1. 创建一个maven项目
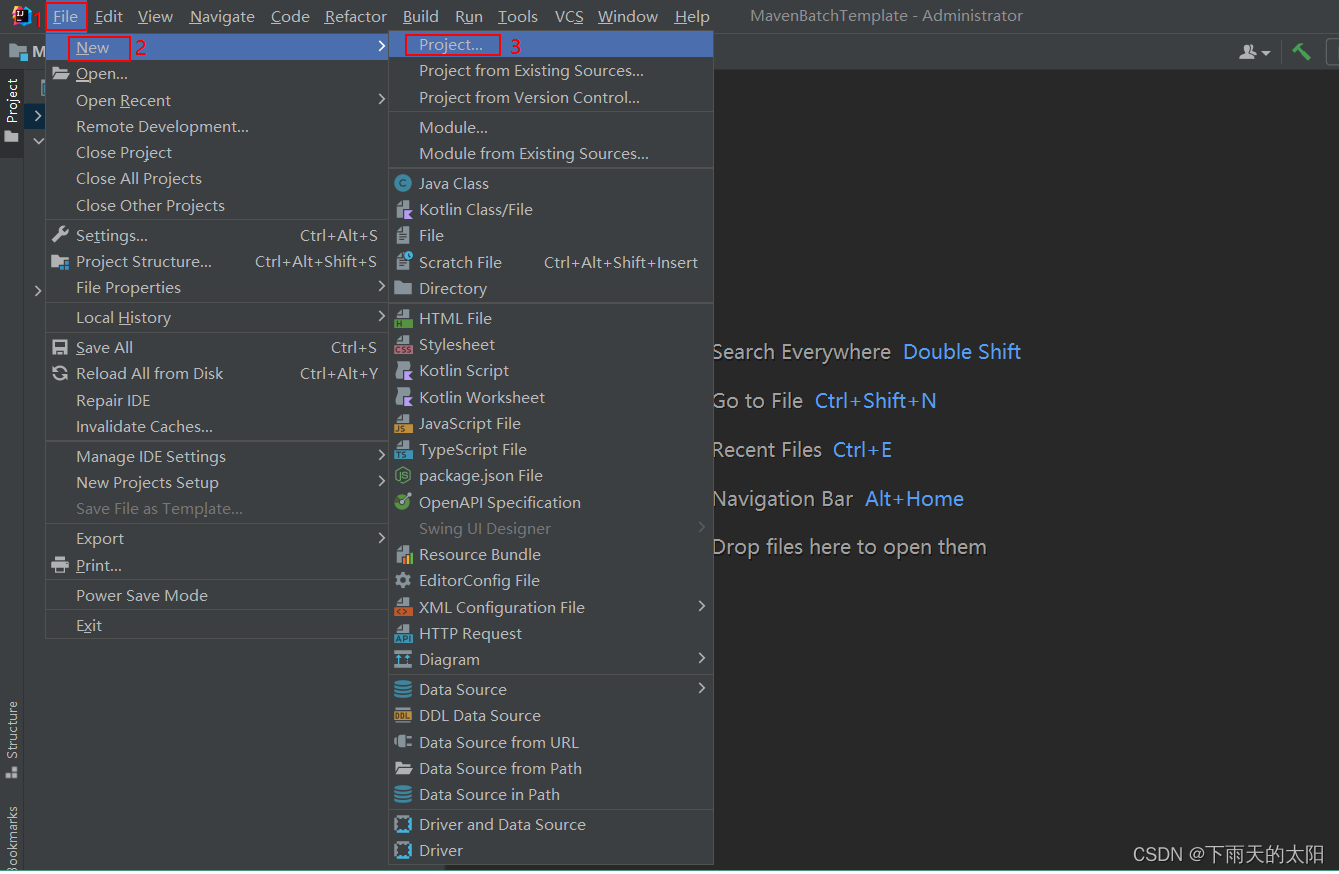 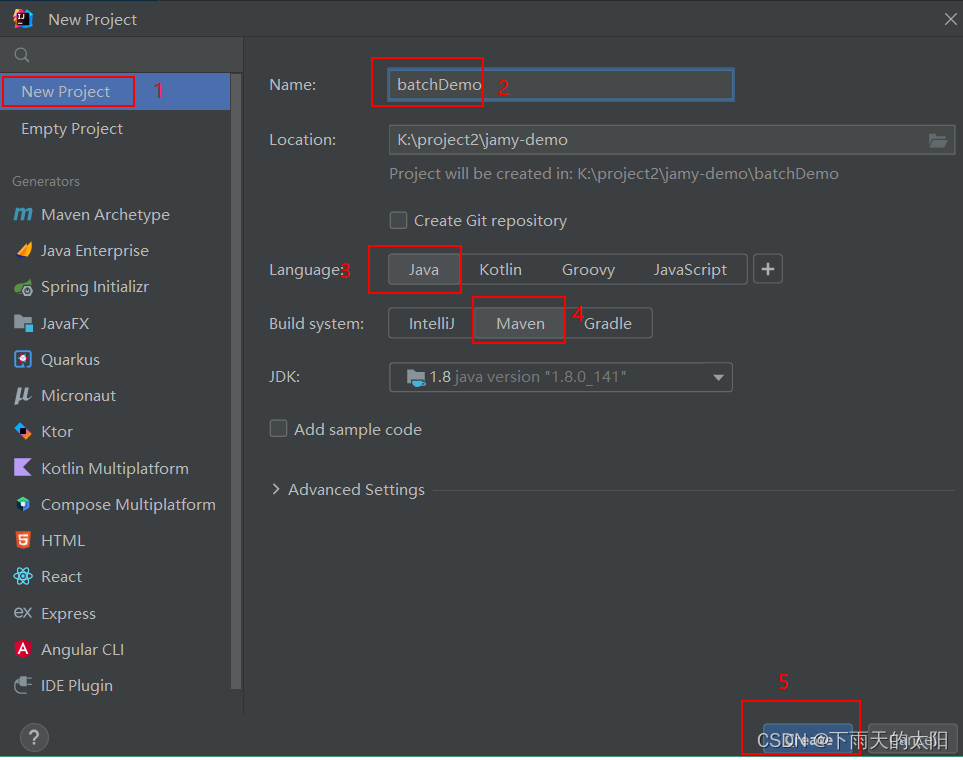 这里可以看到新创建的maven项目 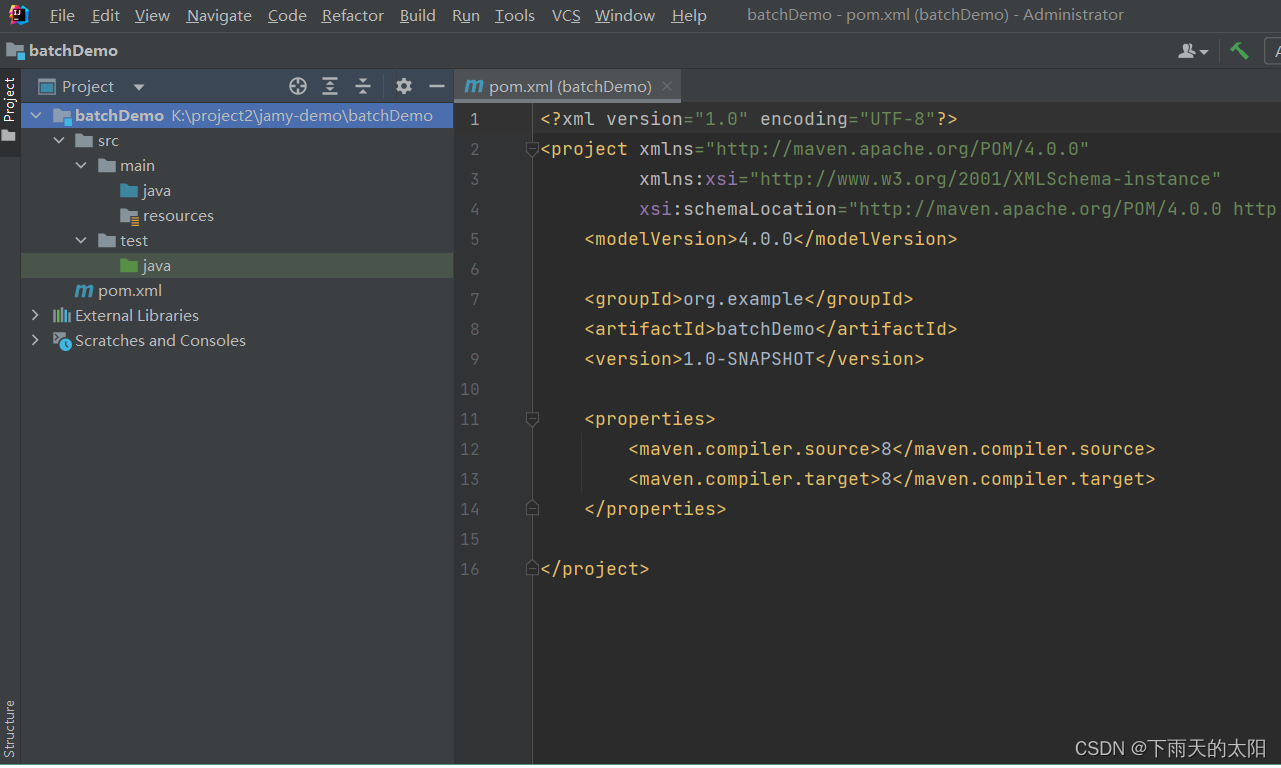
2. 在pom.xml添加项目需要的依赖
<dependencies>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.5</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.16</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.80</version>
</dependency>
</dependencies>
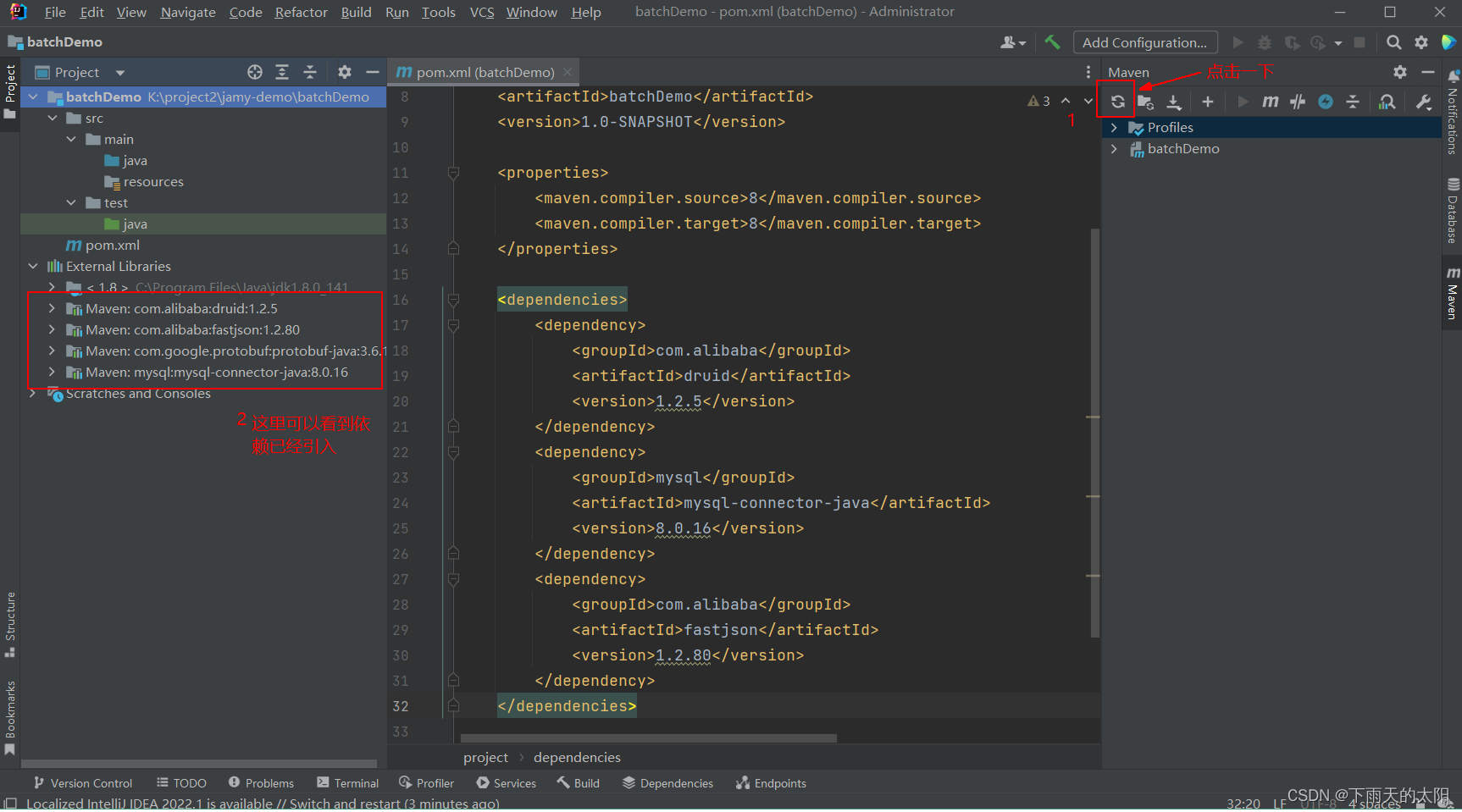
3. 在pom.xml中加入打包插件
<build>
<plugins>
<plugin>
<artifactId>maven-assembly-plugin</artifactId>
<configuration>
<descriptorRefs>
<descriptorRef>jar-with-dependencies</descriptorRef>
</descriptorRefs>
<archive>
<manifest>
<mainClass>com.jamy.song.job.Demo</mainClass>
</manifest>
</archive>
</configuration>
<executions>
