入门
创建一个springboot项目
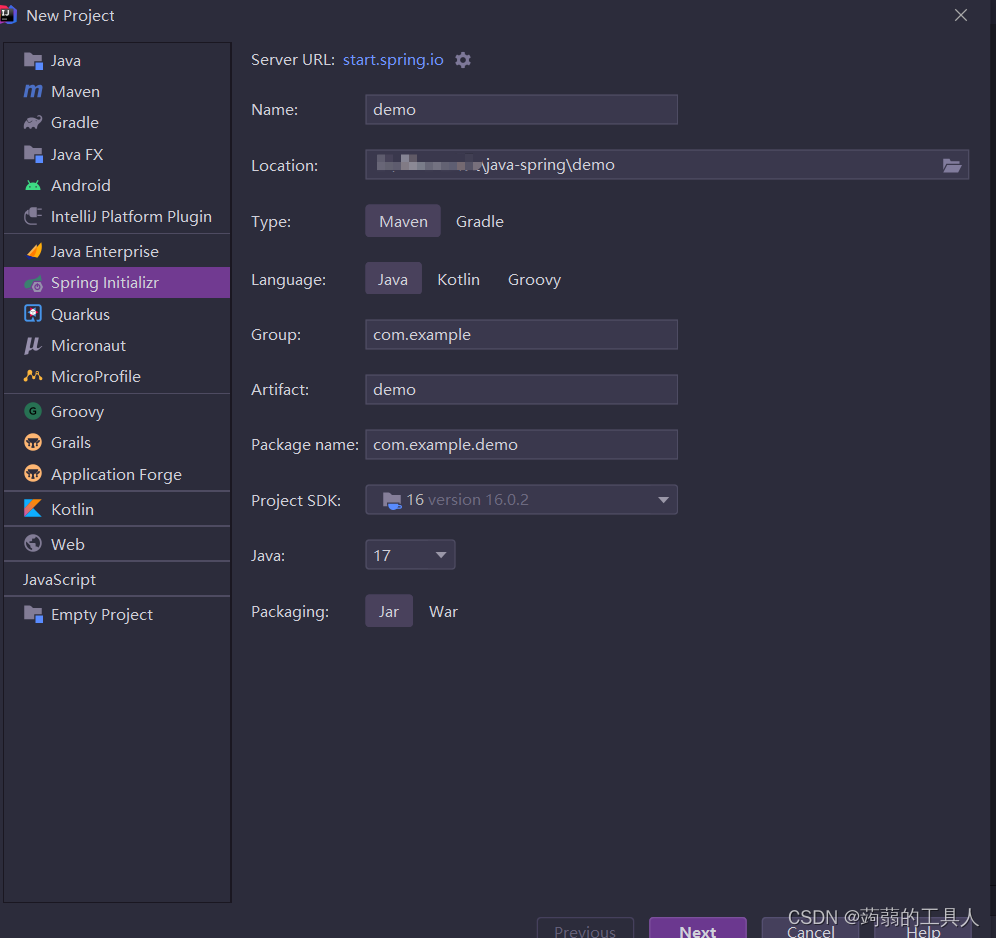
编写一个get接口
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class Hello {
@GetMapping("/hello")
public String Hello() {
return "get-hello";
}
}
添加依赖
在pom.xml文件中添加 例如添加lombok
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
基础
web开发
静态资源访问
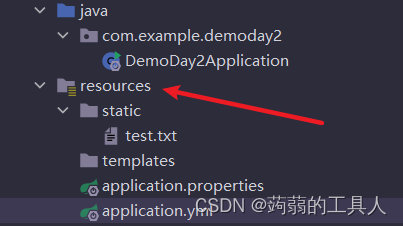
其中resources 下的 /static /public /resource /META-INF 文件下的资源为默认静态资源 在网页中可直接访问 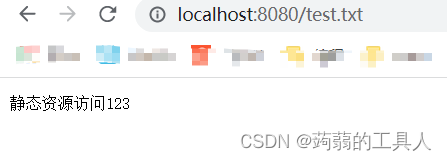
当url相同时候,先请求动态资源,才会请求静态资源
改变静态资源默认路径
spring:
mvc:
static-path-pattern: /res/**
web:
resources:
static-locations:
[classpath:/stat/]
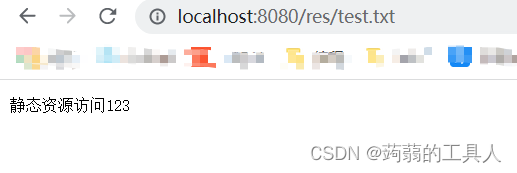 如出现中文乱码,修改一下配置 1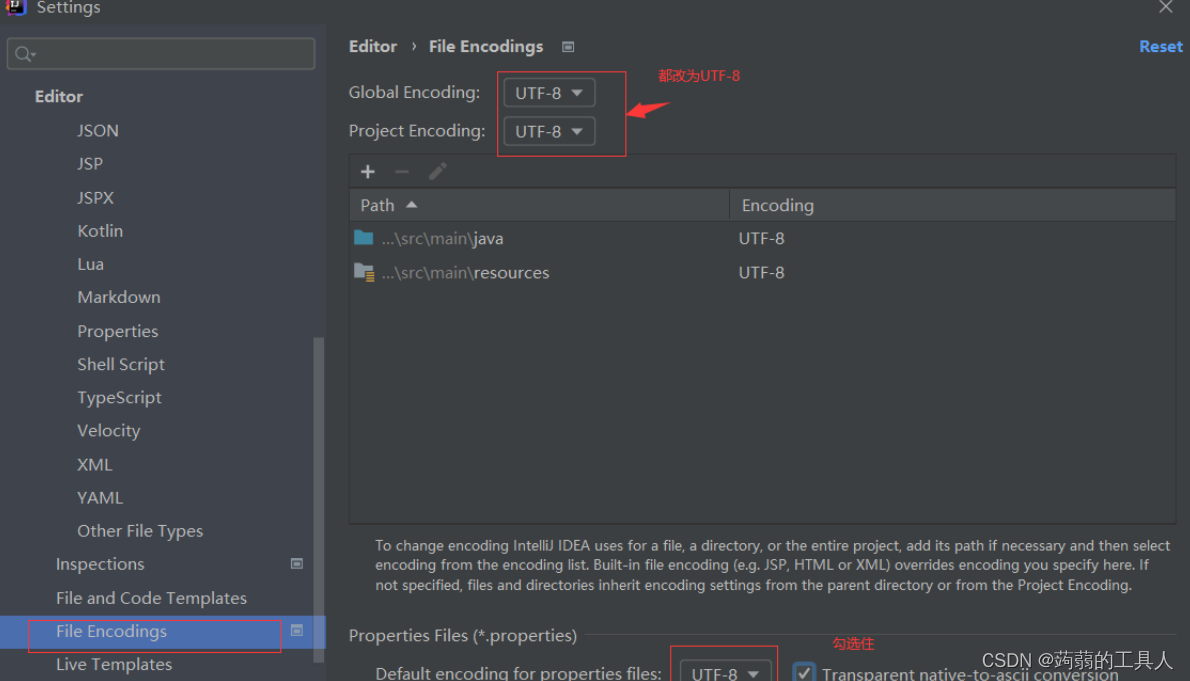 2 application.properties配置
server.servlet.encoding.charset=utf-8
server.servlet.encoding.force=true
server.servlet.encoding.enabled=true
欢迎页
静态资源路径下访问index.html 不能自定义访问前缀 可以自定义默认文件
mvc:
static-path-pattern: /res
否则无法正确访问 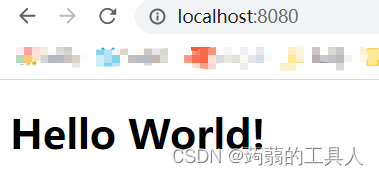
请求方式
Controller.Hello 四种请求方式
package com.example.demoday3.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class Hello {
@RequestMapping(value = "/user",method = RequestMethod.GET)
