1.请求域request
1.1pom.xml
<!-- web场景jar包 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- 热部署 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<!-- 配置文件自定义bean数据提示等 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<!-- get()、set()、toString、slf4j等 -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<!-- 测试包@Test -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- 将js以jar包形式导入项目,比如:[http://localhost:8081/webjars/**jquery/3.5.1/jquery.js**]-->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.5.1</version>
</dependency>
1.2controller
@Controller
public class RequestController {
//获取request域中的属性数据,HttpServletRequest设置数值,RequestAttribute注解获取数值。通常前端通过el表达式获取
@GetMapping("/goto")
public String goToPage(HttpServletRequest httpServletRequest){
httpServletRequest.setAttribute("msg","成功了..");
httpServletRequest.setAttribute("encoding",200);
return "forward:/success";
}
@ResponseBody
@GetMapping("/success")
public HashMap getRequest(@RequestAttribute("msg") String msg,
@RequestAttribute("encoding") String encoding,
HttpServletRequest httpServletRequest){
Object httpMsg = httpServletRequest.getParameter("msg");
Object httpEncoding = httpServletRequest.getParameter("encoding");
System.out.println(httpMsg+" || "+httpEncoding);
HashMap<String,Object> map = new HashMap<>();
map.put("msg",msg);
map.put("encoding",encoding);
return map;
}
}
forward:/success:重定向路径并返回告知浏览器success的路径
HttpServletRequest:设置请求域中属性数值
@RequestAttribute:获取请求域中属性的数值
1.3接口测试结果展示
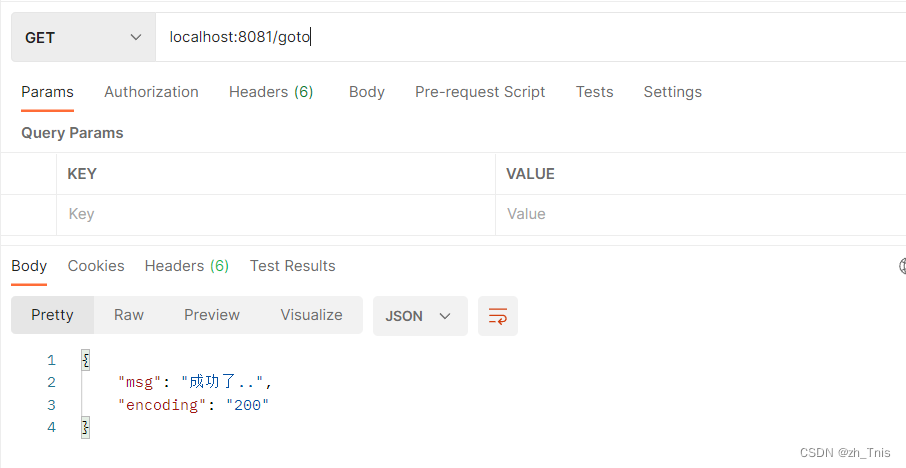
?
