springboot整合mybatis有源码保证能跑
说明
网上一大片教程,不写版本号也没有源码,缺斤少两,调试半天还是跑不通,我真的会谢。
本教程使用flyway,良心推荐使用flyway进行数据库版本管理,用了都说好,可惜就是没有回滚版本功能。
flyway整合教程参考这里
mybatis整合
数据库表建立之后: 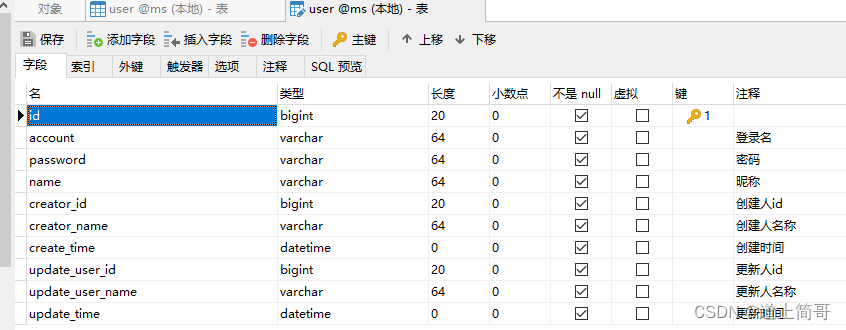
项目目录
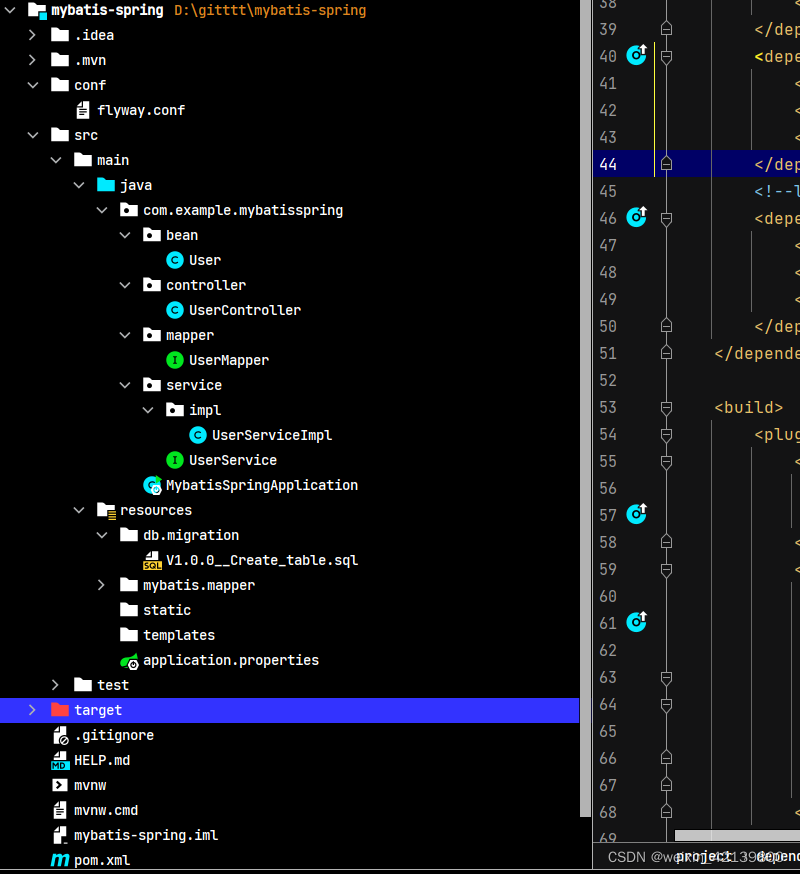
引入依赖
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
MyBatis配置
SpringBoot的application.properties添加配置
# 指resources/mybatis/mapper/下
mybatis.mapper-locations=classpath:mybatis/mapper/*.xml
# 指定POJO扫描包目录来让mybatis自动扫描到自定义的POJO
mybatis.type-aliases-package=com.example.mybatisspring.bean
# 开启驼峰命名法
mybatis.configuration.map-underscore-to-camel-case=true
创建user实体类
lombok包,提供@Data注解,自动生成set-get方法 加入依赖
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
user类如下
@Data
public class User {
private Long id;
private String account;
private String password;
private String name;
private Long creatorId;
private String creatorName;
private LocalDateTime createTime;
private Long updateUserId;
private String updateUserName;
private LocalDateTime updateTime;
}
创建mapper接口,使用@Mapper注解
package com.example.mybatisspring.mapper;
import com.example.mybatisspring.bean.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserMapper {
User getByAccountAndPassword(User user);
}
创建Mapper映射文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mybatisspring.mapper.UserMapper">
<resultMap id="BaseResultMap" type="User">
<id column="id" jdbcType="BIGINT" property="id"/>
<result column="account" jdbcType="VARCHAR" property="account"/>
<result column="password" jdbcType="VARCHAR" property="password"/>
<result column="name" jdbcType="VARCHAR" property="name"/>
</resultMap>
<sql id="Base_Column_List">
id, account, password, name
</sql>
<!--根据用户名密码查询用户信息-->
<!--application.yml 中通过 type-aliases-package 指定了实体类的为了,因此-->
<select id="getByAccountAndPassword" resultType="User">
select *
from user
where account = #{account,jdbcType=VARCHAR}
and password = #{password,jdbcType=VARCHAR}
</select>
</mapper>
- mapper 映射文件中 namespace 必须与对应的 mapper 接口的完全限定名一致。
- mapper 映射文件中 statement 的 id 必须与 mapper 接口中的方法的方法名一致
- mapper 映射文件中 statement 的 parameterType 指定的类型必须与 mapper 接口中方法的参数类型一致。
- mapper 映射文件中 statement 的 resultType 指定的类型必须与 mapper 接口中方法的返回值类型一致。
MyBatis JdbcType介绍
创建userService
package com.example.mybatisspring.service;
import com.example.mybatisspring.bean.User;
public interface UserService {
public User getByAccountAndPassword(User user);
}
创建impl实现类
package com.example.mybatisspring.service.impl;
import com.example.mybatisspring.bean.User;
import com.example.mybatisspring.mapper.UserMapper;
import com.example.mybatisspring.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service("userService")
public class UserServiceImpl implements UserService {
@Autowired
UserMapper userMapper;
@Override
public User getByAccountAndPassword(User user) {
return userMapper.getByAccountAndPassword(user);
}
}
创建controller
package com.example.mybatisspring.controller;
import com.example.mybatisspring.bean.User;
import com.example.mybatisspring.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
UserService userService;
@GetMapping("/user")
public User query(User user) {
return userService.getByAccountAndPassword(user);
}
}
参考
参考MyBatis整合spring
|