前言:
小伙伴们,大家好,我是狂奔の蜗牛rz,当然你们可以叫我蜗牛君,我是一个学习Java快一年时间的小菜鸟,同时还有一个伟大的梦想,那就是有朝一日,成为一个优秀的Java架构师。
首先给各位粉丝朋友们道个歉,在2022年上半年中,我因为参加实习、做毕设和写论文,以及毕业答辩等诸多原因,不得不停更之前的博客系列,不过现在我忙完后就又回来了,后续将会给大家分享更多的编程干货。
最近这段时间我会将在毕设项目的编写过程中所遇到的问题以及解决问题的方法进行总结,整理成这个 SpringBoot+LayUI前后端分离项目实战系列 。
特别提醒:如果对 SpringBoot+LayUI前后端分离项目实战系列感兴趣的,可以阅读本系列往期博客:
第一篇:SpringBoot+LayUI模板引擎+MybatisPlus 前后端分离 实现系统公告通知
第二篇:SpringBoot+LayUI+MybatisPlus+Echarts图表 前后端分离 实现数据统计功能
今天分享的问题是:如何使用SpringBoot框架、LayUI框架和MybatisPlus框架来实现排名统计功能,具体解决方案如下,请各位小伙伴们耐心观看:
1.SpringBoot后端主要实现代码
1.1 视图对象ResultSortVo的实现代码
package com.rz.sport_manager.entity.vo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.io.Serializable;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class ResultSortVo implements Serializable {
private String departName;
private Integer scoreSum;
}
1.2 ResultInfoMapper接口实现代码和ResultInfoMapper.xml映射文件
1.2.1 ResultInfoMapper接口实现代码
package com.rz.sport_manager.mapper;
import com.rz.sport_manager.entity.pojo.ResultInfo;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.rz.sport_manager.entity.pojo.UserInfo;
import com.rz.sport_manager.entity.vo.ResultSortVo;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Repository
@Mapper
public interface ResultInfoMapper extends BaseMapper<ResultInfo> {
public List<ResultSortVo> getDepartScoreSumSortList();
}
1.2.2 ResultInfoMapper.xml映射文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.rz.sport_manager.mapper.ResultInfoMapper">
<select id="getDepartScoreSumSortList" resultMap="resultSortMap">
select ti.team_name as depart_name ,sum(ri.score) as score_sum from sport_manager01.result_info ri
left join sport_manager01.team_info ti on ti.team_id = ri.team_id
left join sport_manager01.sport_info si on si.sport_id = ri.sport_id
where ri.group_id in(1,2,3) and ri.status = 1 and ri.publish = 1 and si.status = 1
group by depart_name order by score_sum desc;
</select>
<resultMap id="resultSortMap" type="com.rz.sport_manager.entity.vo.ResultSortVo">
<result property="departName" column="depart_name"/>
<result property="scoreSum" column="score_sum"/>
</resultMap>
</mapper>
1.3 ResultInfoService服务层接口和其实现类的主要实现代码
1.3.1 ResultInfoService服务层接口的实现代码
package com.rz.sport_manager.service;
import com.rz.sport_manager.entity.pojo.ResultInfo;
import com.baomidou.mybatisplus.extension.service.IService;
import com.rz.sport_manager.entity.vo.*;
import com.rz.sport_manager.utils.JsonResult;
import java.util.List;
public interface ResultInfoService extends IService<ResultInfo> {
public JsonResult<List<ResultSortVo>> getTeamScoreSumSortList();
}
1.3.2 ResultInfoServiceImpl服务层接口实现类的实现代码
package com.rz.sport_manager.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.rz.sport_manager.entity.vo.*;
import com.rz.sport_manager.mapper.*;
import com.rz.sport_manager.service.ResultInfoService;
