一、基础环境
1.1 版本对应
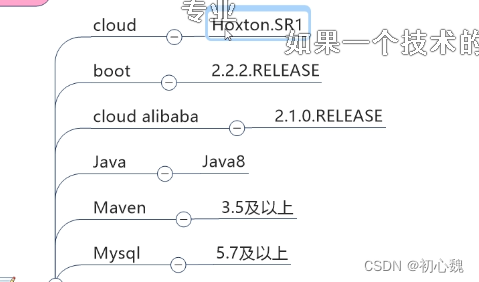
1.2 搭建项目
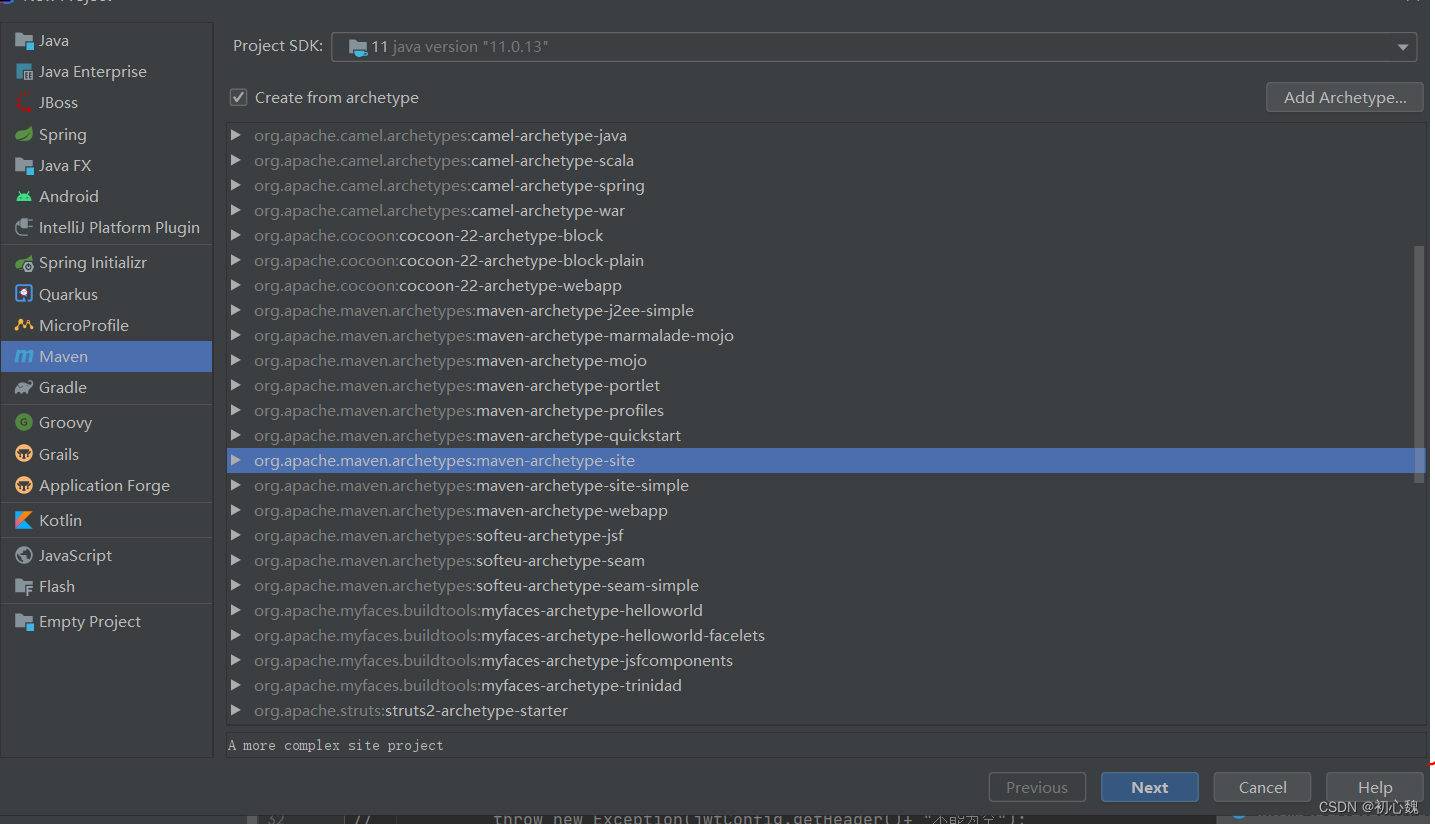
1.3 项目配置
- 字符编码
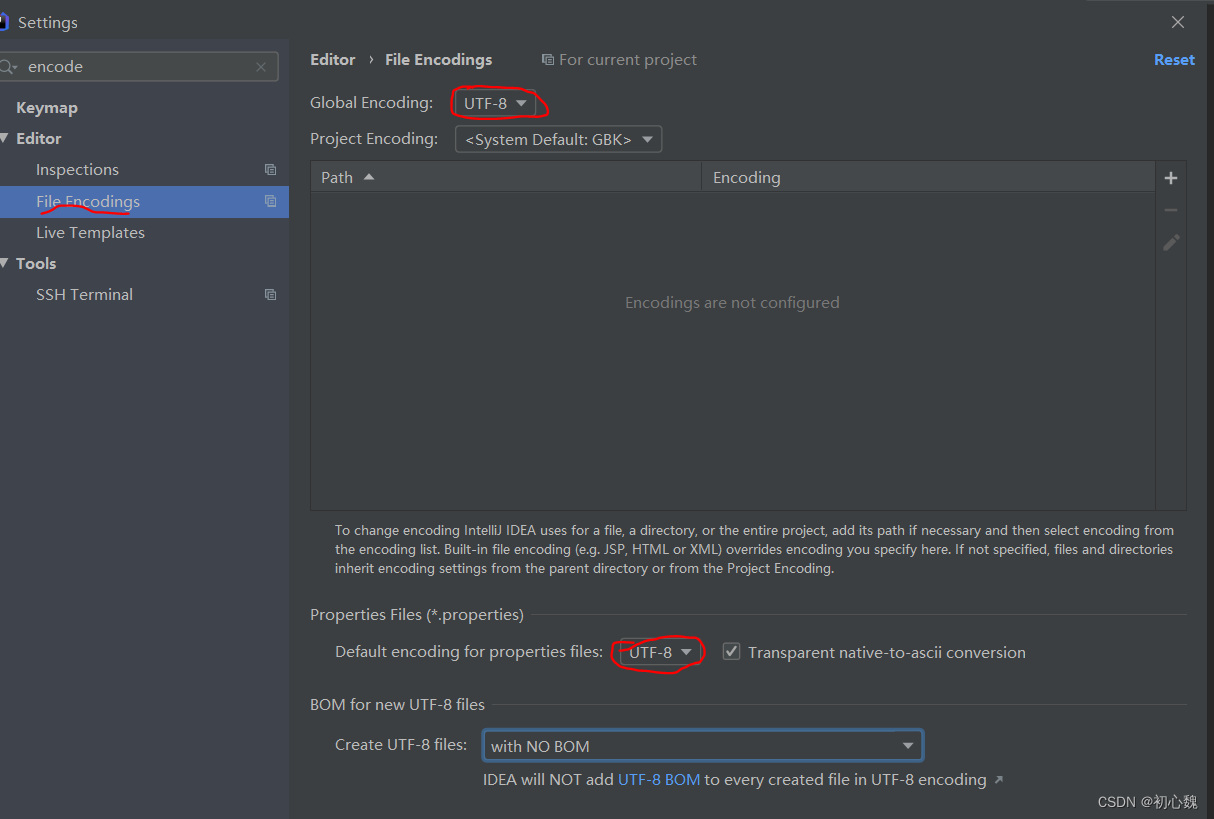 - 注解激活生效
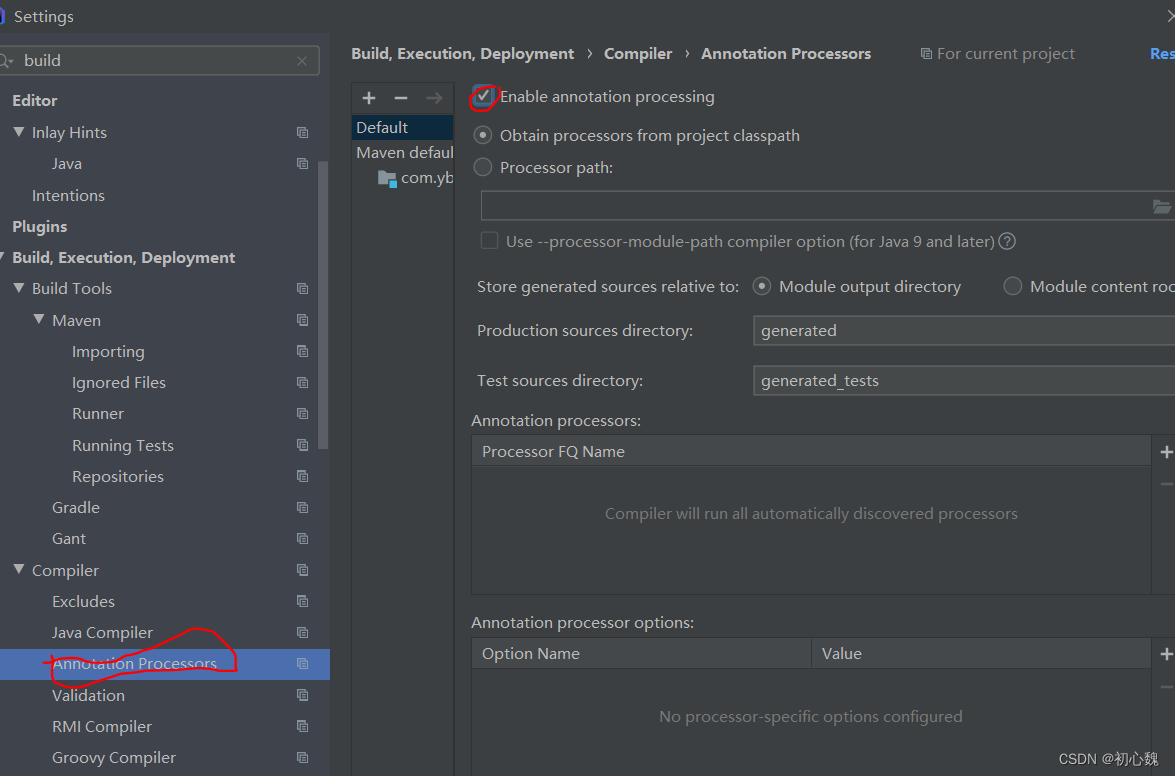
1.4 dependencemanager
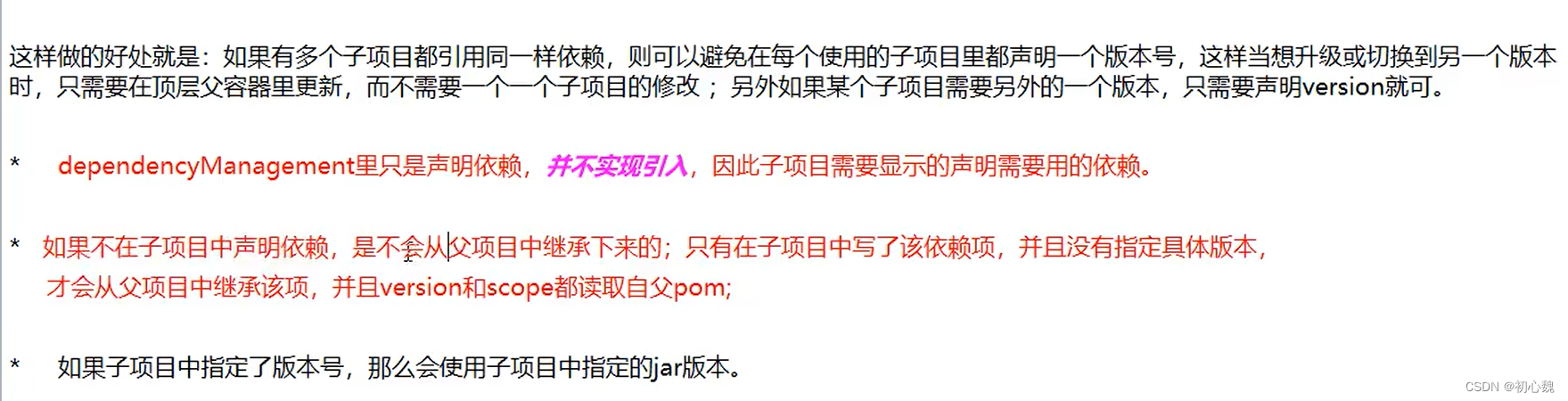
1.5 跳过单元测试
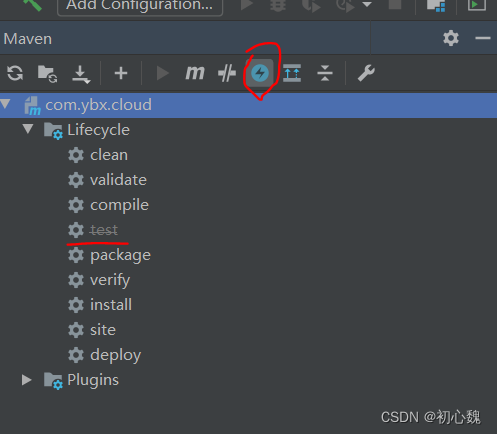
1.6 mvn:install
将父工程发布到仓库中
1.7 项目当前架构
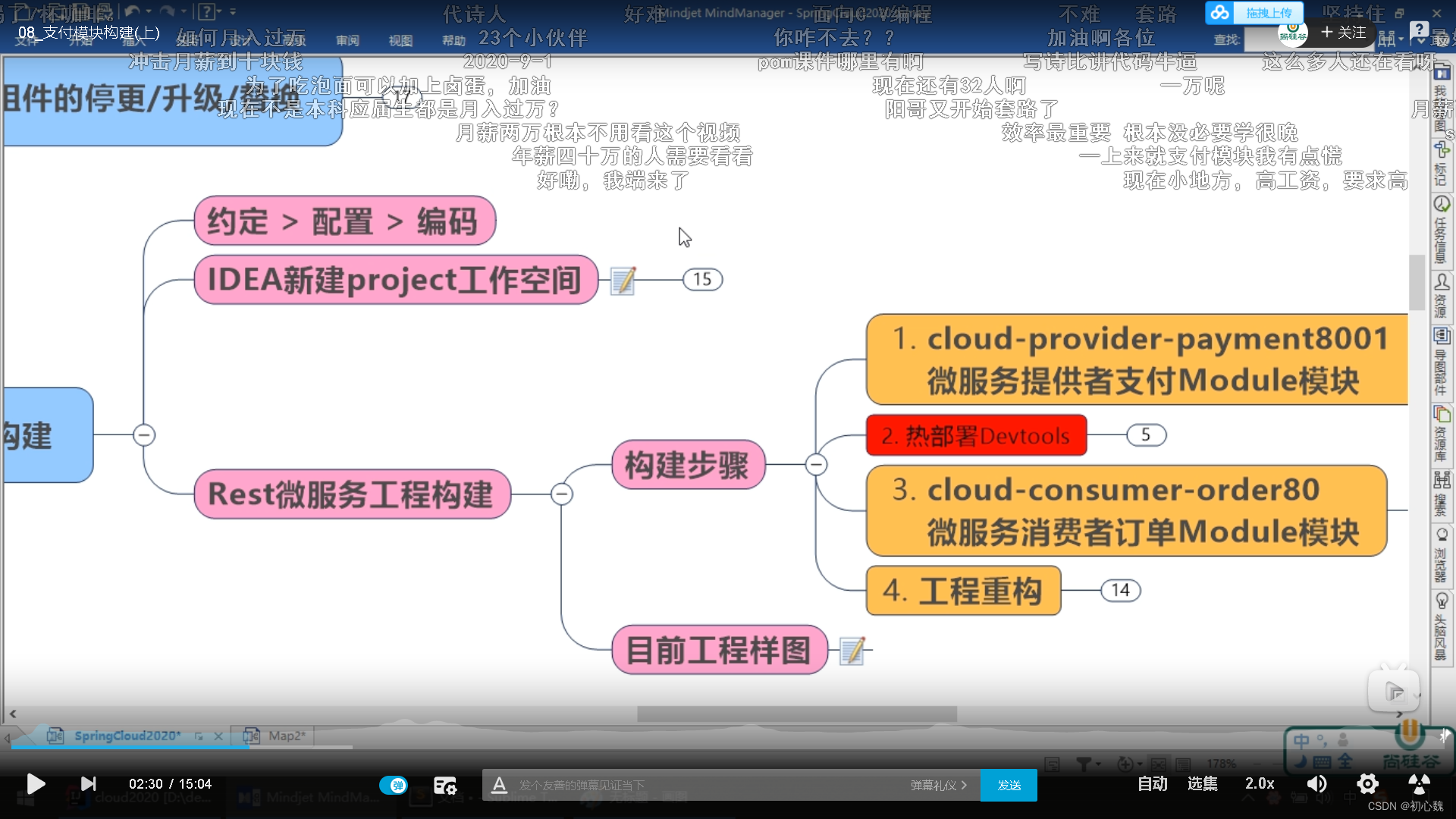
1.8 pom配置repository
<repositories>
<repository>
<id>central</id>
<name>Central Repository</name>
<url>https://repo1.maven.org/maven2/</url>
</repository>
</repositories>
1.9 热部署插件devtools
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<version>2.6.7</version>
