配置文件
前面我们使用了springboot的核心配置文件application.properties ,springboot除了可以在全局编写一个application.properties 配置文件外,他还兼容另一种配置文件格式yaml。yaml非常适合用来做以数据为中心的配置文件,相比于xml,他语法更简洁,占用空间更小,更省资源
基本语法
key: value; kv之间有空格- 大小写敏感
- 使用缩进表示层级关系
- 缩进不允许使用tab,只允许空格
- 缩进的空格数不重要,只要相同层级的元素左对齐即可
# 表示注释- 字符串无需加引号,如果要加,
'' 与"" 表示字符串内容,会被转义,/ 不转义。不用"" 或'' 引起来,默认使用"" 引起来。
数据类型
一、字面量:单个的、不可再分的值。date 、boolean 、string 、number 、null
k: v
二、对象:键值对的集合。map 、hash 、set 、object 行内写法:k: {k1:v1,k2:v2,k3:v3} 或:
k:
k1: v1
k2: v2
k3: v3
三、数组:一组按次序排列的值。array 、list 、queue 行内写法:k: [v1,v2,v3] 或:
k:
- v1
- v2
- v3
四、实例 Person.java 中的代码:
@ConfigurationProperties(prefix = "person")
@Component
@ToString
@Data
public class Person {
private String userName;
private Boolean boss;
private Date birth;
private Integer age;
private Pet pet;
private String[] interests;
private List<String> animal;
private Map<String, Object> score;
private Set<Double> salarys;
private Map<String, List<Pet>> allPets;
}
Pet.java 中的代码:
@ToString
@Data
public class Pet {
private String name;
private Double weight;
}
yaml文件的后缀可以是.yml 也可以是.yaml ,yaml文件和application.properties 不冲突,二者可以同时存在同时生效,但application.properties 优先级更高,如果两个文件中定义了同一个属性值,会以application.properties 中的为准,application.yml 代码如下:
person:
userName: zhangsan
boss: false
birth: 2019/12/12 20:12:33