import com.rz.sport_manager.utils.JsonResult;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.*;
@Service
public class ResultInfoServiceImpl extends ServiceImpl<ResultInfoMapper, ResultInfo> implements ResultInfoService {
@Autowired
private ResultInfoMapper resultInfoMapper;
@Override
public JsonResult<List<ResultSortVo>> getTeamScoreSumSortList() {
List<ResultSortVo> resultSortVoList = resultInfoMapper.getDepartScoreSumSortList();
return JsonResult.batchDataSuccess(resultSortVoList.size(),resultSortVoList);
}
}
1.4 ResultInfoController控制层的实现代码
package com.rz.sport_manager.controller;
import com.rz.sport_manager.entity.vo.*;
import com.rz.sport_manager.service.ResultInfoService;
import com.rz.sport_manager.utils.JsonResult;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@Controller
@RequestMapping("/sport_manager/result_info")
public class ResultInfoController {
@GetMapping("/getTeamScoreSumSortList")
@ResponseBody
public JsonResult<List<ResultSortVo>> getTeamScoreSumSortList(){
return resultInfoService.getTeamScoreSumSortList();
}
}
2.LayUI前端主要实现代码
2.1 排名统计的前端主要实现代码
<style>
.welcome .layui-card {border:1px solid #f2f2f2;border-radius:5px;}
.welcome .icon {margin-right:10px;color:#1aa094;}
.welcome .layuimini-qiuck-module a i {display:inline-block;width:100%;height:60px;line-height:60px;text-align:center;border-radius:2px;font-size:30px;background-color:#F8F8F8;color:#333;transition:all .3s;-webkit-transition:all .3s;}
.welcome .layuimini-qiuck-module a cite {position:relative;top:2px;display:block;color:#666;text-overflow:ellipsis;overflow:hidden;white-space:nowrap;font-size:14px;}
.welcome .main_btn > p {height:40px;}
</style>
<div class="layuimini-container layuimini-page-anim">
<div class="layuimini-main welcome">
<div class="layui-row layui-col-space20">
<div class="layui-col-md12">
<div class="layui-row layui-col-space15">
<div class="layui-col-md3">
<div class="layui-card" style="width: 100%;min-height:300px">
<div class="layui-card-header"><i class="fa fa-fa icon"></i>各分院竞赛总分排名</div>
<div class="layui-card-body" id="table1">
<script type="text/html" id="tableTemplate1">
<!-- table表格 -->
<table class="table1" width="100%" border="0" cellspacing="0" cellpadding="0">
<!-- 表格内容 -->
<tbody>
<!-- 表格头部行 -->
<tr>
<!-- 表格头部列 -->
<th style="border-bottom: 1px solid rgb(211,211,211); font-size: 16px; color:rgb(0,0,0); font-weight: normal; padding:0 0 10px 0;">排名</th>
<th style="border-bottom: 1px solid rgb(211,211,211); font-size: 16px; color:rgb(0,0,0); font-weight: normal; padding:0 0 10px 0;">分院名称</th>
<th style="border-bottom: 1px solid rgb(211,211,211); font-size: 16px; color:rgb(0,0,0); font-weight: normal; padding:0 0 10px 0;">竞赛总分</th>
</tr>
<!-- 使用layui的each循环, 遍历数据集合信息(注意: 这里一定要使用d.data; 参数index表示集合下标, item表示集合元素) -->
