从零构建一个JAVA(SpringBoot)项目
1.创建一个Maven项目
1.1打开IDEA,点击创建项目
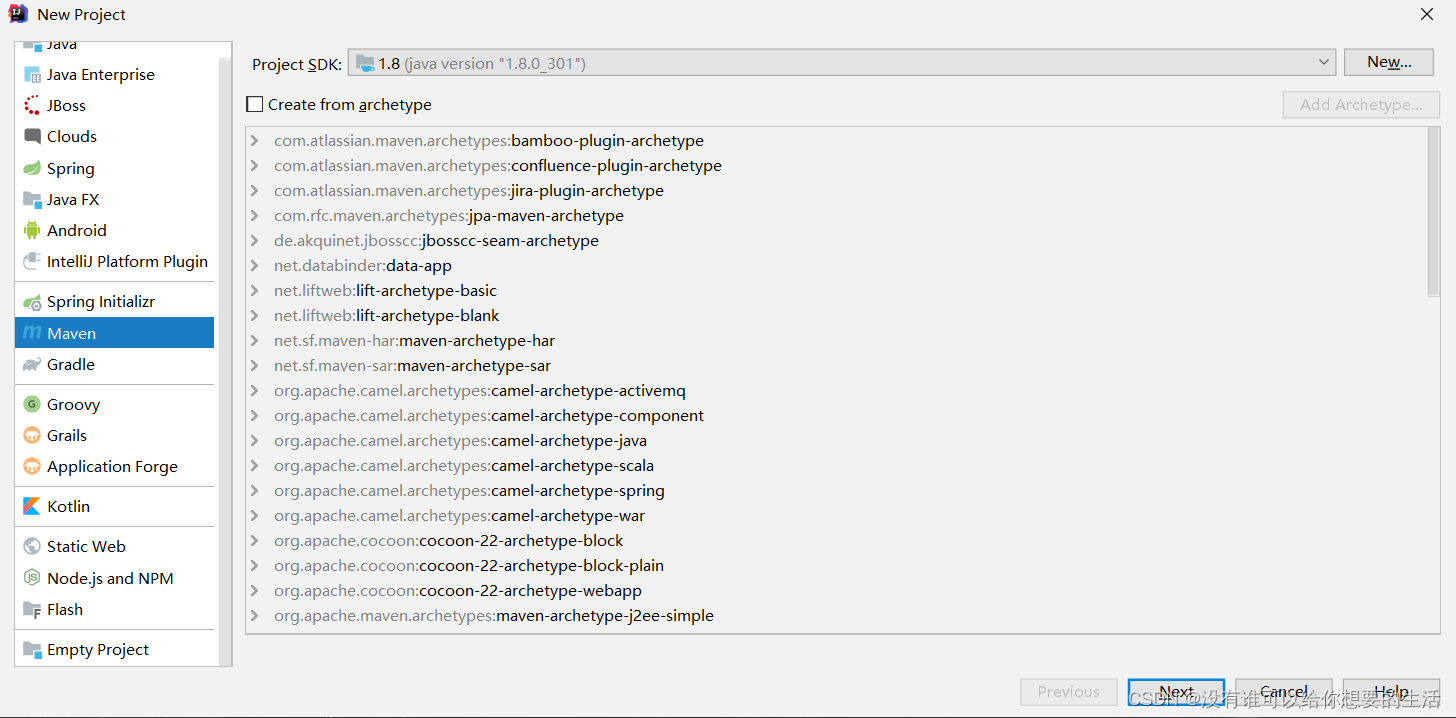
1.2 编写Hello World测试项目是否构建成功
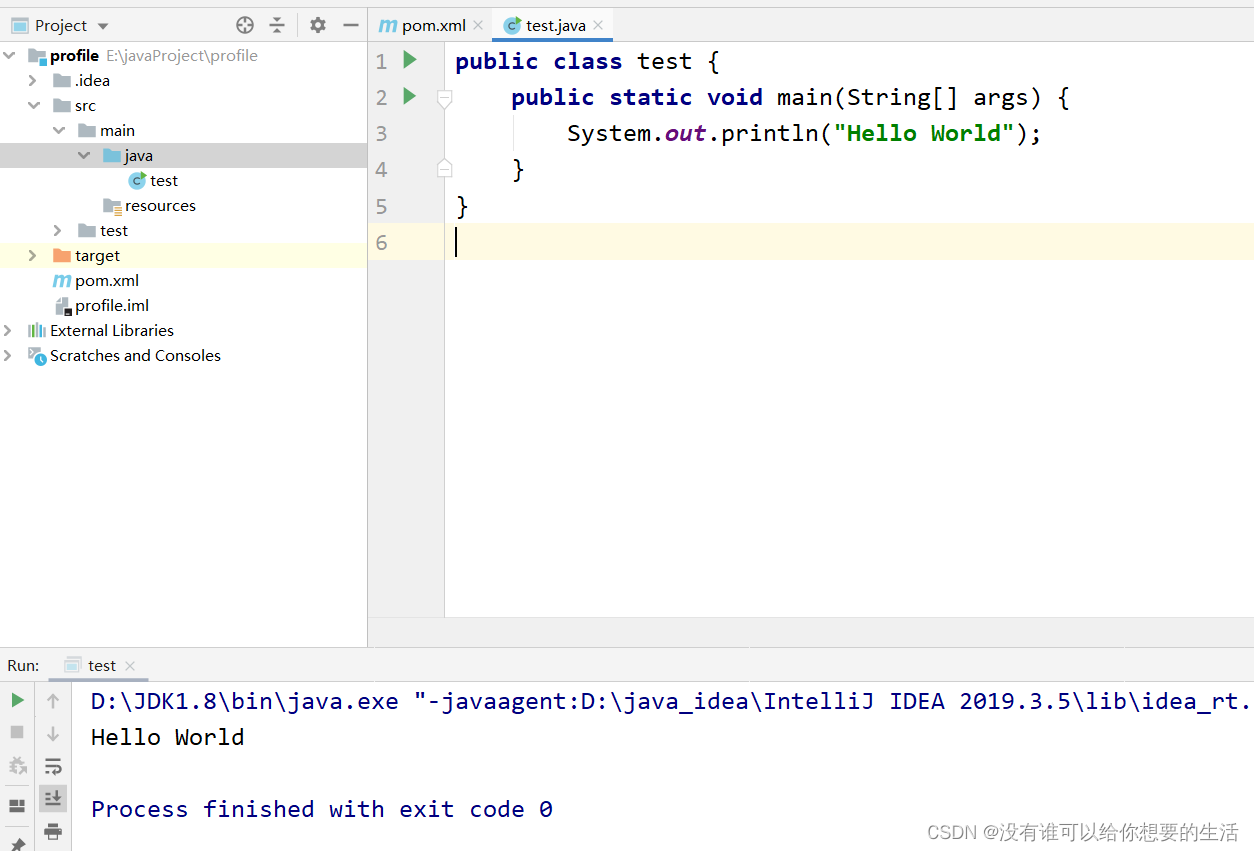
1.3 修改Maven本地仓库地址
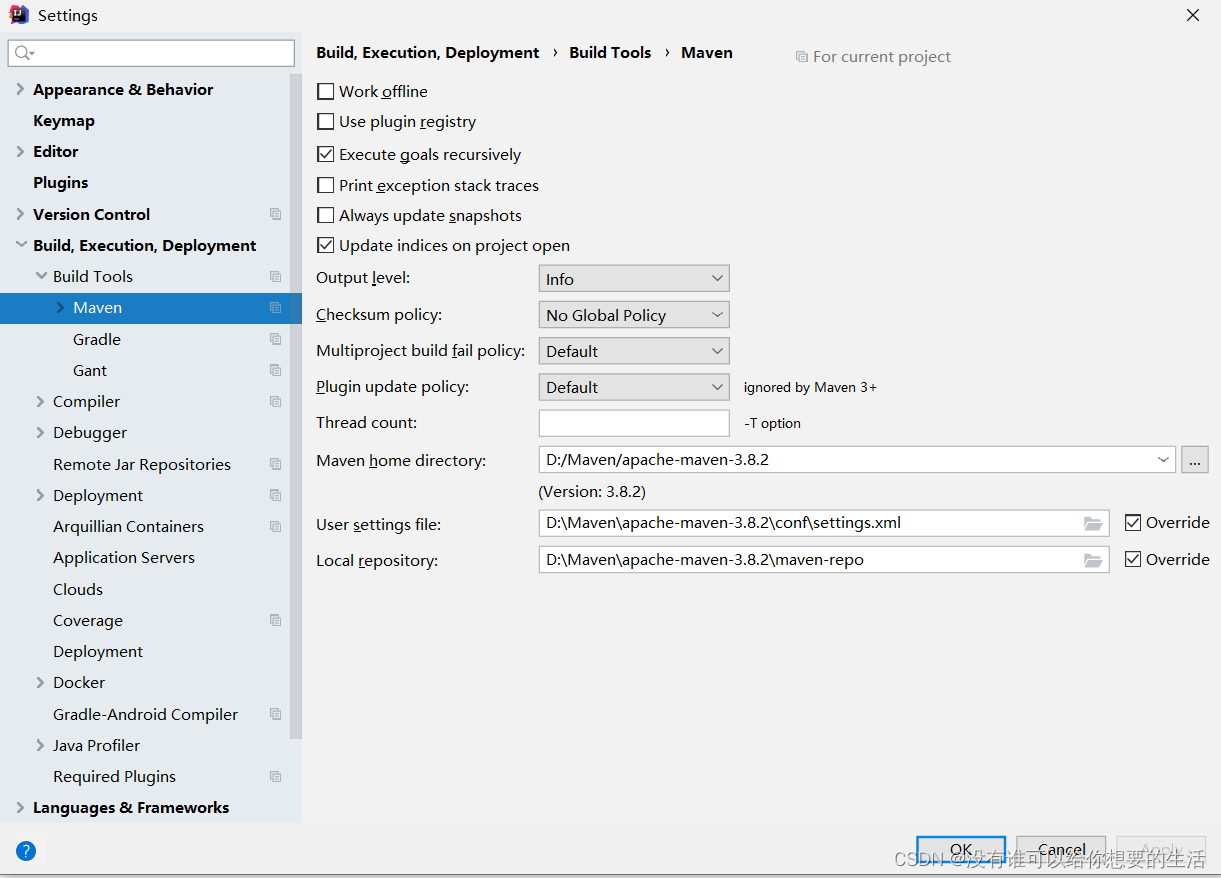
1.4 把项目提交到码云
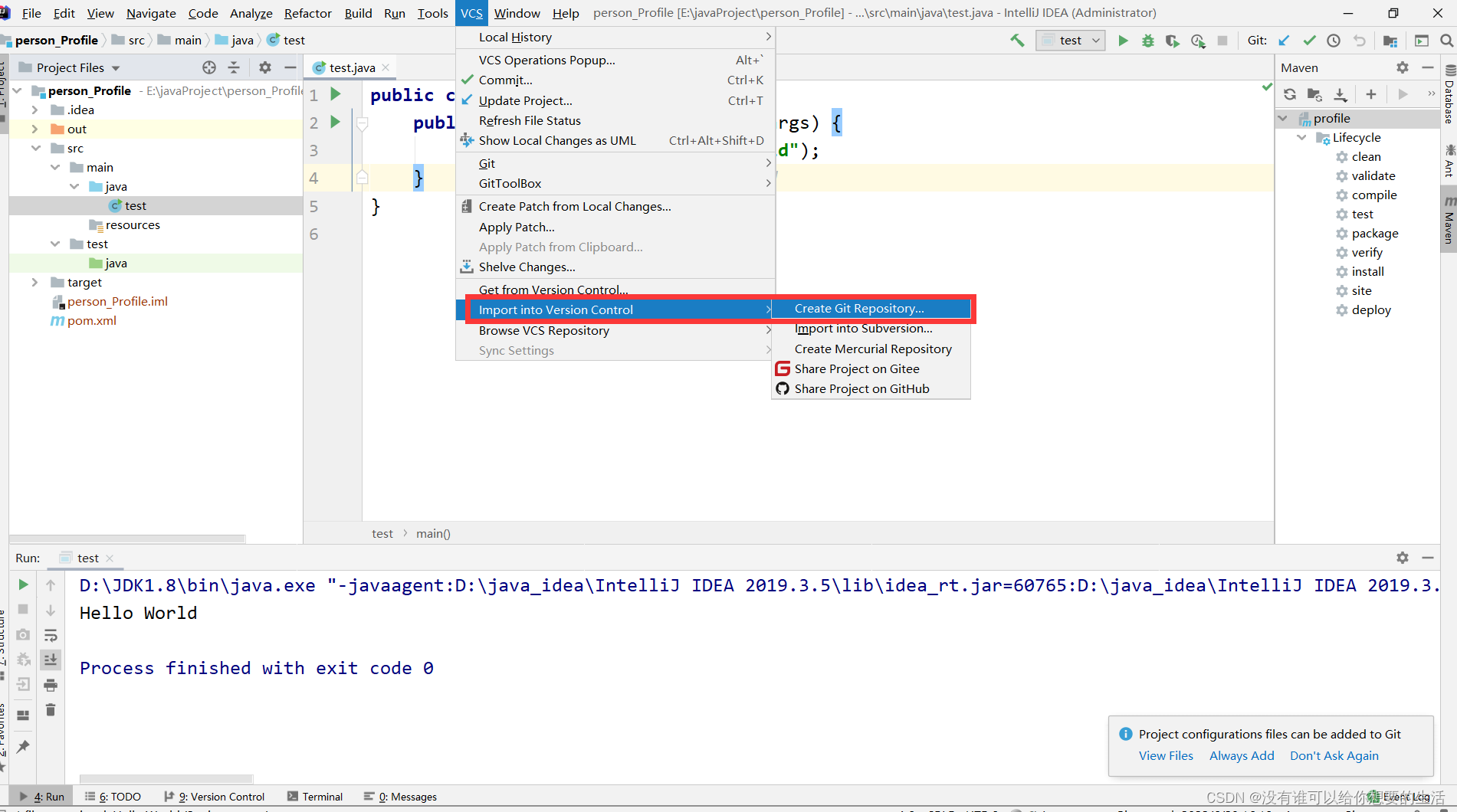
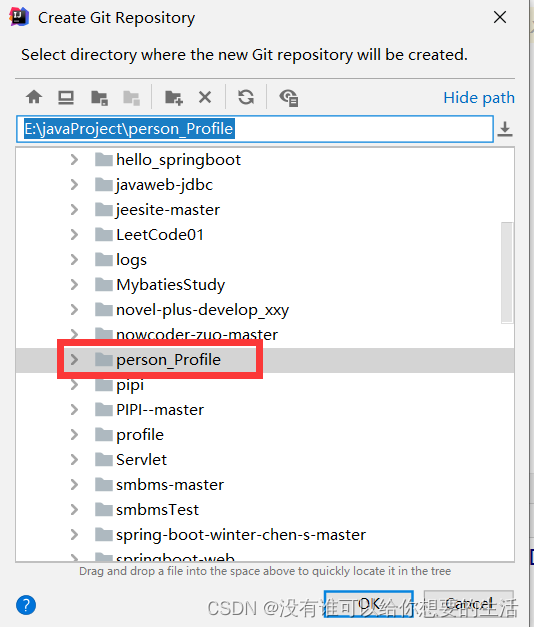
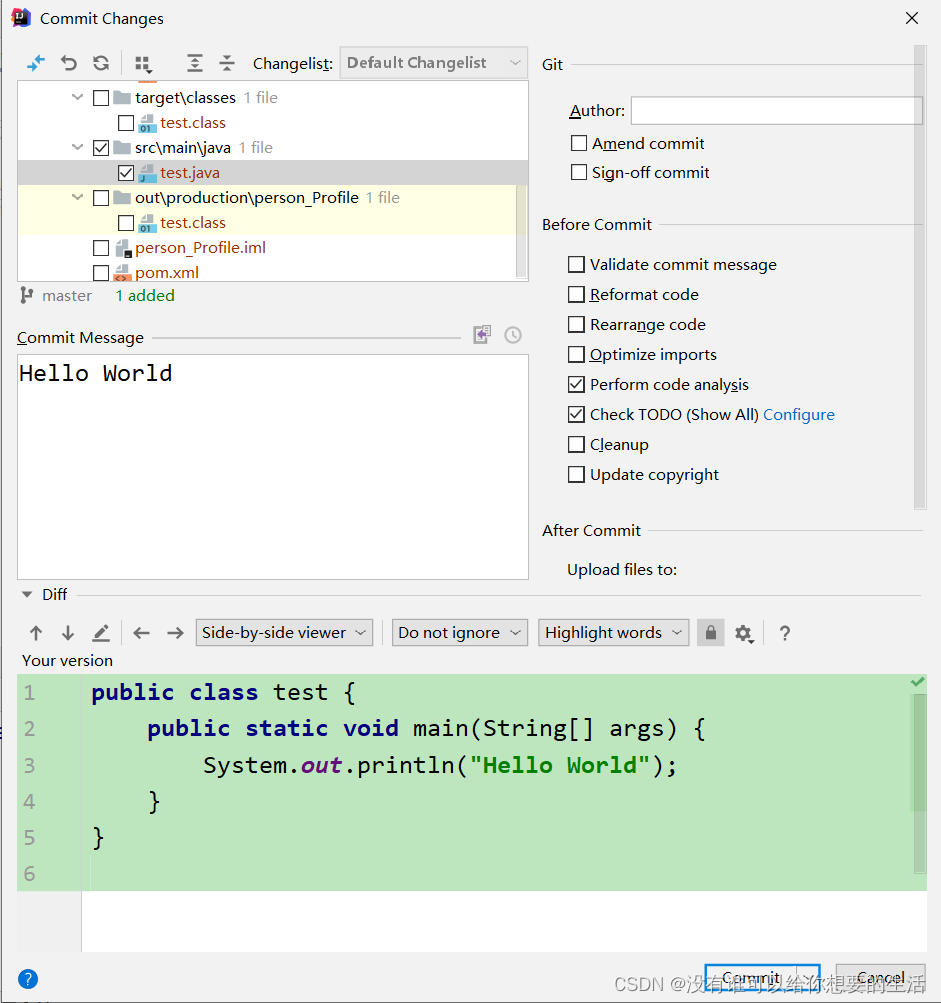
1.5 推送到远程仓库
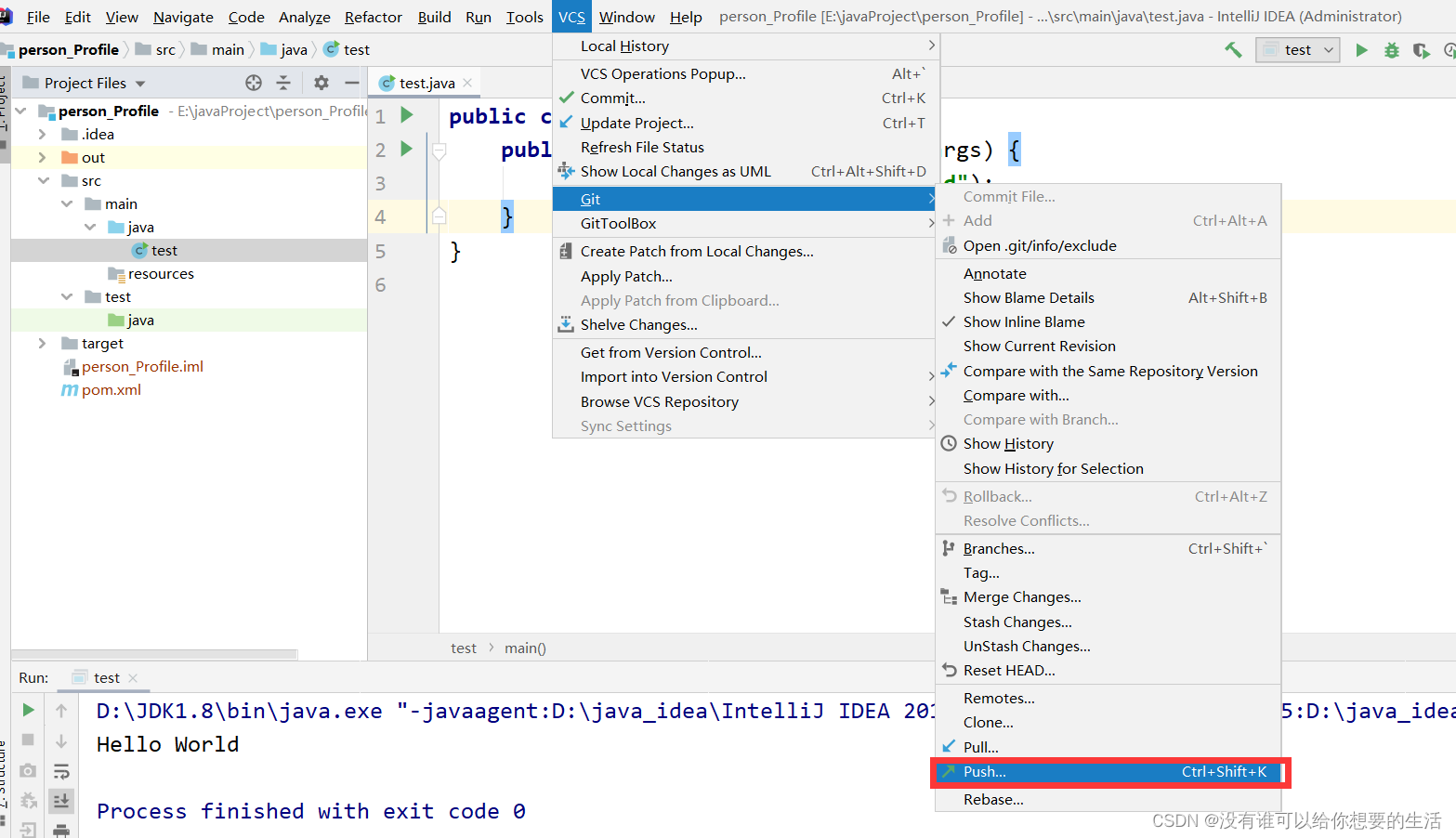
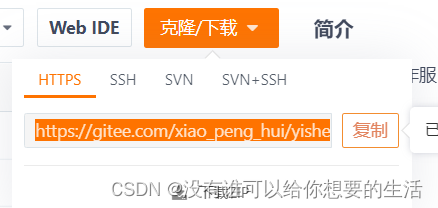
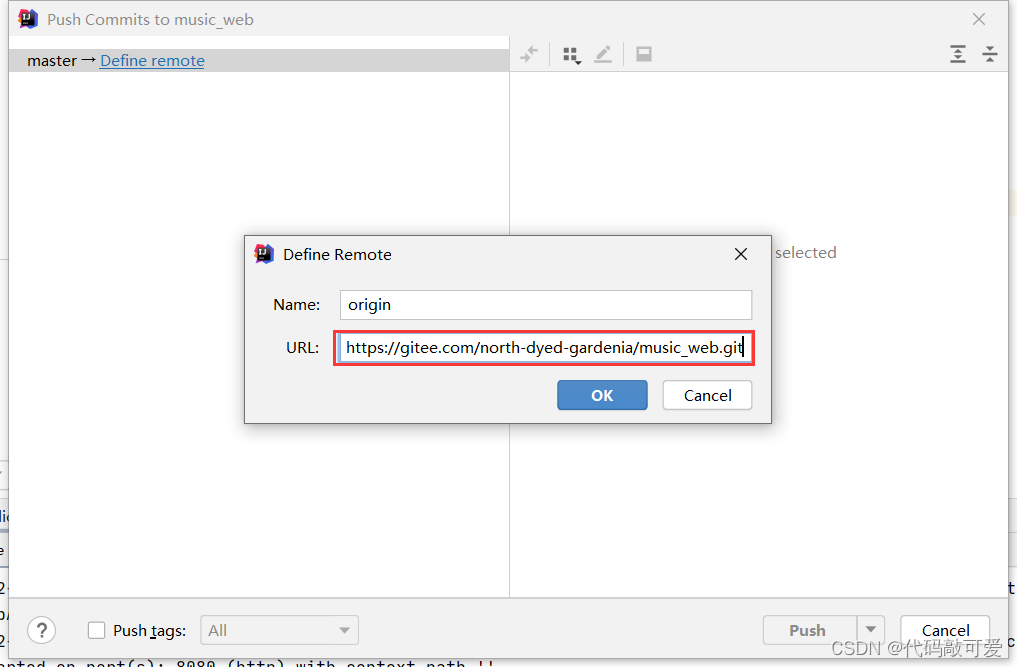