<execution>
<id>make-assembly</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
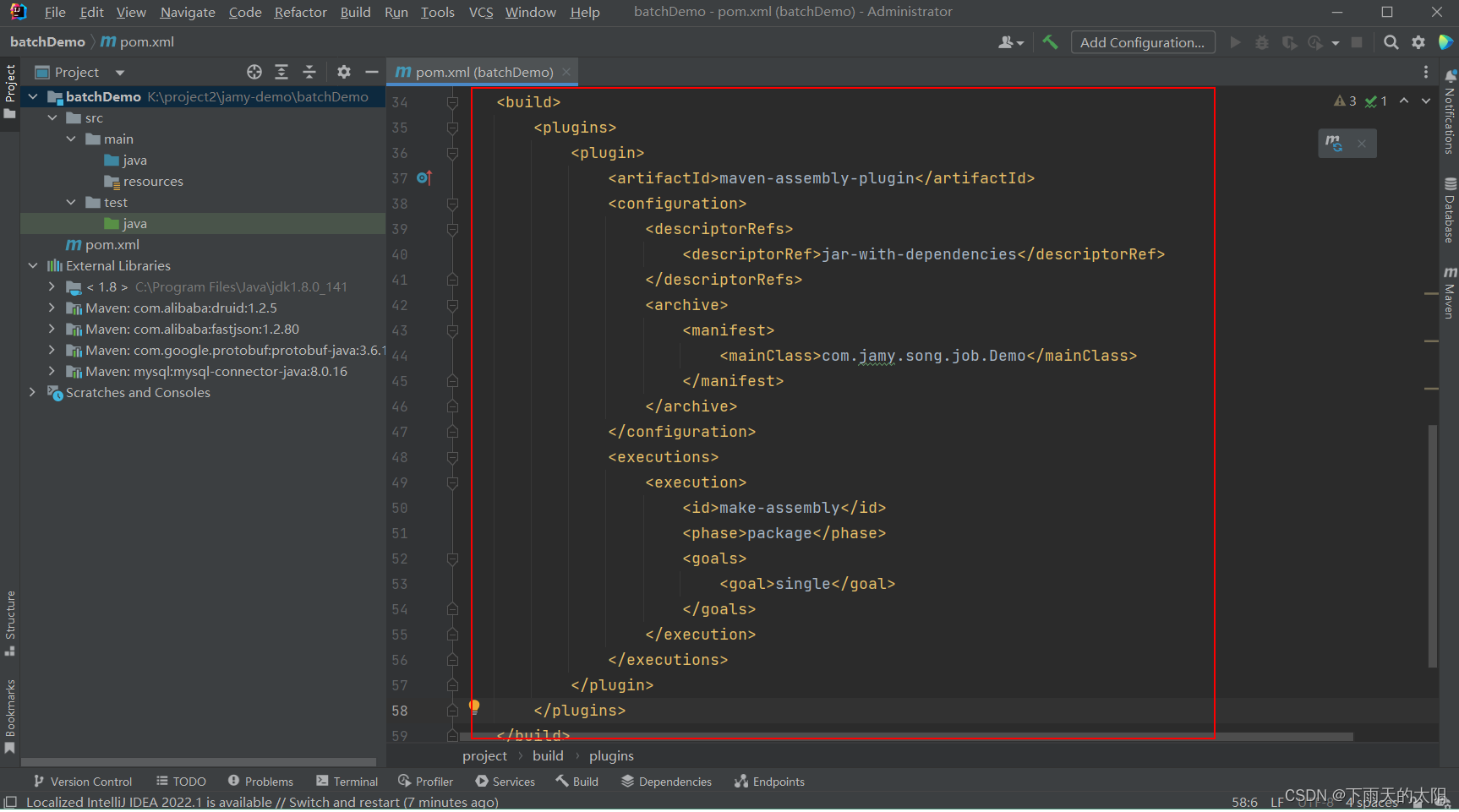
4. 编写代码和添加配置文件
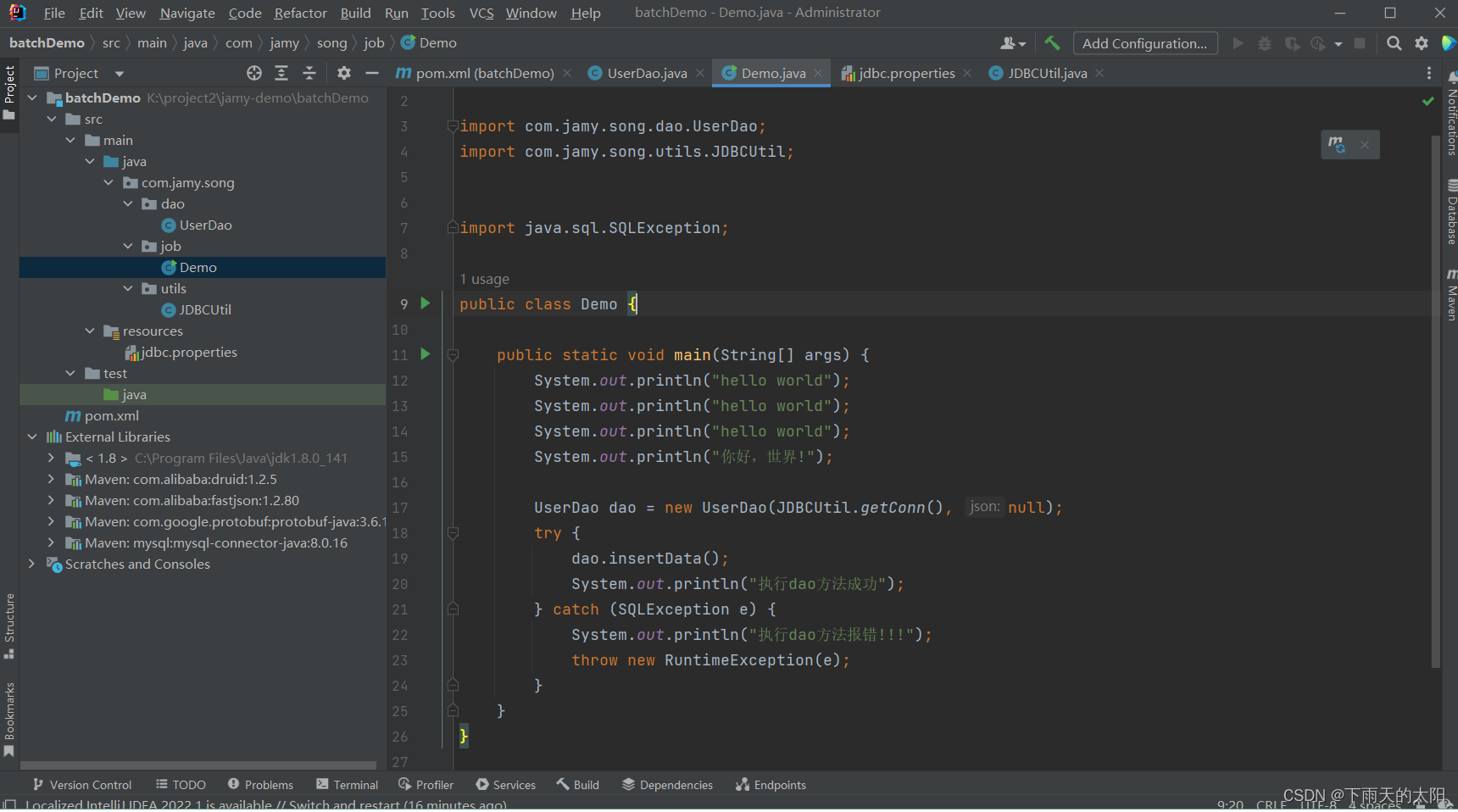
主类
public class Demo {
public static void main(String[] args) {
System.out.println("hello world");
System.out.println("hello world");
System.out.println("hello world");
System.out.println("你好,世界!");
UserDao dao = new UserDao(JDBCUtil.getConn(), null);
try {
dao.insertData();
System.out.println("执行dao方法成功");
} catch (SQLException e) {
System.out.println("执行dao方法报错!!!");
throw new RuntimeException(e);
}
}
}
JDBC工具类
public class JDBCUtil {
private static final ThreadLocal<Connection> threadLocal = new ThreadLocal<Connection>();
private static Properties prop = new Properties();
