1.Objects
操作对象
1.1 对象判空
Integer integer = new Integer(1);
if (Objects.isNull(integer)) {
System.out.println("对象为空");
}
if (Objects.nonNull(integer)) {
System.out.println("对象不为空");
}
1.2 对象为空抛异常
Integer integer1 = new Integer(128);
Objects.requireNonNull(integer1);
Objects.requireNonNull(integer1, "参数不能为空");
Objects.requireNonNull(integer1, () -> "参数不能为空");
1.3 判断两个对象是否相等
Integer integer1 = new Integer(1);
Integer integer2 = new Integer(1);
System.out.println(Objects.equals(integer1, integer2));
true
Integer integer1 = new Integer(1);
Long integer2 = new Long(1);
System.out.println(Objects.equals(integer1, integer2));
false
1.4 获取对象的hashCode
String str = new String("abc");
System.out.println(Objects.hashCode(str));
96354
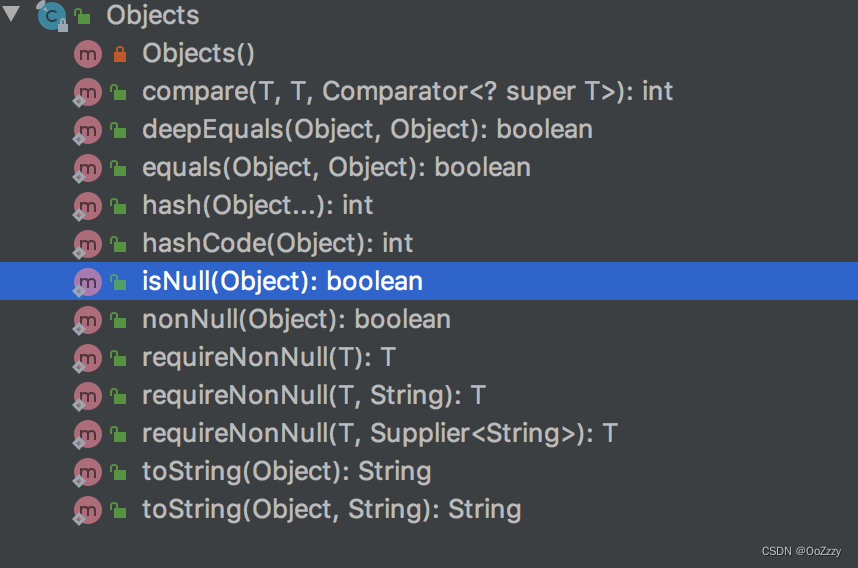
2.BooleanUtils
BooleanUtils类处理布尔值
2.1 判断true或false
Boolean aBoolean = new Boolean(true);
System.out.println(BooleanUtils.isTrue(aBoolean));
