前言
本系列记录Java从入门开始的知识点,线程状态:创建状态、就绪状态、阻塞状态、运行状态、死亡状态。
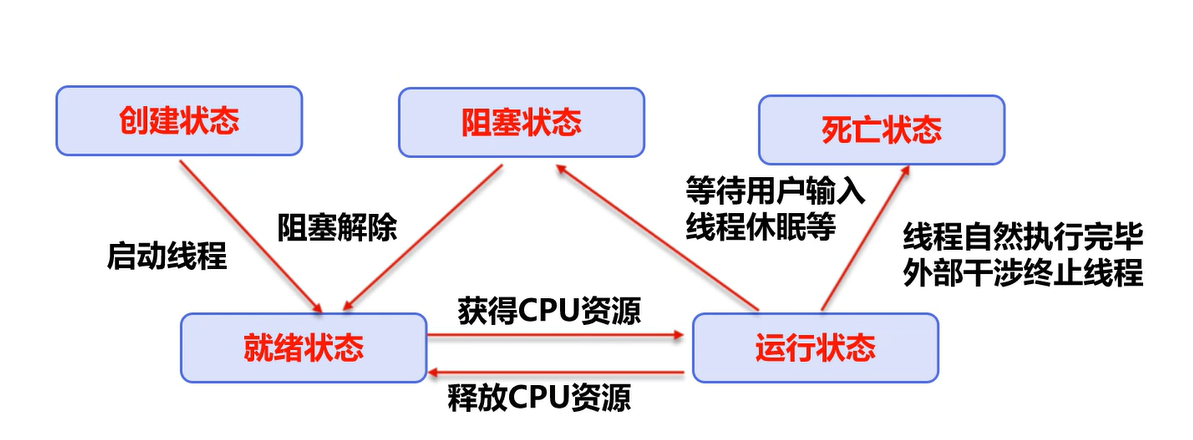 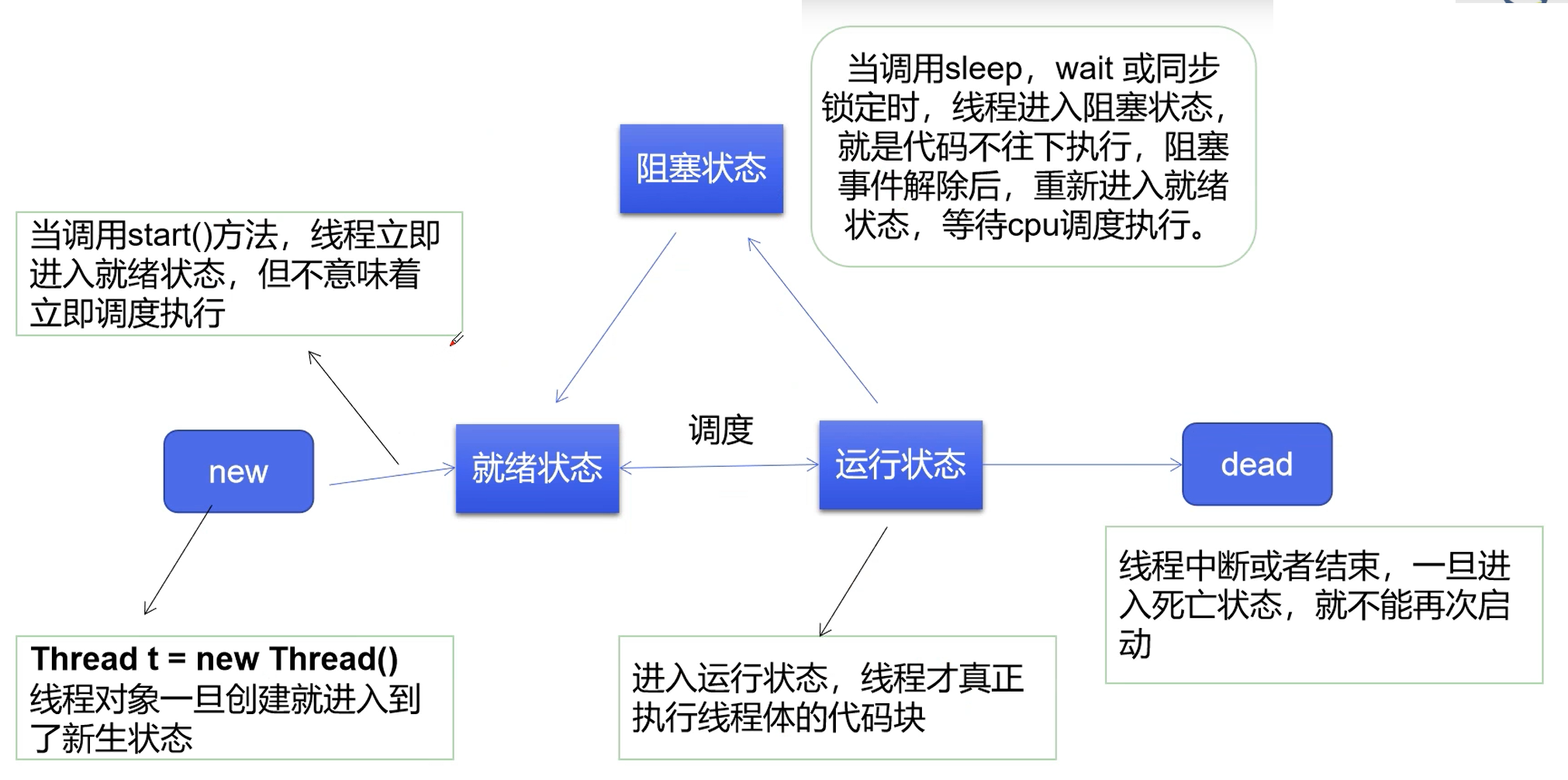
一、停止线程
- 不推荐使用JDK提供的stop()、destory()方法,已经废弃了。
- 推荐线程自己停止下来。
- 建议使用标志位进行终止变量。
public class TestStop implements Runnable{
private boolean flag = true;
@Override
public void run() {
int i=0;
while(flag){
System.out.println("run Thread" + i++);
}
}
public void stop(){
this.flag = false;
}
public static void main(String[] args) {
TestStop testStop = new TestStop();
new Thread(testStop).start();
for (int i = 0; i < 1000; i++) {
System.out.println("main i="+i);
if(i == 900){
System.out.println("线程停止");
testStop.stop();
}
}
}
}
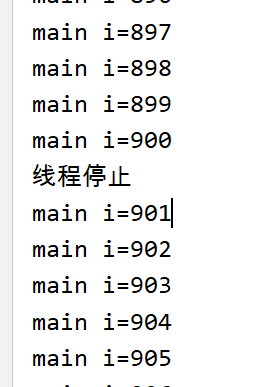
二、线程休眠
- sleep指定当前线程阻塞的毫秒数;
- sleep存在异常InterruptedException,要抛出异常;
- sleep时间达到后线程进入就绪状态;
- sleep可以模拟网络延时、倒计时等;
- 每一个对象都有一个锁,sleep不会释放锁
1、模拟网络延时
public class TestSleep implements Runnable{
private int tickeNums = 10;
@Override
public void run() {
while(true){
if(tickeNums<=0){break;}
try {
Thread.sleep(200);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+"-->拿到了第"+tickeNums--+"张票");
}
}
public static void main(String[] args) {
TestSleep ticket = new TestSleep();
new Thread(ticket,"法外狂徒张三").start();
new Thread(ticket,"不明真相李四").start();
new Thread(ticket,"实在冤枉王五").start();
}
}
这样才能发现,代码是有问题的。 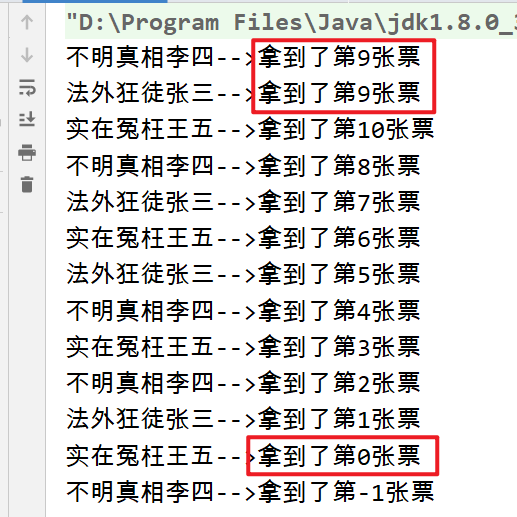
2、模拟倒计时
public class TestSleep2 {
public static void main(String[] args) {
try {
tenDown();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void tenDown() throws InterruptedException{
int num = 10;
while(true){
Thread.sleep(1000);
System.out.println(num--);
if(num<=0){
break;
}
}
