整理不易感谢支持,WecChat/QQ: 574373426 学编程找星哥
SpringBoot实现多数据源
在项目中经常会遇到一个项目需要操作多个数据库 实现的方式有很多,例如使用AOP注解控制,使用配置文件创建数据源等,本文采用的是创建数据源的配置文件来指定
实现:使用指定包下的mapper时走对应的数据库(mapper的包路径与指定数据库对应) AMapper走的是A数据库 BMapper走B数据库,以此思路来实现多数据源
SpringBoot配置文件 配置多个数据库 分别为 his/pt/lis
spring:
datasource:
his:
driver-class-name: com.microsoft.sqlserver.jdbc.SQLServerDriver
jdbc-url: jdbc:sqlserver://192.168.200.200\HIS;DatabaseName=his_fy
username: sa
password: 123456
initial-size: 5
min-idle: 5
max-active: 20
max-wait: 60000
time-between-eviction-runs-millis: 60000
min-evictable-idle-time-millis: 30000
max-evictable-idle-time-millis: 300000
pt:
driver-class-name: com.microsoft.sqlserver.jdbc.SQLServerDriver
jdbc-url: jdbc:sqlserver://192.168.200.200\HIS;DatabaseName=his_pt_data
username: sa
password: 123456
initial-size: 5
min-idle: 5
max-active: 20
max-wait: 60000
time-between-eviction-runs-millis: 60000
min-evictable-idle-time-millis: 30000
max-evictable-idle-time-millis: 300000
lis:
driver-class-name: com.microsoft.sqlserver.jdbc.SQLServerDriver
jdbc-url: jdbc:sqlserver://192.168.200.200\HIS;DatabaseName=LIS
username: sa
password: 123456
initial-size: 5
min-idle: 5
max-active: 20
max-wait: 60000
time-between-eviction-runs-millis: 60000
min-evictable-idle-time-millis: 30000
max-evictable-idle-time-millis: 300000
创建his对应的配置文件
@Configuration
@MapperScan(
basePackages = {"com.thwy.mapper.his"},
sqlSessionFactoryRef = "hisSqlSessionFactory"
)
public class HisDataSourceConfig {
@Bean(name = "hisDataSource")
@ConfigurationProperties(prefix = "spring.datasource.his")
public DataSource hisDataSource(){