System.out.println(BooleanUtils.isFalse(aBoolean));
2.2 判断不为true或不为false
Boolean aBoolean = new Boolean(true);
Boolean aBoolean1 = null;
System.out.println(BooleanUtils.isNotTrue(aBoolean));
System.out.println(BooleanUtils.isNotTrue(aBoolean1));
System.out.println(BooleanUtils.isNotFalse(aBoolean));
System.out.println(BooleanUtils.isNotFalse(aBoolean1));
false
true
true
true
2.3 转换成数字
Boolean aBoolean = new Boolean(true);
Boolean aBoolean1 = new Boolean(false);
System.out.println(BooleanUtils.toInteger(aBoolean));
System.out.println(BooleanUtils.toInteger(aBoolean1));
1
0
2.4 Boolean转换成布尔值
包装类Boolean对象,转换成原始的boolean对象
Boolean aBoolean = new Boolean(true);
Boolean aBoolean1 = null;
System.out.println(BooleanUtils.toBoolean(aBoolean));
System.out.println(BooleanUtils.toBoolean(aBoolean1));
System.out.println(BooleanUtils.toBooleanDefaultIfNull(aBoolean1, false));
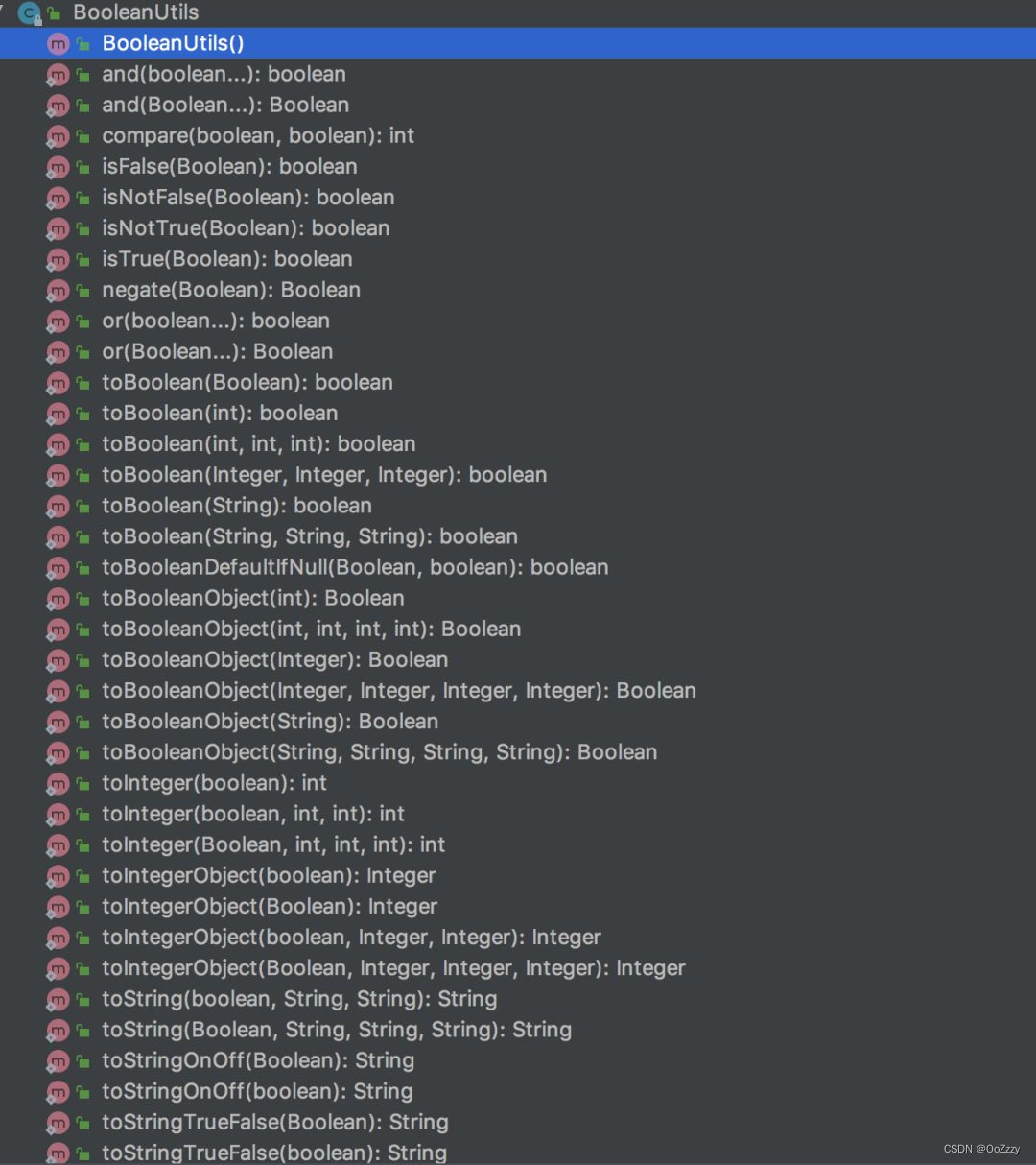
3.StringUtils
