springboot ftp上传
一、搭建ftp服务
基础环境docker docker-compose
1、创建docker-compose.yml
version: '3'
services:
vsftpd:
image: fauria/vsftpd
hostname: vsftpd
container_name: vsftpd
restart: always
environment:
- FTP_USER=root
- FTP_PASS=123456
- PASV_ADDRESS=192.168.0.90
- PASV_MIN_PORT=21100
- PASV_MAX_PORT=21110
volumes:
- /home/uploadfile/upgrade:/home/vsftpd/root
- /etc/localtime:/etc/localtime:ro
- /usr/share/zoneinfo/Asia/Shanghai:/etc/timezone:ro
ports:
- "20:20"
- "21:21"
- "21100-21110:21100-21110"
privileged: true
2、启动ftp服务器
docker-compose up -d
3、测试连接ftp
在文件夹 地址栏输入 ftp://192.168.0.90

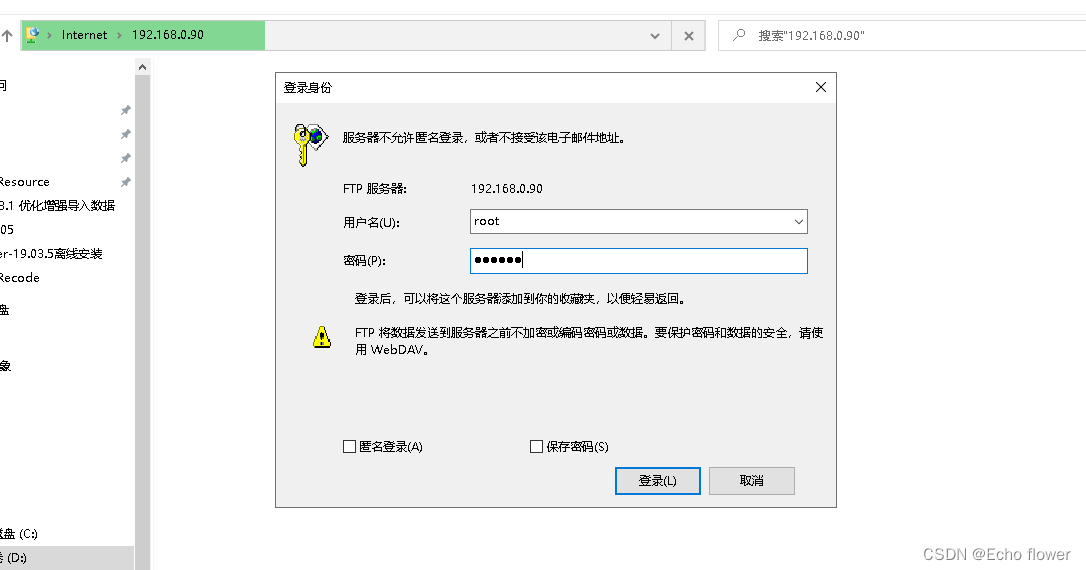
连接成功
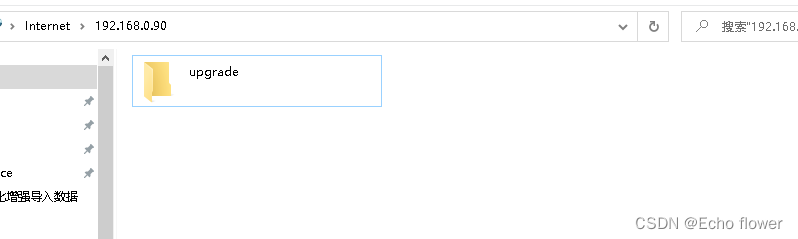
二、springboot ftp上传
1、引入pom
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
2、配置yml
magic:
export:
ftpIp: 192.168.0.90
ftpPort: 21
ftpUser: root
ftpPass: 123456
3、FtpProperties
@Data
@Component
@ConfigurationProperties("magic.export")
public class FtpProperties {
private String ftpIp;
private Integer ftpPort;
private String ftpUser;
private String ftpPass;
private String remotePath="/magic";
private String serverCharset="ISO-8859-1";
}
4、FtpUtilComponent
@Data
@Slf4j
@Component
public class FtpUtilComponent {
@Autowired
private FtpProperties ftpProperties;
private boolean connectServer(FTPClient ftpClient){
Boolean isSuccess=false;
try {
ftpClient.connect(ftpProperties.getFtpIp(),ftpProperties.getFtpPort());
isSuccess=ftpClient.login(ftpProperties.getFtpUser(),ftpProperties.getFtpPass());
} catch (IOException e) {
log.error("连接ftp服务器失败 参数 {} 异常{}", ftpProperties.toString(),e);
}
return isSuccess;
}
public boolean uploadFile(String remotePath, List<File> fileList) throws IOException {
boolean upload=true;
FTPClient ftpClient = new FTPClient();
FileInputStream fileInputStream=null;
if (connectServer(ftpClient)){
try {
ftpClient.changeWorkingDirectory(remotePath);
ftpClient.setBufferSize(1024);
ftpClient.setControlEncoding("UTF-8");
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE);
ftpClient.enterLocalPassiveMode();
for (File fileItem:fileList
) {
fileInputStream=new FileInputStream(fileItem);
ftpClient.storeFile(fileItem.getName(),fileInputStream);
}
} catch (IOException e) {
log.error("上传文件异常",e);
upload=false;
}finally {
fileInputStream.close();
ftpClient.disconnect();
}
}
return upload;
}
private static String ZN_CHARSET = "GBK";
private static String LOCAL_CHARSET = "GBK";
public boolean uploadToFtp(String remotePath, String fileName, File file) throws IOException {
boolean upload = true;
FTPClient ftpClient = new FTPClient();
if (connectServer(ftpClient)) {
try {
String newFileName = new String(fileName.getBytes(ZN_CHARSET),ftpProperties.getServerCharset());
if (FTPReply.isPositiveCompletion(ftpClient.sendCommand("OPTS UTF8", "ON"))) {
LOCAL_CHARSET = "UTF-8";
}
ftpClient.setControlEncoding(LOCAL_CHARSET);
ftpClient.changeWorkingDirectory(remotePath);
ftpClient.setBufferSize(1024);
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE);
ftpClient.enterLocalPassiveMode();
upload = ftpClient.storeFile(newFileName, new FileInputStream(file));
log.info("{} 文件导入 {} 路径 成功",fileName,remotePath);
} catch (IOException e) {
log.error("{} 文件导入 {} 路径 失败{}",fileName,remotePath,e);
upload = false;
} finally {
ftpClient.disconnect();
}
}
return upload;
}
}
5、FtpControllerTest测试类
@Test
public void demo() {
File file = new File("D:\\Dept\\README.md");
String name = file.getName();
String fileName= "中文2sas"+name.substring(name.lastIndexOf("."));
try {
ftpUtilComponent.uploadToFtp(ftpUtilComponent.getFtpProperties().getRemotePath(),fileName,file);
} catch (IOException e) {
e.printStackTrace();
}
}
6、测试成功
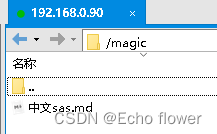 代码下载
|