1. 字符串相关类String 、StringBuffer 、StringBuilder
1.1 String
概述
- String声明为final的,不可被继承
- String实现了Serializable接口:表示字符串是支持序列化的。 实现了Comparable接口:表示String可以比较大小。
- String内部定义了final char[] value用于存储字符串数据。
- 通过字面量的方式(区别于new给一个字符串赋值,此时的字符串值声明在字符串常量池中)。
- 字符串常量池中是不会存储相同内容(使用String类的equals()比较,返回true)的字符串的。
String实例化 String实例化有如下4种,
String s1 = "Hello World";
Strng s2 = new String();
String s3 = new String(String对象);
String s4 = new String(char[] a);
String s5 = new String(char[] a,int startIndex, int endIndex);
每种的内存解析如下: 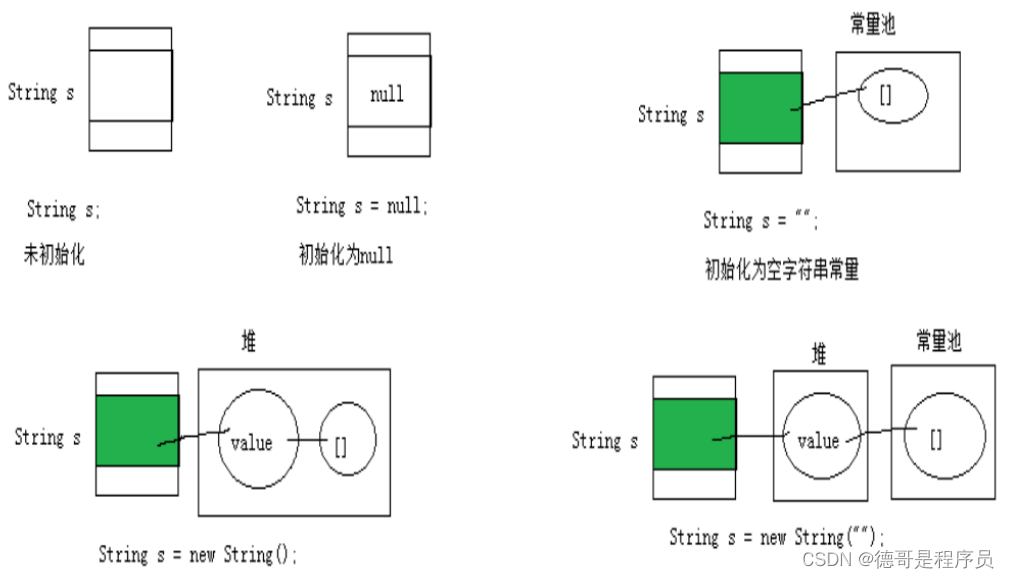 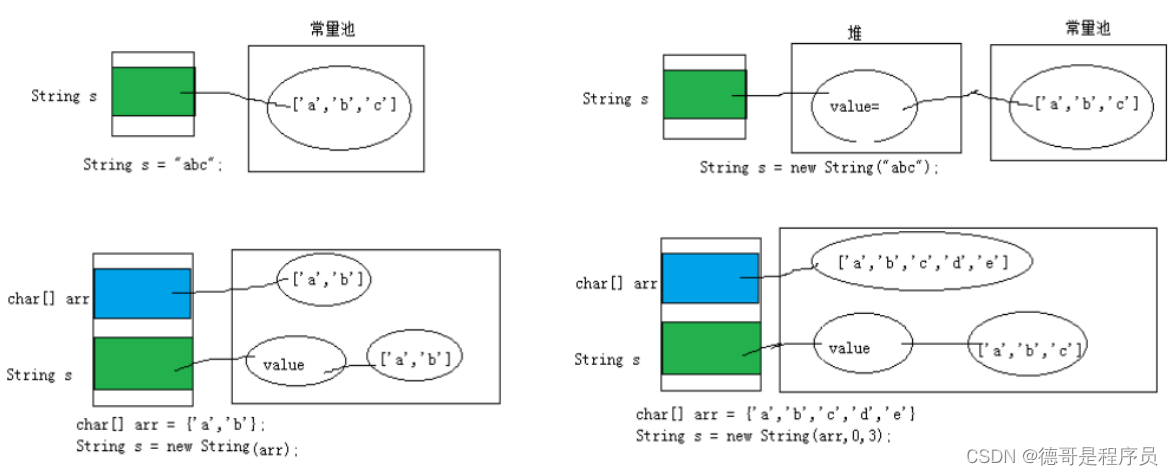 小练习 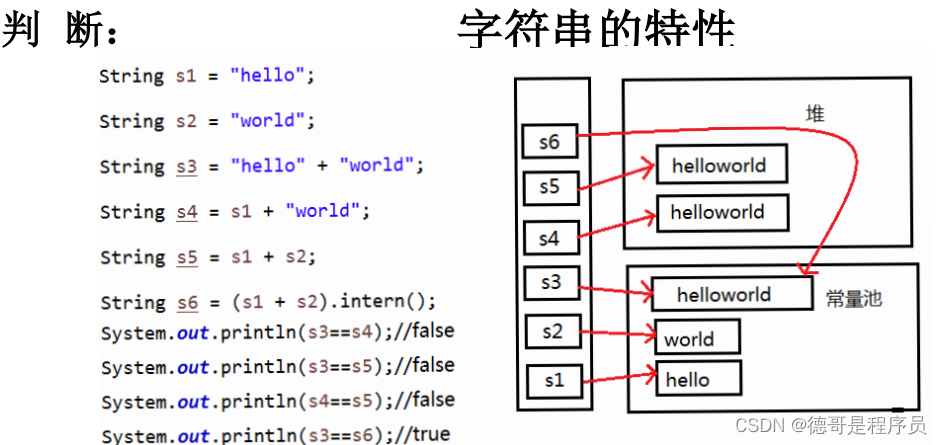 结论:
- 常量与常量的拼接结果在常量池。且常量池中不会存在相同内容的常量。
- 只要其中有一个是变量,结果就在堆中
- 如果拼接的结果调用intern()方法,返回值就在常量池中
常用方法 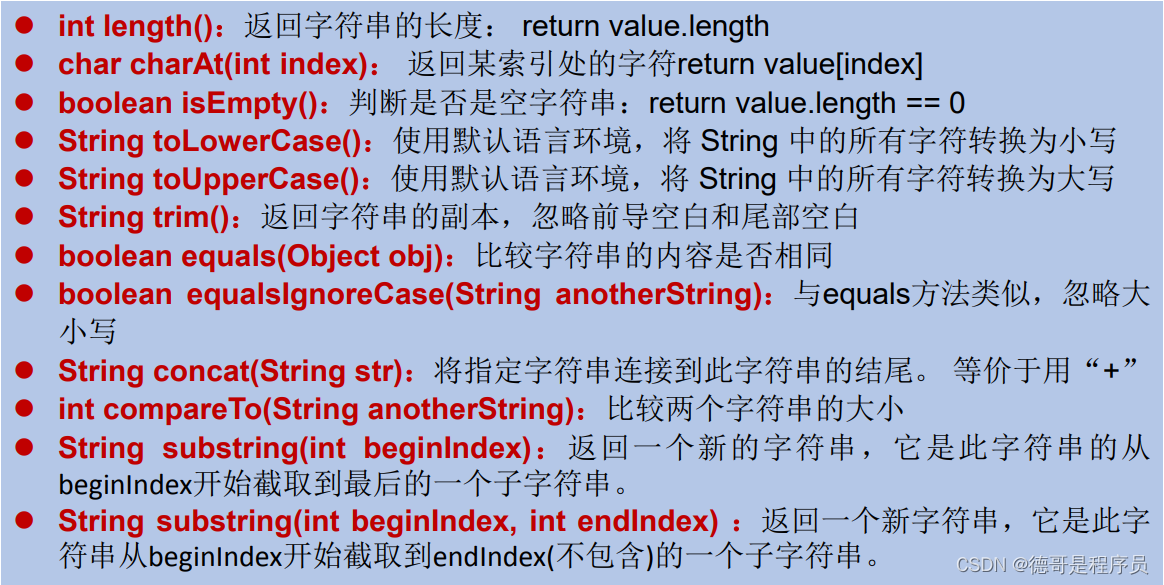 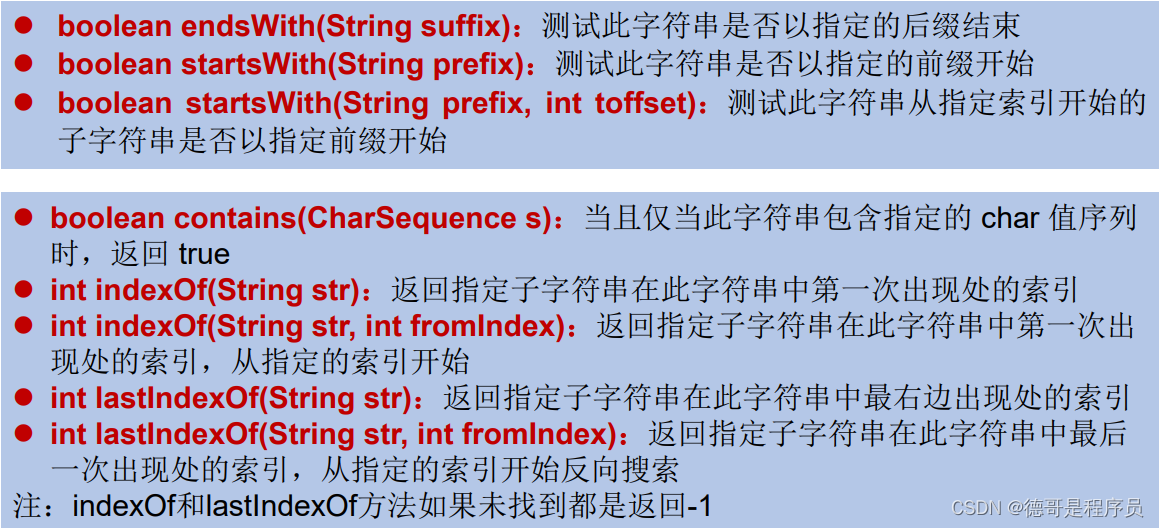 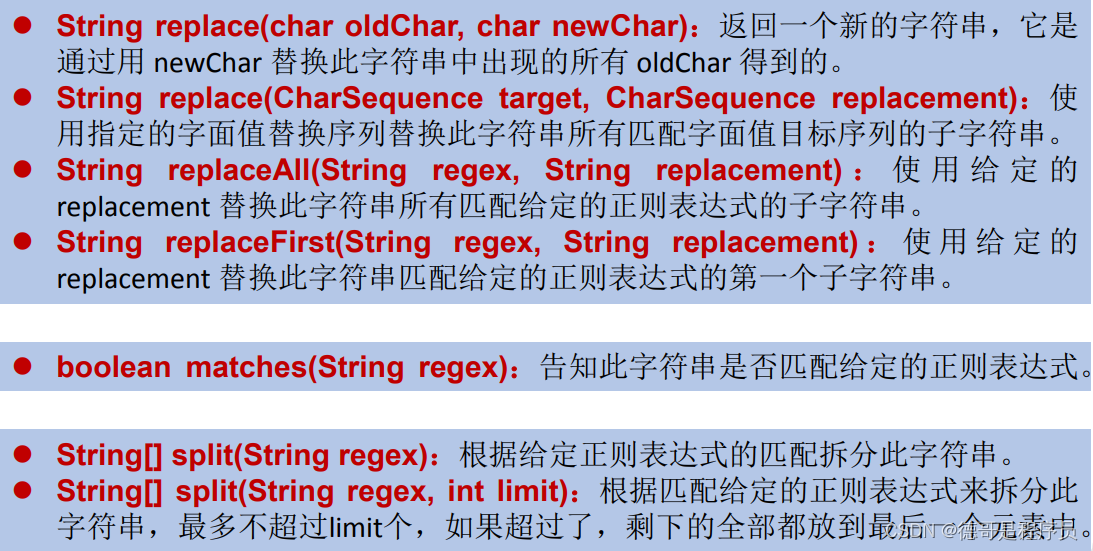
1.2 StringBuffer && StringBuilder
java.lang.StringBuffer代表可变的字符序列,JDK1.0中声明,可以对字符串内容进行增删,此时不会产生新的对象,很多方法与String相同。作为参数传递时,方法内部可以改变值。StringBuffer类不同于String,其对象必须使用构造器生成。有三个构造器:
StringBuffer():初始容量为16的字符串缓冲区 StringBuffer(int size):构造指定容量的字符串缓冲区 StringBuffer(String str):将内容初始化为指定字符串内容