org.apache.commons.lang3包下的StringUtils工具类
3.1 字符串判空
null, “”," ",“null”
String str1 = null;
String str2 = "";
String str3 = " ";
String str4 = "abc";
System.out.println(StringUtils.isEmpty(str1));
System.out.println(StringUtils.isEmpty(str2));
System.out.println(StringUtils.isEmpty(str3));
System.out.println(StringUtils.isEmpty(str4));
System.out.println("=====");
System.out.println(StringUtils.isNotEmpty(str1));
System.out.println(StringUtils.isNotEmpty(str2));
System.out.println(StringUtils.isNotEmpty(str3));
System.out.println(StringUtils.isNotEmpty(str4));
System.out.println("=====");
System.out.println(StringUtils.isBlank(str1));
System.out.println(StringUtils.isBlank(str2));
System.out.println(StringUtils.isBlank(str3));
System.out.println(StringUtils.isBlank(str4));
System.out.println("=====");
System.out.println(StringUtils.isNotBlank(str1));
System.out.println(StringUtils.isNotBlank(str2));
System.out.println(StringUtils.isNotBlank(str3));
System.out.println(StringUtils.isNotBlank(str4));
true
true
false
false
=====
false
false
true
true
=====
true
true
true
false
=====
false
false
false
true
优先推荐使用isBlank和isNotBlank方法,因为它会把" "也考虑进去。
3.2 分隔字符串
分隔字符串是常见需求,如果直接使用String类的split方法,就可能会出现空指针异常。
String str1 = null;
System.out.println(StringUtils.split(str1,","));
System.out.println(str1.split(","));
null
Exception in thread "main" java.lang.NullPointerException
at com.sue.jump.service.test1.UtilTest.main(UtilTest.java:21)
使用StringUtils的split方法会返回null,而使用String的split方法会报指针异常。
3.3 判断是否纯数字
给定一个字符串,判断它是否为纯数字,可以使用isNumeric方法。
String str1 = "123";
String str2 = "123q";
String str3 = "0.33";
System.out.println(StringUtils.isNumeric(str1));
System.out.println(StringUtils.isNumeric(str2));
System.out.println(StringUtils.isNumeric(str3));
true
false
false
3.4 将集合拼接成字符串
List<String> list = Lists.newArrayList("a", "b", "c");
