一、新建Java项目
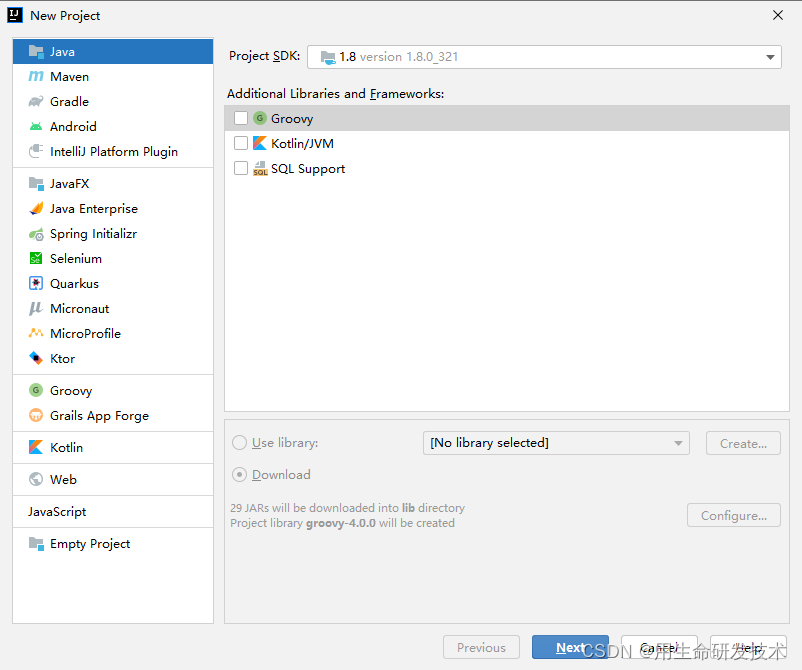 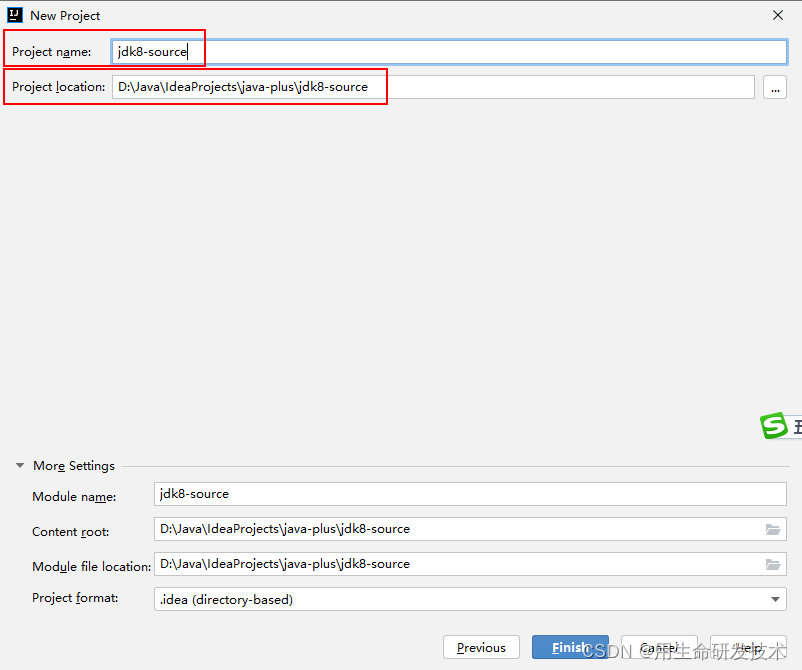 新建包
- com.java521.source:用于存放源码
- com.java521.test:用于存放测试代码
二、导入JDK源码
JDK源码就在JAVA_HOME/src.zip 中,解压即可用。 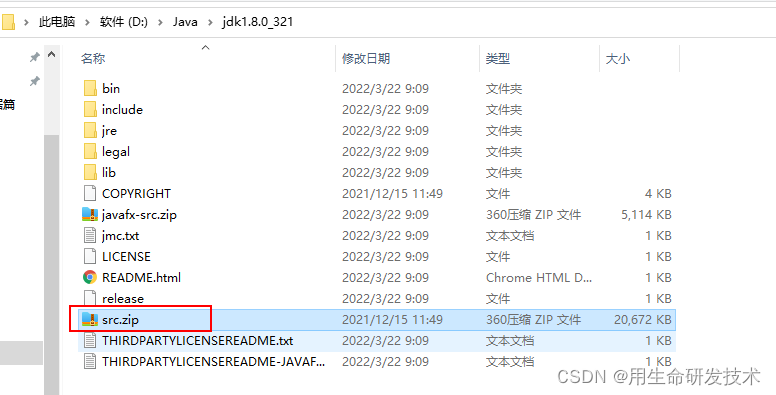
三、替换JDK关联
移除Sourcepath中关联的src.zip 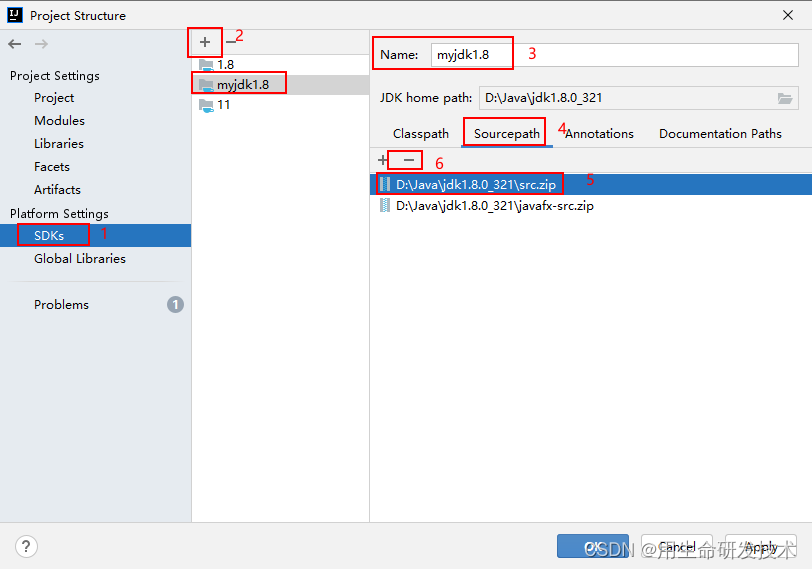 将项目中的JDK源码关联到Sourcepath 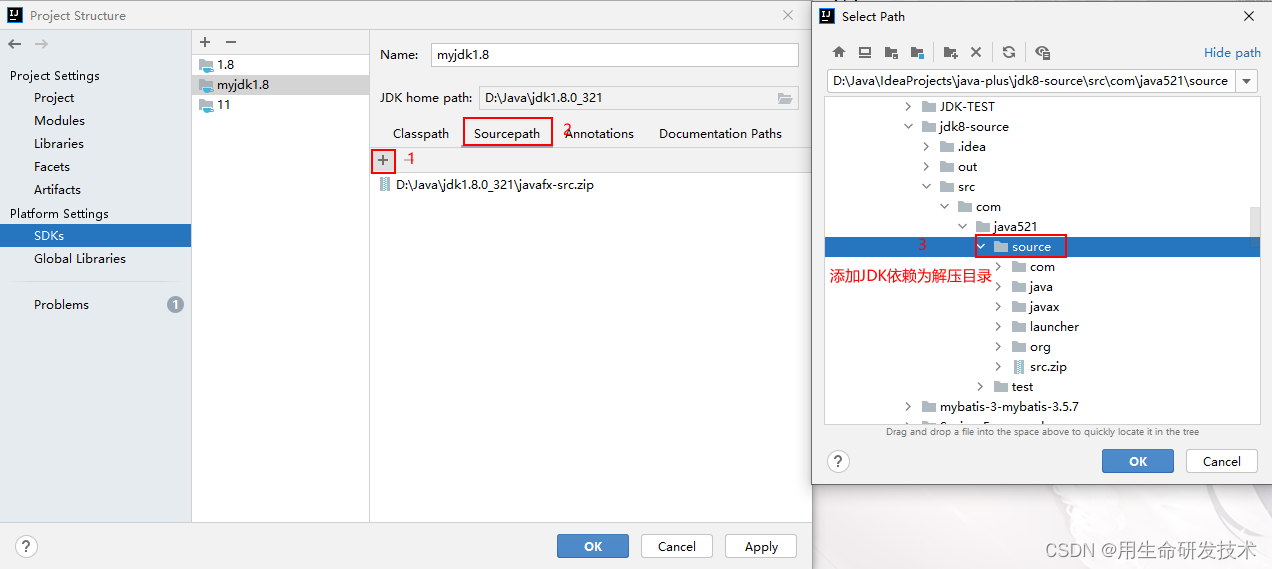 修改项目模块JDK为myjdk1.8 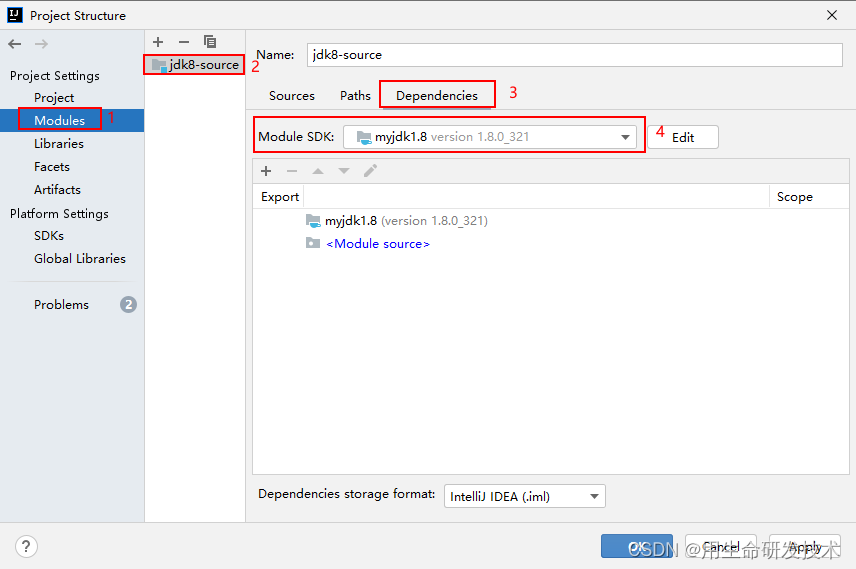
四、测试代码
编写测试代码,执行测试代码。
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, String> map = new HashMap<>();
map.put("k1", "v1");
}
}
报错 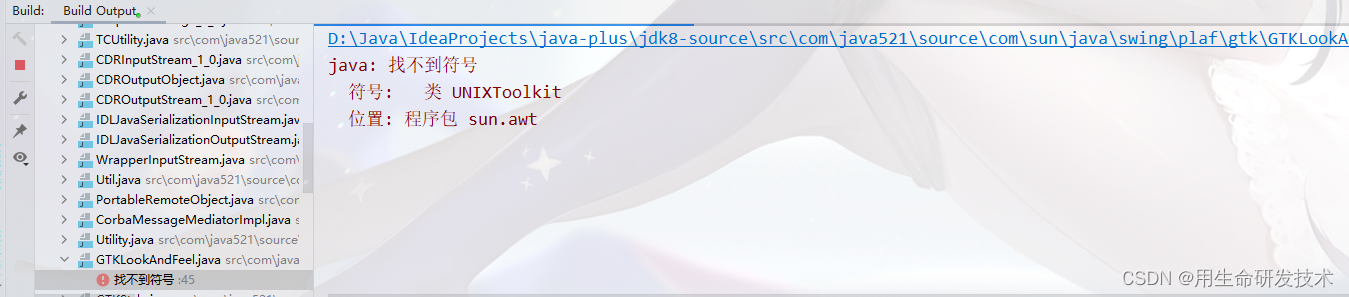
五、解决找不到sun.awt.UNIXToolkit和sun.font.FontConfigManager
在项目中新建 sun.awt 包和 sun.font 包,分别新建 UNIXToolkit.java 和 FontConfigManager.java 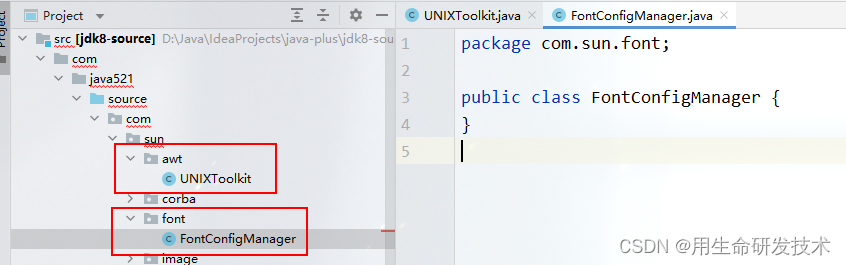
到OpenJDK寻找sun.awt.UNIXToolkit和sun.font.FontConfigManager的源码,分别复制到上面新建的两个类里 OpenJDK:http://openjdk.java.net/ 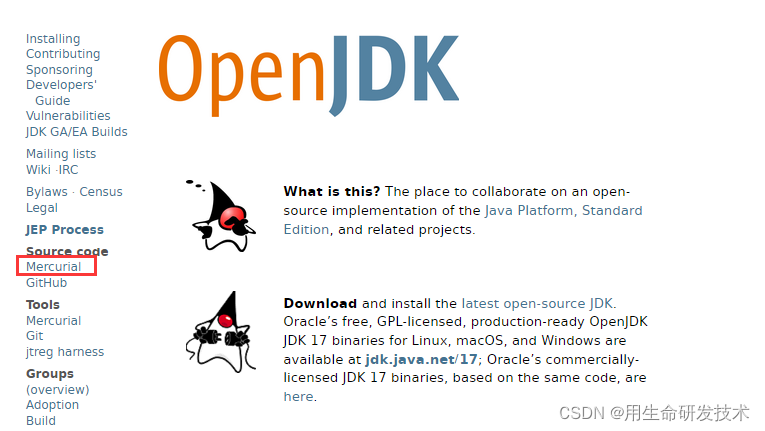 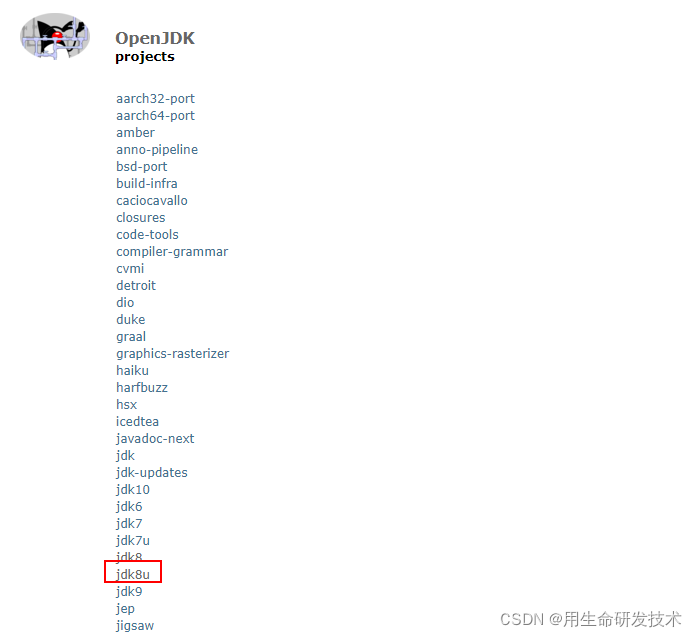 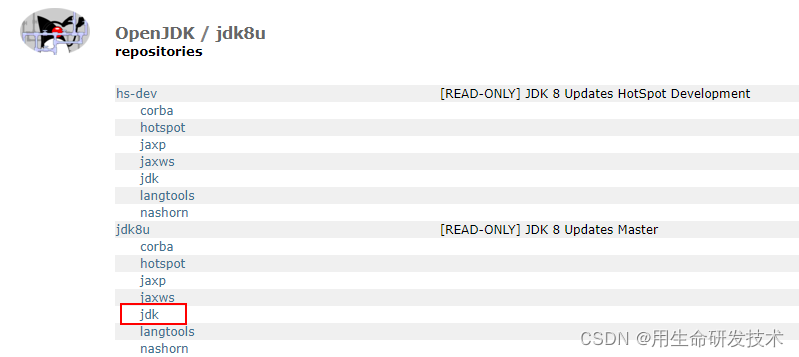 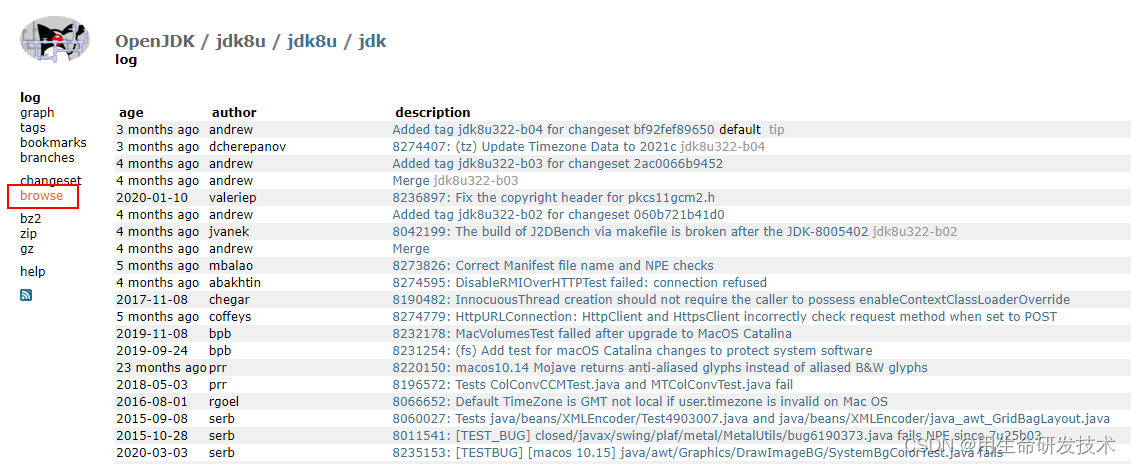 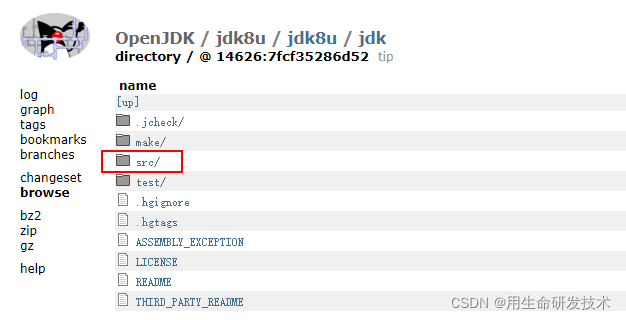 路径:directory /src/solaris/classes/sun/awt/ 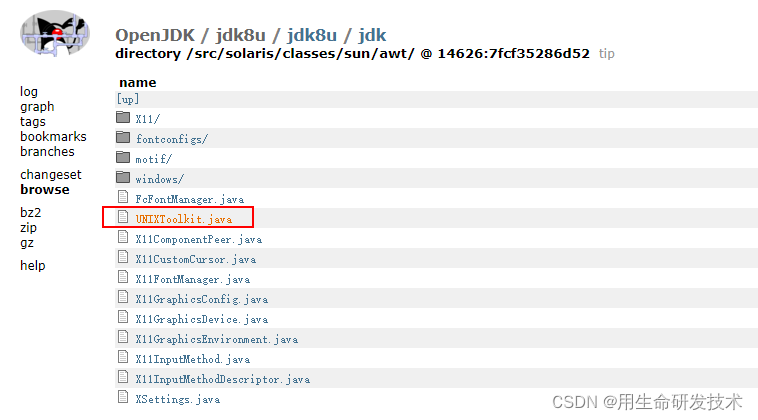 FontConfigManager同理,路径:src/solaris/classes/sun/font/FontConfigManager.java 。之后清理idea缓,这里我直接将这两个类的代码贴下来。
package sun.awt;
import java.awt.RenderingHints;
import static java.awt.RenderingHints.*;
import java.awt.color.ColorSpace;
import java.awt.image.*;
import java.security.AccessController;
import java.security.PrivilegedAction;
import sun.security.action.GetIntegerAction;
import com.sun.java.swing.plaf.gtk.GTKConstants.TextDirection;
import sun.java2d.opengl.OGLRenderQueue;
import sun.security.action.GetPropertyAction;
public abstract class UNIXToolkit extends SunToolkit {
public static final Object GTK_LOCK = new Object();
private static final int[] BAND_OFFSETS = {0, 1, 2};
private static final int[] BAND_OFFSETS_ALPHA = {0, 1, 2, 3};
private static final int DEFAULT_DATATRANSFER_TIMEOUT = 10000;
