目录
一、修改pom.xml文件
二、添加启动类
三、SpringBoot常用相关依赖
四、配置文件常用配置
五、总结
一、修改pom.xml文件
1.指定父级依赖
<!-- 指定父级依赖,所有springboot项目都必须继承 -->
? ?<parent>
? ? ? ?<artifactId>spring-boot-starter-parent</artifactId>
? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ?<!-- springBoot版本 -->
? ? ? ?<version>2.5.6</version>
? ?</parent>
2.添加spring-web启动器依赖
<!-- spring-Web启动器 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ? ? ?<artifactId>spring-boot-starter-web</artifactId>
? ? ? ? ? ?<version>2.5.4</version>
? ? ? ?</dependency>
3.最终pom.xml文件配置如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
? ? ? ? xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
? ? ? ? xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
? ?<modelVersion>4.0.0</modelVersion>
?
? ?<groupId>com.klix</groupId>
? ?<artifactId>Idea-Test</artifactId>
? ?<version>1.0</version>
?
? ?<!-- 指定父级依赖,所有springboot项目都必须继承 -->
? ?<parent>
? ? ? ?<artifactId>spring-boot-starter-parent</artifactId>
? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ?<!-- springBoot版本 -->
? ? ? ?<version>2.5.6</version>
? ?</parent>
?
? ?<properties>
? ? ? ?<maven.compiler.source>8</maven.compiler.source>
? ? ? ?<maven.compiler.target>8</maven.compiler.target>
? ?</properties>
? ?<dependencies>
? ? ? ?<!-- spring-Web启动器 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ? ? ?<artifactId>spring-boot-starter-web</artifactId>
? ? ? ? ? ?<version>2.5.4</version>
? ? ? ?</dependency>
? ?</dependencies>
?
</project>
二、添加启动类
package org.demo;
?
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
?
@SpringBootApplication
//说明这是springboot的启动类,同时还说明这时一个配置类
//主类所在的包就是springboot应用的根包
public class MyApp {
? ?public static void main(String[] args) {
?
? ? ? ?SpringApplication.run(MyApp.class,args);//启动类的主类
? ? ? ?
? }
}
至此,SpringBoot项目已经可以正常启动了
三、SpringBoot常用相关依赖
1.连接数据库的依赖
<!-- MySQL连接依赖 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>mysql</groupId>
? ? ? ? ? ?<artifactId>mysql-connector-java</artifactId>
? ? ? ?</dependency>
然后要在配置文件里配置数据库连接
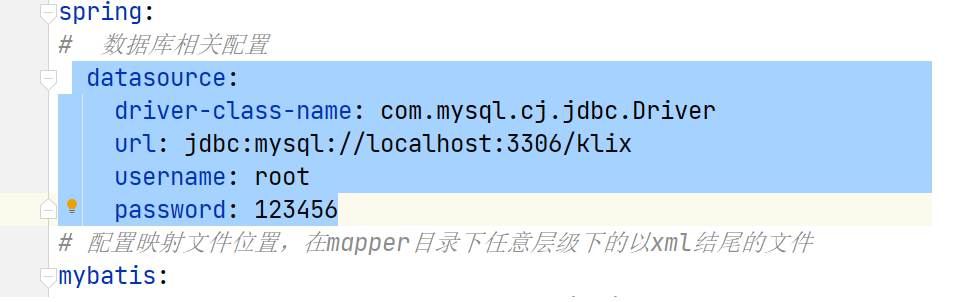
2.Mybatis依赖
<dependency>
? ? ? ? ? ?<groupId>org.mybatis.spring.boot</groupId>
? ? ? ? ? ?<artifactId>mybatis-spring-boot-starter</artifactId>
? ? ? ? ? ?<version>2.2.2</version>
? ? ? ?</dependency>
写完要在启动类里配置mapper扫描(让dao接口编译时生成响应的实现类)
以及配置文件里配置mapper的位置
2.1启动类配置mapper扫描
???????