{{# layui.each(d.data,function(index,item){ }}
<!-- 表格内容行 -->
<tr>
<!-- 表格内容列 -->
<!-- 判断当前元素下标是否为0(即分数排行的第一名), 若为0, 则设置为以下样式 -->
{{# if(index == 0) { }}
<td style="font-size: 16px; color:rgb(88,87,86); padding: 15px 0 0 5px;">
<!-- 排名数字的背景颜色设置为红色 -->
<span style="width: 24px; height: 24px; border-radius: 3px; display: block; background: #878787; color: #fff; line-height: 24px; text-align: center; background: #ed405d">1</span>
</td>
<!-- 判断当前元素下标是否为1(即分数排行的第二名), 若为1, 则设置为以下样式 -->
{{# } else if(index == 1) { }}
<td style="font-size: 16px; color:rgb(88,87,86); padding: 15px 0 0 5px;">
<!-- 排名数字的背景颜色设置为橙色 -->
<span style="width: 24px; height: 24px; border-radius: 3px; display: block; background: #878787; color: #fff; line-height: 24px; text-align: center; background: #f78c44">2</span>
</td>
<!-- 判断当前元素下标是否为2(即分数排行的第三名), 若为2, 则设置为以下样式 -->
{{# } else if(index == 2) { }}
<td style="font-size: 16px; color:rgb(88,87,86); padding: 15px 0 0 5px;">
<!-- 排名数字的背景颜色设置为蓝色 -->
<span style="width: 24px; height: 24px; border-radius: 3px; display: block; background: #878787; color: #fff; line-height: 24px; text-align: center; background: #49bcf7">3</span>
</td>
{{# } else { }}
<td style="font-size: 16px; color:rgb(88,87,86); padding: 15px 0 0 5px;">
<!-- 其他名次的排名数字的背景颜色设置为灰色 -->
<span style="width: 24px; height: 24px; border-radius: 3px; display: block; background: #878787; color: #fff; line-height: 24px; text-align: center;">{{ index+1 }}</span>
</td>
{{# } }}
<!-- 遍历每个元素值均使用 {{ item.字段名 }} 格式(注意: {{后无需加#号) -->
<td style="font-size: 16px; color:rgb(88,87,86); padding: 15px 0 0 20px;">
<!-- 获取分院名称 -->
<span style="font-size: 16px; color:rgb(88,87,86);padding: 15px 0 0 20px;">{{ item.departName }}</span>
</td>
<td style="font-size: 16px; color:rgb(88,87,86); padding: 15px 0 0 15px;">
<!-- 获取竞赛分数 -->
<span style="font-size: 16px; color:rgb(88,87,86);padding: 15px 0 0 15px;">{{ item.scoreSum }}</span>
</td>
</tr>
<!-- 循环结束记得加上这个 -->
{{# }); }}
</tbody>
</table>
</script>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
layui.use(['layer','jquery','laytpl'], function () {
var $ = layui.jquery,
layer = layui.layer,
laytpl = layui.laytpl;
let resultInfoList1;
$.ajax({
type: 'GET',
url: 'http://localhost:8080/sport_manager/result_info/getTeamScoreSumSortList',
dataType: 'json',
contentType: 'application/json',
async: false,
success: function (data) {
if(data.code === 0) {
resultInfoList1 = data;
} else {
layer.msg('获取竞赛成绩排名数据失败!', {icon: 2});
}
}
});
let tableTpl = tableTemplate1.innerHTML,
table = document.getElementById('table1');
laytpl(tableTpl).render(resultInfoList1, function(html) {
table.innerHTML = html;
});
});
</script>
2.2 排名统计的前端页面显示效果
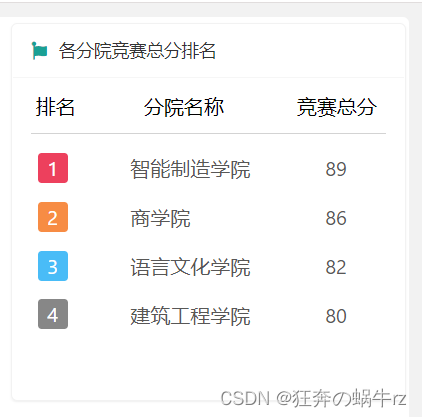
以上就是如何使用SpringBoot框架、LayUI框架和MybatisPlus框架来实现排名统计功能的所有分享内容了。欢迎各位小伙伴讨论和学习,觉得还不错的不妨给蜗牛君点个关注,顺便来个一键三连。我们下期见,拜拜啦!
|