public enum GtkVersions {
ANY(0),
GTK2(Constants.GTK2_MAJOR_NUMBER),
GTK3(Constants.GTK3_MAJOR_NUMBER);
static class Constants {
static final int GTK2_MAJOR_NUMBER = 2;
static final int GTK3_MAJOR_NUMBER = 3;
}
final int number;
GtkVersions(int number) {
this.number = number;
}
public static GtkVersions getVersion(int number) {
switch (number) {
case Constants.GTK2_MAJOR_NUMBER:
return GTK2;
case Constants.GTK3_MAJOR_NUMBER:
return GTK3;
default:
return ANY;
}
}
public int getNumber() {
return number;
}
}
;
private Boolean nativeGTKAvailable;
private Boolean nativeGTKLoaded;
private BufferedImage tmpImage = null;
public static int getDatatransferTimeout() {
Integer dt = (Integer) AccessController.doPrivileged(
new GetIntegerAction("sun.awt.datatransfer.timeout"));
if (dt == null || dt <= 0) {
return DEFAULT_DATATRANSFER_TIMEOUT;
} else {
return dt;
}
}
@Override
public boolean isNativeGTKAvailable() {
synchronized (GTK_LOCK) {
if (nativeGTKLoaded != null) {
return nativeGTKLoaded;
} else if (nativeGTKAvailable != null) {
return nativeGTKAvailable;
} else {
boolean success = check_gtk(getEnabledGtkVersion().getNumber());
nativeGTKAvailable = success;
return success;
}
}
}
public boolean loadGTK() {
synchronized (GTK_LOCK) {
if (nativeGTKLoaded == null) {
nativeGTKLoaded = load_gtk(getEnabledGtkVersion().getNumber(),
isGtkVerbose());
}
}
return nativeGTKLoaded;
}
protected Object lazilyLoadDesktopProperty(String name) {
if (name.startsWith("gtk.icon.")) {
return lazilyLoadGTKIcon(name);
}
return super.lazilyLoadDesktopProperty(name);
}
protected Object lazilyLoadGTKIcon(String longname) {
Object result = desktopProperties.get(longname);
if (result != null) {
return result;
}
String str[] = longname.split("\\.");
if (str.length != 5) {
return null;
}
int size = 0;
try {
size = Integer.parseInt(str[3]);
} catch (NumberFormatException nfe) {
return null;
}
TextDirection dir = ("ltr".equals(str[4]) ? TextDirection.LTR :
TextDirection.RTL);
BufferedImage img = getStockIcon(-1, str[2], size, dir.ordinal(), null);
