1,?@Query?的使用
@Repository
public interface PostRepository extends JpaRepository<PostEntity, String>, JpaSpecificationExecutor<PostEntity> {
//根据name 来查询
public List<PostEntity> findByName(String name);
//根据nameid 来查询
public PostEntity findByNameId(String id);
//查询id为 参数 且name中含有 参数 的用户
public PostEntity findByNameIdAndNameLike(String id,String name);
//使用Query注解来写sql,更加灵活。默认是hsql, 使用nativeQuery = true,来表明它是原生sql
@Query(value="select * from post t where t.delect='0'", nativeQuery = true)
public List<PostEntity> selectAll();
//更新、插入、删除 要添加 @Modifying @Transactional 注解
@Modifying
@Transactional
@Query(value="update post t set t.delect='1' where t.id in (:collection)", nativeQuery = true)
public void deletePost(@Param("collection") Collection<String> collection);
}
@Override
public boolean deletePost(JSONObject params) {
if( params != null){
try {
List<String> idslist = (List<String>)params.get("ids");
postRepository.deletePost(idslist);
return true;
} catch (Exception e) {
return false;
}
}
return true;
}
2,方法名查询:只需要按照SpringDataJpa提供的方法名称规则去定义方法,在dao接口中定义方法即可。
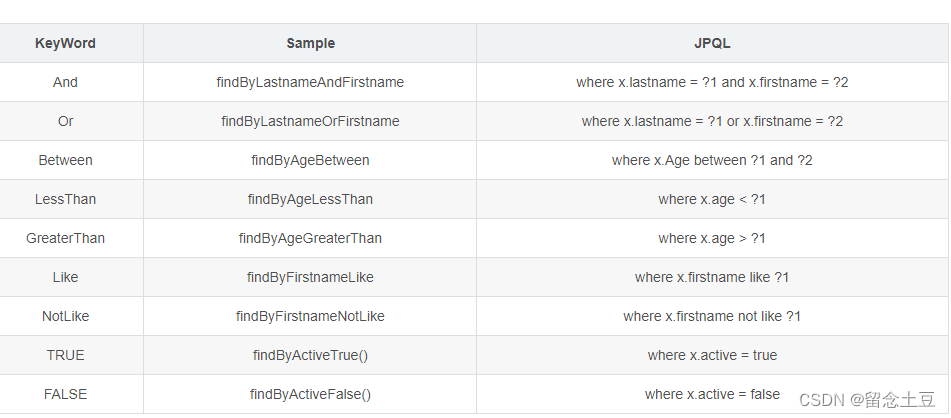
3, 复杂查询
实体类?
import java.io.Serializable;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.SequenceGenerator;
import javax.persistence.Table;
import org.hibernate.annotations.GenericGenerator;
/**
*
* @SystemName 帖子信息实体类
* @ModuleName
* @ClassName postEntity
* @author liunn
* @Date 2022年5月5日 下午15:33:58
* @version V1.0.0
* @Description
*/
@Entity
@Table(name="post")
@SequenceGenerator(name = "LOG_ID_SEQ",sequenceName = "SEQ_OPERATION_LOG",allocationSize = 1)
public class PostEntity implements Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
//帖子信息id
@Id
@GeneratedValue(generator = "idGenerator")
@GenericGenerator(name = "idGenerator", strategy = "uuid")
private String id;
//帖子信息图片的路径
private String pictureurl;
//帖子的名字
private String there;
//创建帖子的时间
private String created;
//帖子的内容
private String info;
//创建帖子的用户ID
private String userid;
//创建帖子的用户
private String username;
//是否删除 0.没有删除,1删除
private String delect = "0";
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getCreated() {
return created;
}
public void setCreated(String created) {
this.created = created;
}
public String getUserid() {
return userid;
}
public void setUserid(String userid) {
this.userid = userid;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getDelect() {
return delect;
}
public void setDelect(String delect) {
this.delect = delect;
}
public String getPictureurl() {
return pictureurl;
}
public void setPictureurl(String pictureurl) {
this.pictureurl = pictureurl;
}
public String getThere() {
return there;
}
public void setThere(String there) {
this.there = there;
}
public String getInfo() {
return info;
}
public void setInfo(String info) {
this.info = info;
}
}
@Override
public Map<String, Object> selectByUser(Map<String, Object> params) {
Specification<PostEntity> specification = new Specification<PostEntity>() {
private static final long serialVersionUID = 1L;
