第一步建立数据库建立数据库的表格:
建立数据库的第一张表
建立数据库的第二张表
建立项目:
构建MVC+SSM框架的三层架构
SSM框架所需要的架包
第二步:配置文件:
MyBatis框架:操作数据访问层.
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/testdb
jdbc.username=root
jdbc.password=123456
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<!-- 设置延迟加载开关,默认false(立即加载) -->
<setting name="lazyLoadingEnabled" value="false" />
<!-- 开启MyBatis二级缓存配置,默认已经开启,可以省略 -->
<setting name="cacheEnabled" value="true" />
<!-- 设置驼峰命名规则,会将表字段名user_name自动映射到属性名userName -->
<setting name="mapUnderscoreToCamelCase" value="true" />
</settings>
</configuration>
Spring框架:操作mvc三层.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<!--开启组件扫描 -->
<context:component-scan
base-package="Com.Day0604.Service" />
<!-- 引入外面的properties常量配置文件 -->
<context:property-placeholder
location="classpath:db.properties" />
<!-- 数据源配置 -->
<bean id="dataSource"
class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${jdbc.driver}" />
<property name="url" value="${jdbc.url}" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
</bean>
<!-- 事务管理器 -->
<bean id="transactionManager"
class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource" />
</bean>
<!-- 开启基于注解配置的事务管理 -->
<tx:annotation-driven
transaction-manager="transactionManager" />
<!-- 扫描mapper接口文件 -->
<bean id="mapperScannerConfigurer"
class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="Com.Day0604.Dao" />
</bean>
<!-- 创建sqlSession工厂 -->
<bean id="sessionFactory"
class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<!-- <property name="typeAliasesPackage" value="com.yhh.domain" /> -->
<!-- 如果还有一些专门针对于mybatis的配置,需要引入 -->
<property name="configLocation"
value="classpath:mybatis-config.xml" />
<!-- 配置mybatis分页插件PageHelper -->
<property name="plugins">
<array>
<bean class="com.github.pagehelper.PageInterceptor">
<property name="properties">
<value></value>
</property>
</bean>
</array>
</property>
</bean>
</beans>
SpringMvc框架:操作控制层.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!--开启组件扫描 -->
<context:component-scan
base-package="Com.Day0604.Controller" />
<!--开启mvc注解支持 -->
<mvc:annotation-driven />
<!--释放静态资源 -->
<mvc:default-servlet-handler />
</beans>
第三步:配置web-xml文件信息:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<welcome-file-list>
<welcome-file>index.html</welcome-file>
</welcome-file-list>
<!-- 监听并加载spring配置文件 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!--配置前端控制器-->
<servlet>
<servlet-name>dispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<!--配置DispatcherServlet需要的配置文件-->
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
<!--加载时机-->
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcherServlet</servlet-name>
<!-- 配置/意味着拦截除了jsp之外的所有请求 -->
<url-pattern>/</url-pattern>
</servlet-mapping>
<!--配置POST请求中文乱码过滤器-->
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<!--指定编码-->
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>*.do</url-pattern>
</filter-mapping>
</web-app>
第四步:查询操作:
<div align="center">
<h1>商品管理系统</h1>
<div id="navdiv">
<p> 一级目录:<input type="search" name="one" class="num"> </p>
<p> 二级目录:<input type="search" name="one" class="num"> </p>
<p><input type="button" value="查询"></p>
</div>
<table>
<tr>
<th>编号</th>
