持续学习&持续更新中…
守破离
【Java从零到架构师第③季】【48】SpringBoot-Swagger
接口文档—Swagger
https://swagger.io/
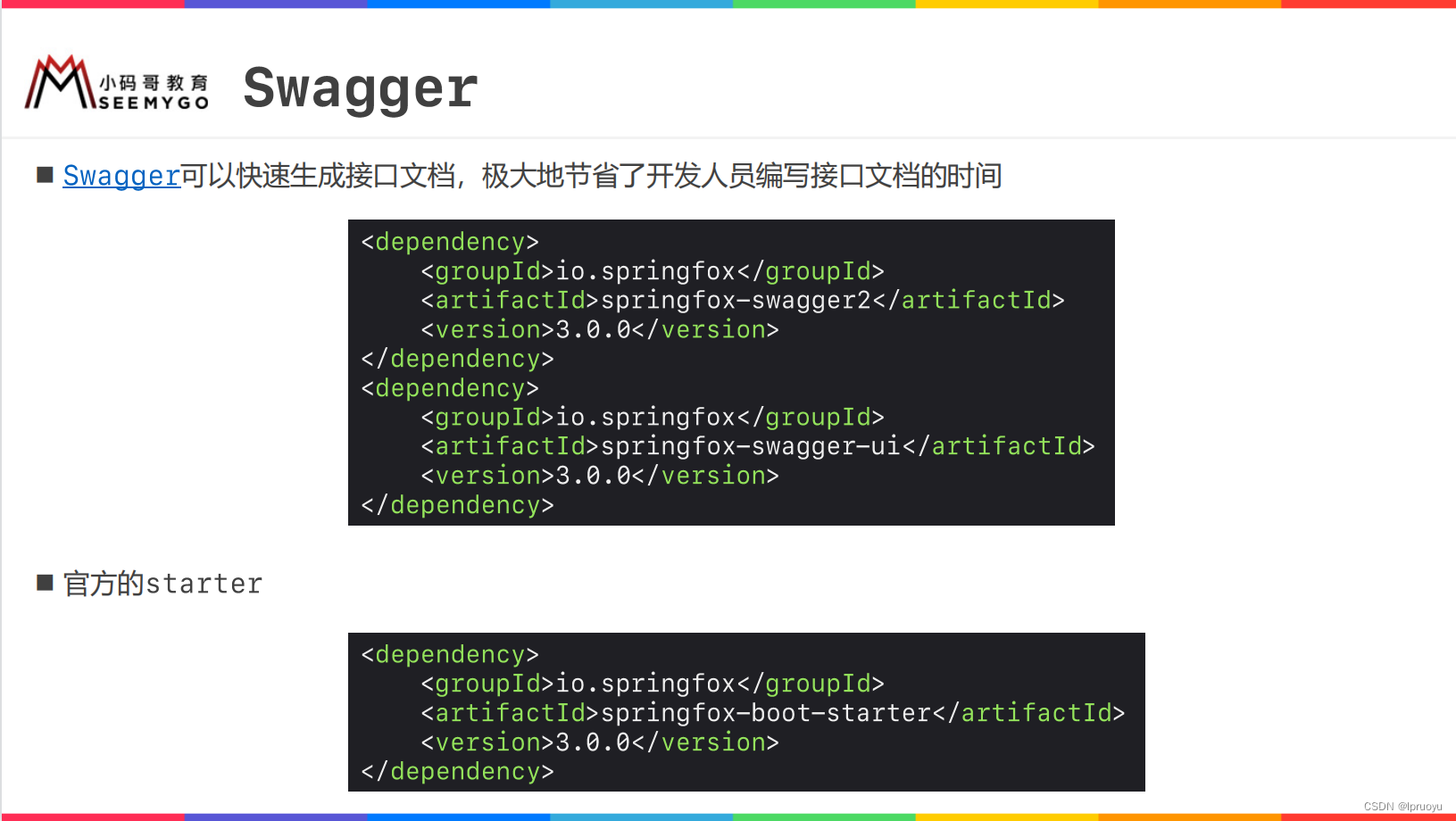
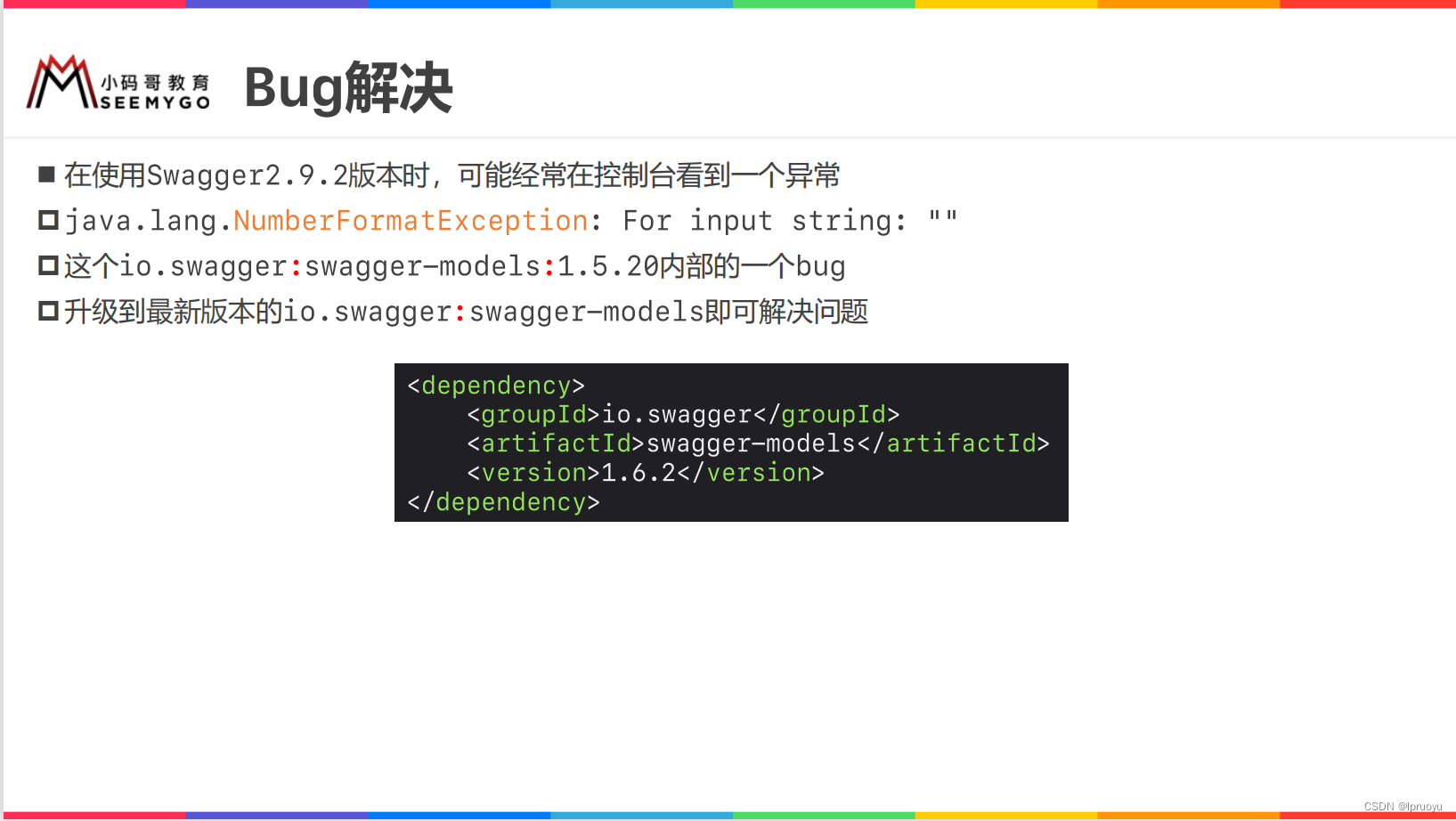
<dependency>
<groupId>io.swagger</groupId>
<artifactId>swagger-models</artifactId>
<version>1.6.2</version>
</dependency>
基本使用
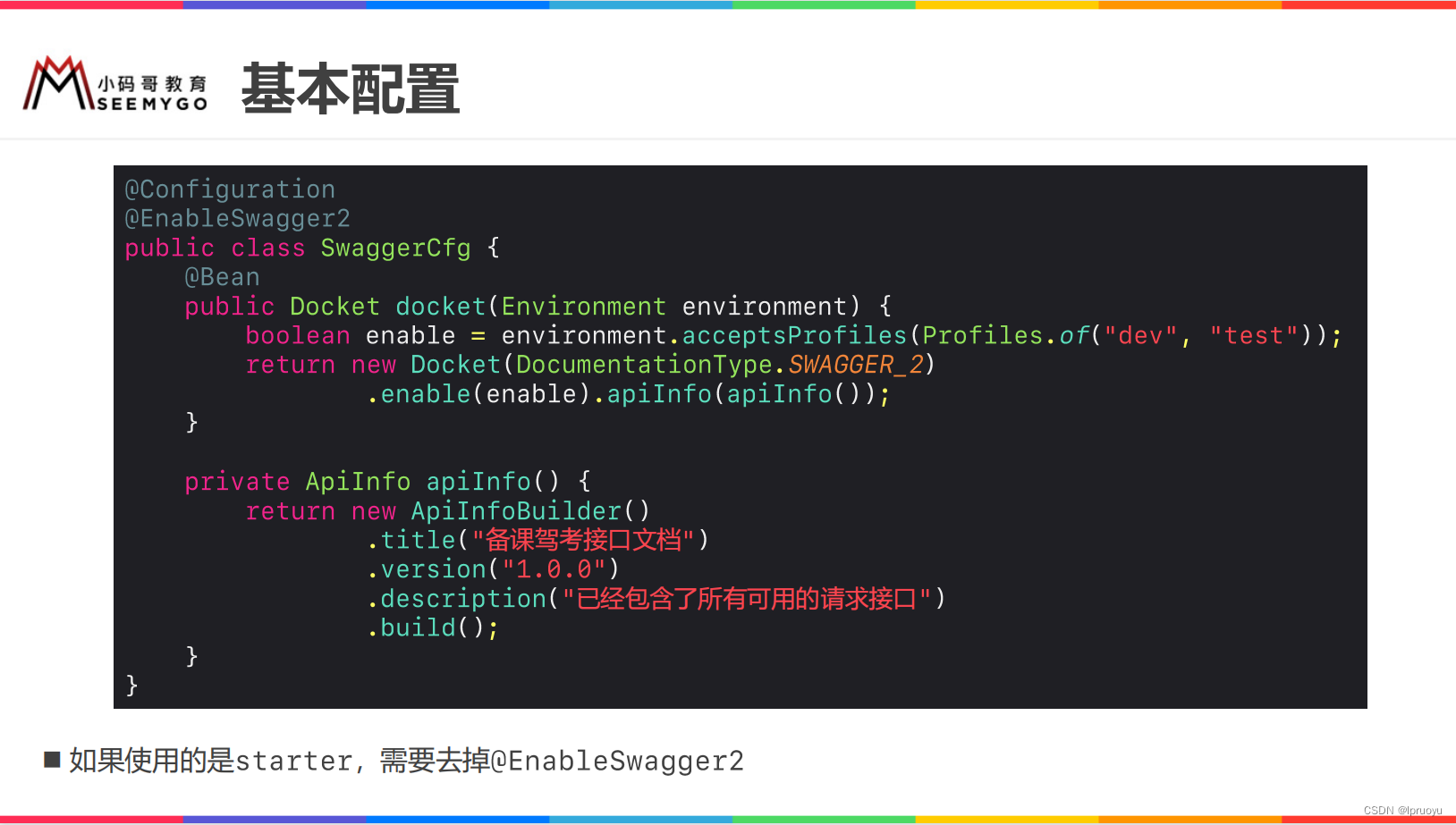
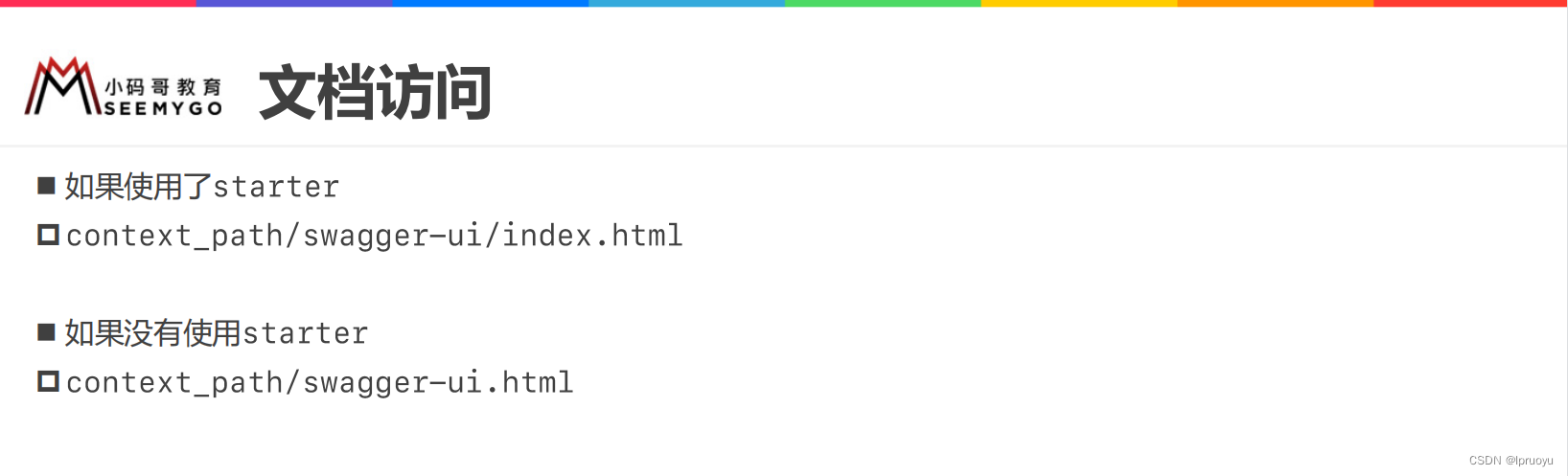
不使用starter
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("LP驾考")
.description("这是一份详细的API接口文档")
.version("1.0.0")
.build();
}
}
使用starter(Swagger3.0)
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
@Configuration
@EnableOpenApi
public class SwaggerConfig {