age: 18
pet:
name: tomcat
weight: 23.4
interests: [篮球,游泳]
animal:
- jerry
- mario
score:
english:
first: 30
second: 40
third: 50
math: [131,140,148]
chinese: {first: 128,second: 136}
salarys: [3999,4999.98,5999.99]
allPets:
sick:
- {name: tom}
- {name: jerry,weight: 47}
health: [{name: mario,weight: 47}]
测试一下,HelloController.java 代码如下:
@RestController
public class HelloController {
@Autowired
Person person;
@RequestMapping("/person")
public Person person(){
String userName = person.getUserName();
System.out.println(userName);
return person;
}
}
运行代码后,在浏览器中输入http://localhost:8080/person ,打印如下结果:  写字符串时无需用"" 或'' 引起来,引不引都一样,但是如果有转义字符,双引号不会转义,单引号会转义:
person:
userName: "zhangsan \n 李四"
输出:zhangsan 李四
person:
userName: 'zhangsan \n 李四'
输出:userName: "zhangsan \n 李四" 所以单引号会将\n 作为字符串输出,双引号会将\n ,作为换行输出。
配置提示
自定义的类和配置文件绑定一般没有提示。,要想有提示,在pom.xml中配置:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
打包的时候不要把配置处理器打包进去,在pom.xml 中配置:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
Web开发
soringboot为springMVC提供了很多自动化配置
简单功能分析
静态资源访问
静态资源目录
springboot为我们提供了静态资源目录:类路径下的/static(或/public或/resources或/META-INF/resources),只要我们把JS、图片、视频、CSS等等放在以上文件夹中,在访问当前项目根路径/+静态资源名 时,就能直接访问静态资源了,这是因为他底层使用Spring MVC中的ResourceHttpRequestHandler ,因此您可以通过添加自己的 webmvcconfigureer 和重写resourcehandlers 方法来修改该行为 原理:这是因为静态资源映射的是/** 。