if (img != null) {
setDesktopProperty(longname, img);
}
return img;
}
public BufferedImage getGTKIcon(final String filename) {
if (!loadGTK()) {
return null;
} else {
synchronized (GTK_LOCK) {
if (!load_gtk_icon(filename)) {
tmpImage = null;
}
}
}
return tmpImage;
}
public BufferedImage getStockIcon(final int widgetType, final String stockId,
final int iconSize, final int direction,
final String detail) {
if (!loadGTK()) {
return null;
} else {
synchronized (GTK_LOCK) {
if (!load_stock_icon(widgetType, stockId, iconSize, direction, detail)) {
tmpImage = null;
}
}
}
return tmpImage;
}
public void loadIconCallback(byte[] data, int width, int height,
int rowStride, int bps, int channels, boolean alpha) {
tmpImage = null;
DataBuffer dataBuf = new DataBufferByte(data, (rowStride * height));
WritableRaster raster = Raster.createInterleavedRaster(dataBuf,
width, height, rowStride, channels,
(alpha ? BAND_OFFSETS_ALPHA : BAND_OFFSETS), null);
ColorModel colorModel = new ComponentColorModel(
ColorSpace.getInstance(ColorSpace.CS_sRGB), alpha, false,
ColorModel.TRANSLUCENT, DataBuffer.TYPE_BYTE);
tmpImage = new BufferedImage(colorModel, raster, false, null);
}
private static native boolean check_gtk(int version);
private static native boolean load_gtk(int version, boolean verbose);
private static native boolean unload_gtk();
private native boolean load_gtk_icon(String filename);
private native boolean load_stock_icon(int widget_type, String stock_id,
int iconSize, int textDirection, String detail);
private native void nativeSync();
private static native int get_gtk_version();
@Override
public void sync() {
nativeSync();
OGLRenderQueue.sync();
}
public static final String FONTCONFIGAAHINT = "fontconfig/Antialias";
@Override
protected RenderingHints getDesktopAAHints() {
Object aaValue = getDesktopProperty("gnome.Xft/Antialias");
if (aaValue == null) {
aaValue = getDesktopProperty(FONTCONFIGAAHINT);
if (aaValue != null) {
