SpringBoot 操作数据: Spring Data 也是与之齐名的项目。
说明:在 springboot 2.X 之后,原来使用的 Jedis 被替换成了 lettuce
源码:
public class RedisAutoConfiguration {
@Bean
@ConditionalOnMissingBean(name = "redisTemplate")
@ConditionalOnSingleCandidate(RedisConnectionFactory.class)
public RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<Object, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
return template;
}
@Bean
@ConditionalOnMissingBean
@ConditionalOnSingleCandidate(RedisConnectionFactory.class)
public StringRedisTemplate stringRedisTemplate(RedisConnectionFactory redisConnectionFactory) {
return new StringRedisTemplate(redisConnectionFactory);
}
}
整合测试
1、导入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2、配置文件
# 配置 Redis
spring.redis.host=192.168.40.25
spring.redis.port=6379
# 源码中 Jedis 根本没生效,不能使用 Jedis 的配置
# spring.redis.jedis.pool.enabled=true
# 使用 lettuce 的配置
# spring.redis.lettuce.pool.enabled=true
3、使用测试
@SpringBootTest
class Redis02SpringbootApplicationTests {
@Autowired
private RedisTemplate redisTemplate;
@Test
void contextLoads() {
redisTemplate.opsForValue().set("key", "Hello redis!");
System.out.println(redisTemplate.opsForValue().get("key"));
}
}
源码中的序列化配置:
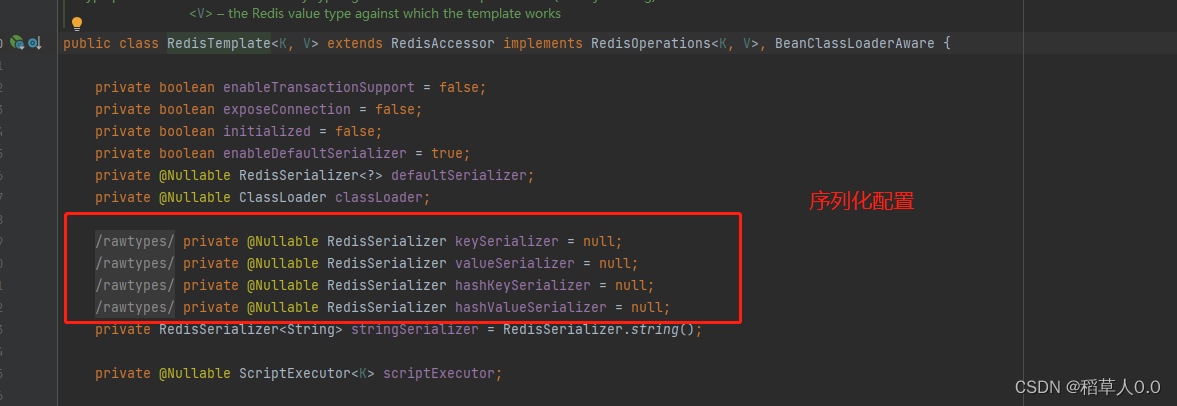
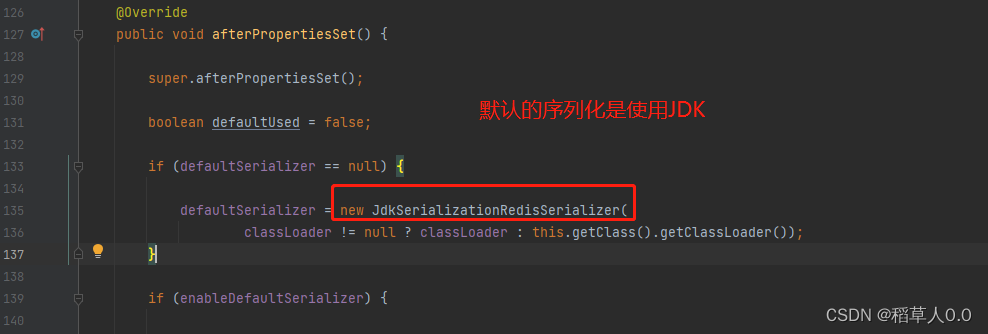
默认的序列化是使用 JDK 序列化,我们可能使用 JSON 来序列化!
