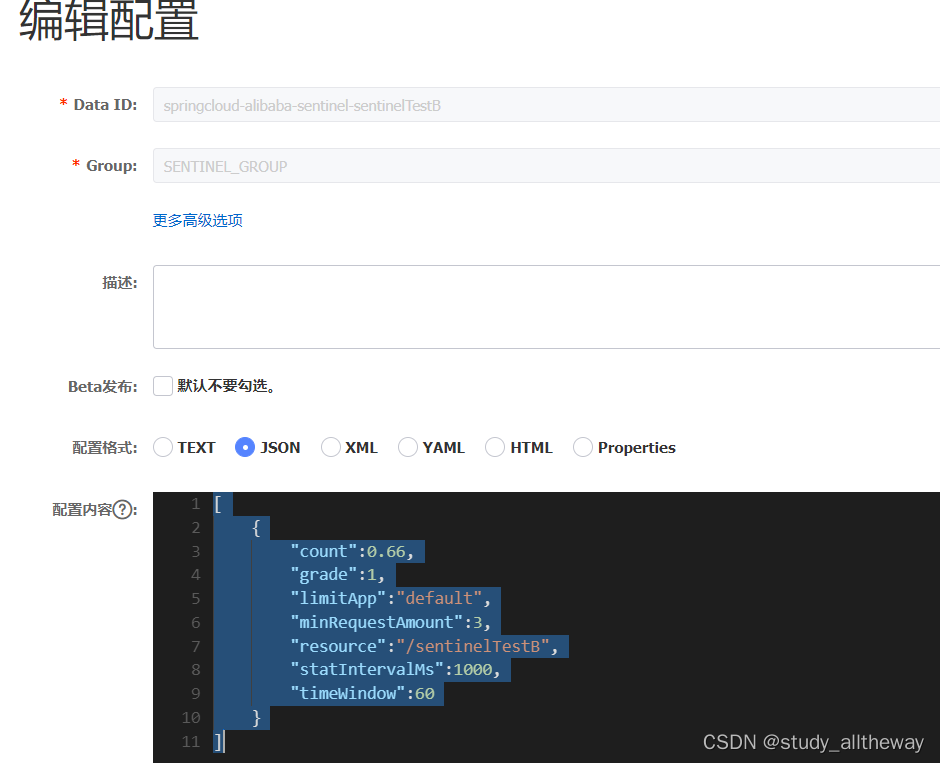
4.sentinel规则同步到nacos
https://blog.didispace.com/spring-cloud-alibaba-sentinel-2-4/
|