List<Integer> list2 = Lists.newArrayList(1, 2, 3);
System.out.println(StringUtils.join(list, ","));
System.out.println(StringUtils.join(list2, " "));
a,b,c
1 2 3
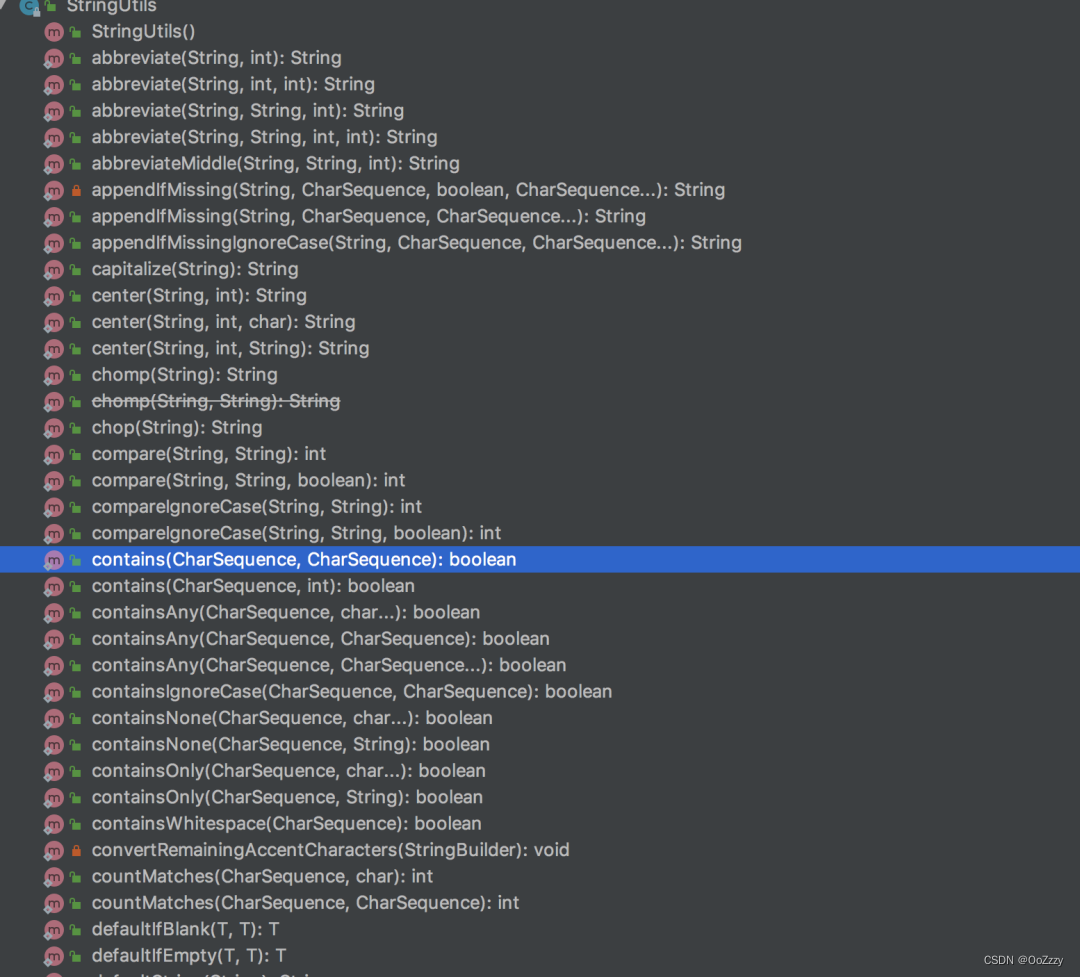
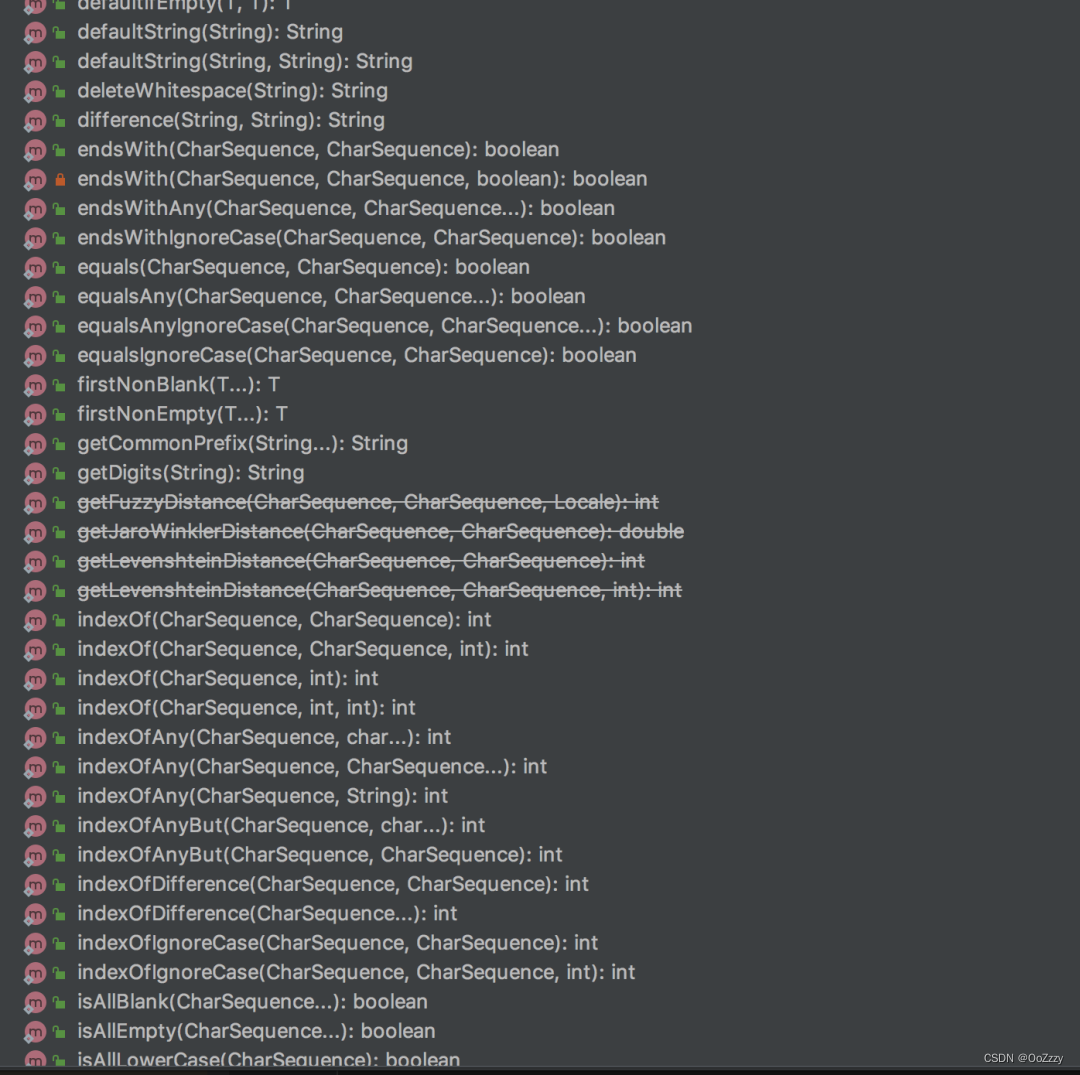
4.Assert
spring给我们提供了Assert类,它表示断言 如果不满足条件,则抛异常
4.1 断言参数是否为空
String str = null;
Assert.isNull(str, "str必须为空");
Assert.isNull(str, () -> "str必须为空");
Assert.notNull(str, "str不能为空");
如果不满足条件就会抛出IllegalArgumentException异常。
4.2 断言集合是否为空
List<String> list = null;
Map<String, String> map = null;
Assert.notEmpty(list, "list不能为空");
Assert.notEmpty(list, () -> "list不能为空");
Assert.notEmpty(map, "map不能为空");
如果不满足条件就会抛出IllegalArgumentException异常。
4.3 断言条件是否为空
断言是否满足某个条件,如果不满足条件,则直接抛异常。
List<String> list = null;
Assert.isTrue(CollectionUtils.isNotEmpty(list), "list不能为空");
Assert.isTrue(CollectionUtils.isNotEmpty(list), () -> "list不能为空");
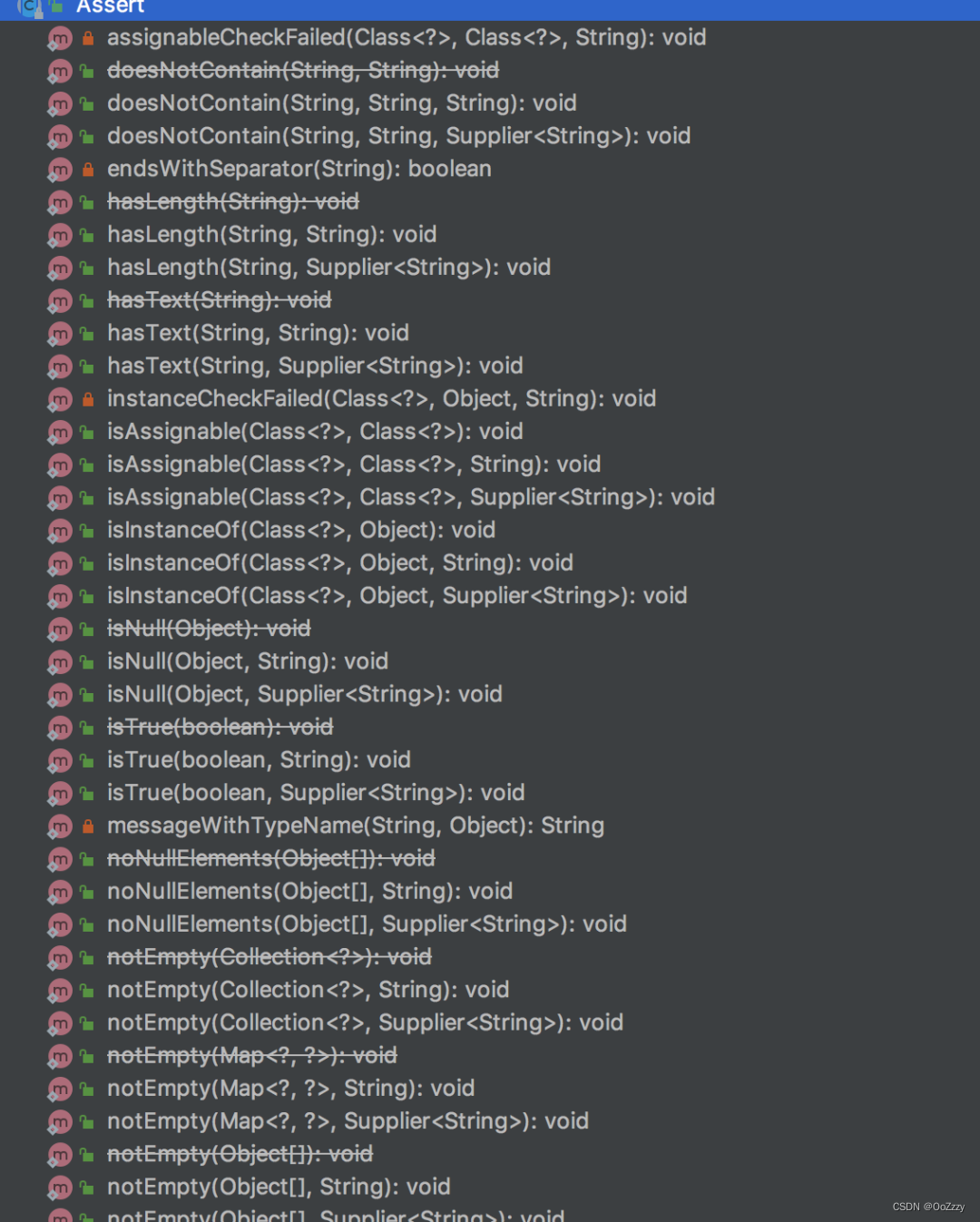
|