常用方法   补充说明:当使用insert、append增加元素时,底层数组容量不足时,默认情况下,扩容为原来容量的2倍 + 2,同时将原数组中的元素复制到新的数组中。
至于StringBuilder,和 StringBuffer 非常类似,均代表可变的字符序列,而且提供相关功能的方法也一样,在此不多赘述。 String、StringBuffer、StringBuilder比较?
String:不可变的字符序列;底层使用char[]存储 StringBuffer:可变的字符序列;线程安全的,效率低;底层使用char[]存储 StringBuilder:可变的字符序列;jdk5.0新增的,线程不安全的,效率高;底层使用char[]存储
2. JDK8前的时间日期API
架构图 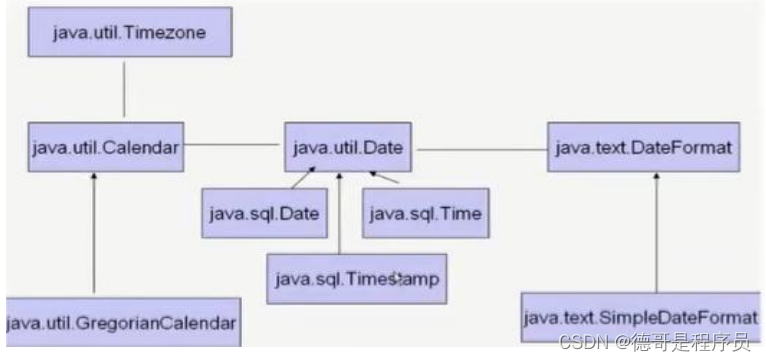 1. java.lang.System类 System类提供的public static long currentTimeMillis()用来返回当前时间与1970年1月1日0时0分0秒之间以毫秒为单位的时间差。此方法适于计算时间差。 栗子:
long start = System.currentTimeMillis();
for (int i = 0; i < 10000; i++) {
}
long end = System.currentTimeMillis();
System.out.println("循环花费时间:" + (end - start) + "ms");
2. java.util.Date类
Date():使用无参构造器创建的对象可以获取本地当前时间。 Date(long date)
getTime():返回自 1970 年 1 月 1 日 00:00:00 GMT 以来此 Date 对象表示的毫秒数。 toString():把此 Date 对象转换为以下形式的 String: dow mon dd hh:mm:ss zzz yyyy 其中: dow 是一周中的某一天 (Sun, Mon, Tue,Wed, Thu, Fri, Sat),zzz是时间标准。 其它很多方法都过时了。
Date date = new Date();
System.out.println(date);
long time = date.getTime();
System.out.println(time);
System.out.println(date.toString());
3. java.text.SimpleDateFormat类
- Date类的API不易于国际化,大部分被废弃了,java.text.SimpleDateFormat类是一个不与语言环境有关的方式来格式化和解析日期的具体类。它允许进行格式化:日期->文本、解析:文本->日期