2.路径域
(1).路径传参变量值
1).controller
@GetMapping("/car/{id}/onwer/{name}")
public Map getCarResult(@PathVariable("id") Integer id,
@PathVariable("name") String name,
@PathVariable HashMap<String,String> hm,
@RequestHeader("User-Agent") String userAgent,
@RequestHeader HashMap<String,String> ua,
@RequestParam("age") Integer age,
@RequestParam("inters") List<String> inters,
//HashMap特性,无序,key唯一
@RequestParam HashMap<String,String> parmes
// ,@CookieValue("_ga") String _ga, //记得浏览器别屏蔽cookie
// @CookieValue("_ga") Cookie cookie
){
HashMap<String,Object> map = new HashMap<>();
map.put("id",id);
map.put("name",name);
map.put("hm",hm);
map.put("userAgent",userAgent);
map.put("ua",ua);
// System.out.println(cookie.getName()+" || "+cookie.getValue());
return map;
}
localhost:8080/car/3/onwer/zhangsan/?age=18&inters=basketball&inters=vallball @PathVariable:获取路径变量数据。 @RequestHeader:获取请求头参数数据。 @RequestParam:获取请求参数数据。 @CookieValue:获取cookie值。
2)接口测试结果展示
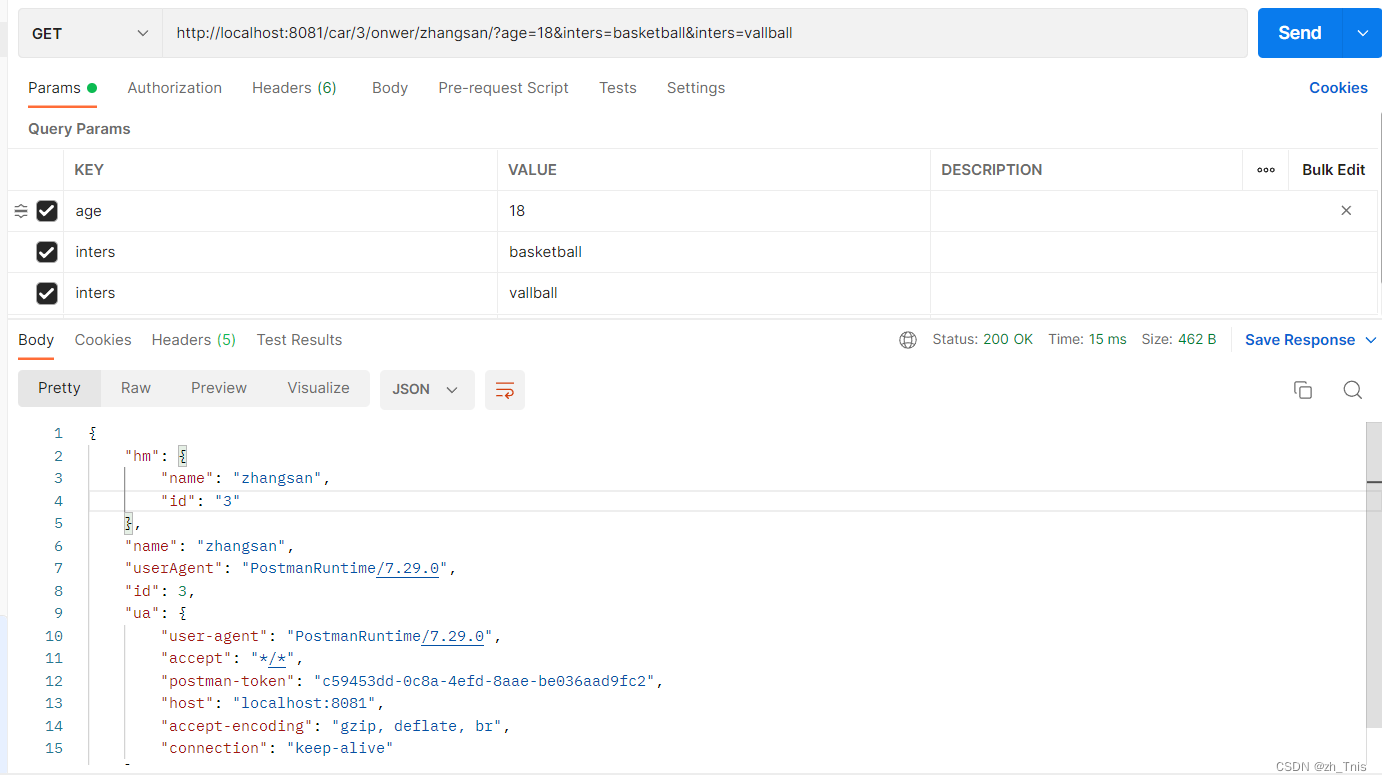
(2).表单提交
1).controller
//from表单提交,获取表单数据。RequestBody
@PostMapping("/save")
public Map getPostMethod(@RequestBody String content){
HashMap<String,Object> map = new HashMap<>();
map.put("content",content);
return map;
}
@PostMapping:post请求
@RequestBody:接收传递的请求体,json字符串中的全部数据。通常与@RequestParam注解使用
2)接口测试结果展示
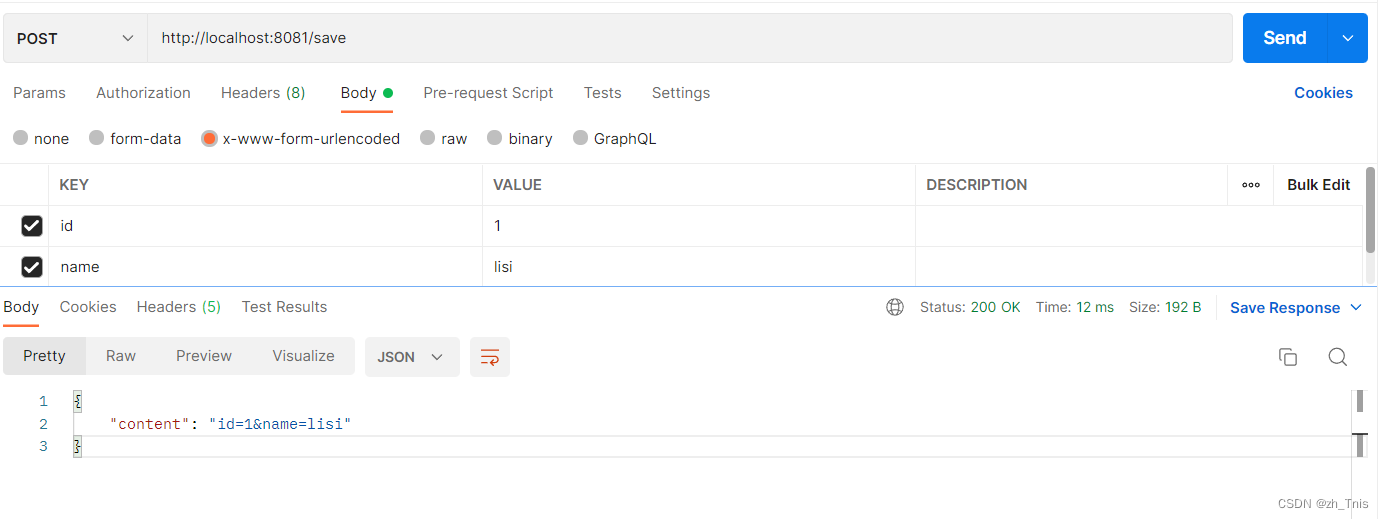
(3).矩阵变量