@Autowired
private Environment environment;
@Bean
public Docket docket() {
return new Docket(DocumentationType.OAS_30)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("LP驾考")
.description("这是一份详细的API接口文档")
.version("1.0.0")
.build();
}
}
API选择
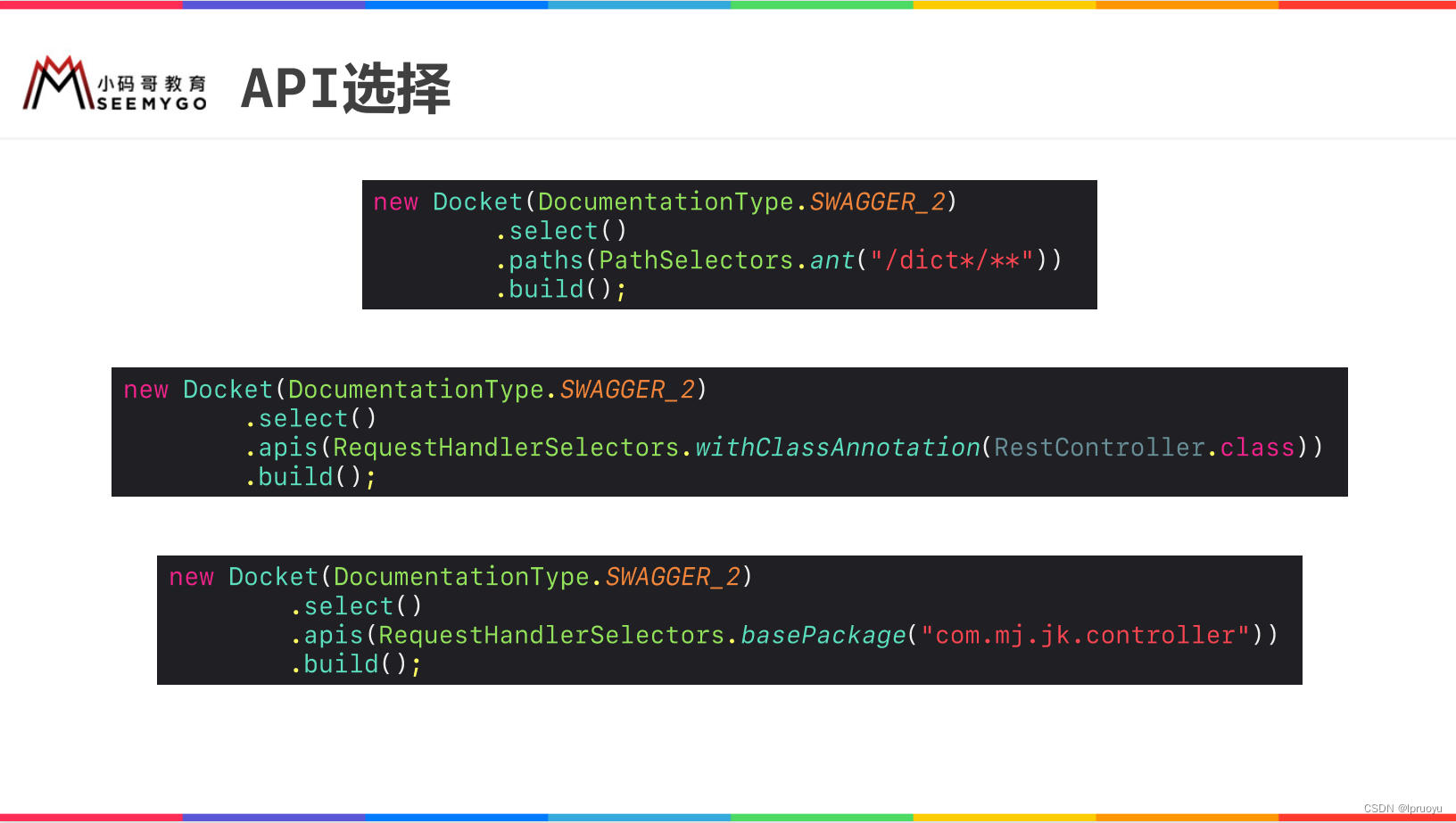
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo())
.select()
.paths(PathSelectors.ant("/dict*/**"))
.build();
}
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo())
.select()
.paths(PathSelectors.regex("/dict.+"))
.build();
}
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("programmer.lp.jk.controller"))
.build();
