0. 代码下载
代码下载链接:mybatis-plus-demo的代码 里面有数据库表的生成文件 注意可能需要新建一个blog-demo的数据库
1.项目所需的依赖
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.1</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.70</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
2.配置文件
server:
port: 8082
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: mysql
url: jdbc:mysql://localhost:3306/blog-demo?useUnicode=true&characterEncoding=utf-8&serverTimezone=Asia/Shanghai
mvc:
view:
prefix: /
suffix: .html
mybatis-plus:
type-aliases-package: hhf.mybatisplusdemo.entity
mapper-locations: classpath:/hhf/mybatisplusdemo/mapper/*.xml
3.实体类
@Data
@NoArgsConstructor
@AllArgsConstructor
public class Student implements Serializable {
private static final long serialVersionUID = 1L;
@TableId(value = "id", type = IdType.AUTO)
private Integer id;
private Integer stuNum;
private String stuName;
private Integer age;
private String gender;
private LocalDate birthday;
}
4. mapper
StudentMapper继承了mybatis-plus的
@Mapper
public interface StudentMapper extends BaseMapper<Student> {
}
在springboot的启动类上添加注解 @MapperScan(“mapper接口的相对路径”)
5.配置mapper.xml文件
与mapper接口路径一致 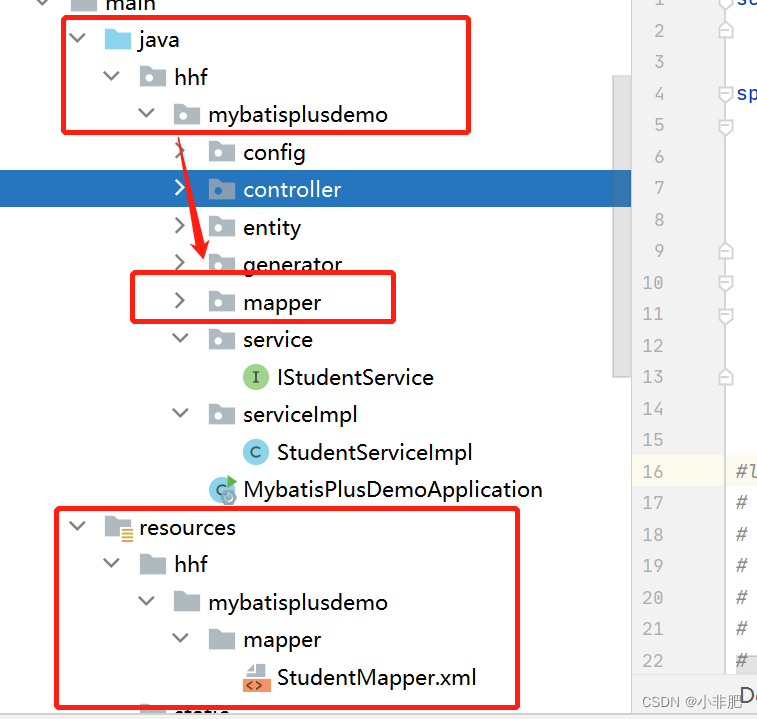 里面没有填写方法,是个空的
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="hhf.mybatisplusdemo.mapper.StudentMapper">
</mapper>
6.service
IStudentService继承了mybatis-plus的IService
@Service
public interface IStudentService extends IService<Student> {
}
7.serviceImpl
serviceImpl继承了mybatis-plus的serviceImpl并实现了IStudentService
@Service("IStudentService")
public class StudentServiceImpl extends ServiceImpl<StudentMapper, Student> implements IStudentService {
}
8.controller
controller类中写明了方法
@RestController
@RequestMapping("/student")
public class StudentController {
@Resource
private IStudentService iStudentService;
@GetMapping("/save")
public void save(){
Student student = new Student(44, 232323, "小芳", 19, "女", LocalDate.now());
boolean save = iStudentService.save(student);
}
@GetMapping("/deleteById")
public void deleteById(@PathParam("id") Integer id){
boolean b = iStudentService.removeById(id);
}
@GetMapping("/updateById")
public void updateById(){
Student student = new Student(44, 3453454, "小明", 19, "女", LocalDate.now());
boolean b = iStudentService.updateById(student);
}
@GetMapping("/getById")
public String getById(@PathParam("id") Integer id){
Student student = iStudentService.getById(id);
System.out.println(student);
return student.toString();
}
@GetMapping("/getOneById")
public Student getOneById(@PathParam("id") Integer id){
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("id",id);
Student student = iStudentService.getOne(queryWrapper);
return student;
}
@GetMapping("/getOneByStuName")
public Student getOneByStuName(@PathParam("stuName") String stuName){
System.out.println("------------------------------------------");
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("stu_name",stuName);
Student student = iStudentService.getOne(queryWrapper);
System.out.println("-----------------------------------------");
System.out.println(student.toString());
return student;
}
@GetMapping("/getStudentListBySex")
public List<Student> list(@PathParam("gender") String gender){
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("gender", gender);
List<Student> list = iStudentService.list(queryWrapper);
return list;
}
@GetMapping("/listStudentListByAge")
public List<Student> listStudentListByAge(@PathParam("age") Integer age){
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("age", age);
List<Student> list = iStudentService.list(queryWrapper);
return list;
}
}
|