- 格式化:
SimpleDateFormat() :默认的模式和语言环境创建对象 public SimpleDateFormat(String pattern):该构造方法可以用参数pattern 指定的格式创建一个对象,该对象调用: public String format(Date date):方法格式化时间对象date
public Date parse(String source):从给定字符串的开始解析文本,以生成 一个日期。
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日");
System.out.println(sdf.format(date));
String text = "2022年06月13日";
try {
System.out.println(sdf.parse(text));
} catch (ParseException e) {
e.printStackTrace();
}
4. java.util.Calendar(日历)类
- Calendar是一个抽象基类,主用用于完成日期字段之间相互操作的功能。
- 获取Calendar实例的方法
使用Calendar.getInstance()方法 调用它的子类GregorianCalendar的构造器。
- 一个Calendar的实例是系统时间的抽象表示,通过get(int field)方法来取得想要的时间信息。比如YEAR、MONTH、DAY_OF_WEEK、HOUR_OF_DAY 、MINUTE、SECOND
public void set(int field,int value) public void add(int field,int amount) public final Date getTime() public final void setTime(Date date)
获取月份时:一月是0,二月是1,以此类推,12月是11 获取星期时:周日是1,周二是2 , 。。。。周六是7
Calendar calendar = Calendar.getInstance();
System.out.println(calendar.getTime());
System.out.println(calendar.get(Calendar.DAY_OF_MONTH));
Date date = new Date(123456543234L);
calendar.setTime(date);
System.out.println(calendar.getTime());