}
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.withClassAnnotation(RestController.class))
.build();
}
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.withMethodAnnotation(GetMapping.class))
.build();
}
忽略参数
如果我们想在构建文档时忽略Controller中方法的某些参数:
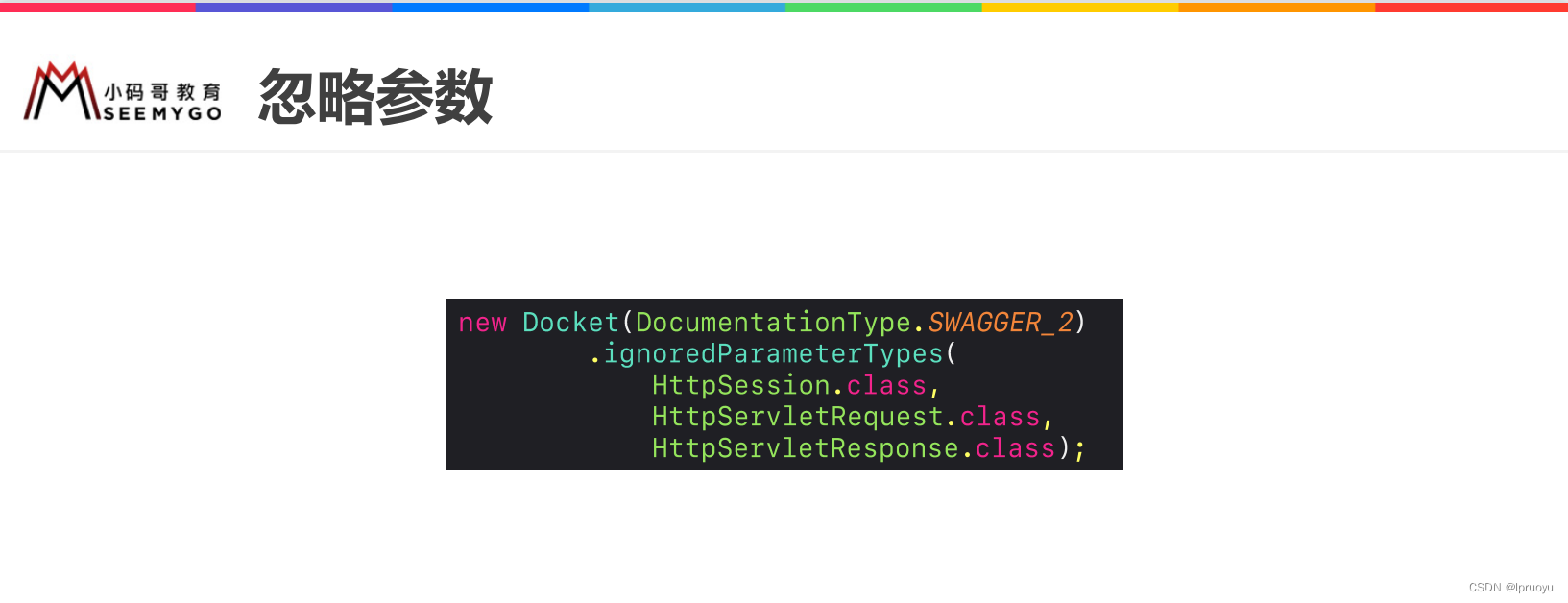
@Bean
public Docket docket(Environment environment) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(!environment.acceptsProfiles(Profiles.of("prd")))
.apiInfo(apiInfo())
.ignoredParameterTypes(
HttpSession.class,
HttpServletRequest.class,
HttpServletResponse.class
)
.select()
.apis(RequestHandlerSelectors.basePackage("programmer.lp.jk.controller"))