<th>商品名称</th>
<th>买点</th>
<th>售价</th>
<th>数量</th>
<th>更新日期</th>
<th>增加一条记录</th>
<th>修改一条记录</th>
<th>删除一条记录</th>
</tr>
</table>
</div>

开始访问服务器:编写Ajax请求:要求查询到的数据查询到本页面上:
//让服务器查数据 返回页面
$(function () {
$.ajax({
url: "list.do",
type: "GET",
data: "",
success: function (json) {
},
error: function () {
alert("请求失败")
},
dataType: "json"
});
});
控制器定义方法来接收浏览器的任务:
package Com.Day0604.Controller;
import Com.Day0604.Service.IItemService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
import java.util.Map;
/**
* 控制层
*/
@Controller
public class ItemController {
@Autowired
IItemService service;
// 定义方法
@RequestMapping("list.do")
@ResponseBody
public Object getList(){
System.out.println("开始查询数据");
List<Map<String, Object>> list=service.getList();
//list集合 [{},{},{},{},{}]
return ;
}
}
业务逻辑层的接口:
package Com.Day0604.Service;
import java.util.List;
import java.util.Map;
/**
* 业务层接口
*/
public interface IItemService {
List<Map<String, Object>> getList();
}
业务逻辑层的接口的实现方法的类:
package Com.Day0604.Service;
import Com.Day0604.Dao.IItemDao;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Map;
@Service(value = "sService")
public class ItemServiceImp implements IItemService{
@Autowired
IItemDao dao;
@Override
public List<Map<String, Object>> getList() {
return dao.getList();
}
}
数据访问层的接口:
package Com.Day0604.Dao;
import org.apache.ibatis.annotations.Select;
import java.util.List;
import java.util.Map;
/**
* 数据访问层
*/
public interface IItemDao {
@Select("select * from item")
List<Map<String, Object>> getList();
}
层层返回到控制层:
package Com.Day0604.Controller;
import Com.Day0604.Service.IItemService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
import java.util.Map;
/**
* 控制层
*/
@Controller
public class ItemController {
@Autowired
IItemService service;
// 定义方法
@RequestMapping("list.do")
@ResponseBody
public Object getList(){
System.out.println("开始查询数据");
List<Map<String, Object>> list=service.getList();
//list集合 [{},{},{},{},{}]
return list;
}
}
在index.html页面中定义方法解析JSON:
// 定义方法解析json格式
function parseTable(json) {
for (var i = 0; i < json.length; i++) {
$("table").append("<tr>" +
"<td>" + json[i].id + "</td>" +
"<td>" + json[i].title + "</td>" +
"<td>" + json[i].sell_point + "</td>" +
"<td>" + json[i].price + "</td>" +
"<td>" + json[i].num + "</td>" +
"<td>" + json[i].updated + "</td>" +
"<td><input type='button' value='增加一条记录' class='I'></td>" +
"<td><input type='button' value='修改一条记录' class='U'></td>" +
"<td><input type='button' value='删除一条记录' class='D'></td>" +
"</tr>")
}
}
调用方法:
//让服务器查数据 返回页面
$(function () {
$.ajax({
url: "list.do",
type: "GET",
data: "",
success: function (json) {
//list集合 [{},{},{},{},{}]
// 解析数据
parseTable(json);
},
error: function () {
alert("请求失败")
},
dataType: "json"
});
});
运行结果:
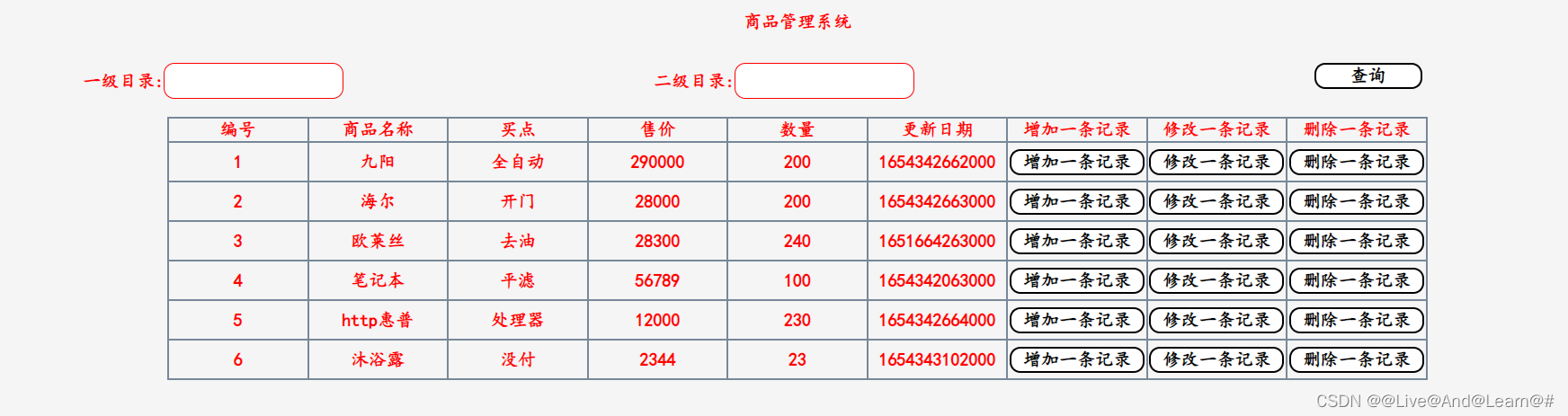
?总结 第一点:查询数据的核心要义: ? ? ?* ?如果用户要查整张表的信息要做以下分析 ? ? ?* 1 表中查到的是一条记录 那你又可以这样做 在java中定义了 Map集合 Map<String, Object> ? ? ?* 2 如果查到的是两条记录或者是多条记录 外表用到是list集合 ?里面是MAP集合 ? ? ?* 3所以多条记录利用的是 list中调用map集合 ?List<Map<String, Object>> getCarList() ? ? ?* 如果用户查询表的一个字段 中有多条记录 ? ? ?* 1多条记录利用List集合 一个字段利用的是String存放 ? ? ?* 2所以使用的是list中调用String ? ?List<String>
查询功能到这里结束了:各位学习者要多学多去写几遍:主要是思路的理解:
?
|