1.IOC容器
控制反转:把对象的创建和对象之间的调用过程都交给Spring进行管理,目的是为了降低耦合度;
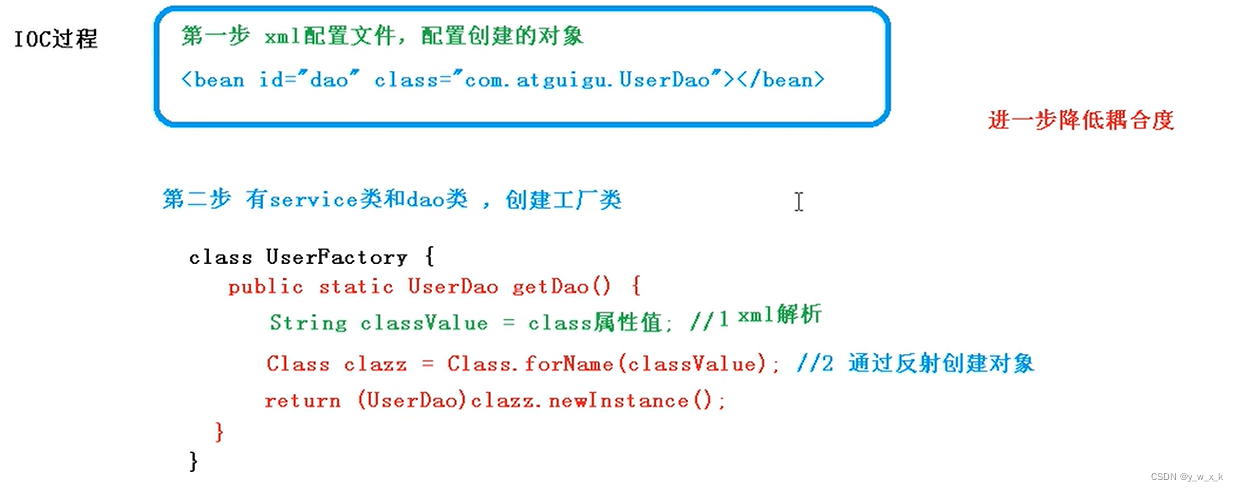
IOC思想基于IOC容器完成,IOC容器底层就是对象工厂
建议放到容器中的对象:dao类,service类,controller类,工具类,spring容器中的对象都是单例,容器中每个对象只存在一个;
不建议放到容器中的对象:
- 实体类对象,实体类对象的数据来自数据库,应该是在程序运行过程中创建
- servlet,filter,listener,这些类应该由tomcat负责创建与调用
将对象放到容器中有两种方式
2.Bean标签创建容器对象
通过容器使用对象:
- 在resources目录下配置mybeans.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="WarMovie" class="com.ywxk.spring5.service.impl.WarMovieInterImpl"></bean>
<bean id="myDate" class="java.util.Date"></bean>
</beans>
ApplicationContext context=new ClassPathXmlApplicationContext("beans.xml");
WarMovieInterImpl warMovie =context.getBean("WarMovie",WarMovieInterImpl.class);
warMovie.seeMovie();
Date date=(Date)context.getBean("myDate");
3.依赖注入(Dependency Injection)DI
bean实例在调用无参数构造方法创建对象后,就要对bean对象的属性进行初始化,初始化是由容器完成的,称为依赖注入,根据注入的方式分为set注入,构造注入;
set注入(设值注入):spring调用类的set方法,在set方法可以实现属性的赋值,实际开发中80%都是使用该方式;
//简单类型
<bean id="director" class="com.ywxk.spring5.service.T1.Director">
<property name="name" value="路阳"></property>
<property name="age" value="35"></property>
</bean>
//引用类型,property里面用ref="beanid"
<bean id="company" class="com.ywxk.spring5.service.T1.Company">
<property name="addr" value="北京市"></property>
<property name="companyName" value="北京文化"></property>
<property name="registerMoney" value="2000000"></property>
</bean>
<bean id="director" class="com.ywxk.spring5.service.T1.Director">
<property name="name" value="路阳"></property>
<property name="age" value="35"></property>
<property name="nick" value="烧水水"></property>
<property name="com" ref="company"></property>
</bean>
构造注入:spring调用类的有参构造方法,创建对象,在构造方法中完成属性的赋值;
<bean class="com.ywxk.spring5.service.T1.Player" id="player">
<constructor-arg name="pName" value="刘德华"></constructor-arg>
<constructor-arg index="1" value="53"></constructor-arg>
<constructor-arg name="sex" value="true"></constructor-arg>
<constructor-arg name="com" ref="company"></constructor-arg>
</bean>
public Player(String pName, int age, boolean sex,Company com) {
this.pName = pName;
this.age = age;
this.sex = sex;
this.com= com;
}
用构造注入获取file对象
<bean name="myFile" class="java.io.File">
<constructor-arg name="parent" value="C:\Users\Administrator\Desktop\临时"></constructor-arg>