return DataSourceBuilder.create().build();
}
@Bean(name = "hisSqlSessionFactory")
public SqlSessionFactory hisSqlSessionFactory(@Qualifier("hisDataSource") DataSource hisDataSource) throws Exception{
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(hisDataSource);
org.apache.ibatis.session.Configuration config = new org.apache.ibatis.session.Configuration();
config.setMapUnderscoreToCamelCase(true);
sqlSessionFactoryBean.setConfiguration(config);
sqlSessionFactoryBean.setMapperLocations(
new PathMatchingResourcePatternResolver().getResources("classpath:mapper/his/*.xml"));
return sqlSessionFactoryBean.getObject();
}
public SqlSessionTemplate hisSqlSessionTemplate(@Qualifier("hisSqlSessionFactory") SqlSessionFactory hisSqlSessionFactory){
return new SqlSessionTemplate(hisSqlSessionFactory);
}
}
创建pt对应的配置文件
@Configuration
@MapperScan(
basePackages = {"com.thwy.mapper.pt"},
sqlSessionFactoryRef = "ptSqlSessionFactory"
)
public class PTDataSourceConfig {
@Bean(name = "ptDataSource")
@ConfigurationProperties(prefix = "spring.datasource.pt")
public DataSource ptDataSource(){
return DataSourceBuilder.create().build();
}
@Bean(name = "ptSqlSessionFactory")
public SqlSessionFactory ptSqlSessionFactory(@Qualifier("ptDataSource") DataSource ptDataSource) throws Exception{
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(ptDataSource);
org.apache.ibatis.session.Configuration config = new org.apache.ibatis.session.Configuration();
config.setMapUnderscoreToCamelCase(true);
sqlSessionFactoryBean.setConfiguration(config);
sqlSessionFactoryBean.setMapperLocations(