}
}
3、获取当时系统时间
public class TestSleep3 {
public static void main(String[] args) {
Date startTime = new Date(System.currentTimeMillis());
while (true){
try {
Thread.sleep(1000);
System.out.println(new SimpleDateFormat("HH:mm:ss").format(startTime));
startTime = new Date(System.currentTimeMillis());
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
三、线程礼让
- 礼让线程,让当前正在执行的线程暂停,但不阻塞。
- 将线程从运行状态转为就绪状态。
- 礼让不一定成功。
public class TestYield {
public static void main(String[] args) {
MyYield myYield = new MyYield();
new Thread(myYield,"小红").start();
new Thread(myYield,"小明").start();
}
}
class MyYield implements Runnable{
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+"线程开始执行");
Thread.yield();
System.out.println(Thread.currentThread().getName()+"线程停止执行");
}
}
礼让成功: 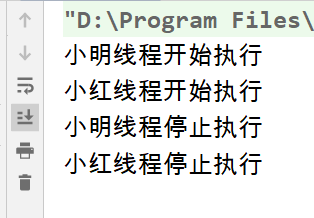 礼让失败: 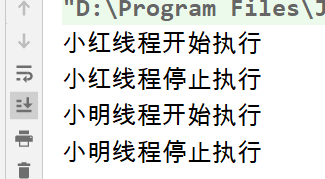
四、Join
理解成线程插队
public class TestJoin implements Runnable{
@Override
public void run() {
for (int i=0;i<1000;i++) {
System.out.println("线程VIP来了" + i);
}
}
public static void main(String[] args) throws InterruptedException{
TestJoin testJoin = new TestJoin();
Thread thread = new Thread(testJoin);
for (int i = 0; i < 1000; i++) {
if(i == 500){
thread.start();
thread.join();
}
System.out.println("main:"+i);
}
}
}
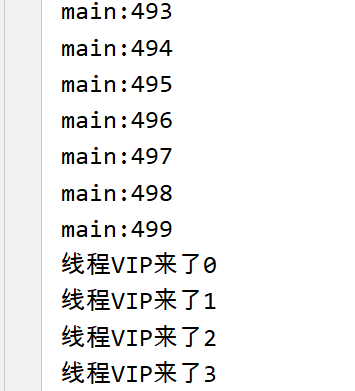
五、监测线程状态
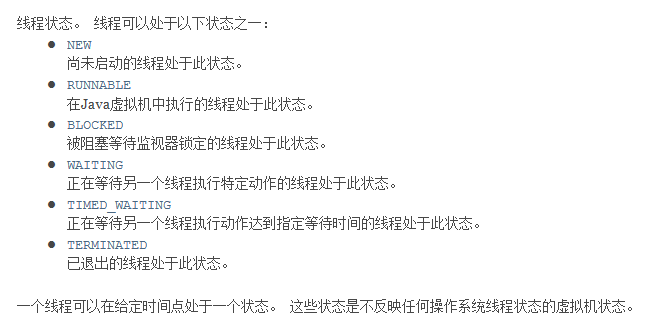
public class TestState {
public static void main(String[] args) throws InterruptedException{
Thread thread = new Thread(()->{
for (int i = 0; i < 5; i++) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(".........................");
});
Thread.State state = thread.getState();