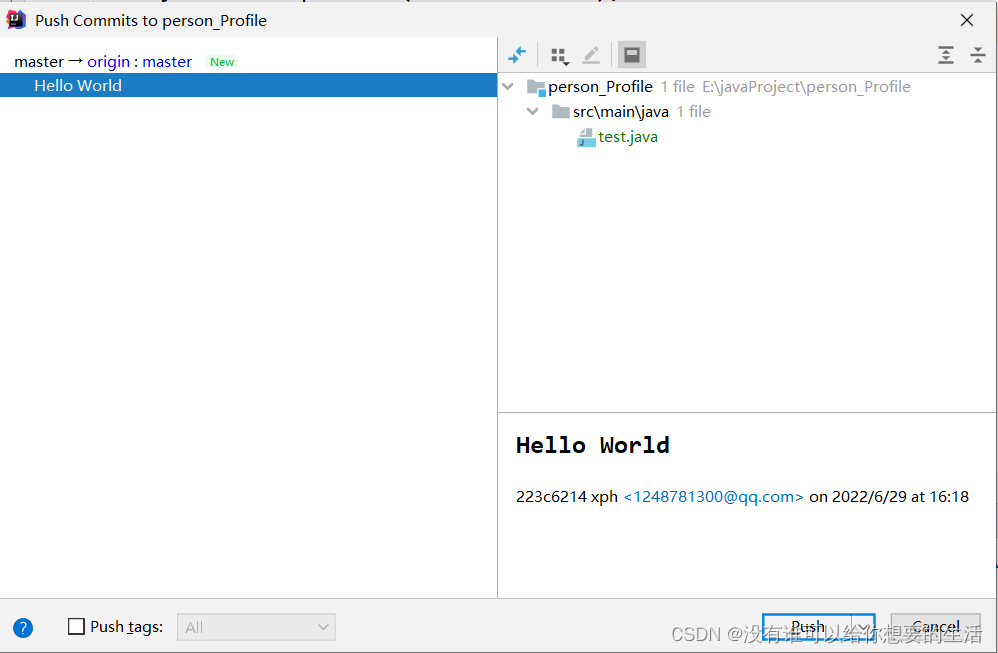
问题:
1.Push rejected Push to origin/master was rejected
大概原因是:初始化项目时,远程仓库我建了README.md文件,而本地仓库与远程仓库尚未进行文件关联,因此需要将两个仓库的文件进行关联后提交。
1.切换到自己项目所在的目录,右键选择GIT BASH Here,Idea中可使用Alt+F12
2.在terminl窗口中依次输入命令:
git pull
git pull origin master
git pull origin master --allow-unrelated-histories
3.在idea中重新push自己的项目
git push -u origin master -f
2.Git 中 出现couldn’t find remote ref –allow-unrelated-histories的解决办法