</dependency>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<fork>true</fork>
<addResources>true</addResources>
</configuration>
</plugin>
</plugins>
</build>
二、微服务之间的简单调用
2.1 RestTemplate
 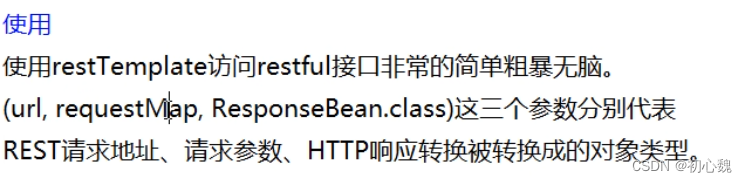
2.1.1 restTemplate实例注入
package com.order.skill.Config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class ApplicationContext {
@Bean
public RestTemplate getRestTemplate(){
return new RestTemplate();
}
}
2.1.2 通过restemPlate调用远程接口
package com.order.skill.Controller;
import com.order.skill.entities.CommenResult;
import com.order.skill.entities.Payment;
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
import javax.annotation.Resource;
@RestController
@Slf4j
public class OrderController {
public static final String PAYMENT_URL = "http://localhost:8081";
@Resource
private RestTemplate restTemplate;
@PostMapping("/consumer/addPayment")
public CommenResult<Payment> create(@RequestParam("serial")String serial){
Payment payment = new Payment(serial);
return restTemplate.postForObject(PAYMENT_URL + "/addPayment", payment, CommenResult.class);
}
@GetMapping("/consumer/getPayment/{id}")
public CommenResult<Payment> getPayment(@PathVariable("id") int id){
return restTemplate.getForObject(PAYMENT_URL + "/getPayment?id=" + id, CommenResult.class);
}
}
2.2 远程接口,注意@RequestBody注解