.build();
}
分组
有多少个分组就得有多少个Docket对象:
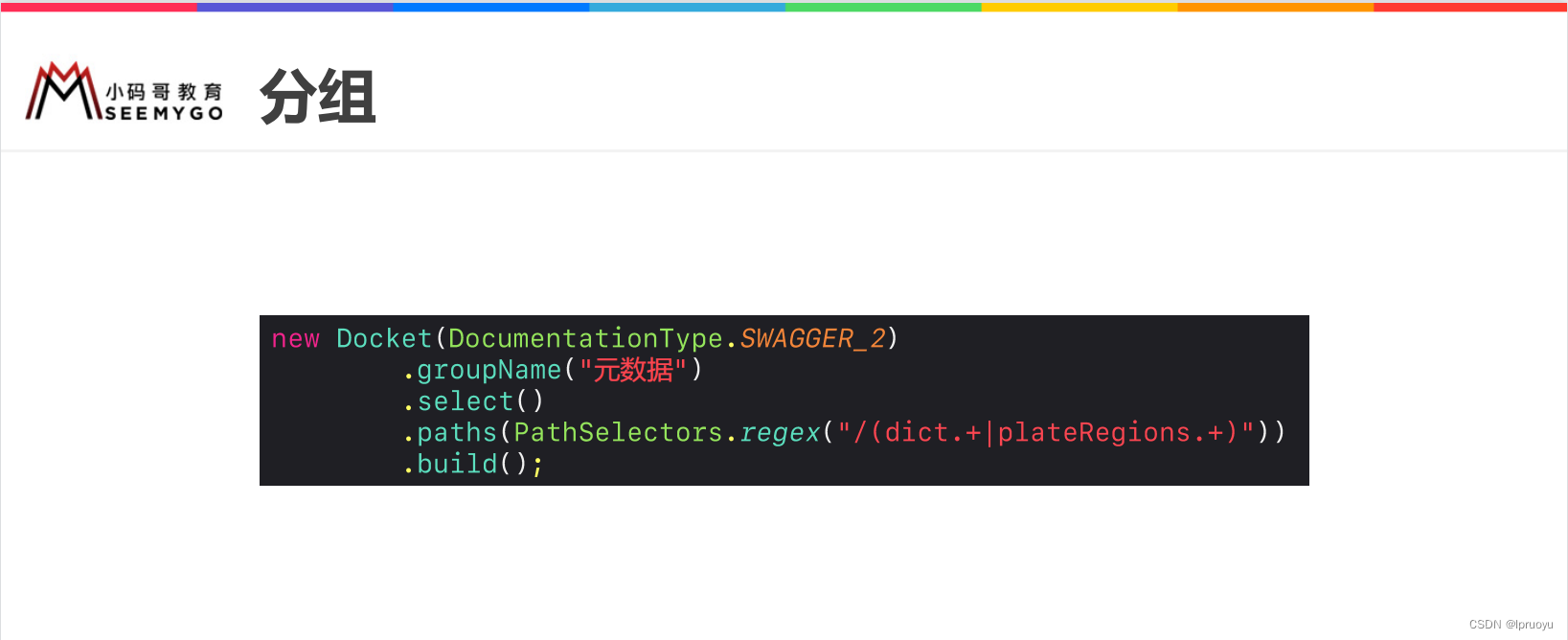
@Component
@Data
@ConfigurationProperties("swagger")
public class SwaggerProperties {
String title;
String version;
String name;
String url;
String email;
List<String> ignoredParameterTypes;
}
swagger:
email: xxxx@foxmail.com
ignored-parameter-types:
- javax.servlet.http.HttpSession
- javax.servlet.http.HttpServletRequest
- javax.servlet.http.HttpServletResponse
name: lpruoyu
url: https://blog.csdn.net/weixin_44018671
version: 1.0.0
title: LP驾考
@Configuration
@EnableSwagger2
public class SwaggerConfig implements InitializingBean {
@Bean