3. JDK8中新日期时间API
旧时间API面临的问题如下: 可变性:像日期和时间这样的类应该是不可变的。 偏移性:Date中的年份是从1900开始的,而月份都从0开始。 格式化:格式化只对Date有用,Calendar则不行。 此外,它们也不是线程安全的;不能处理闰秒等。
新时间API涉及到的包: 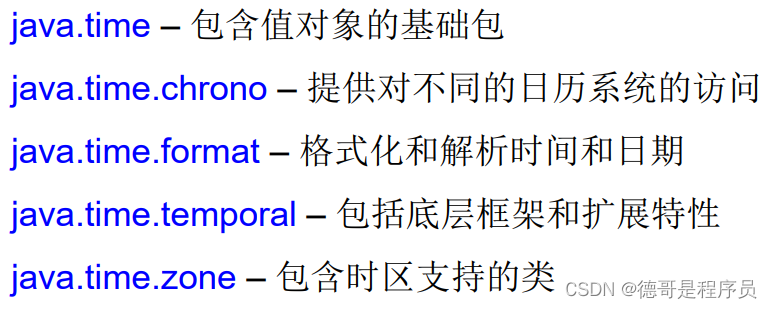
3.1 本地日期、本地时间、本地日期时间的使用:LocalDate / LocalTime / LocalDateTime
常用的方法: 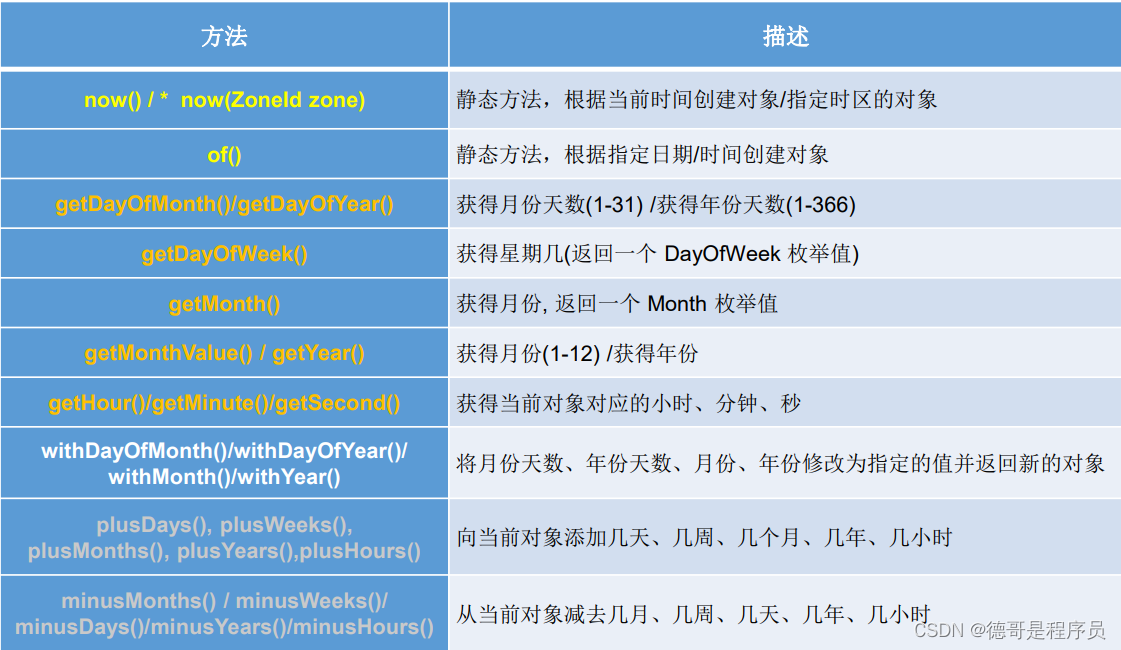
LocalDate localDate = LocalDate.now();
LocalTime localTime = LocalTime.now();
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println(localDate);
System.out.println(localTime);
System.out.println(localDateTime);
LocalDateTime localDateTime1 = LocalDateTime.of(2020, 10, 6, 13, 23, 43);
System.out.println(localDateTime1);
System.out.println(localDateTime.getDayOfMonth());
System.out.println(localDateTime.getDayOfWeek());
System.out.println(localDateTime.getMonth());
System.out.println(localDateTime.getMonthValue());
System.out.println(localDateTime.getMinute());
LocalDate localDate1 = localDate.withDayOfMonth(22);
System.out.println(localDate);
System.out.println(localDate1);
LocalDateTime localDateTime2 = localDateTime.withHour(4);
System.out.println(localDateTime);
System.out.println(localDateTime2);
LocalDateTime localDateTime3 = localDateTime.plusMonths(3);