private static DruidDataSource dataSource = new DruidDataSource();
private static Connection conn = null;
static {
try{
prop.load(JDBCUtil.class.getClassLoader().getResourceAsStream("jdbc.properties"));
}catch(IOException e){
System.out.println("数据库参数文件读取错误");
e.printStackTrace();
}
dataSource.setUsername(prop.getProperty("jdbc.username"));
dataSource.setUrl(prop.getProperty("jdbc.url"));
dataSource.setPassword(prop.getProperty("jdbc.password"));
try{
dataSource.setDriverClassName(prop.getProperty("jdbc.driverClass"));
}catch(Exception e){
System.out.println("连接池构造异常1!");
e.printStackTrace();
}
dataSource.setInitialSize(Integer.valueOf(prop.getProperty("jdbc.initialSize")));
dataSource.setMinIdle(Integer.valueOf(prop.getProperty("jdbc.minPoolSize")));
dataSource.setMaxActive(Integer.valueOf(prop.getProperty("jdbc.maxPoolSize")));
dataSource.setMaxWait(Integer.valueOf(prop.getProperty("jdbc.maxWait")));
dataSource.setTimeBetweenEvictionRunsMillis(Integer.valueOf(prop.getProperty("jdbc.timeBetweenEvictionRunsMillis")));
dataSource.setMinEvictableIdleTimeMillis(Integer.valueOf(prop.getProperty("jdbc.minEvictableIdleTimeMillis")));
try {
threadLocal.set(dataSource.getConnection());
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public static Connection getConn(){
conn = threadLocal.get();
System.out.println("threadLocal获取连接:" + conn);
try{
if (conn == null || conn.isClosed()) {
Class.forName(prop.getProperty("jdbc.driverClass")).toString();