public Docket examDocket() {
return getDocket("考试",
"包含模块:考场、科1科4、科2科3",
"/exam.*");
}
@Bean
public Docket dictDocket() {
return getDocket("数据字典",
"包含模块:数据字典类型、数据字典条目、省份、城市",
"/(dict.*|plate.*)");
}
@Autowired
private SwaggerProperties swaggerProperties;
@Autowired
private Environment environment;
private Class[] ignoredParameterTypes;
private boolean enable;
private Class[] getIgnoredParameterTypes() {
final List<Class<?>> iptClasses = new ArrayList<>();
swaggerProperties.getIgnoredParameterTypes().forEach(v -> {
try {
iptClasses.add(Class.forName(v));
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
});
return iptClasses.toArray(new Class[iptClasses.size()]);
}
@Override
public void afterPropertiesSet() throws Exception {
ignoredParameterTypes = getIgnoredParameterTypes();
enable = !environment.acceptsProfiles(Profiles.of("prd"));
}
private Docket getDocket(String groupName, String description, String pathRegex) {
return new Docket(DocumentationType.SWAGGER_2)
.enable(enable)
.groupName(groupName)
.ignoredParameterTypes(ignoredParameterTypes)
.select()
.paths(PathSelectors.regex(pathRegex))
.build()
.apiInfo(apiInfo(swaggerProperties.getTitle() + groupName, description));
}
private ApiInfo apiInfo(String title, String description) {