return new RenderingHints(KEY_TEXT_ANTIALIASING, aaValue);
} else {
return null;
}
}
boolean aa = ((aaValue instanceof Number)
&& ((Number) aaValue).intValue() != 0);
Object aaHint;
if (aa) {
String subpixOrder =
(String) getDesktopProperty("gnome.Xft/RGBA");
if (subpixOrder == null || subpixOrder.equals("none")) {
aaHint = VALUE_TEXT_ANTIALIAS_ON;
} else if (subpixOrder.equals("rgb")) {
aaHint = VALUE_TEXT_ANTIALIAS_LCD_HRGB;
} else if (subpixOrder.equals("bgr")) {
aaHint = VALUE_TEXT_ANTIALIAS_LCD_HBGR;
} else if (subpixOrder.equals("vrgb")) {
aaHint = VALUE_TEXT_ANTIALIAS_LCD_VRGB;
} else if (subpixOrder.equals("vbgr")) {
aaHint = VALUE_TEXT_ANTIALIAS_LCD_VBGR;
} else {
aaHint = VALUE_TEXT_ANTIALIAS_ON;
}
} else {
aaHint = VALUE_TEXT_ANTIALIAS_DEFAULT;
}
return new RenderingHints(KEY_TEXT_ANTIALIASING, aaHint);
}
private native boolean gtkCheckVersionImpl(int major, int minor,
int micro);
public boolean checkGtkVersion(int major, int minor, int micro) {
if (loadGTK()) {
return gtkCheckVersionImpl(major, minor, micro);
}
return false;
}
public static GtkVersions getEnabledGtkVersion() {
String version = AccessController.doPrivileged(
new GetPropertyAction("jdk.gtk.version"));
if (version == null) {
return GtkVersions.ANY;
} else if (version.startsWith("2")) {
return GtkVersions.GTK2;
} else if ("3".equals(version)) {
return GtkVersions.GTK3;
}
return GtkVersions.ANY;
}
public static GtkVersions getGtkVersion() {
return GtkVersions.getVersion(get_gtk_version());
}
public static boolean isGtkVerbose() {
return AccessController.doPrivileged((PrivilegedAction<Boolean>) ()
-> Boolean.getBoolean("jdk.gtk.verbose"));
}
}
package sun.font;
import java.util.Locale;
import sun.awt.SunHints;
import sun.awt.SunToolkit;
import sun.util.logging.PlatformLogger;
public class FontConfigManager {
static boolean fontConfigFailed = false;
private static final FontConfigInfo fcInfo = new FontConfigInfo();
public static class FontConfigFont {
public String familyName;
public String styleStr;
public String fullName;