@Override
public Predicate toPredicate(Root<PostEntity> root, CriteriaQuery<?> query, CriteriaBuilder cb) {
List<Predicate> preList = new ArrayList<Predicate>();
if (params != null && !params.isEmpty()) {
//获取 map 的 key 和value , key = kv.getKey(); value = kv.getValue()
for(Map.Entry<String,Object> kv : params.entrySet()){
if(!"pageIndex".equals(kv.getKey()) && !"pageSize".equals(kv.getKey())) {
Path<String> namePath = root.get(kv.getKey());
Predicate predicate = cb.equal(namePath, kv.getValue());
preList.add(predicate);
}
}
}
//过滤掉 删除的数据 1代表删除,0代表没有删除。
Path<String> namePath = root.get("delect");
Predicate predicate = cb.equal(namePath, "0");
preList.add(predicate);
query.where(preList.toArray(new Predicate[preList.size()]));
preList.clear();
return query.getRestriction();
}
};
Sort sort =new Sort(Sort.Direction.DESC, "created");
int pageIndex = 0, pageSize = 1;
if (params.get("pageIndex") != null && params.get("pageSize") != null) {
pageIndex = (int) params.get("pageIndex");
pageSize = (int) params.get("pageSize");
}
Pageable pageable =PageRequest.of(pageIndex , pageSize, sort);
Page<PostEntity> Files =postRepository.findAll(specification, pageable);
List<PostEntity> result = new ArrayList<PostEntity>();
long count = 0;
result = Files.getContent();
count = Files.getTotalElements();
Map<String, Object> resultMap = new HashMap<String, Object>();
resultMap.put("result", result);
resultMap.put("count", count);
return resultMap;
}
需要注意:root.get()?括号里面的值要在表字段。否则它会在数据库表创建。
? ? ? ? ? ? ? ? 排序使用new Sort ()? ??
? ? ? ? ? ? ? ? 分页使用PageRequest.of()
? ? ? ? ? ? ? ? 不同版本的springboot?创建不同,低版本的是?new?PageRequest()
? ? ? ? ? ? ? ? 下面这句话意思?就是,查询?字段delect为0。
Path<String> namePath = root.get("delect");
Predicate predicate = cb.equal(namePath, "0");
????????????????模糊查询
Path<String> namePath1 = root.get("info");
Predicate predicate1 = cb.like(namePath1, "%北京%");
通过ID来查询
@Override
public PostEntity selectById(Map<String, String> params) {
String id = params.get("id");
Optional<PostEntity> optional=postRepository.findById(id);
if(optional.isPresent()) {
PostEntity postEntity=optional.get();
return postEntity;
}else {
return null;
}
}
保存
@Override
public boolean savePost(PostEntity postEntity) {
// java8新提供的类:LocalDate、LocalTime、LocalDateTime。不存在线程不安全问题。
DateTimeFormatter pattern = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
//LocalDateTime 转字符串
LocalDateTime dateTime = LocalDateTime.now();
String dateTimeStr = dateTime.format(pattern);
postEntity.setCreated(dateTimeStr);
boolean r = postRepository.save(postEntity) != null ? true : false;
return r;
}
保存或更新,如果ID有值,它会看数据库那个ID有没有数据,没有就新增,有就更新
@Override
public boolean updata(PostEntity postEntity) {
boolean r = postRepository.saveAndFlush(postEntity) != null ? true : false;
return r;
}
多表关联查询,需要了解Hibernate的一对多,一对一,多对多,多对一,而且,有关联关系的话,Hibernate会给你的表添加外键,外键有利有弊。而且比较复杂可以参考下面两个链接
参考:https://blog.csdn.net/qq_36639232/article/details/106116274
参考:https://blog.csdn.net/q990609179/article/details/103313933
待?增加
|