/**
* pdf转图片
*
* @param pdfPath pdf地址
* @param imgPath 图片地址
* @param mergeType 1,横向拼接图片,2,纵向
*/
public static void test(String pdfPath, String imgPath, int mergeType) throws IOException {
//利用PdfBox生成图像
PDDocument pdDocument = PDDocument.load(new File(pdfPath));
PDFRenderer renderer = new PDFRenderer(pdDocument);
// 获取pdf总页数
int pdfPages = pdDocument.getNumberOfPages();
// 图片集合
BufferedImage[] bufferedImageArray = new BufferedImage[pdfPages];
for (int i = 0; i < pdfPages; i++) {
// 第一张
BufferedImage image = renderer.renderImageWithDPI(i, 200, ImageType.RGB);
// 获取图像的宽度和高度
int width = image.getWidth();
int height = image.getHeight();
// 获取计算好的rgb
int[] rgb = image.getRGB(0, 0, width, height, null, 0, width);
BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
// 写入流中
bufferedImage.setRGB(0, 0, width, height, rgb, 0, width);
// 添加到数组
bufferedImageArray[i] = bufferedImage;
}
int[][] ImageArrays = new int[bufferedImageArray.length][];
for (int i = 0; i < bufferedImageArray.length; i++) {
int width = bufferedImageArray[i].getWidth();
int height = bufferedImageArray[i].getHeight();
ImageArrays[i] = new int[width * height];
ImageArrays[i] = bufferedImageArray[i].getRGB(0, 0, width, height, ImageArrays[i], 0, width);
}
// 获取拼接尺寸
int newHeight = 0;
int newWidth = 0;
for (int i = 0; i < bufferedImageArray.length; i++) {
// 横向
if (mergeType == 1) {
newHeight = Math.max(newHeight, bufferedImageArray[i].getHeight());
newWidth += bufferedImageArray[i].getWidth();
}
// 纵向
else if (mergeType == 2) {
newWidth = Math.max(newWidth, bufferedImageArray[i].getWidth());
newHeight += bufferedImageArray[i].getHeight();
}
}
// 新图片
BufferedImage ImageNew = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_RGB);
int height_i = 0;
int width_i = 0;
for (int i = 0; i < bufferedImageArray.length; i++) {
if (mergeType == 1) {
// set新图片得RGB数据
ImageNew.setRGB(width_i, 0, bufferedImageArray[i].getWidth(), newHeight, ImageArrays[i], 0, bufferedImageArray[i].getWidth());
width_i += bufferedImageArray[i].getWidth();
} else if (mergeType == 2) {
ImageNew.setRGB(0, height_i, newWidth, bufferedImageArray[i].getHeight(), ImageArrays[i], 0, newWidth);
height_i += bufferedImageArray[i].getHeight();
}
}
// 输出图片
ImageIO.write(ImageNew, "png", new File(imgPath + File.separator + StringUtils.getRandomNumber() + ".png"));
}
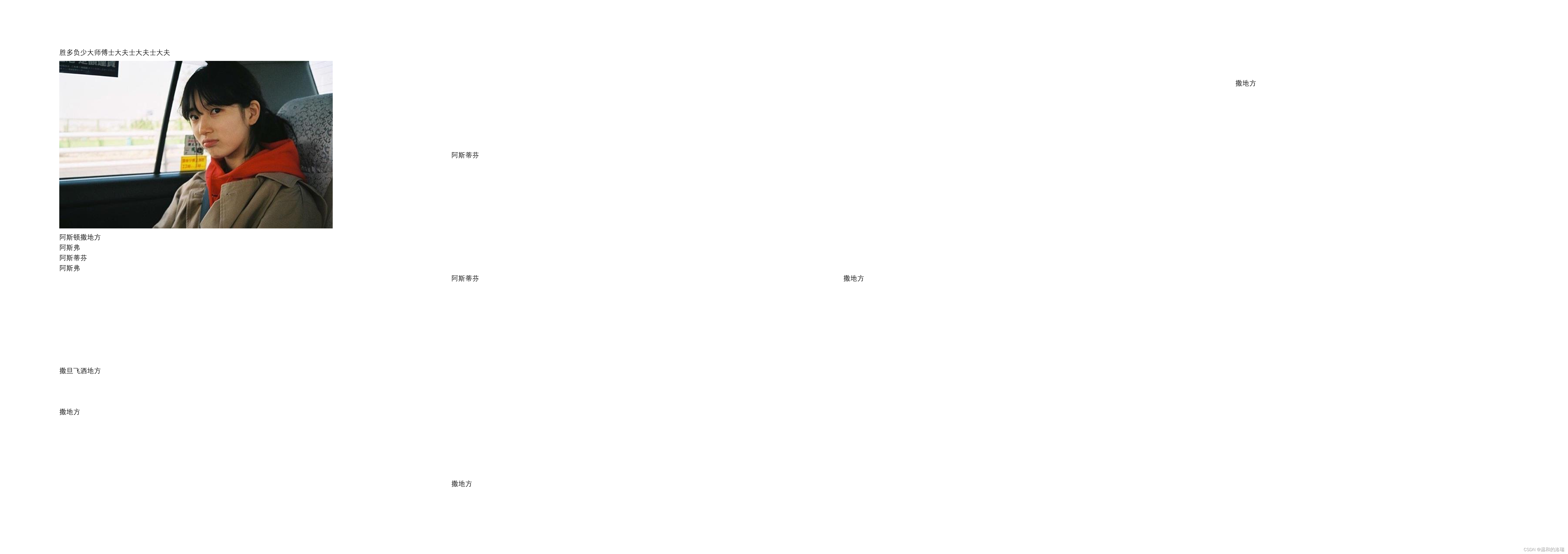
?
?
|