return new ApiInfoBuilder()
.title(title)
.description(description)
.version(swaggerProperties.getVersion())
.contact(new Contact(
swaggerProperties.getName(),
swaggerProperties.getUrl(),
swaggerProperties.getEmail()))
.build();
}
}
参数类型
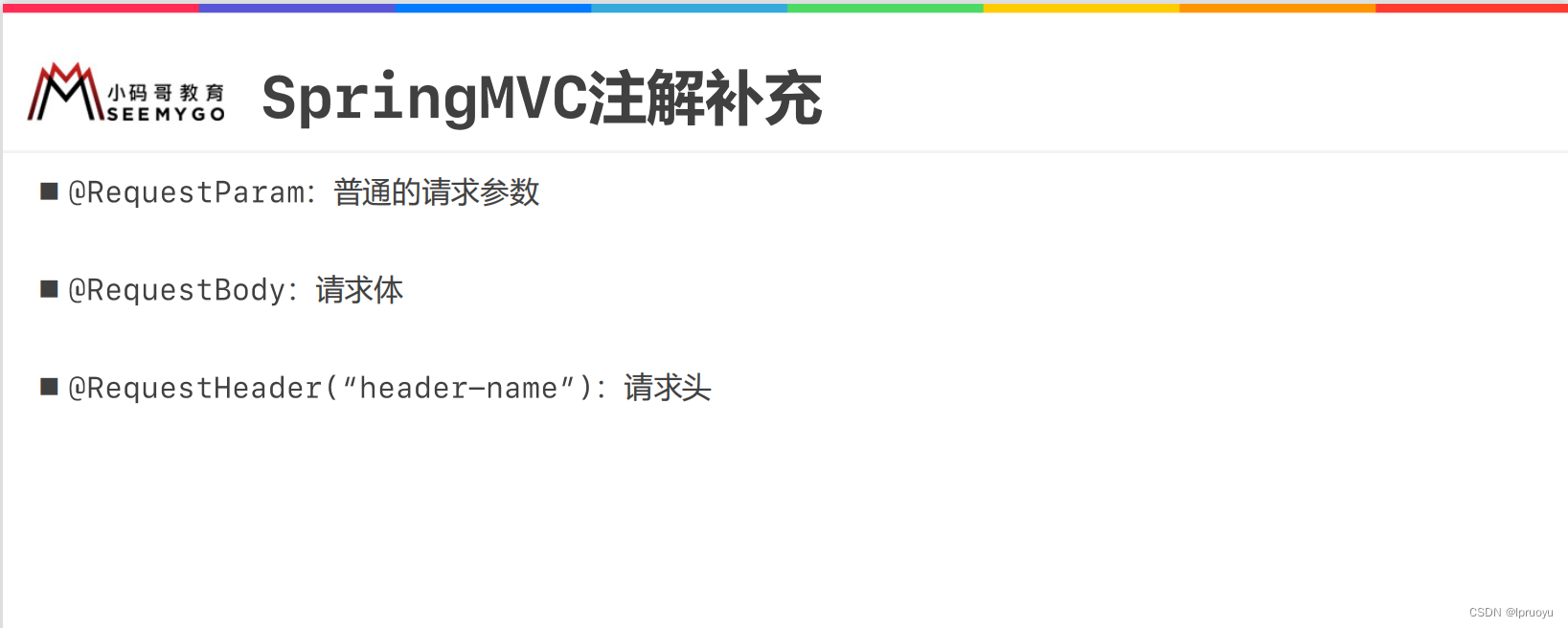
参数类型有:
- query 对应 @RequestParam 类型的参数
- body 对应 @RequestBody 类型的参数
- header 对应 @RequestHeader 类型的参数
全局参数
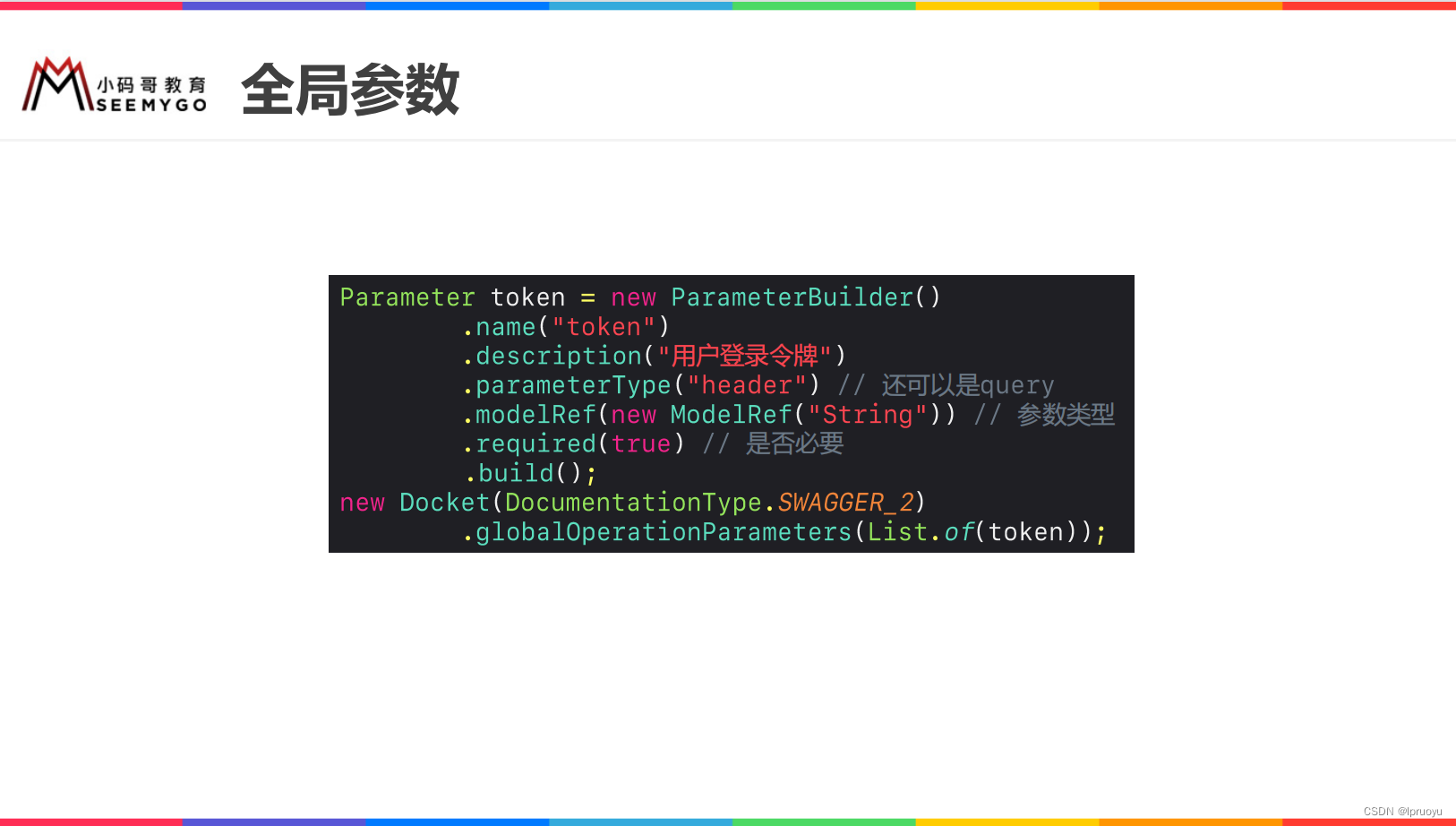
使用Swagger-boot-starter 3.0:
public Docket basicDocket() {
RequestParameter tokenParam = new RequestParameterBuilder()
.name(TokenFilter.TOKEN_HEADER)
.description("用户登录令牌")
.in(ParameterType.HEADER)
.build();