public String getUser(){
return "getUser";
}
@RequestMapping(value = "/user",method = RequestMethod.POST)
public String postUser(){
return "postUser";
}
@RequestMapping(value = "/user",method = RequestMethod.DELETE)
public String deleteUser(){
return "deleteUser";
}
@RequestMapping(value = "/user",method = RequestMethod.PUT)
public String putUser(){
return "putUser";
}
}
static的index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="/user" method="post">
<input type="text" name="userName"/>
<input type="submit">
</form>
</body>
</html>
因为得form表单method只有get和post方式 所以要在配置添加
spring:
mvc:
hiddenmethod:
filter:
enabled: true
<form action="/user" method="post">
<input name="_method" type="hidden" value="delete"/>
<input type="text" name="userName"/>
<input type="submit" value=delete>
</form>
<form action="/user" method="post">
<input name="_method" type="hidden" value="PUT"/>
<input type="text" name="userName"/>
<input type="submit" value="put">
</form>
如果使用postman,可以直接使用delete、put方式,不用添加以上 filter配置。
另外,可以自定义 name="_method"的名称。 方法如下 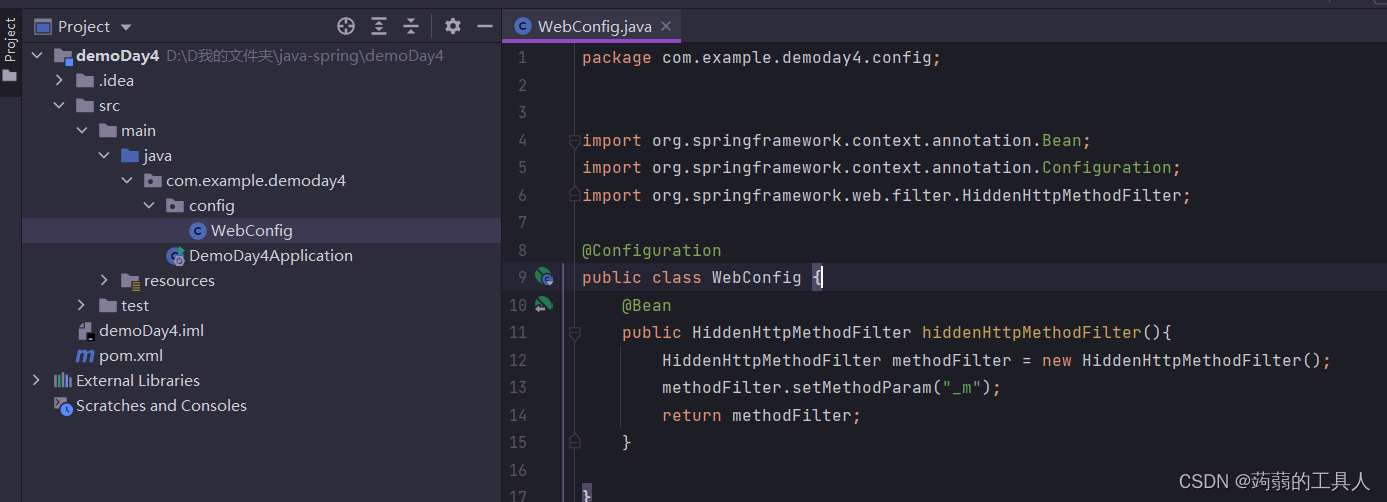
|