📝 个人主页:程序员阿红🔥 👑 生活平淡,用心就会发光,岁月沉闷,跑起来,就会有风 📣 推荐文章: 📚📚📚 【毕业季·进击的技术er】忆毕业一年有感 📚📚📚 Java 学习路线一条龙版 📚📚📚 一语详解SpringBoot全局配置文件
实战技能补充:lombok
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.12</version>
<scope>provided</scope>
</dependency>
需求:实现用户的CRUD功能
1. 创建springboot工程
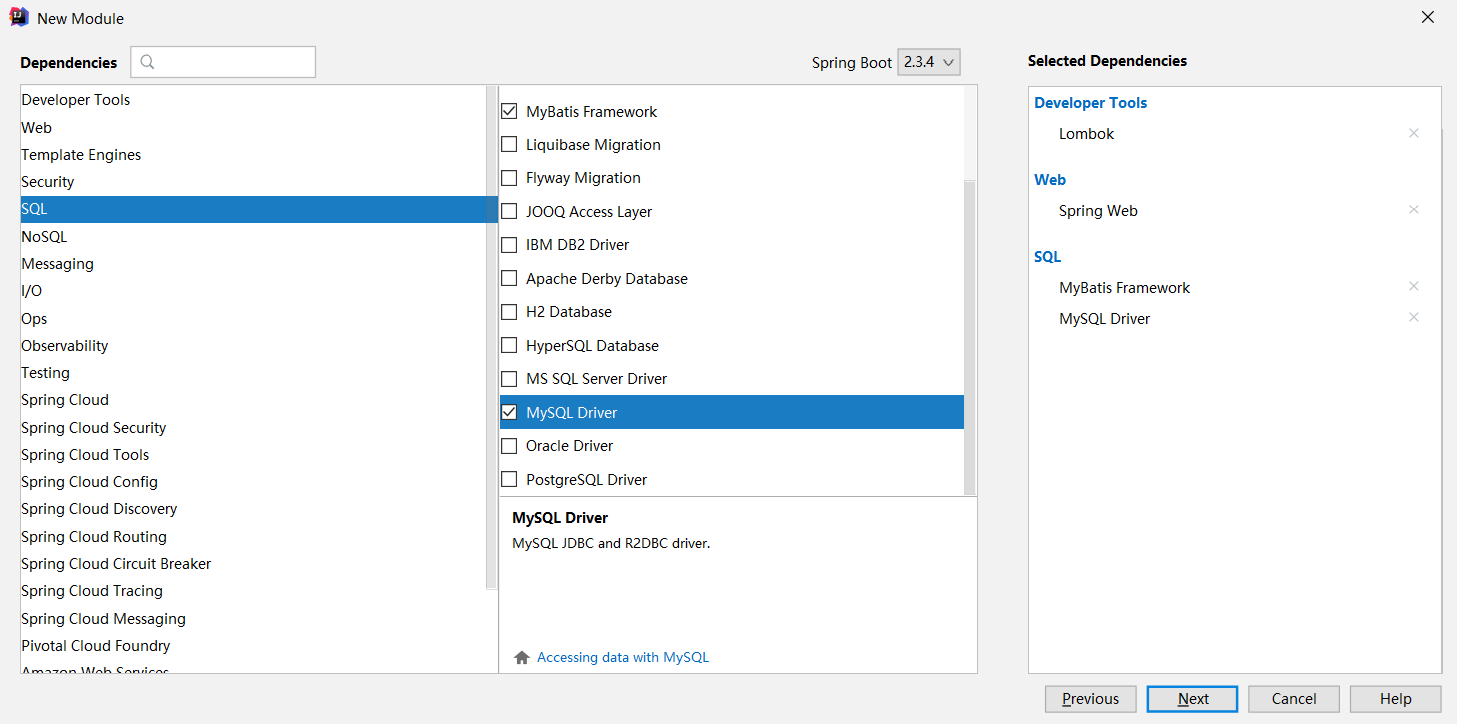
2. 导入pom.xml
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.3</version>
</dependency>
3. User实体类编写
@Data
public class User implements Serializable {
private Integer id;
private String username;
private String password;
private String birthday;
private static final long serialVersionUID = 1L;
}
4.UserMapper编写及Mapper文件
public interface UserMapper {
int deleteByPrimaryKey(Integer id);
int insert(User record);
int insertSelective(User record);
User selectByPrimaryKey(Integer id);
int updateByPrimaryKeySelective(User record);
int updateByPrimaryKey(User record);
List<User> queryAll();
}
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.base.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.base.pojo.User">
<id column="id" jdbcType="INTEGER" property="id" />
<result column="username" jdbcType="VARCHAR" property="username" />
<result column="password" jdbcType="VARCHAR" property="password" />
<result column="birthday" jdbcType="VARCHAR" property="birthday" />
</resultMap>
<sql id="Base_Column_List">
id, username, password, birthday
</sql>
<select id="selectByPrimaryKey" parameterType="java.lang.Integer"
resultMap="BaseResultMap">
select
<include refid="Base_Column_List" />
from user
where id = #{id,jdbcType=INTEGER}
</select>
<select id="queryAll" resultType="com.example.base.pojo.User">
select <include refid="Base_Column_List" />
from user
</select>
.......