System.out.println(localDateTime);
System.out.println(localDateTime3);
LocalDateTime localDateTime4 = localDateTime.minusDays(6);
System.out.println(localDateTime);
System.out.println(localDateTime4);
3.2 瞬时类:Instant
java.time包通过值类型Instant提供机器视图,不提供处理人类意义上的时间单位。Instant表示时间线上的一点,而不需要任何上下文信息,它只是简单的表示自1970年1月1日0时0分0秒(UTC)开始的秒 数。因为java.time包是基于纳秒计算的,所以Instant的精度可以达到纳秒级。 常用方法: 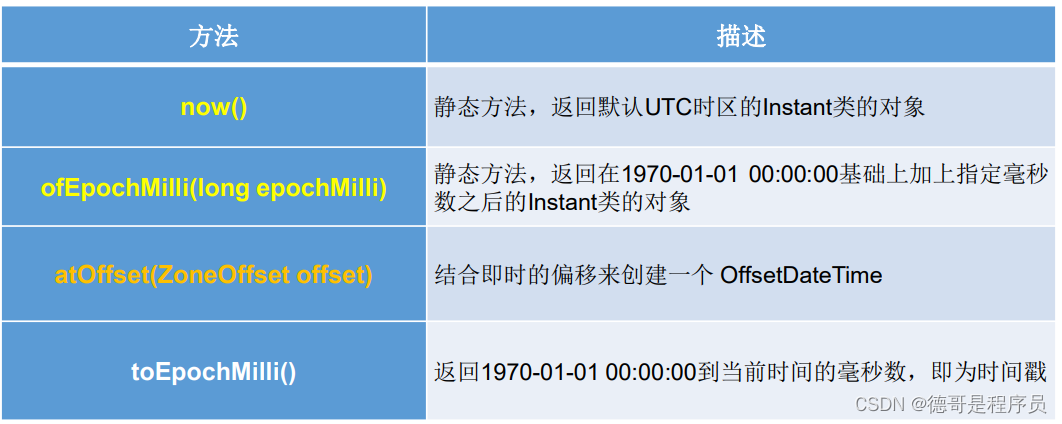
Instant instant = Instant.now();
System.out.println(instant);
OffsetDateTime offsetDateTime = instant.atOffset(ZoneOffset.ofHours(8));
System.out.println(offsetDateTime);
long milli = instant.toEpochMilli();
System.out.println(milli);
Instant instant1 = Instant.ofEpochMilli(1550475314878L);
System.out.println(instant1);
3.3. 日期时间格式化类:DateTimeFormatter
实例化: 预定义的标准格式。如:ISO_LOCAL_DATE_TIME;ISO_LOCAL_DATE;ISO_LOCAL_TIME 本地化相关的格式。如:ofLocalizedDateTime(FormatStyle.LONG) 自定义的格式。如:ofPattern(“yyyy-MM-dd hh:mm:ss”) 常用方法: 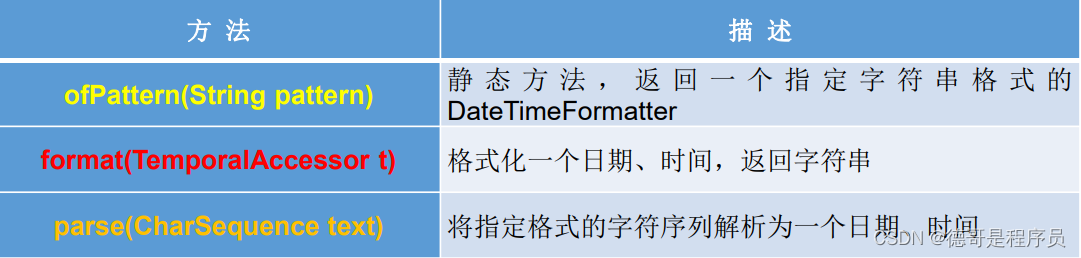
DateTimeFormatter formatter = DateTimeFormatter.ISO_LOCAL_DATE_TIME;
LocalDateTime localDateTime = LocalDateTime.now();
String str1 = formatter.format(localDateTime);
System.out.println(localDateTime);
