根据<狂神说>讲解做了改动:狂神说链接 1、创建业务类接口
package com.kuang.service;
public interface UserService {
public void add();
public void select();
public void update();
public void delete();
}
1、创建业务类实现类
package com.kuang.service;
import org.springframework.stereotype.Service;
public class UserServiceImpl implements UserService{
@Override
public void add() {
System.out.println("新增");
}
@Override
public void select() {
System.out.println("查看");
}
@Override
public void update() {
System.out.println("修改");
}
@Override
public void delete() {
System.out.println("删除");
}
}
3、增强AOP类
package com.kuang.ann;
import com.kuang.service.UserService;
import com.kuang.service.UserServiceImpl;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Aspect
public class AnnotationPointcut {
@Before("execution(* com.kuang.service.UserServiceImpl.*(..))")
public void beforeAop() {
System.out.println("执行了一个方法前=======");
}
@After("execution(* com.kuang.service.UserServiceImpl.*(..))")
public void afterAop() {
System.out.println("一个方法执行完毕后======");
}
@Around("execution(* com.kuang.service.UserServiceImpl.*(..))")
public Object around(ProceedingJoinPoint jp) throws Throwable {
System.out.println("环绕前");
System.out.println("签名:" + jp.getSignature());
Object proceed = jp.proceed();
System.out.println("环绕后");
System.out.println(proceed);
return proceed;
}
}
4、使用注解注入到Spring容器类
package com.kuang.config;
import com.kuang.ann.AnnotationPointcut;
import com.kuang.service.UserServiceImpl;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@EnableAspectJAutoProxy
@Configuration
public class AnnotationConfig {
@Bean
public UserServiceImpl getUser() {
return new UserServiceImpl();
}
@Bean
public AnnotationPointcut getAnno() {
return new AnnotationPointcut();
}
}
5、测试类
import com.kuang.ann.AnnotationPointcut;
import com.kuang.config.AnnotationConfig;
import com.kuang.service.UserService;
import com.kuang.service.UserServiceImpl;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AnnotationConfig.class);
UserService userService = (UserService) context.getBean("getUser");
userService.add();
}
}
注意:spring的版本号不同,会导致通知的执行顺序不同。 图片参考:https://blog.csdn.net/weixin_41979002/article/details/123572637 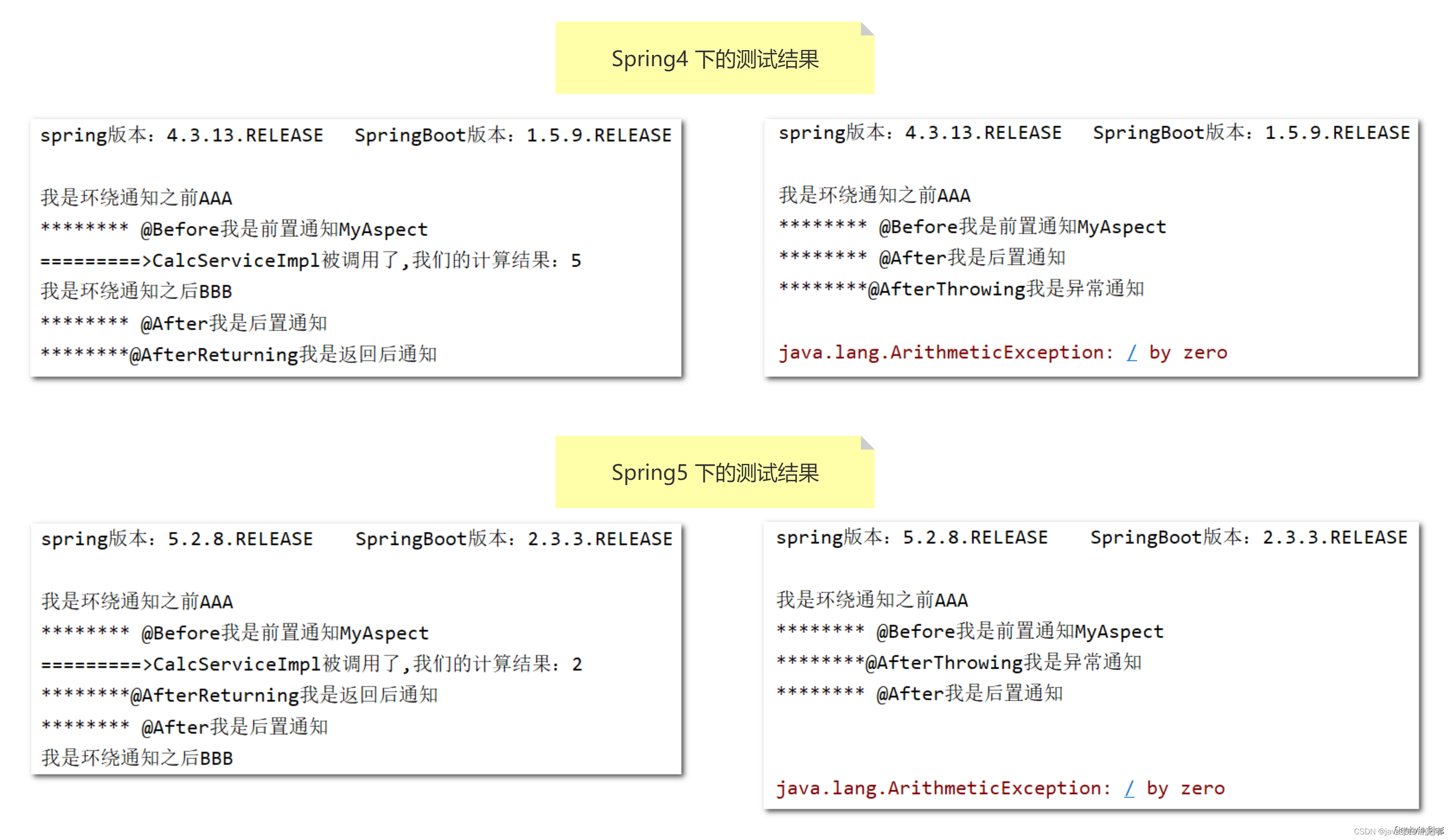
|