环境
- 操作系统:Ubuntu 22.04
- IntelliJ IDEA 2022.1.3
- JDK 17.0.3
准备
新建Maven项目 test0626_1 。
打开 pom.xml 文件,添加如下依赖:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.10</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.29</version>
</dependency>
这些依赖都是在 https://mvnrepository.com 查找并复制的,使用的都是最新版本。
junit :JUnit单元测试mybatis :MyBatismysql-connector-java :MySQL驱动
创建POJO MyObject.java 文件如下:
package com.example.demo.pojo;
public class MyObject {
private int c1;
private String c2;
public int getC1() {
return c1;
}
public void setC1(int c1) {
this.c1 = c1;
}
public String getC2() {
return c2;
}
public void setC2(String c2) {
this.c2 = c2;
}
@Override
public String toString() {
return "MyObject{" +
"c1=" + c1 +
", c2='" + c2 + '\'' +
'}';
}
}
创建 MyMapper.java 文件如下:
package com.example.demo.dao;
import com.example.demo.pojo.MyObject;
import java.util.List;
public interface MyMapper {
List<MyObject> read();
}
在相同目录下创建 MyMapper.xml 文件如下:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.dao.MyMapper">
<select id = "read" resultType="com.example.demo.pojo.MyObject">
select c1, c2 from t1
</select>
</mapper>
在 resources 目录下创建 mybatis-config.xml 文件如下:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="mysql">
<environment id="mysql">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/repo"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper class="com.example.demo.dao.MyMapper"/>
</mappers>
</configuration>
创建测试 TestMybatis.java 文件如下(注意是在 src/test/java 下):
package com.example.demo.test;
import com.example.demo.dao.MyMapper;
import com.example.demo.pojo.MyObject;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class TestMybatis {
@Test
public void Test1() throws IOException {
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession sqlSession = sqlSessionFactory.openSession(true);
try {
MyMapper mapper = sqlSession.getMapper(MyMapper.class);
List<MyObject> list = mapper.read();
list.forEach(System.out::println);
} finally {
sqlSession.close();
}
}
}
运行测试,此时会报错如下:
org.apache.ibatis.binding.BindingException: Invalid bound statement (not found): com.example.demo.dao.MyMapper.read
查看 target/classes/com/example/demo/dao 目录,只有 MyMapper.class ,并没有 MyMapper.xml 文件(但是 mybatis-config.xml 文件是存在的)。
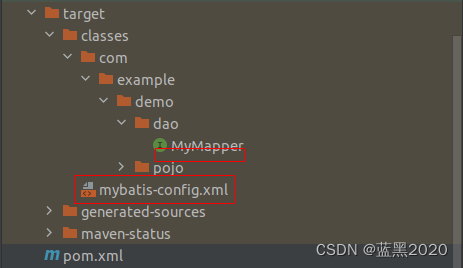
这是因为默认 Resource Folders 设置的是 src/main/resources 目录。该目录下的资源文件(非Java文件)会被复制到 target 目录下。
解决办法,在 pom.xml 文件中添加:
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
但是,该修改只设置了静态资源目录为 src/main/java ,这样的话 src/main/resources 就不是资源目录了。如果刷新Maven,做一下compile,则可见 MyMapper.xml 和 mybatis-config.xml 文件都存在了。但是,注意compile并不会清理target目录,只会添加新文件。如果先做clean(清理掉 target 目录)再做compile,就会发现 MyMapper.xml 存在,但是 mybatis-config.xml 不见了。
所以,需要把 src/main/resources 也设置为资源目录:
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
刷新Maven,做clean和compile,如下:
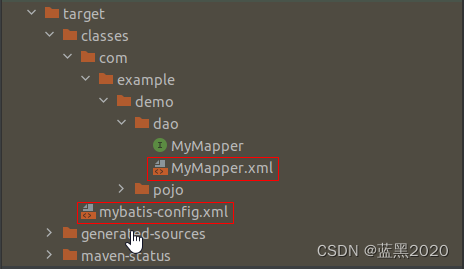
运行测试,如下:
MyObject{c1=1, c2='aaa'}
MyObject{c1=2, c2='bbb'}
总结:使用MyBatis访问数据库的方法如下:
- 通过
SqlSessionFactoryBuilder 创建 SqlSessionFactory - 通过
SqlSessionFactory 打开 sqlSession - 通过
sqlSession 获取Mapper - 调用Mapper的方法
Spring整合MyBatis
新建Maven项目 test0626_2 ,把刚才 test0626_1 的文件统统复制过来,我们将在其基础上修改。
要把MyBatis整合到Spring里,首先要在 pom.xml 里添加3个依赖:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.7</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.21</version>
</dependency>
- spring-webmvc
- mybatis-spring
- spring-jdbc
删除 mybatis-config.xml 文件,我们将在Spring里配置数据源和Mapper。
首先配置数据源:
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/repo"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</bean>
然后配置SqlSessionFactory:
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="mapperLocations" value="classpath:com/example/demo/dao/*.xml"/>
</bean>
注意: SqlSessionFactoryBean 是一个工厂bean。所谓工厂bean,当调用 getBean("xxx") 时,容器返回的不是它自身,而是其 getObject() 的返回值。
它的property里:
- dataSource
- mapperLocation
- configLocation
方法1(MapperFactoryBean)
配置 MyMapper :
<bean id="myMapper" class="org.mybatis.spring.mapper.MapperFactoryBean">
<property name="mapperInterface" value="com.example.demo.dao.MyMapper" />
<property name="sqlSessionFactory" ref="sqlSessionFactory" />
</bean>
同理,通过 MapperFactoryBean 生成 MyMapper ,同时把 sqlSessionFactory 注入。
现在就可以测试了。删除 TestMybatis.java ,创建 TestSpring.java (在 src/test/java 目录下):
package com.example.demo.test;
import com.example.demo.dao.MyMapper;
import com.example.demo.pojo.MyObject;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.io.IOException;
import java.util.List;
public class TestSpring {
@Test
public void Test1() throws IOException {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
List<MyObject> list = context.getBean("myMapper", MyMapper.class).read();
list.forEach(System.out::println);
}
}
运行测试,如下:
MyObject{c1=1, c2='aaa'}
MyObject{c1=2, c2='bbb'}
总结:原先是在 mybatis-config.xml 里配置数据源和Mapper,在代码中创建 SqlSessionFactory ,现在都是在Spring中配置了。
方法2(SqlSessionTemplate)
SqlSessionTemplate 是 SqlSession 的一个实现,可以使用它无缝代替代码中已经在使用的 SqlSession 。
在Spring中,可以直接配置 SqlSessionTemplate :
<bean id="sqlSession" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg ref="sqlSessionFactory"/>
</bean>
把 SqlSessionFactory 作为构造参数注入(没有set方法)。
现在,就可以使用 SqlSessionTemplate 来访问数据库了。
创建 MyMapperImpl.java 如下:
package com.example.demo.dao;
import com.example.demo.pojo.MyObject;
import org.mybatis.spring.SqlSessionTemplate;
import java.util.List;
public class MyMapperImpl implements MyMapper{
private SqlSessionTemplate sqlSession;
public void setSqlSession(SqlSessionTemplate sqlSession) {
this.sqlSession = sqlSession;
}
@Override
public List<MyObject> read() {
return sqlSession.getMapper(MyMapper.class).read();
}
}
在Spring中配置:
<bean id="myMapper2" class="com.example.demo.dao.MyMapperImpl">
<property name="sqlSession" ref="sqlSession"/>
</bean>
测试:
@Test
public void Test2() throws IOException {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
List<MyObject> list = context.getBean("myMapper2", MyMapper.class).read();
list.forEach(System.out::println);
}
运行测试,如下:
MyObject{c1=1, c2='aaa'}
MyObject{c1=2, c2='bbb'}
方法3(SqlSessionDaoSupport)
注:该方法是方法2的变种,通过继承 SqlSessionDaoSupport ,隐式使用 SqlSession (需注入 SqlSessionFactory )。
创建 MyMapperImpl2.java 文件如下:
package com.example.demo.dao;
import com.example.demo.pojo.MyObject;
import org.mybatis.spring.support.SqlSessionDaoSupport;
import java.util.List;
public class MyMapperImpl2 extends SqlSessionDaoSupport implements MyMapper {
@Override
public List<MyObject> read() {
return getSqlSession().getMapper(MyMapper.class).read();
}
}
在Spring中配置(Spring里不用配置 SqlSession ):
<bean id="myMapper3" class="com.example.demo.dao.MyMapperImpl2">
<property name="sqlSessionFactory" ref="sqlSessionFactory"/>
</bean>
测试:
@Test
public void Test3() throws IOException {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
List<MyObject> list = context.getBean("myMapper3", MyMapper.class).read();
list.forEach(System.out::println);
}
运行测试,如下:
MyObject{c1=1, c2='aaa'}
MyObject{c1=2, c2='bbb'}
总结
- 方法1比较简单,无需额外的代码。
- 但是方法1只适用于接口,如果Dao中还有额外的代码逻辑,就可以考虑使用类来实现接口,用方法2或者方法3。
- 如果需要显式拿到SqlSession,则可以使用方法2或者方法3。
- 方法3是方法2的变种,稍微简化了一些。
参考
- http://mybatis.org/spring
- http://mybatis.org/spring/zh/index.html (中文版)
|