System.out.println(str1);
TemporalAccessor parse = formatter.parse("2019-02-18T15:42:18.797");
System.out.println(parse);
DateTimeFormatter formatter1 = DateTimeFormatter.ofLocalizedDateTime(FormatStyle.LONG);
String str2 = formatter1.format(localDateTime);
System.out.println(str2);
DateTimeFormatter formatter2 = DateTimeFormatter.ofLocalizedDate(FormatStyle.MEDIUM);
String str3 = formatter2.format(LocalDate.now());
System.out.println(str3);
DateTimeFormatter formatter3 = DateTimeFormatter.ofPattern("yyyy-MM-dd hh:mm:ss");
String str4 = formatter3.format(LocalDateTime.now());
System.out.println(str4);
TemporalAccessor accessor = formatter3.parse("2019-02-18 03:52:09");
System.out.println(accessor);
4. Java比较器
4.1 自然排序:实现Comparable接口
实现Comparable接口要重写compareTo方法,重写规则如下:
如果当前对象this大于形参对象obj,则返回正整数, 如果当前对象this小于形参对象obj,则返回负整数, 如果当前对象this等于形参对象obj,则返回零。
栗子:
public class Test {
public static void main(String[] args) {
Goods[] goodArr = new Goods[4];
goodArr[0] = new Goods("红楼梦",100);
goodArr[1] = new Goods("西游记",85);
goodArr[2] = new Goods("水浒传",90);
goodArr[3] = new Goods("三国演义",95);
Arrays.sort(goodArr);
for (Goods good : goodArr) {