当执行git中的“git pull origin master –allow-unrelated-histories”命令时,会出现“ couldn’t find remote ref –allow-unrelated-histories”的错误,输入如下命令即可解决: git pull --rebase origin master git push origin maste
2.构建SpringBoot项目架构
2.1创建数据库
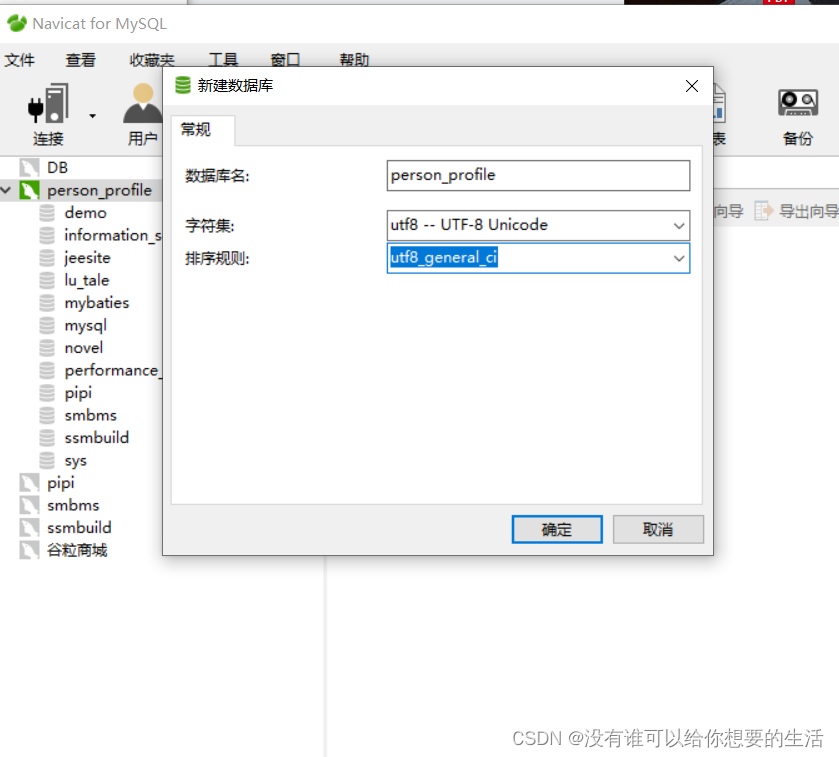
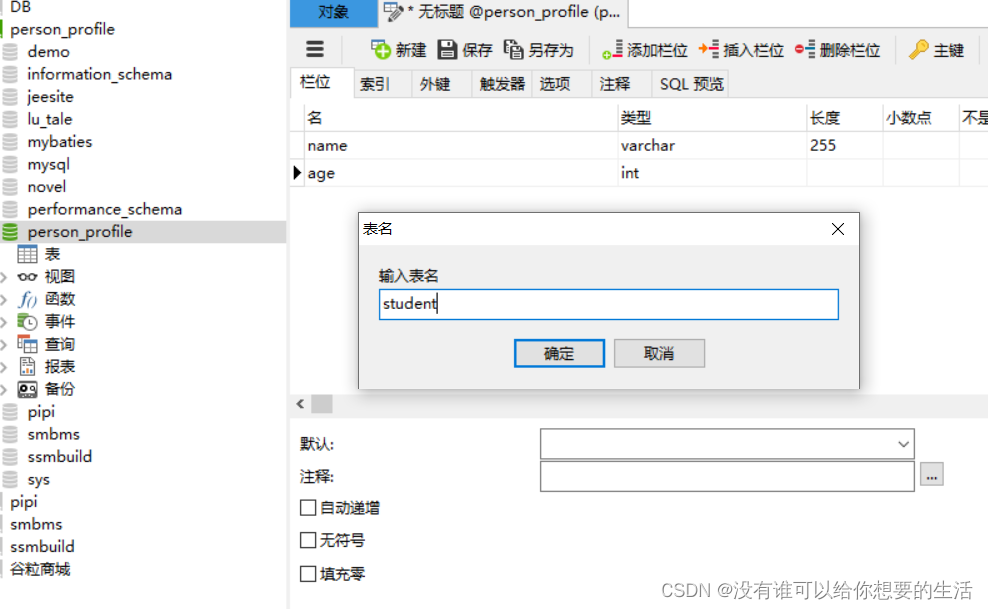
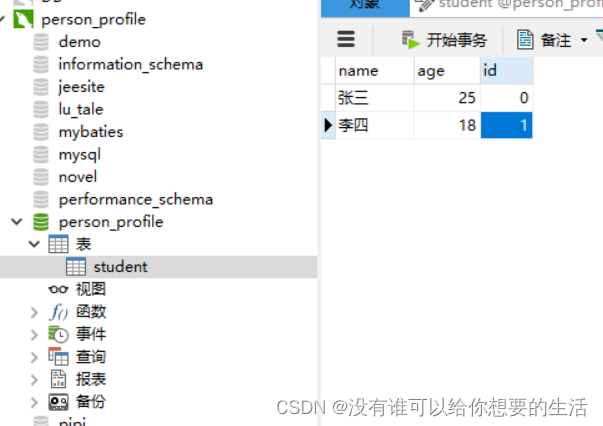
也可以通过sql创建表,添加字段信息,可以按F6切换到sql界面
2.2创建SpringBoot脚手架
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.1.RELEASE</version>
</parent>
<groupId>org.xph</groupId>