new PathMatchingResourcePatternResolver().getResources("classpath:mapper/pt/*.xml"));
return sqlSessionFactoryBean.getObject();
}
public SqlSessionTemplate ptSqlSessionTemplate(@Qualifier("ptSqlSessionFactory") SqlSessionFactory ptSqlSessionFactory){
return new SqlSessionTemplate(ptSqlSessionFactory);
}
}
创建lis对应的配置文件
@Configuration
@MapperScan(
basePackages = {"com.thwy.mapper.lis"},
sqlSessionFactoryRef = "lisSqlSessionFactory"
)
public class LisDataSourceConfig {
@Bean(name = "lisDataSource")
@ConfigurationProperties(prefix = "spring.datasource.lis")
public DataSource lisDataSource(){
return DataSourceBuilder.create().build();
}
@Bean(name = "lisSqlSessionFactory")
public SqlSessionFactory lisSqlSessionFactory(@Qualifier("lisDataSource") DataSource lisDataSource) throws Exception{
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(lisDataSource);
org.apache.ibatis.session.Configuration config = new org.apache.ibatis.session.Configuration();
config.setMapUnderscoreToCamelCase(true);
sqlSessionFactoryBean.setConfiguration(config);
sqlSessionFactoryBean.setMapperLocations(
new PathMatchingResourcePatternResolver().getResources("classpath:mapper/lis/*.xml"));
return sqlSessionFactoryBean.getObject();
}
public SqlSessionTemplate lisSqlSessionTemplate(@Qualifier("lisSqlSessionFactory") SqlSessionFactory lisSqlSessionFactory){
return new SqlSessionTemplate(lisSqlSessionFactory);
}
}
实现 在配置文件中我们配置了 mapper的包扫描与xml文件的存放路径 此时当我们执行指定包下的mapper中的方法时,就会走与之对应的数据库 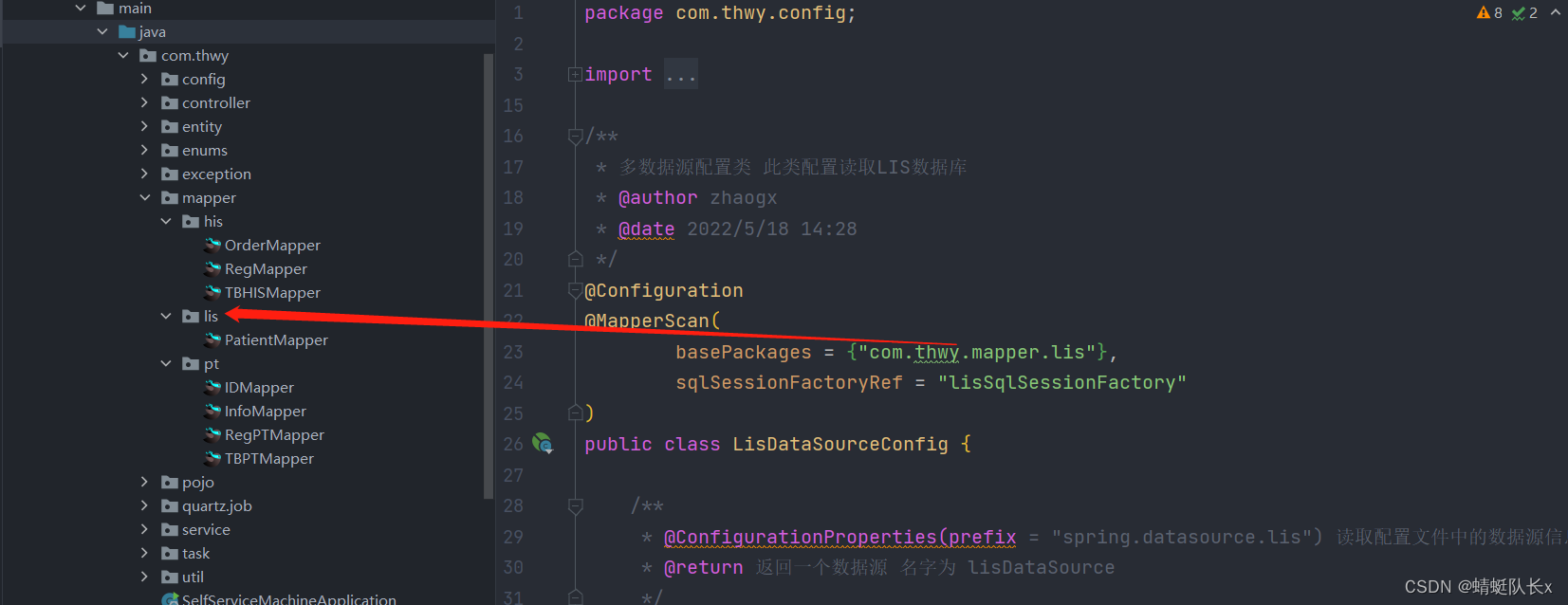 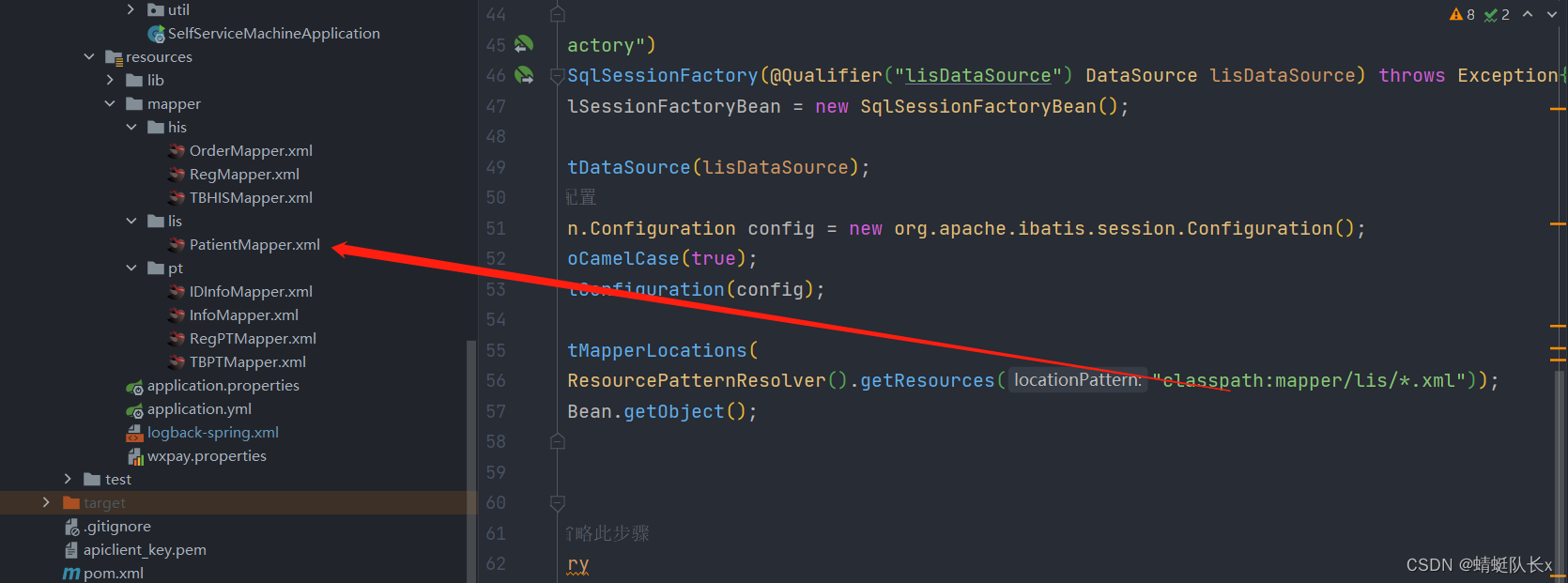
|