conn = DriverManager.getConnection(prop.getProperty("jdbc.url").toString(),
prop.getProperty("jdbc.username").toString(),
prop.getProperty("jdbc.password").toString());
try{
dataSource.setDriverClassName(prop.getProperty("jdbc.driverClass"));
}catch(Exception e){
System.out.println("连接池构造异常2!");
e.printStackTrace();
throw new IllegalStateException();
}
}
System.out.println("conn:"+conn);
}catch(Exception e){
System.out.println("获取数据库连接异常!");
e.printStackTrace();
throw new IllegalStateException();
}
return conn;
}
public static void closeConn(){
try{
if(threadLocal.get() != null && !threadLocal.get().isClosed()){
threadLocal.get().close();
threadLocal.remove();
}
}catch(Exception e){
System.out.println("关闭数据库连接异常!");
}
}
public static void beginTransaction(){
try{
getConn().setAutoCommit(false);
}catch(SQLException e){
System.out.println("开始事务异常");
throw new IllegalStateException();
}
}
public static void commit(){
try{
getConn().commit();
}catch(SQLException e){
System.out.println("提交事务异常");
throw new IllegalStateException();
}
}
public static void rollback(){
try{
Connection conn = getConn();
conn.setAutoCommit(false);
conn.rollback();
conn.setAutoCommit(true);
}catch(SQLException e){
System.out.println("事务回滚异常");
throw new IllegalStateException();
}
}
}
JDBC操作类
public class UserDao {
private Connection conn;
private JSONObject json;
PreparedStatement ps = null;
public UserDao(Connection conn, JSONObject json) {
this.conn = conn;
this.json = json;
}
public void insertData() throws SQLException {