请求进来,先去找Controller看能不能处理。不能处理的所有请求又都交给静态资源处理器。静态资源也找不到则响应404页面 假如我在类路径下的/META-INF/resources文件夹中有一个src/main/resources/META-INF/resources/bug.jpg 图片,又在HelloController.java 中配置了拦截/bug.jpg 的请求,HelloController.java 中代码:
@RestController
public class HelloController {
@RequestMapping("/bug.jpg")
public String hello(){
return "aaaa";
}
}
启动项目后,在浏览器输入http://localhost:8080/bug.jpg 页面会展示"aaaa" 静态资源的目录默认是在/static(或/public或/resources或/META-INF/resources),如果改变默认的静态资源路径,在application.yaml中配置:
spring:
resources:
static-locations: [classpath:/haha/]
以后,启动项目后,在浏览器输入http://localhost:8080/res/zhifubao.png 页面会展示src/main/resources/haha/zhifubao.png 这张图片。
静态资源访问前缀
静态资源默认是当前项目根路径/+静态资源名 ,如果你想给静态资源加前缀,这样我们可以让拦截器放行以指定前缀为路径的所有请求,在application.yaml中配置:
spring:
mvc:
static-path-pattern: /res/**
以后会去当前项目 + static-path-pattern + 静态资源名 = 静态资源文件夹下找 静态资源,也就是说启动项目后,在浏览器输入http://localhost:8080/res/timg.jpg 页面会展示相应的图片。 至此,application.yaml中完整代码:
spring:
mvc:
static-path-pattern: /res/**
resources:
static-locations: [classpath:/haha/]
webjar
webjar:集合bootstrap、jQuery的jar包,然后搜索你想要的,找到之后将其导入,下图我随便框了一个types/jquery 的依赖 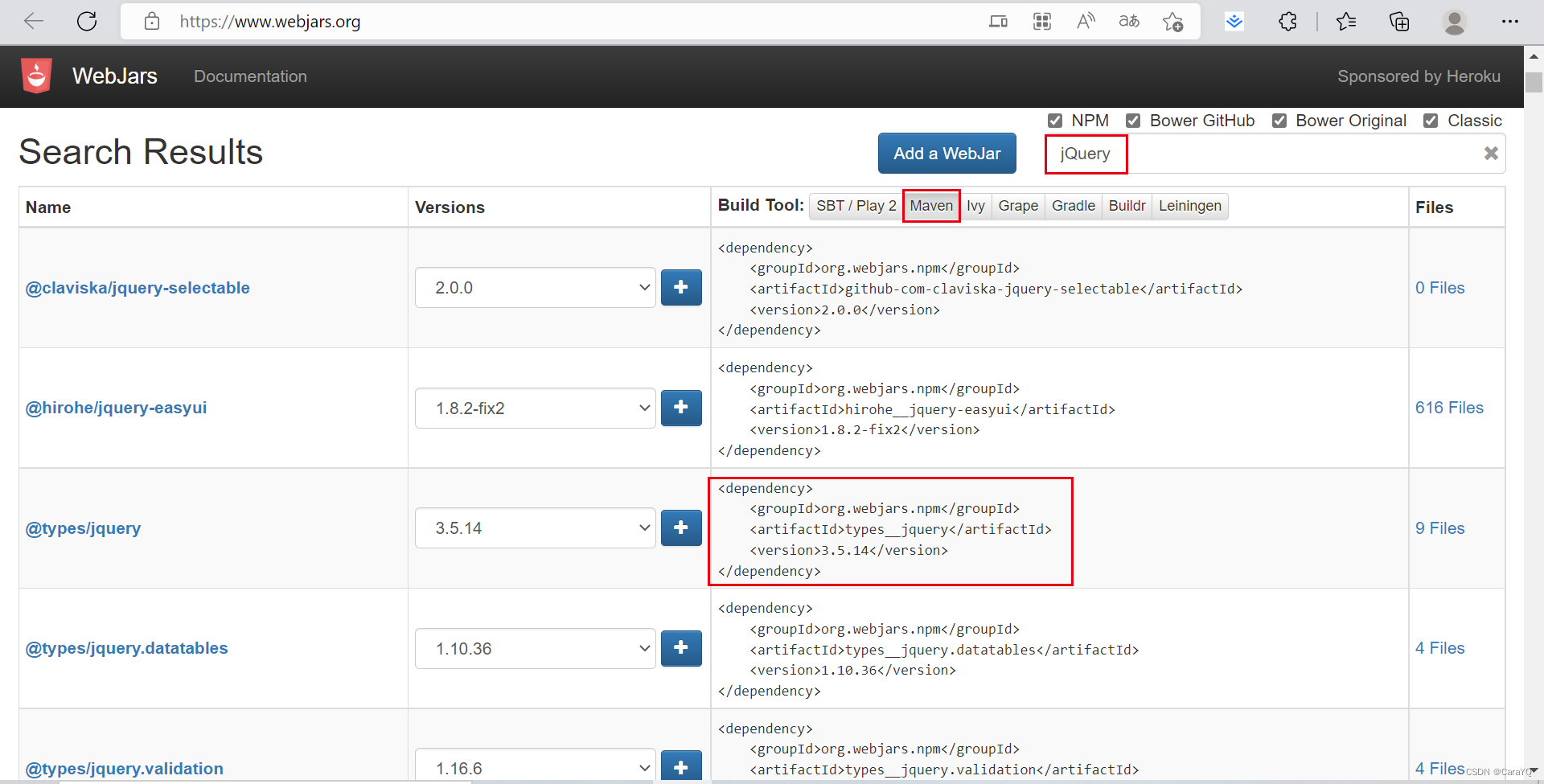 在pom.xml 中导入真正的jQuery依赖(我上图框的那个是假的):
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.5.1</version>