自定义 redisTemplate
测试
@Test
public void test() throws JsonProcessingException {
User user = new User();
user.setName("mianbao");
user.setAge(18);
redisTemplate.opsForValue().set("user", user);
System.out.println(redisTemplate.opsForValue().get("user"));
}
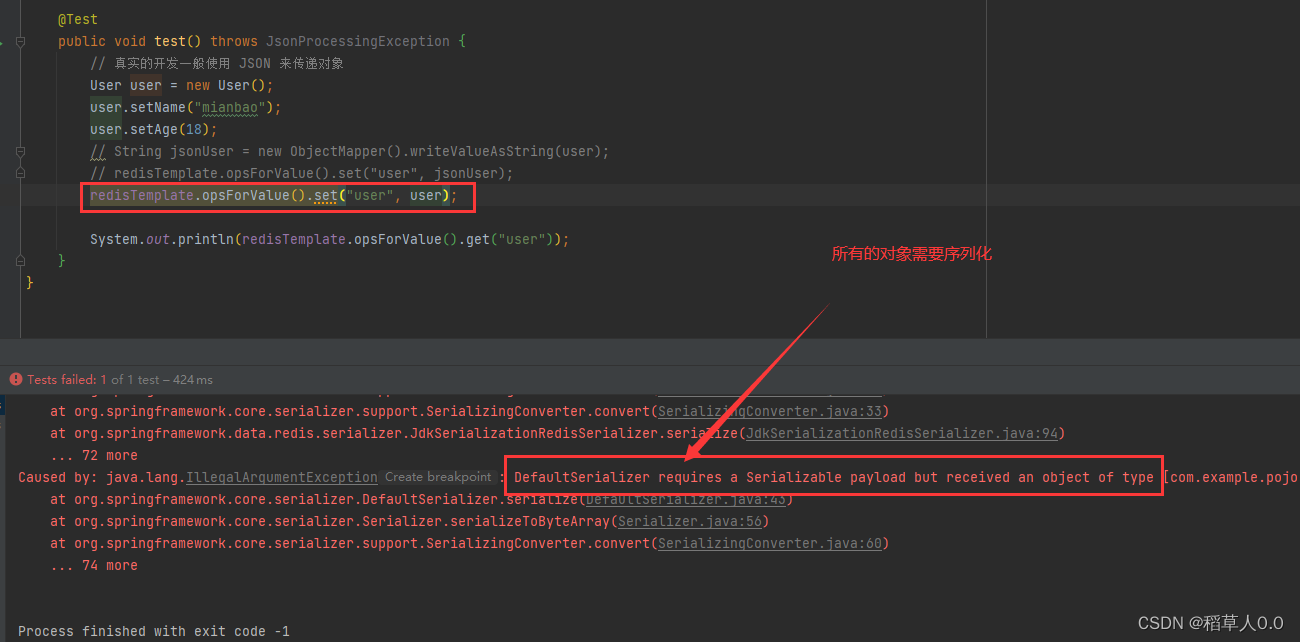
对象需要实现Serializable 接口
public class User implements Serializable {
private String name;
private int age;
}
序列化接口的实现类:
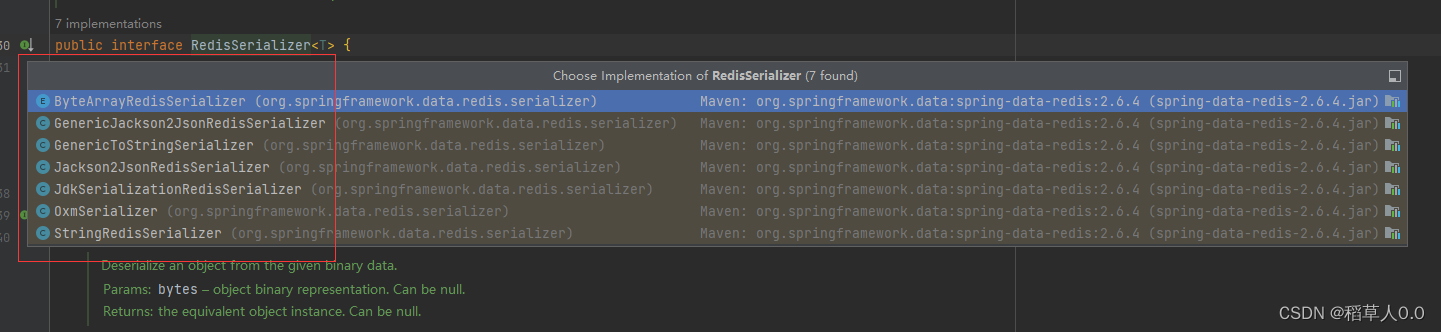
自定义的RedisTempalte
@Configuration
public class RedisConfig {
@Bean
@SuppressWarnings("all")
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.activateDefaultTyping(LaissezFaireSubTypeValidator.instance,ObjectMapper.DefaultTyping.NON_FINAL, JsonTypeInfo.As.WRAPPER_ARRAY);
jackson2JsonRedisSerializer.setObjectMapper(om);
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
template.setKeySerializer(stringRedisSerializer);
template.setHashKeySerializer(stringRedisSerializer);
template.setValueSerializer(jackson2JsonRedisSerializer);
template.setHashValueSerializer(jackson2JsonRedisSerializer);
template.afterPropertiesSet();
return template;
}
}
使用默认的 redisTemplate User在 redis中的内容
127.0.0.1:6379> keys *
1) "\xac\xed\x00\x05t\x00\x04user"
使用自定义的 redisTemplate User在 redis中的内容
127.0.0.1:6379> keys *
1) "user"
|