<constructor-arg name="child" value="aa.txt"></constructor-arg>
</bean>
4.引用类型的自动注入
实际开发中可能会碰到一个类里面有多个引用属性,如果逐个配置标签会导致代码冗余,所以spring提供了一种自动注入引用类型的功能,该功能只能为引用属性自动赋值;
-
byName(按名称)方式:如果java类中引用类型的属性名与bean中的类的id一致,且数据类型一致,那么spring就能对该属性进行自动注入 <bean id="WarMovie" class="com.ywxk.spring5.service.impl.WarMovieInterImpl" autowire="byName"></bean>
public class WarMovieInterImpl implements MovieInter {
private Player player;
private Director director;
...
}
<bean id="director" class="com.ywxk.spring5.service.T1.Director">
<property name="name" value="路阳"></property>
<property name="age" value="35"></property>
<property name="nick" value="烧水水"></property>
<property name="com" ref="company"></property>
</bean>
<bean id="player" class="com.ywxk.spring5.service.T1.Player" >
<constructor-arg value="刘德华"></constructor-arg>
<constructor-arg index="1" value="53"></constructor-arg>
<constructor-arg value="true"></constructor-arg>
<constructor-arg ref="company"></constructor-arg>
</bean>
-
byType(按类型)方式:如果java类中引用类型的属性类型与bean中的类的class是同源的,那么spring就能对该属性进行自动注入 这里的同源指的是:
- java类中引用类型的属性类型与bean中的类的class同一个类;
- java类中引用类型的属性类型是bean中的类的class的父类;
- java类中引用类型的属性类型与bean中的类的class是接口与实现类的关系;
<bean id="WarMovie" class="com.ywxk.spring5.service.impl.WarMovieInterImpl" autowire="byType"></bean>
<bean id="playerSon" class="com.ywxk.spring5.service.T1.PlayerSon" >
<constructor-arg value="刘德华"></constructor-arg>
<constructor-arg index="1" value="53"></constructor-arg>
<constructor-arg value="true"></constructor-arg>
<constructor-arg ref="company"></constructor-arg>
</bean>
public class WarMovieInterImpl implements MovieInter {
private Player player;
...
}
public class PlayerSon extends Player{
public PlayerSon(String pName, int age, boolean sex, Company com) {
super(pName, age, sex, com);
System.out.println("player子类");
}
}
5.多配置文件
如果项目较为庞大,可以使用多个配置文件
- 可以按功能模块,一个模块一个配置文件
- 按类的功能,数据库相关一个配置文件,事物功能一个配置文件,service功能一个配置文件…
<import resource="classpath:package1/moviePackage1.xml"></import>
<import resource="companyPackage1.xml"></import>
<import resource="classpath:package1/package-*.xml"></import>
<bean id="companyPackage1" class="com.ywxk.spring5.package1.CompanyPackage1"></bean>
<bean id="moviePackage1" class="com.ywxk.spring5.package1.MoviePackage1"></bean>
6.注解方式的依赖注入
|