</dependency>
项目中就会出现jQuery的资源: 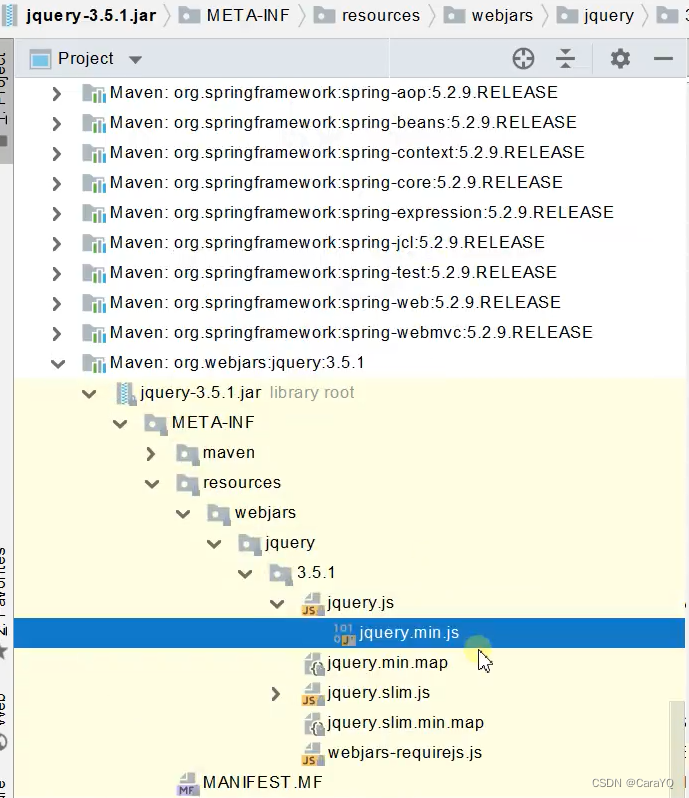 jQuery会被自动映射为/webjars/** ,项目启动后,访问地址:http://localhost:8080/webjars/jquery/3.5.1/jquery.js ,后面地址要按照依赖里面的包路径,页面展示以下内容: 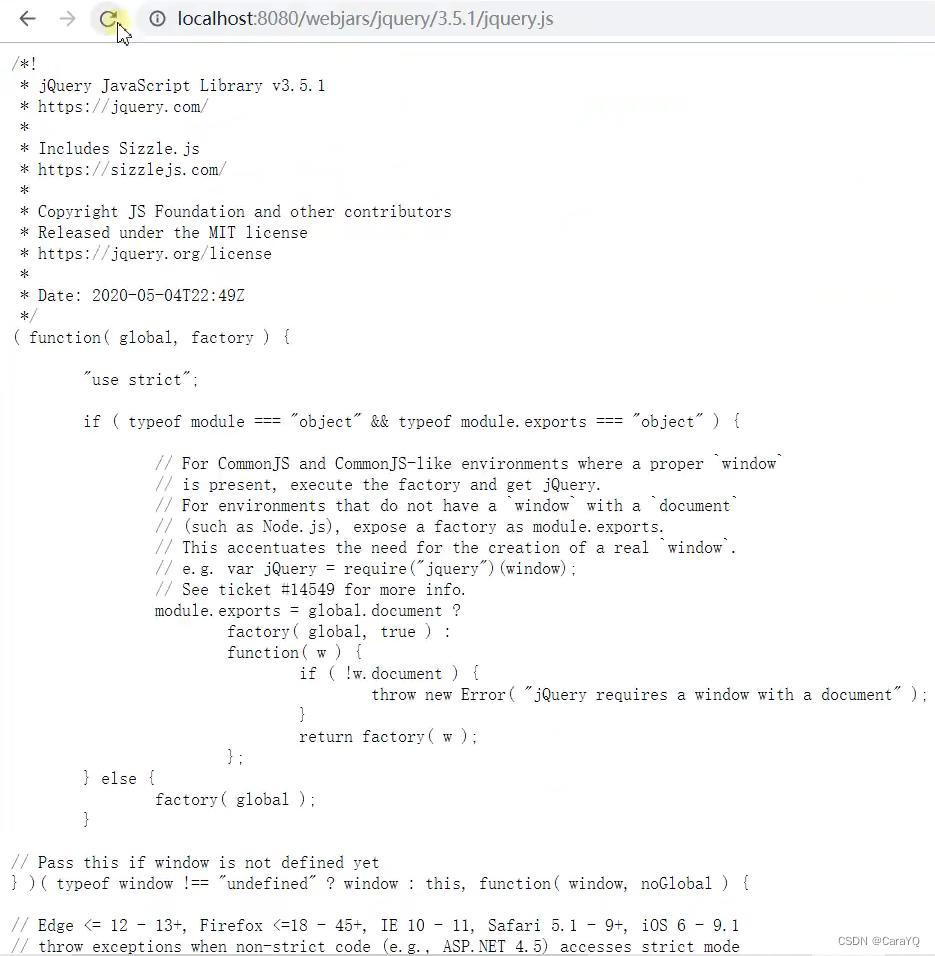
欢迎页支持
springboot支持两种方式的欢迎页:
- 静态方式:如果我们把静态资源文件
index.html 放在静态资源路径下,它就会被当成欢迎页,也就是访问我们项目的根路径默认展示的页面。新建文件src/main/resources/static/index.html ,代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>atguigu,欢迎您</h1>
</body>
</html>
项目启动后,在浏览器输入http://localhost:8080 ,展示以下内容: 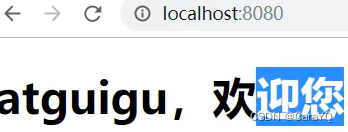 注意:可以配置静态资源路径,application.yaml中代码:
spring:
resources:
static-locations: [classpath:/haha/]
将src/main/resources/static/index.html 放在src/main/resources/haha/index.html 的位置,项目启动后,在浏览器输入http://localhost:8080 ,会去查找src/main/resources/haha/index.html ,页面展示以上内容。但是不可以配置静态资源的访问前缀,application.yaml中不能写代码:
spring:
mvc:
static-path-pattern: /res/**
否则导致index.html不能被默认访问,也就是说,如果写了上面的代码的话,项目启动后,在浏览器输入http://localhost:8080 ,页面会报404错误,并且会导致下面讲的Favicon功能失效,即配置了静态资源的访问前缀,在访问http://localhost:8080 时不会显示小图标 2. 模板方式:如果静态资源路径下没有index.html ,他会去找index模板,也就是去找你写的能处理index请求的controller,把这个能处理index请求的controller作为欢迎页
自定义Favicon
favicon.ico 放在静态资源目录下即可,注意一定要叫favicon.ico 这个名字,会自动把favicon.ico 当作页签小图标
源码分析
静态资源配置原理
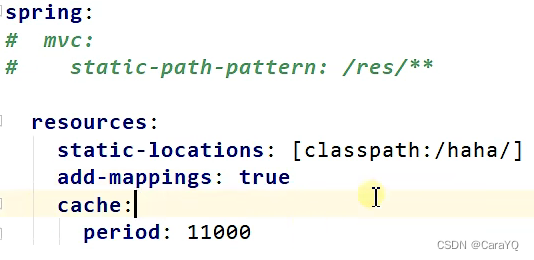
|