<artifactId>profile</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<!-- web-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- mysql数据库驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.17</version>
</dependency>
<!-- 数据库连接池-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.3</version>
</dependency>
<!-- fastjson-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.75</version>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.5.8</version>
</dependency>
</dependencies>
</project>
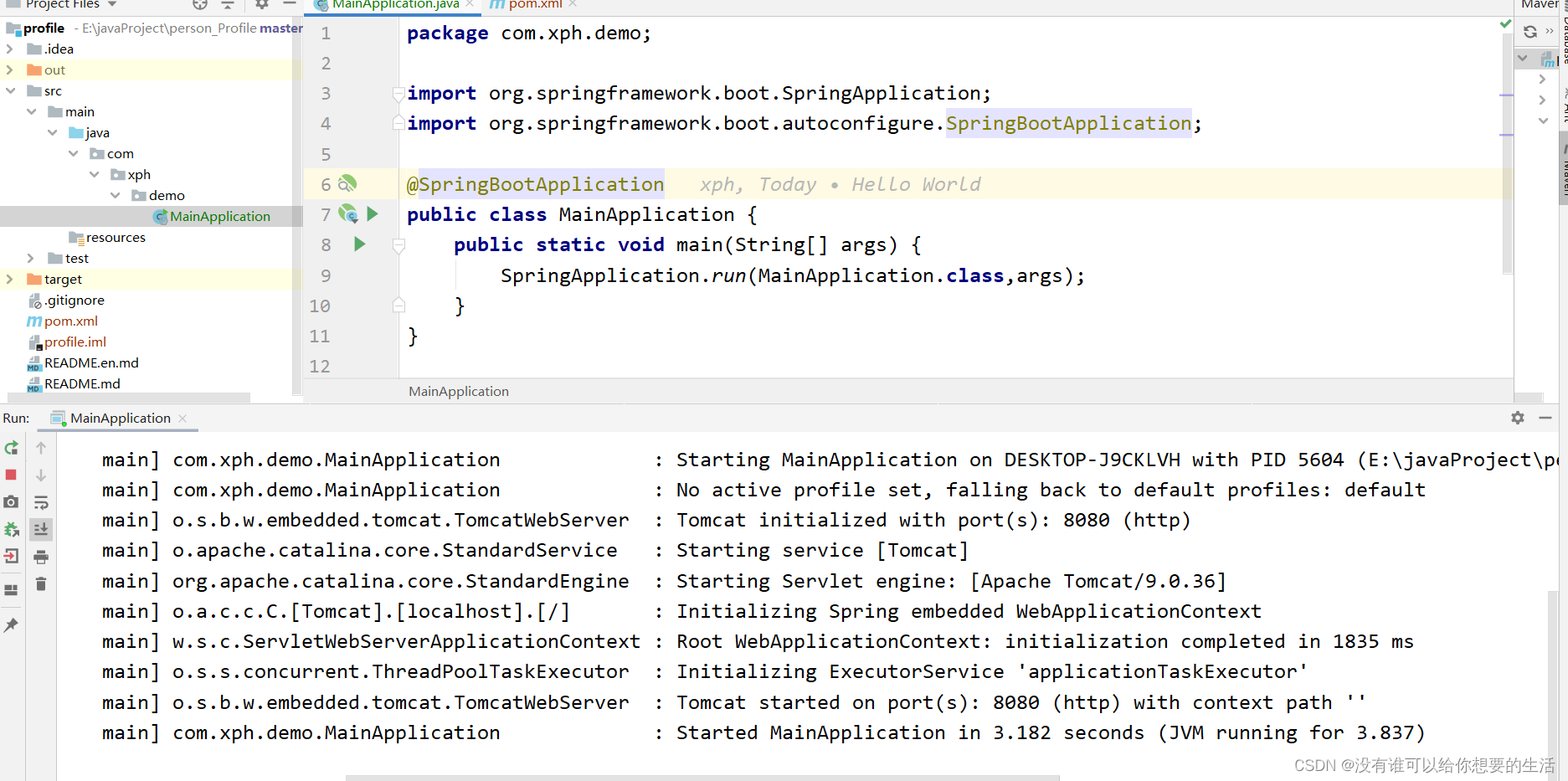
-