System.out.println(good);
}
}
}
class Goods implements Comparable{
private String name;
private int price;
public Goods(String name, int price){
this.name = name;
this.price = price;
}
@Override
public int compareTo(Object o) {
if(o instanceof Goods){
Goods good = (Goods) o;
if(this.price > good.price){
return 1;
}else if(this.price < good.price){
return -1;
}else{
return 0;
}
}
throw new RuntimeException("输入数据异常!");
}
@Override
public String toString(){
return "商品:" + name + " 价格:" + price;
}
}
4.2 定制排序
当元素的类型没实现java.lang.Comparable接口而又不方便修改代码,或者实现java.lang.Comparable接口的排序规则不适合当前的操作,那么可以考虑使用 Comparator 的对象来排序。实现定制排序要重写compare(Object o1,Object o2)方法,比较o1和o2的大小,重写规则如下:
如果方法返回正整数,则表示o1大于o2; 如果返回0,表示相等; 返回负整数,表示o1小于o2。 栗子:
public class Test {
public static void main(String[] args) {
Goods[] goodArr = new Goods[4];
goodArr[0] = new Goods("红楼梦",100);
goodArr[1] = new Goods("西游记",85);
goodArr[2] = new Goods("水浒传",90);
goodArr[3] = new Goods("三国演义",95);
Arrays.sort(goodArr, new Comparator<Goods>() {
@Override
public int compare(Goods o1, Goods o2) {
return o1.getName().compareTo(o2.getName());
}
});
for (Goods good : goodArr) {
System.out.println(good);
}
}
}