public String fontFile;
}
public static class FcCompFont {
public String fcName;
public String fcFamily;
public String jdkName;
public int style;
public FontConfigFont firstFont;
public FontConfigFont[] allFonts;
public CompositeFont compFont;
}
public static class FontConfigInfo {
public int fcVersion;
public String[] cacheDirs = new String[4];
}
private static String[] fontConfigNames = {
"sans:regular:roman",
"sans:bold:roman",
"sans:regular:italic",
"sans:bold:italic",
"serif:regular:roman",
"serif:bold:roman",
"serif:regular:italic",
"serif:bold:italic",
"monospace:regular:roman",
"monospace:bold:roman",
"monospace:regular:italic",
"monospace:bold:italic",
};
private FcCompFont[] fontConfigFonts;
public FontConfigManager() {
}
public static Object getFontConfigAAHint() {
return getFontConfigAAHint("sans");
}
public static Object getFontConfigAAHint(String fcFamily) {
if (FontUtilities.isWindows) {
return null;
} else {
int hint = getFontConfigAASettings(getFCLocaleStr(), fcFamily);
if (hint < 0) {
return null;
} else {
return SunHints.Value.get(SunHints.INTKEY_TEXT_ANTIALIASING,
hint);
}
}
}
private static String getFCLocaleStr() {
Locale l = SunToolkit.getStartupLocale();
String localeStr = l.getLanguage();
String country = l.getCountry();
if (!country.equals("")) {
localeStr = localeStr + "-" + country;
}
return localeStr;
}
public static native int getFontConfigVersion();
public synchronized void initFontConfigFonts(boolean includeFallbacks) {
if (fontConfigFonts != null) {
if (!includeFallbacks || (fontConfigFonts[0].allFonts != null)) {
return;
}
}
if (FontUtilities.isWindows || fontConfigFailed) {
return;
}
long t0 = 0;
if (FontUtilities.isLogging()) {
t0 = System.nanoTime();
}
FcCompFont[] fontArr = new FcCompFont[fontConfigNames.length];
for (int i = 0; i < fontArr.length; i++) {
fontArr[i] = new FcCompFont();
fontArr[i].fcName = fontConfigNames[i];
int colonPos = fontArr[i].fcName.indexOf(':');
fontArr[i].fcFamily = fontArr[i].fcName.substring(0, colonPos);