编写测试Controller类 package com.xph.demo;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class demoController {
@RequestMapping("/hello")
public String hello()
{
return "Hello World";
}
}
@RestController @RestController = @Controller + @ResponseBody组成,等号右边两位同志简单介绍两句,就明白我们@RestController的意义了:
- @Controller 将当前修饰的类注入SpringBoot IOC容器,使得从该类所在的项目跑起来的过程中,这个类就被实例化。当然也有语义化的作用,即代表该类是充当Controller的作用
- @ResponseBody 它的作用简短截说就是指该类中所有的API接口返回的数据,甭管你对应的方法返回Map或是其他Object,它会以Json字符串的形式返回给客户端,本人尝试了一下,如果返回的是String类型,则仍然是String
@RequestMapping 作用:将请求和处理请求的控制器方法关联起来,建立映射关系。 位置: 1、标识类:设置映射请求的请求路径的初试信息 2、表示方法:设置映射请求的请求路径的具体信息 -
输入http://localhost:8080/hello,测试
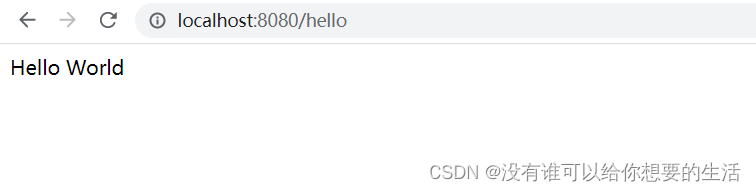
2.3 SpringBoot连接MYSQL数据库
-
添加mybatis-plus依赖
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.2</version>
</dependency>
-
添加完,完整的pom.xml文件如下 <?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.1.RELEASE</version>
</parent>
<groupId>org.xph</groupId>