package com.mypack.payment.Controller;
import com.mypack.payment.Service.PaymentService;
import com.mypack.payment.entities.CommenResult;
import com.mypack.payment.entities.Payment;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import javax.annotation.Resource;
@RestController
public class PaymentController {
@Resource
PaymentService paymentService;
//postmapping好多浏览器不支持
@PostMapping("/addPayment")
public CommenResult addPayment(@RequestBody("serial")String serial){
Payment payment = new Payment(serial);
int i = paymentService.create(payment);
if(i > 0){
return new CommenResult(200,"successcrycry",payment);
}else {
return new CommenResult(500,"error");
}
}
@GetMapping("/getPayment")
public CommenResult getPayment(@RequestParam("id")int id){
Payment payment = paymentService.getPayment(id);
if(payment != null){
return new CommenResult(200,"succ", payment);
}else {
return new CommenResult(500,"error");
}
}
}
2.2.1 远程接口项目结构
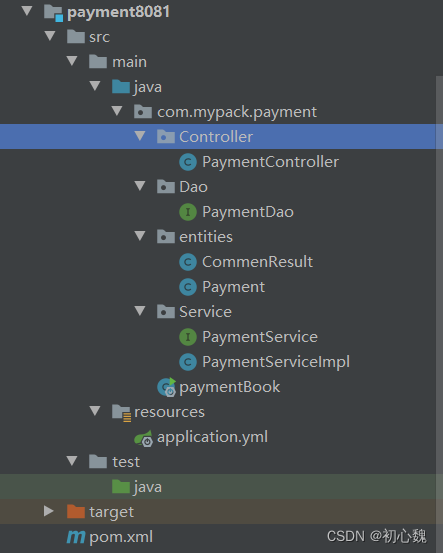
2.2.2 数据层接口
@Mapper
public interface PaymentDao {
@Insert("insert into payment (serial) values (#{serial})")
public int create(Payment payment);
@Select("select * from payment where id = #{id}")
public Payment getPayment(@Param("id")int id);
}
2.3 Spring Dashboard
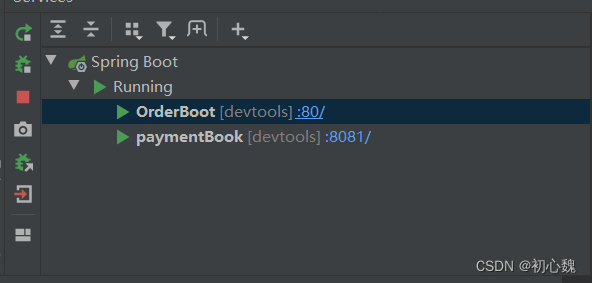
2.4 数据插入数据库失败,记得加requestBody注解