fontArr[i].jdkName = FontUtilities.mapFcName(fontArr[i].fcFamily);
fontArr[i].style = i % 4;
}
getFontConfig(getFCLocaleStr(), fcInfo, fontArr, includeFallbacks);
FontConfigFont anyFont = null;
for (int i = 0; i < fontArr.length; i++) {
FcCompFont fci = fontArr[i];
if (fci.firstFont == null) {
if (FontUtilities.isLogging()) {
PlatformLogger logger = FontUtilities.getLogger();
logger.info("Fontconfig returned no font for " +
fontArr[i].fcName);
}
fontConfigFailed = true;
} else if (anyFont == null) {
anyFont = fci.firstFont;
}
}
if (anyFont == null) {
if (FontUtilities.isLogging()) {
PlatformLogger logger = FontUtilities.getLogger();
logger.info("Fontconfig returned no fonts at all.");
}
fontConfigFailed = true;
return;
} else if (fontConfigFailed) {
for (int i = 0; i < fontArr.length; i++) {
if (fontArr[i].firstFont == null) {
fontArr[i].firstFont = anyFont;
}
}
}
fontConfigFonts = fontArr;
if (FontUtilities.isLogging()) {
PlatformLogger logger = FontUtilities.getLogger();
long t1 = System.nanoTime();
logger.info("Time spent accessing fontconfig="
+ ((t1 - t0) / 1000000) + "ms.");
for (int i = 0; i < fontConfigFonts.length; i++) {
FcCompFont fci = fontConfigFonts[i];
logger.info("FC font " + fci.fcName + " maps to family " +
fci.firstFont.familyName +
" in file " + fci.firstFont.fontFile);
if (fci.allFonts != null) {
for (int f = 0; f < fci.allFonts.length; f++) {
FontConfigFont fcf = fci.allFonts[f];
logger.info("Family=" + fcf.familyName +
" Style=" + fcf.styleStr +
" Fullname=" + fcf.fullName +
" File=" + fcf.fontFile);
}
}
}
}
}
public PhysicalFont registerFromFcInfo(FcCompFont fcInfo) {
SunFontManager fm = SunFontManager.getInstance();
String fontFile = fcInfo.firstFont.fontFile;
int offset = fontFile.length() - 4;
if (offset <= 0) {
return null;
}
String ext = fontFile.substring(offset).toLowerCase();
boolean isTTC = ext.equals(".ttc");
PhysicalFont physFont = fm.getRegisteredFontFile(fontFile);
if (physFont != null) {
if (isTTC) {