?1)config
public class WebConfig implements WebMvcConfigurer {
//方法2
@Bean
public WebMvcConfigurer webMvcConfigurer(){
return new WebMvcConfigurer() {
@Override
public void configurePathMatch(PathMatchConfigurer configurer) {
UrlPathHelper urlPathHelper = new UrlPathHelper();
//设置为不移除分号后边的内容,矩阵变量生效
urlPathHelper.setRemoveSemicolonContent(false);
configurer.setUrlPathHelper(urlPathHelper);
}
};
}
}
SpringBoot默认禁用使用矩阵变量的功能,需要手动开启。定义配置类,注入开启了矩阵变量使用的组件bean到容器中。
2)controller
第一种传参方式
//矩阵变量语法,把cookie的值以矩阵变量方式进行传递
http://localhost:8081/car/sell;brand=byd;color=blue,red,yellow
//矩阵变量,第一种传参方式
@GetMapping("car/{path}")
public Map getMetrixVariable(@MatrixVariable("brand") String brand,
@MatrixVariable("color")List<String> color,
@PathVariable("path") String path){
HashMap<String,Object> map = new HashMap<>();
map.put("brand",brand);
map.put("color",color);
map.put("path",path);
return map;
}
第二种传参方式
http://localhost:8081/car/zhangsan;age=10/lisi;age=20
//矩阵变量,第二种传参方式
@GetMapping("car/{boss}/{emp}")
public Map getMoreMetrixVariable(//获取路径boss下的age值
@MatrixVariable(value = "age",pathVar = "boss") Integer bossAge,
//获取路径emp下的age值
@MatrixVariable(value = "age",pathVar = "emp") Integer empAge){
HashMap<String,Object> map = new HashMap<>();
map.put("bossAge",bossAge);
map.put("empAge",empAge);
return map;
}
@MatrixVariable:矩阵变量获取数值
|