System.out.println(state);
thread.start();
state = thread.getState();
System.out.println(state);
while (state != Thread.State.TERMINATED){
Thread.sleep(100);
state = thread.getState();
System.out.println(state);
}
}
}
六、线程优先级
取值范围 1~10。
public class TestPriority {
public static void main(String[] args) {
System.out.println(Thread.currentThread().getName()+"-->"+Thread.currentThread().getPriority());
MyPriority myPriority = new MyPriority();
Thread t1 = new Thread(myPriority);
Thread t2 = new Thread(myPriority);
Thread t3 = new Thread(myPriority);
Thread t4 = new Thread(myPriority);
Thread t5 = new Thread(myPriority);
Thread t6 = new Thread(myPriority);
t1.start();
t2.setPriority(1);
t2.start();
t3.setPriority(3);
t3.start();
t4.setPriority(Thread.MAX_PRIORITY);
t4.start();
t5.setPriority(8);
t5.start();
t6.setPriority(Thread.MIN_PRIORITY);
t6.start();
}
}
class MyPriority implements Runnable{
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+"-->"+Thread.currentThread().getPriority());
}
}
七、守护线程
- 线程分为
用户线程 和守护线程 ; - 虚拟机必须保证用户线程执行完毕;
- 虚拟机不用等待守护线程执行完毕;
- 如后台记录操作日志,监控内存,垃圾回收等待。
public class TestDaemon {
public static void main(String[] args) {
God god = new God();
You you = new You();
Thread thread = new Thread(god);
thread.setDaemon(true);
thread.start();
new Thread(you).start();
}
}
class God implements Runnable{
@Override
public void run() {
while (true){
System.out.println("上帝一直在");
}
}
}
class You implements Runnable{
@Override
public void run() {
for (int i = 0; i < 36500; i++) {
System.out.println("要开心的活着");
}
System.out.println("============goodbye world!===============");
}
}
|