Font2D f2d = fm.findFont2D(fcInfo.firstFont.familyName,
fcInfo.style,
FontManager.NO_FALLBACK);
if (f2d instanceof PhysicalFont) {
return (PhysicalFont) f2d;
} else {
return null;
}
} else {
return physFont;
}
}
physFont = fm.findJREDeferredFont(fcInfo.firstFont.familyName,
fcInfo.style);
if (physFont == null &&
fm.isDeferredFont(fontFile) == true) {
physFont = fm.initialiseDeferredFont(fcInfo.firstFont.fontFile);
if (physFont != null) {
if (isTTC) {
Font2D f2d = fm.findFont2D(fcInfo.firstFont.familyName,
fcInfo.style,
FontManager.NO_FALLBACK);
if (f2d instanceof PhysicalFont) {
return (PhysicalFont) f2d;
} else {
return null;
}
} else {
return physFont;
}
}
}
if (physFont == null) {
int fontFormat = SunFontManager.FONTFORMAT_NONE;
int fontRank = Font2D.UNKNOWN_RANK;
if (ext.equals(".ttf") || isTTC) {
fontFormat = SunFontManager.FONTFORMAT_TRUETYPE;
fontRank = Font2D.TTF_RANK;
} else if (ext.equals(".pfa") || ext.equals(".pfb")) {
fontFormat = SunFontManager.FONTFORMAT_TYPE1;
fontRank = Font2D.TYPE1_RANK;
}
physFont = fm.registerFontFile(fcInfo.firstFont.fontFile, null,
fontFormat, true, fontRank);
}
return physFont;
}
public CompositeFont getFontConfigFont(String name, int style) {
name = name.toLowerCase();
initFontConfigFonts(false);
if (fontConfigFonts == null) {
return null;
}
FcCompFont fcInfo = null;
for (int i = 0; i < fontConfigFonts.length; i++) {
if (name.equals(fontConfigFonts[i].fcFamily) &&
style == fontConfigFonts[i].style) {
fcInfo = fontConfigFonts[i];
break;
}
}
if (fcInfo == null) {
fcInfo = fontConfigFonts[0];
}
if (FontUtilities.isLogging()) {
FontUtilities.getLogger()
.info("FC name=" + name + " style=" + style +
" uses " + fcInfo.firstFont.familyName +
" in file: " + fcInfo.firstFont.fontFile);
}
if (fcInfo.compFont != null) {
return fcInfo.compFont;
}
FontManager fm = FontManagerFactory.getInstance();
CompositeFont jdkFont = (CompositeFont)
fm.findFont2D(fcInfo.jdkName, style, FontManager.LOGICAL_FALLBACK);
if (fcInfo.firstFont.familyName == null ||