return new Docket(DocumentationType.SWAGGER_2)
.globalRequestParameters(List.of(tokenParam))
.enable(true)
.ignoredParameterTypes(
HttpSession.class,
HttpServletRequest.class,
HttpServletResponse.class);
}
常用注解
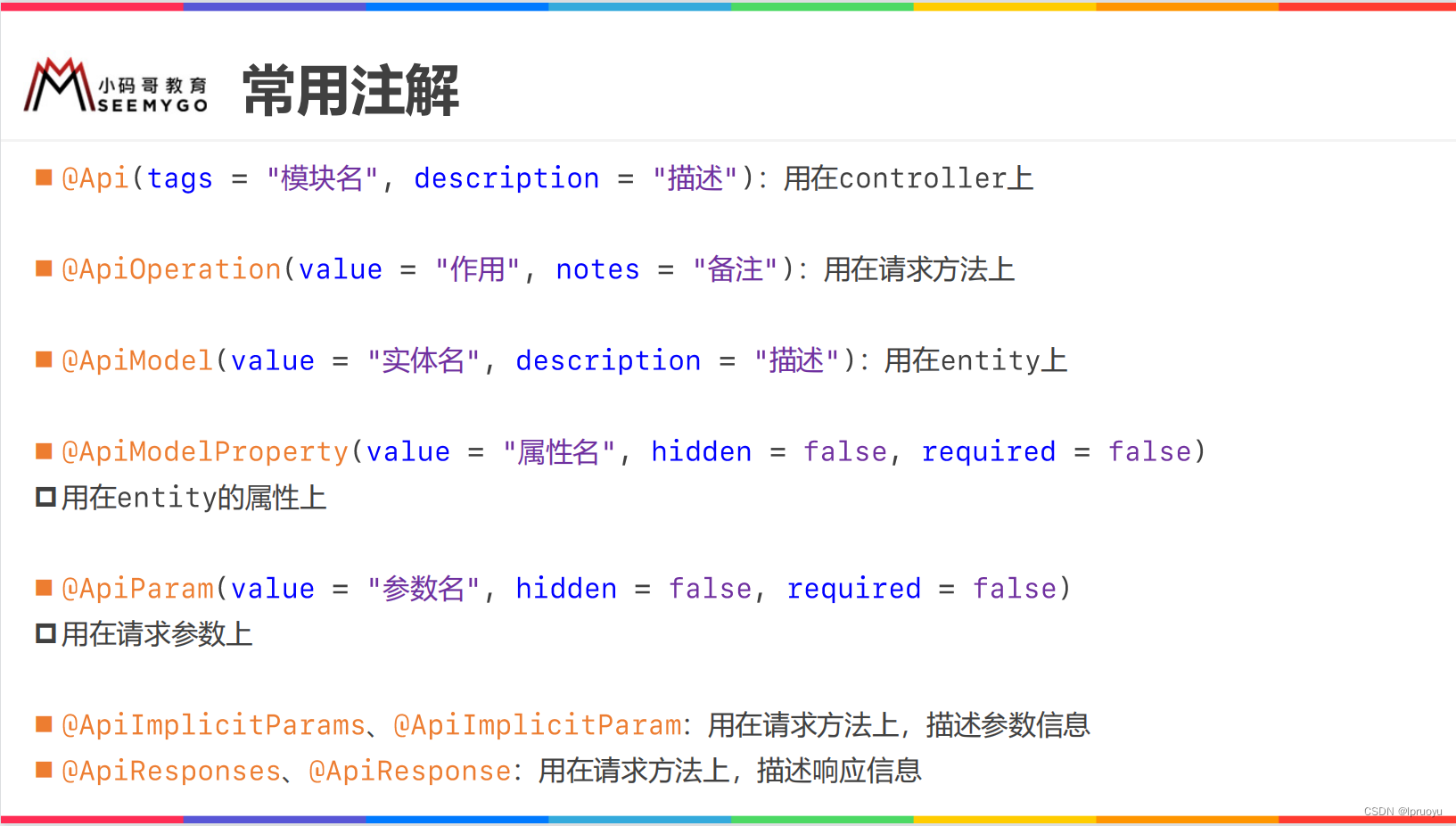
swagger-bootstrap-ui
Swagger默认的接口页面不太好看也不太直观,可以考虑试试这个:
- https://doc.xiaominfo.com/knife4j/documentation/
- https://github.com/xiaoymin/Swagger-Bootstrap-UI
<dependency>
<groupId>com.github.xiaoymin</groupId>
<artifactId>swagger-bootstrap-ui</artifactId>
<version>1.9.6</version>
</dependency>
访问:
http://${host}:${port}/${context_path}/doc.html
注意
参考
小码哥-李明杰: Java从0到架构师③进阶互联网架构师.
本文完,感谢您的关注支持!
|