如果有多个,mapper可以这样写
@MapperScan({"com.jshiming.fun.*.dao","com.jshiming.common.config.dao"})
2.2 配置文件配置映射文件路径
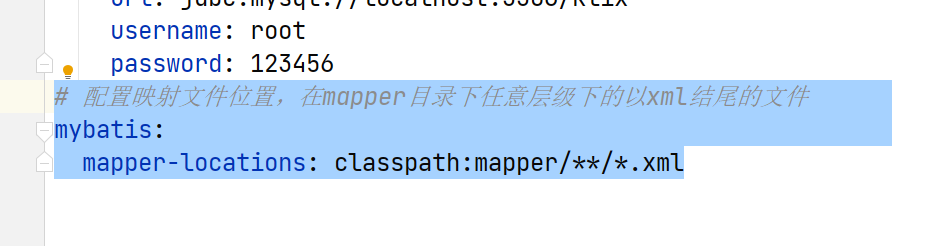
3.SpringBoot项目进行测试的依赖
<!-- SpringBoot 的测试支持 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ? ? ?<artifactId>spring-boot-starter-test</artifactId>
? ? ? ?</dependency>
两个注解
@SpringBootTest
@Test
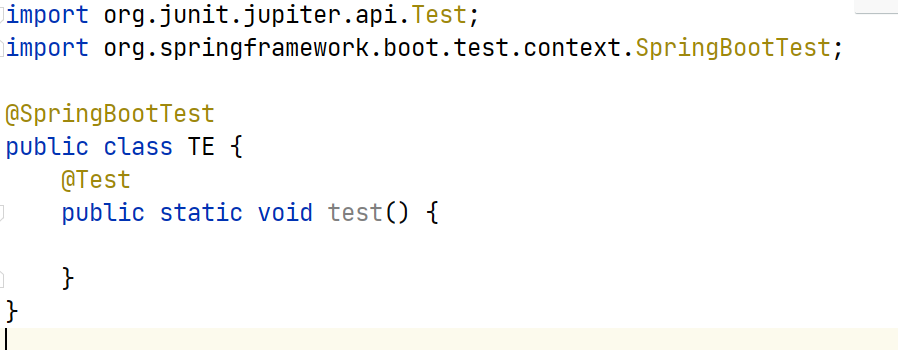
四、配置文件常用配置
配日志(看报错信息)
日志真的是程序员必备好吗,不接受反驳
# 配置Java自带日志的级别
logging:
level:
? root: info
? web: trace
? klix: debug
五、总结
5.1 pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
? ? ? ? xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
? ? ? ? xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
? ?<modelVersion>4.0.0</modelVersion>
?
? ?<groupId>com.klix</groupId>
? ?<artifactId>Idea-Test</artifactId>
? ?<version>1.0</version>
?
? ?<!-- 指定父级依赖,所有springboot项目都必须继承 -->
? ?<parent>
? ? ? ?<artifactId>spring-boot-starter-parent</artifactId>
? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ?<!-- springBoot版本 -->
? ? ? ?<version>2.5.6</version>
? ?</parent>
?
? ?<properties>
? ? ? ?<maven.compiler.source>8</maven.compiler.source>
? ? ? ?<maven.compiler.target>8</maven.compiler.target>
? ?</properties>
? ?<dependencies>
? ? ? ?<!-- spring-Web启动器 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ? ? ?<artifactId>spring-boot-starter-web</artifactId>
? ? ? ? ? ?<version>2.5.4</version>
? ? ? ?</dependency>
? ? ? ?<!-- SpringBoot 的测试支持 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework.boot</groupId>
? ? ? ? ? ?<artifactId>spring-boot-starter-test</artifactId>
? ? ? ?</dependency>
? ? ? ?<!-- MySQL连接依赖 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>mysql</groupId>
? ? ? ? ? ?<artifactId>mysql-connector-java</artifactId>
? ? ? ?</dependency>
? ? ? ?<!-- Mybatis依赖 -->
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.mybatis.spring.boot</groupId>
? ? ? ? ? ?<artifactId>mybatis-spring-boot-starter</artifactId>
? ? ? ? ? ?<version>2.2.2</version>
? ? ? ?</dependency>
?
? ?</dependencies>
?
</project>
5.2 application.yml
# 设置项目端口号
server:
port: 8081
?
# 数据库相关配置
spring:
datasource:
? driver-class-name: com.mysql.cj.jdbc.Driver
? url: jdbc:mysql://localhost:3306/klix
? username: root
? password: 123456
?
# 配置映射文件位置,在mapper目录下任意层级下的以xml结尾的文件
mybatis:
mapper-locations: classpath:mapper/**/*.xml
?
# 配置Java自带日志的级别
logging:
level:
? root: info
? web: trace
? klix: debug
5.3 启动类
package klix;
?
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
?
?
@SpringBootApplication
@MapperScan("klix.fun.*.dao")
public class MyApp {
? ?public static void main(String[] args) {
? ? ? ?SpringApplication.run(MyApp.class,args);
? }
}
|