class Goods implements Comparable{
private String name;
private int price;
public Goods(String name, int price){
this.name = name;
this.price = price;
}
public String getName(){
return name;
}
@Override
public int compareTo(Object o) {
if(o instanceof Goods){
Goods good = (Goods) o;
if(this.price > good.price){
return 1;
}else if(this.price < good.price){
return -1;
}else{
return 0;
}
}
throw new RuntimeException("输入数据异常!");
}
@Override
public String toString(){
return "商品:" + name + " 价格:" + price;
}
}
5. BigInteger与BigDecimal
5.1 BigInteger
java.math包的BigInteger可以表示不可变的任意精度的整数。BigInteger 提供所有 Java 的基本整数操作符的对应物,并提供 java.lang.Math 的所有相关方法。另外,BigInteger 还提供以下运算:模算术、GCD 计算、质数测试、素数生成、位操作以及一些其他操作。 构造器
- BigInteger(String val):根据字符串构建BigInteger对象
常用方法: 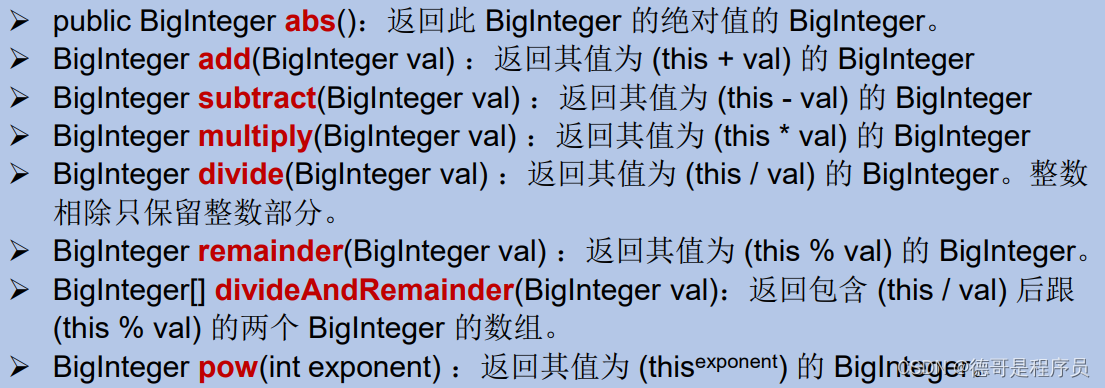
5.2 BigDecimal
一般的Float类和Double类可以用来做科学计算或工程计算,但在商业计算中,要求数字精度比较高,故用到java.math.BigDecimal类。
public BigDecimal(double val) public BigDecimal(String val)
public BigDecimal add(BigDecimal augend) public BigDecimal subtract(BigDecimal subtrahend) public BigDecimal multiply(BigDecimal multiplicand) public BigDecimal divide(BigDecimal divisor, int scale, int roundingMode)
|