fcInfo.firstFont.fontFile == null) {
return (fcInfo.compFont = jdkFont);
}
FontFamily family = FontFamily.getFamily(fcInfo.firstFont.familyName);
PhysicalFont physFont = null;
if (family != null) {
Font2D f2D = family.getFontWithExactStyleMatch(fcInfo.style);
if (f2D instanceof PhysicalFont) {
physFont = (PhysicalFont) f2D;
}
}
if (physFont == null ||
!fcInfo.firstFont.fontFile.equals(physFont.platName)) {
physFont = registerFromFcInfo(fcInfo);
if (physFont == null) {
return (fcInfo.compFont = jdkFont);
}
family = FontFamily.getFamily(physFont.getFamilyName(null));
}
for (int i = 0; i < fontConfigFonts.length; i++) {
FcCompFont fc = fontConfigFonts[i];
if (fc != fcInfo &&
physFont.getFamilyName(null).equals(fc.firstFont.familyName) &&
!fc.firstFont.fontFile.equals(physFont.platName) &&
family.getFontWithExactStyleMatch(fc.style) == null) {
registerFromFcInfo(fontConfigFonts[i]);
}
}
return (fcInfo.compFont = new CompositeFont(physFont, jdkFont));
}
public FcCompFont[] getFontConfigFonts() {
return fontConfigFonts;
}
private static native void getFontConfig(String locale,
FontConfigInfo fcInfo,
FcCompFont[] fonts,
boolean includeFallbacks);
void populateFontConfig(FcCompFont[] fcInfo) {
fontConfigFonts = fcInfo;
}
FcCompFont[] loadFontConfig() {
initFontConfigFonts(true);
return fontConfigFonts;
}
FontConfigInfo getFontConfigInfo() {
initFontConfigFonts(true);
return fcInfo;
}
private static native int
getFontConfigAASettings(String locale, String fcFamily);
}
六、程序包com.sun.tools.javac.api不存在
这是缺少tools的jar包所导致的。 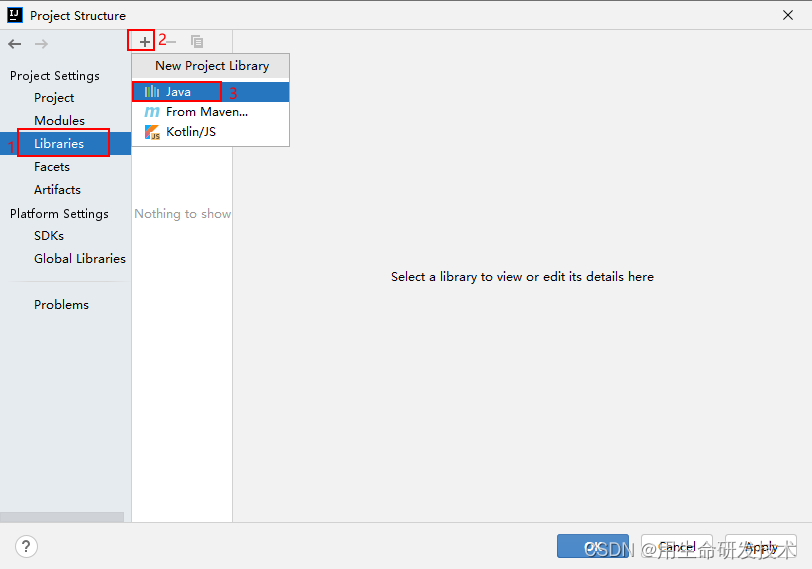 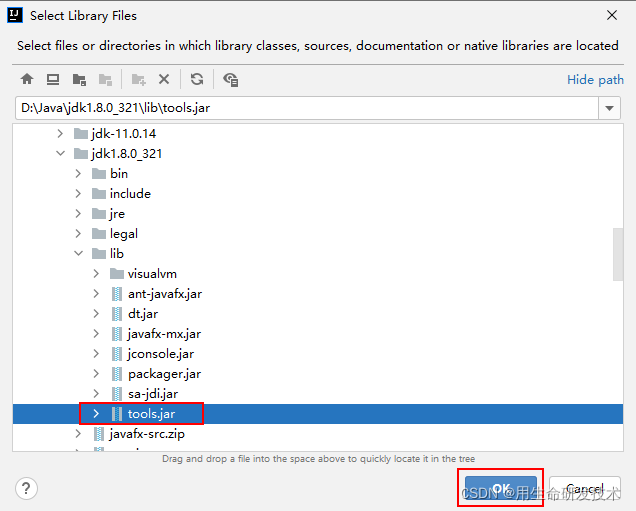
七、Compilation failed: internal java compiler error
将内存调至1700 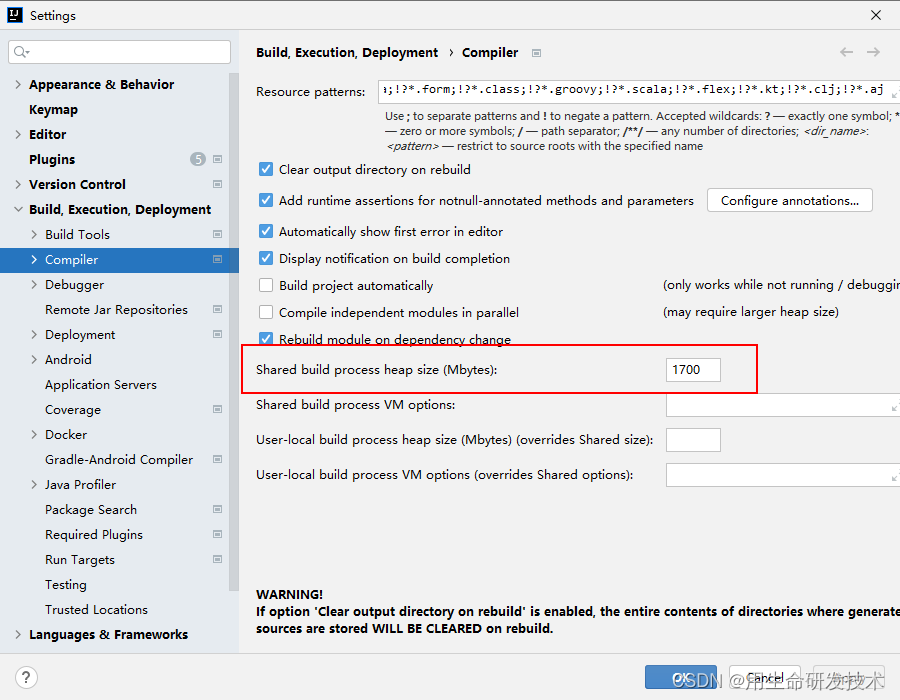
八、允许DEBUG进入源代码
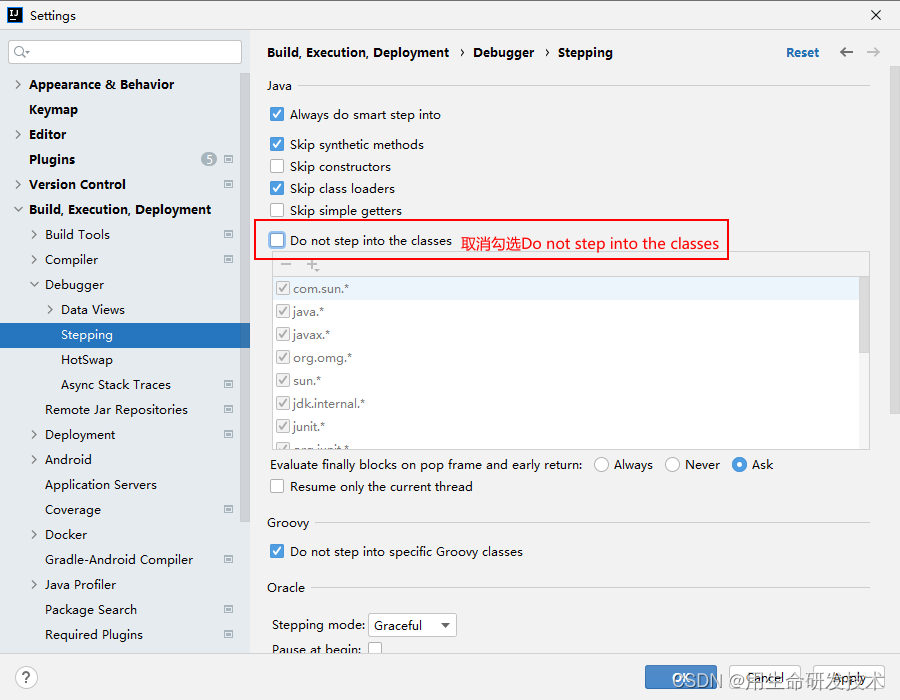
九、源码java: 警告: 源发行版 8 需要目标发行版 1.8
在搭建JDK1.7源码时,一直报源码java: 警告: 源发行版 8 需要目标发行版 1.8
检查Java Complier,修改为7 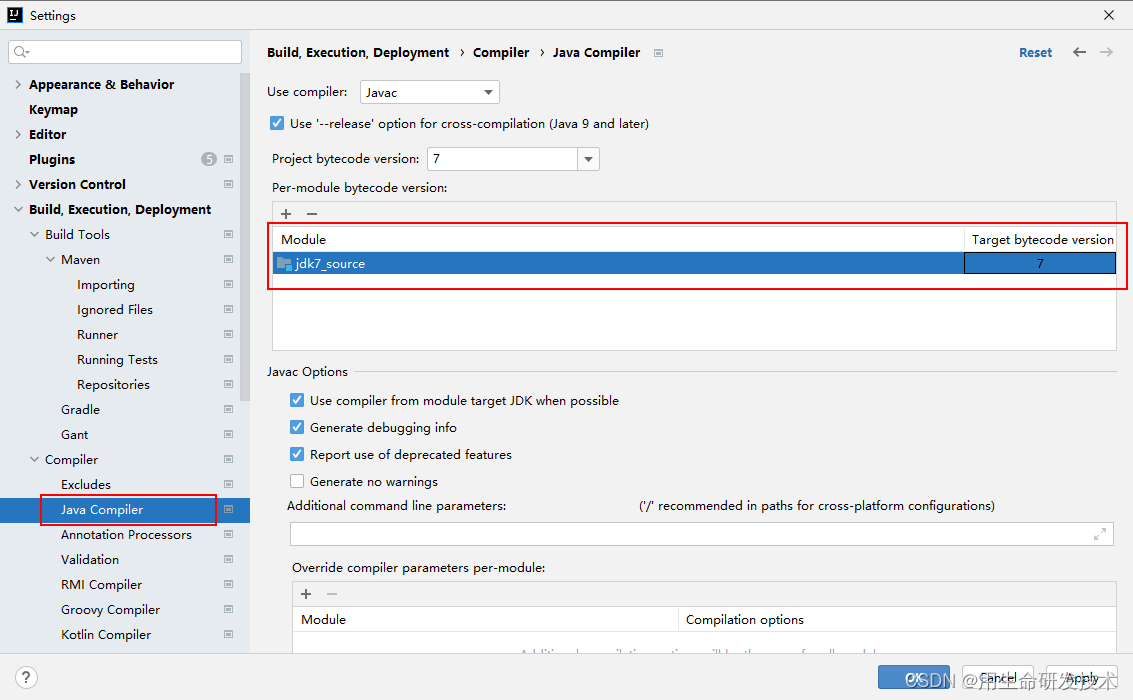 并且,将maven中Runner的JRE也改成JDK1.7,这个是个巨坑,找了好久。 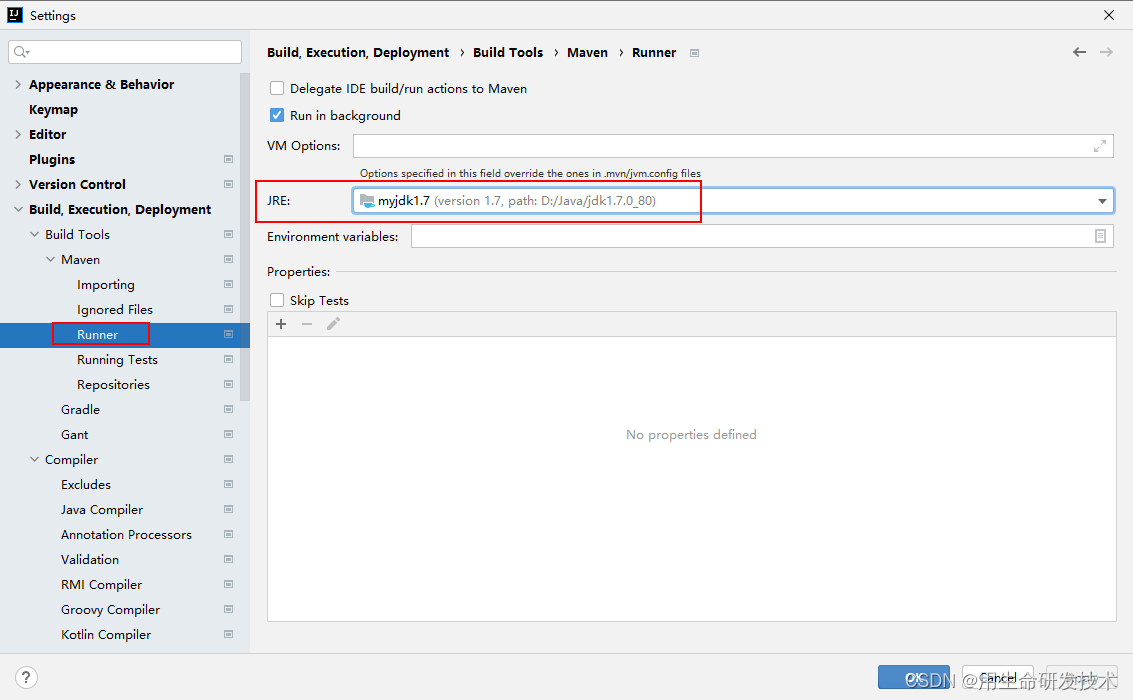
|