2.5 消费者模块结构目录
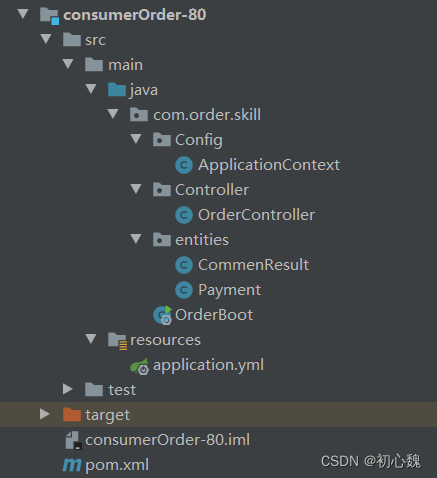
2.6 系统重构,相似的代码提出来
2.6.1 创建公共模块
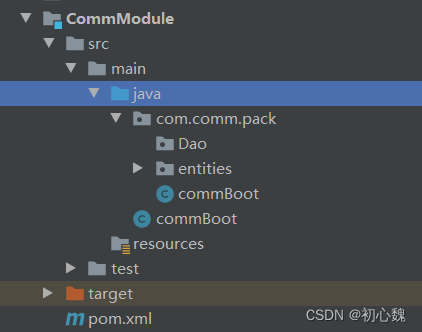
2.6.2 公共模块的pom
<dependencies>
<!-- https://mvnrepository.com/artifact/org.projectlombok/lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/cn.hutool/hutool-all -->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.2.2.RELEASE</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<!--spring cloud Hoxton.SR1-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Hoxton.SR1</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
2.6.3 在comm模块使用mvn clean,mvn install点击闪电标志
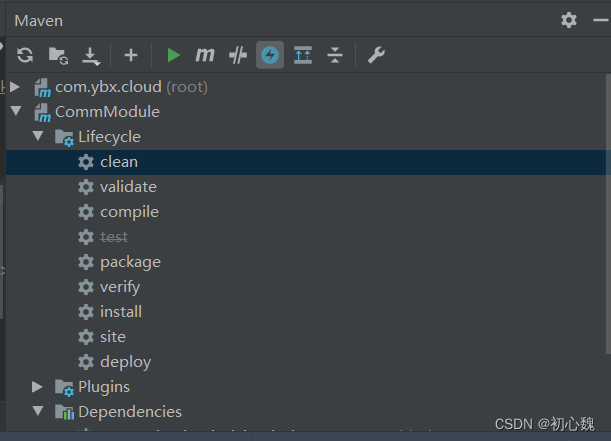