String sql = "insert into tb_user(name,age,address) values(?,?,?)";
ps = conn.prepareStatement(sql);
try{
for (int i=0; i<100; i++) {
ps.setString(1, "张三"+i);
ps.setInt(2, 23);
ps.setString(3, "人民路120号");
ps.addBatch();
}
ps.executeBatch();
}catch(SQLException e){
System.out.println("执行插入数据异常!");
}finally {
if (ps != null) {
ps.close();
}
if (conn != null){
conn.close();
}
}
}
}
配置文件
jdbc.driverClass=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://192.168.227.122:3306/test?useUnicode=true&useSSL=false&characterEncoding=utf8&serverTimezone=GMT%2b8&nullCatalogMeansCurrent=true&allowPublicKeyRetrieval=true
jdbc.username=root
jdbc.password=root
jdbc.initialSize=20
jdbc.minPoolSize=10
jdbc.maxPoolSize=100
#jdbc.MaxStatements=5
#jdbc.MaxIdleTime=600
jdbc.maxWait=60000
jdbc.timeBetweenEvictionRunsMillis=60000
jdbc.minEvictableIdleTimeMillis=300000
5. 打jar包
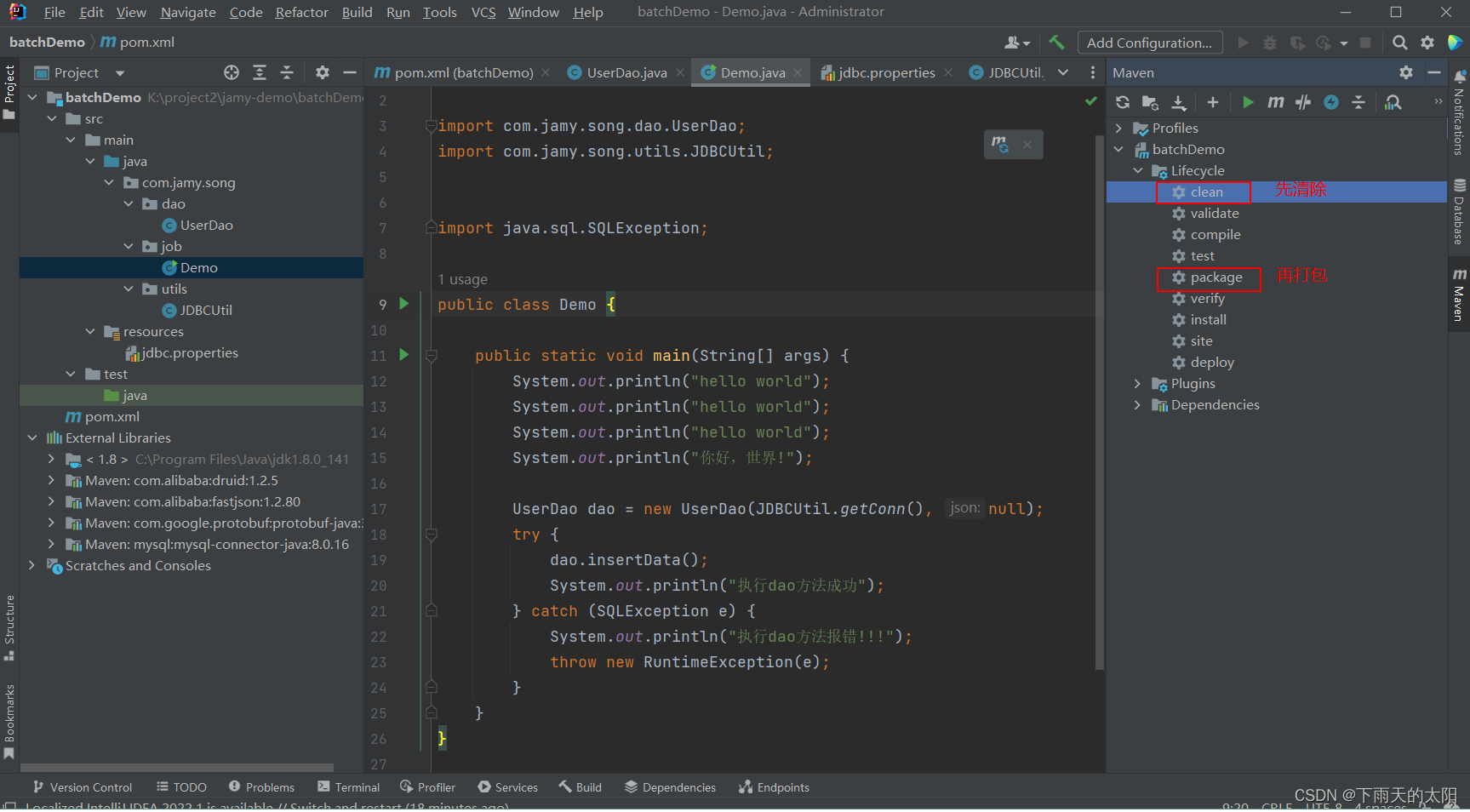 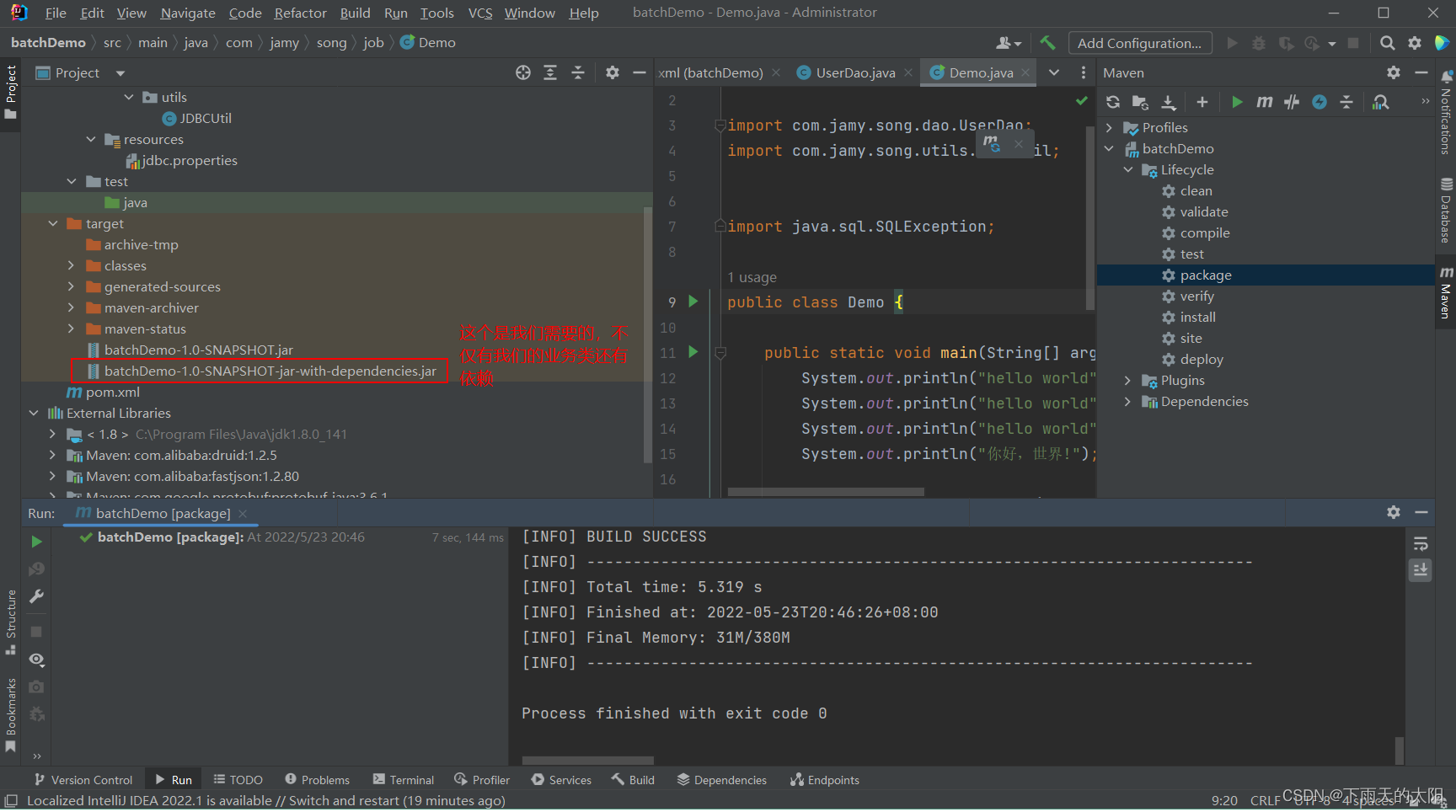 这里用解压缩文件打开jar包看到,依赖和业务类都有,说明我们打的jar包是完整的 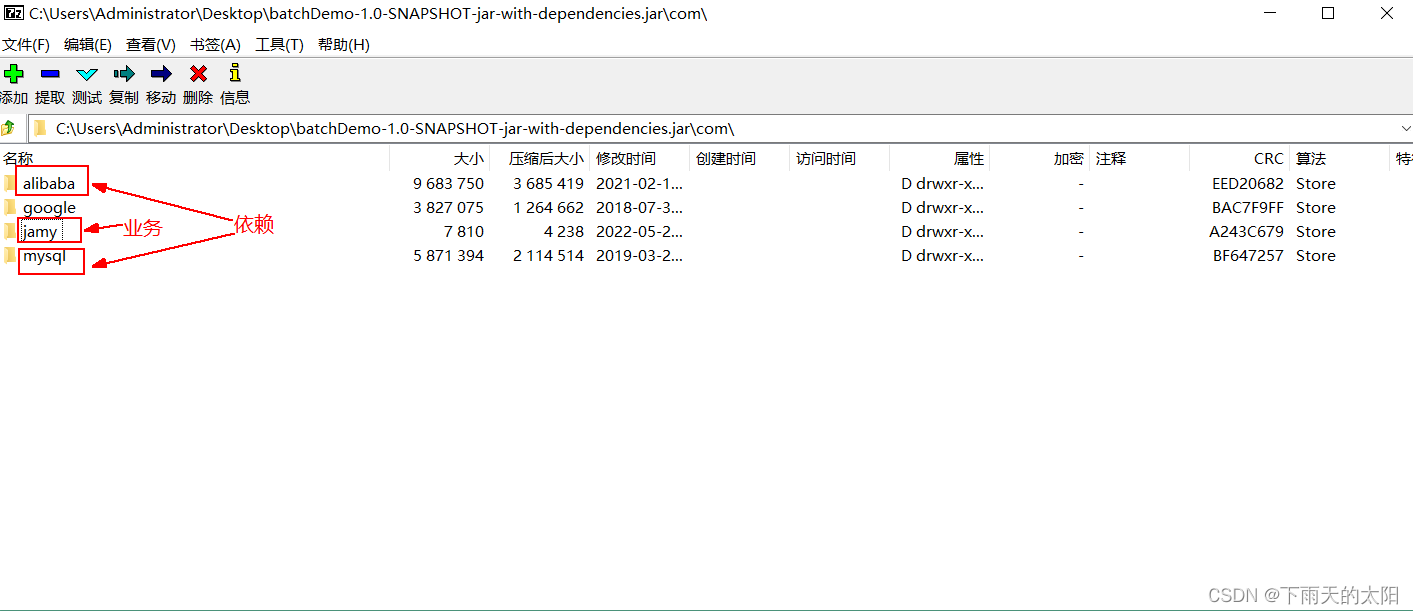 这的jar包名字太长了,这里我把它改成jamy.jar,然后把他上传到linux服务器上(要放在shell脚本里写的位置,否则执行时找不到),我们通过shell脚本来执行下看看,脚本内容如下:
#!/bin/bash
JAVA_HOME=/usr/local/src/jdk1.8
LANG=zh_CN.UTF-8
BATCHROOT=/app/demoBatch
${JAVA_HOME}/bin/java -Xms1024M -Xmx1024M -Dfile.encoding=UTF-8 -cp ${BATCHROOT}/jamy.jar com.jamy.song.job.Demo
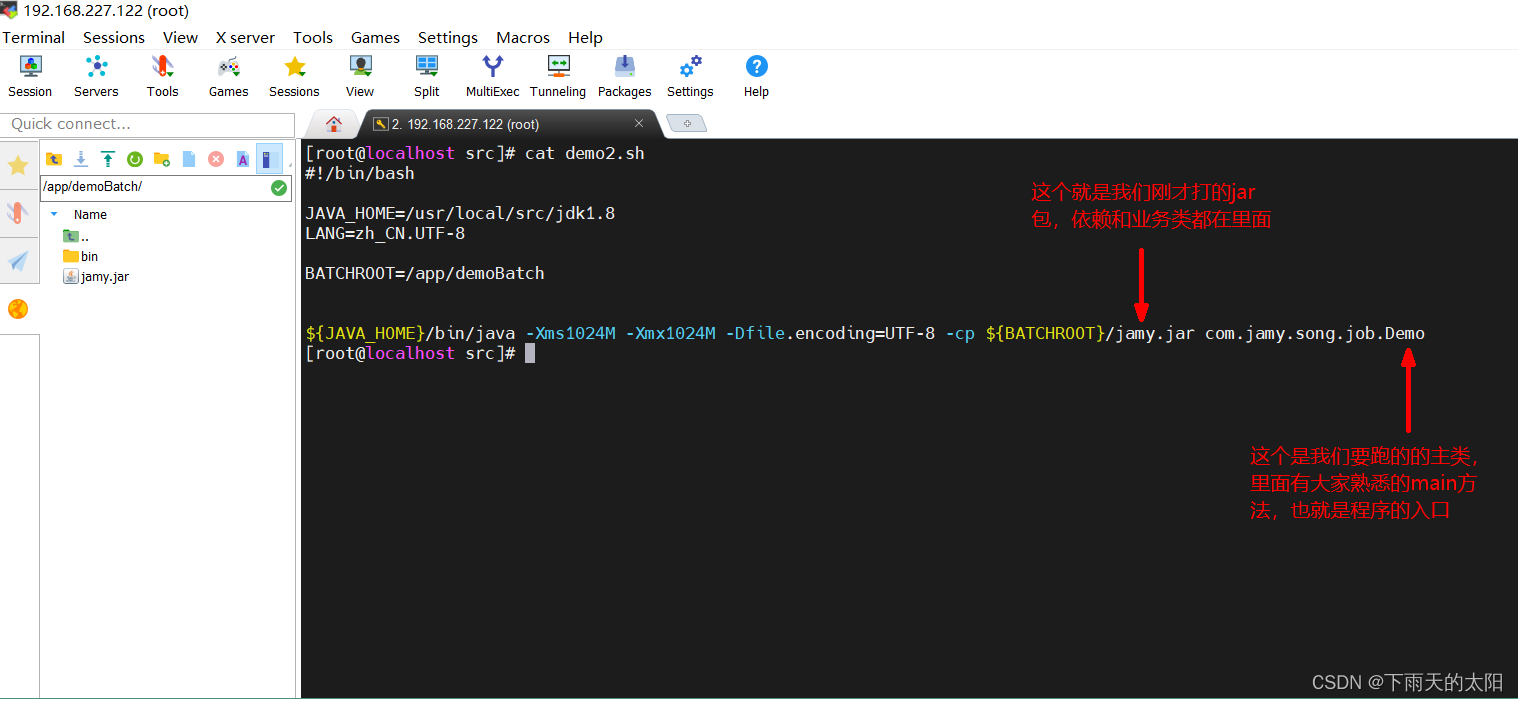 现在来看下执行效果(执行前jdk要配置好,我这里配置的时jdk1.8),执行sh demo2.sh这个命令即可 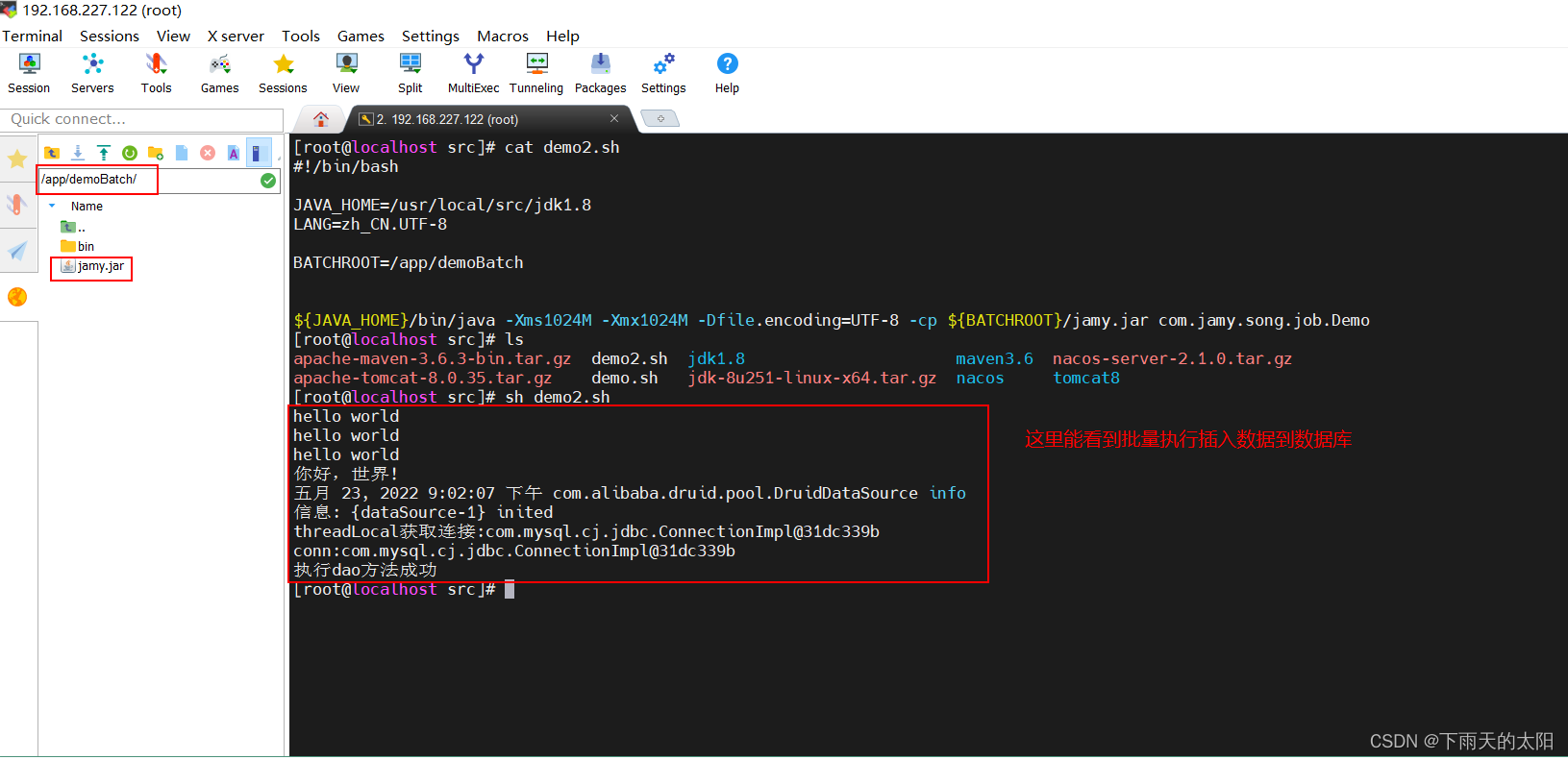 注意:这种打jar包非常适合批处理业务,如果是纯java项目可以参考下面两种方法: 纯java项目打包方法一 纯java项目打包方法二
|