</mapper>
5. UserService接口及实现类编写
import com.lagou.mapper.UserMapper;
import com.lagou.pojo.User;
import com.lagou.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> queryAll() {
return userMapper.queryAll();
}
@Override
public User findById(Integer id) {
return userMapper.selectByPrimaryKey(id);
}
@Override
public void insert(User user) {
userMapper.insertSelective(user);
}
@Override
public void deleteById(Integer id) {
userMapper.deleteByPrimaryKey(id);
}
@Override
public void update(User user) {
userMapper.updateByPrimaryKeySelective(user);
}
}
6. UserController编写
import com.lagou.pojo.User;
import com.lagou.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import org.yaml.snakeyaml.events.Event;
import java.util.List;
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/query")
public List<User> queryAll(){
return userService.queryAll();
}
@GetMapping("/query/{id}")
public User queryById(@PathVariable Integer id){
return userService.findById(id);
}
@DeleteMapping("/delete/{id}")
public String delete(@PathVariable Integer id){
userService.deleteById(id);
return "删除成功";
}
@PostMapping("/insert")
public String insert(User user){
userService.insert(user);
return "新增成功";
}
@PutMapping("/update")
public String update(User user){
userService.update(user);
return "修改成功";
}
}
7. application.yml
##服务器配置
server:
port: 8090
servlet:
context-path: /
##数据源配置
spring:
datasource:
name: druid
type: com.alibaba.druid.pool.DruidDataSource
url: jdbc:mysql://localhost:3306/springbootdata?characterEncoding=utf-
8&serverTimezone=UTC
username: root
password: wu7787879
#整合mybatis
mybatis:
mapper-locations: classpath:mapper/*Mapper.xml
#声明Mybatis映射文件所在的位置
8. 启动类
@SpringBootApplication
@MapperScan("com.lagou.mapper")
public class Springbootdemo5Application {
public static void main(String[] args) {
SpringApplication.run(Springbootdemo5Application.class, args);
}
}
9. 使用Postman测试
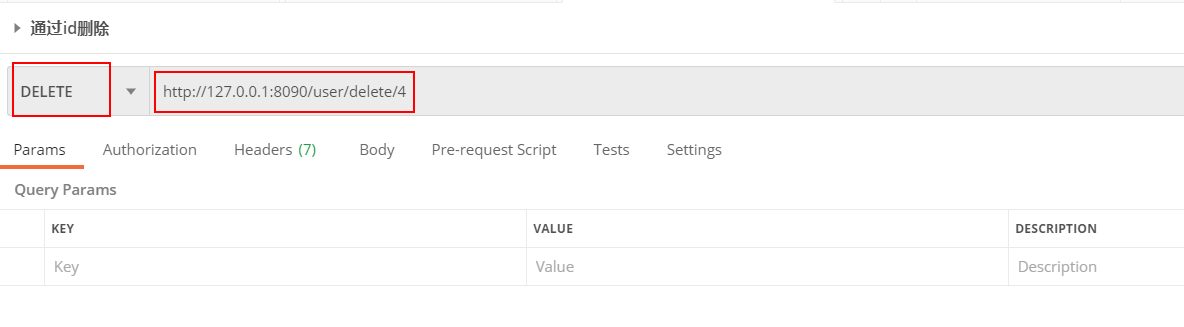
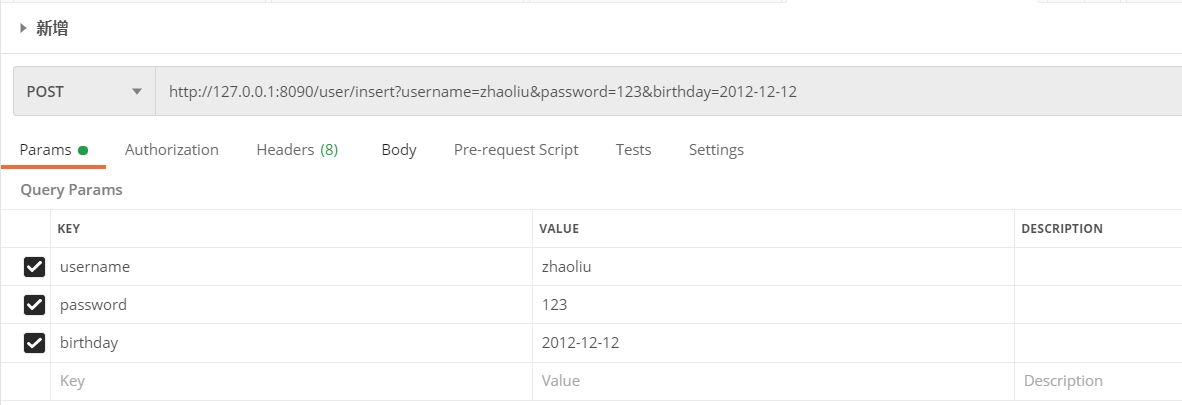
10.Spring Boot项目部署
需求:将Spring Boot项目使用maven指令打成jar包并运行测试
分析:
- 需要添加打包组件将项目中的资源、配置、依赖包打到一个jar包中;可以使用maven的
package ; - 部署:java -jar 包名
步骤实现:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
java -jar 包名
💖💖💖 完结撒花
💖💖💖 如果命运是世上最烂的编剧。 那么你就要争取,做你人生最好的演员
💖💖💖 写作不易,如果您觉得写的不错,欢迎给博主点赞、收藏、评论来一波~让博主更有动力吧
|