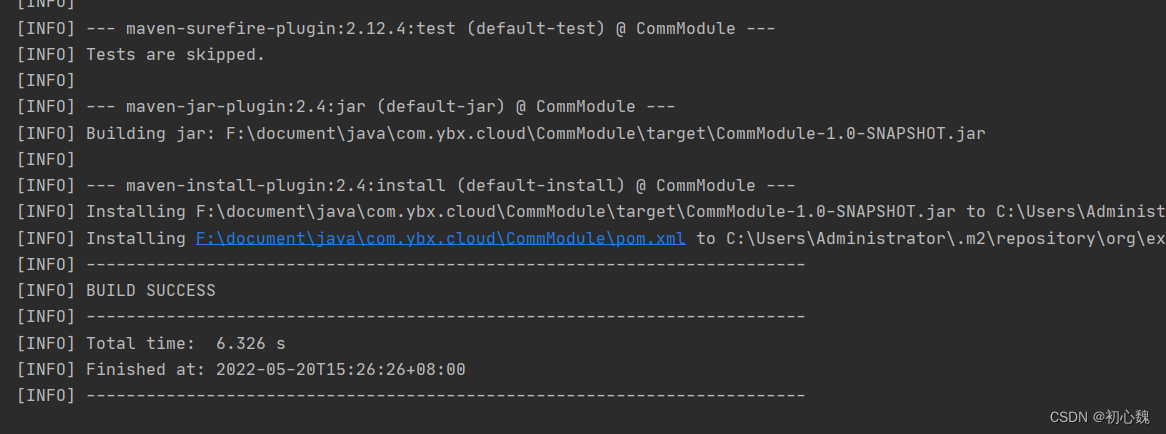
2.6.4 删除冗余的代码,引入公共模块的
三、出错
3.1 dashboard出不来
- idea 项目名右键->show in explorer
- 点进自己的项目->.idea->workspace.xml
- 添加相关配置
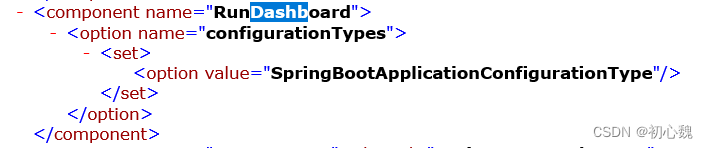
3.2 Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3.1:compile (default-compile) on project CommModule: Fatal error compiling
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
3.3 There was an unexpected error (type=Internal Server Error, status=500).400 : [{“timestamp”:“2022-05-20T07:47:42.395+0000”,“status”:400,“error”:“Bad Request”,“message”:“Required String parameter ‘serial’ is not present”,“trace”:"org.springframework.web.bind.MissingServletRequest… (6011 bytes)]
|