多线程的使用
效果:
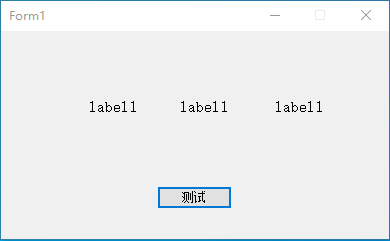
1.要求?
Label_Test1 数字不停的自增,到10的时候停止,然后 Label_Test2 开始自增,到30的时候停止,Label_Test3 则不停的自增。用线程的方式实现。
2.代码
新建一个Winform项目,界面如下,就四个控件
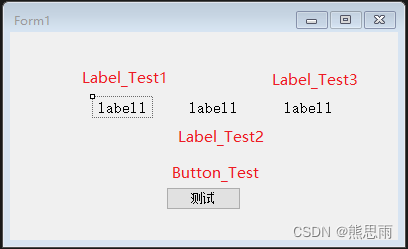
再新建一个脚本Test
using System;
using System.Threading;
namespace WindowsFormsApp1
{
internal class Test
{
#region 字段
private Thread Thread1;
private Thread Thread2;
private Thread Thread3;
private bool ThreadIsRuning = false;
public Action<int> Event_CallBack1;
public Action<int> Event_CallBack2;
public Action<int> Event_CallBack3;
private int value1 = 0;
public int Value1
{
get { return value1; }
set { value1 = value; }
}
private int value2 = 0;
public int Value2
{
get { return value2; }
set { value2 = value; }
}
private int value3 = 0;
public int Value3
{
get { return value3; }
set { value3 = value; }
}
#endregion
private void Init()
{
Thread1 = new Thread(Function1);
Thread1.IsBackground = true;
Thread2 = new Thread(Function2);
Thread2.IsBackground = true;
Thread3 = new Thread(Function3);
Thread3.IsBackground = true;
}
public void StartThread()
{
if(ThreadIsRuning) return;
Thread1.Start();
Thread2.Start();
Thread3.Start();
ThreadIsRuning = true;
}
public void StopAllThread()
{
Thread1.Abort();
Thread2.Abort();
Thread3.Abort();
}
private void Function1()
{
while (true)
{
Thread.Sleep(500);
Value1++;
if(Event_CallBack1 != null)
Event_CallBack1(Value1);
}
}
private void Function2()
{
bool isEnd = false;
while (true)
{
if (Value1 == 10)
{
Thread1.Abort();
isEnd = true;
break;
}
}
while (isEnd)
{
Thread.Sleep(500);
Value2++;
if(Event_CallBack2 != null)
Event_CallBack2(Value2);
if(value2 >= 30)
{
Thread2.Abort();
break;
}
}
}
private void Function3()
{
while (true)
{
Thread.Sleep(500);
Value3++;
if(Event_CallBack3 != null)
Event_CallBack3(Value3);
}
}
public Test()
{
Init();
}
}
}
脚本?Form1
using System;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private Test MyTest;
private void Form1_Load(object sender, EventArgs e)
{
MyTest = new Test();
MyTest.Event_CallBack1 = Callback1;
MyTest.Event_CallBack2 = Callback2;
MyTest.Event_CallBack3 = Callback3;
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
MyTest.StopAllThread();
}
/// <summary>
/// 按钮点击事件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void Button_Test_Click(object sender, EventArgs e)
{
MyTest.StartThread();
}
private void Callback1(int value)
{
Console.WriteLine("回调1:" + value);
if (this.InvokeRequired)
{
this.Invoke(new MethodInvoker(delegate
{
Label_Test1.Text = value.ToString();
}));
}
else
{
Label_Test1.Text = value.ToString();
}
}
private void Callback2(int value)
{
Console.WriteLine("回调2:" + value);
if (this.InvokeRequired)
{
this.Invoke(new MethodInvoker(delegate
{
Label_Test2.Text = value.ToString();
}));
}
else
{
Label_Test2.Text = value.ToString();
}
}
private void Callback3(int value)
{
Console.WriteLine("回调3:" + value);
if (this.InvokeRequired)
{
this.Invoke(new MethodInvoker(delegate
{
Label_Test3.Text = value.ToString();
}));
}
else
{
Label_Test3.Text = value.ToString();
}
}
}
}
3.效果
运行
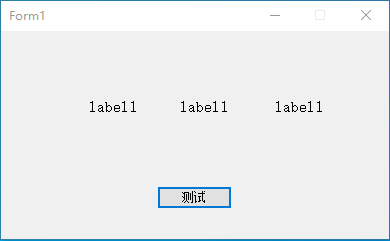
源码下载:点击下载
end
|