<artifactId>profile</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.17</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.3</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.75</version>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.5.8</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.2</version>
</dependency>
</dependencies>
</project>
-
配置数据库相关设置 spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
username: root
password: root
url: jdbc:mysql://localhost:3306/person_profile?useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8
mybatis-plus:
mapper-locations: classpath*:/mapper/**/*.xml
configuration:
map-underscore-to-camel-case: true
cache-enabled: true
lazy-loading-enabled: true
multiple-result-sets-enabled: true
log-impl: org.apache.ibatis.logging.nologging.NoLoggingImpl
global-config:
db-config:
id-type: assign_id
table-underline: true
banner: false
enable-sql-runner: true
configuration-properties:
prefix:
blobType: BLOB
boolValue: true
2.4建立规范化的SpringBoot模块
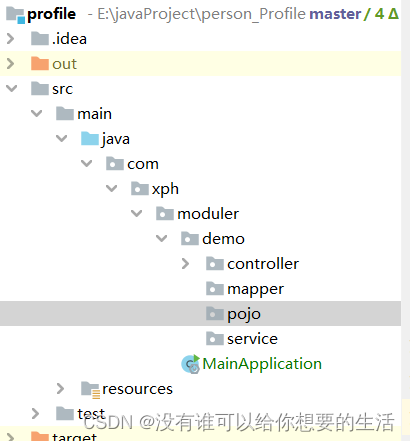
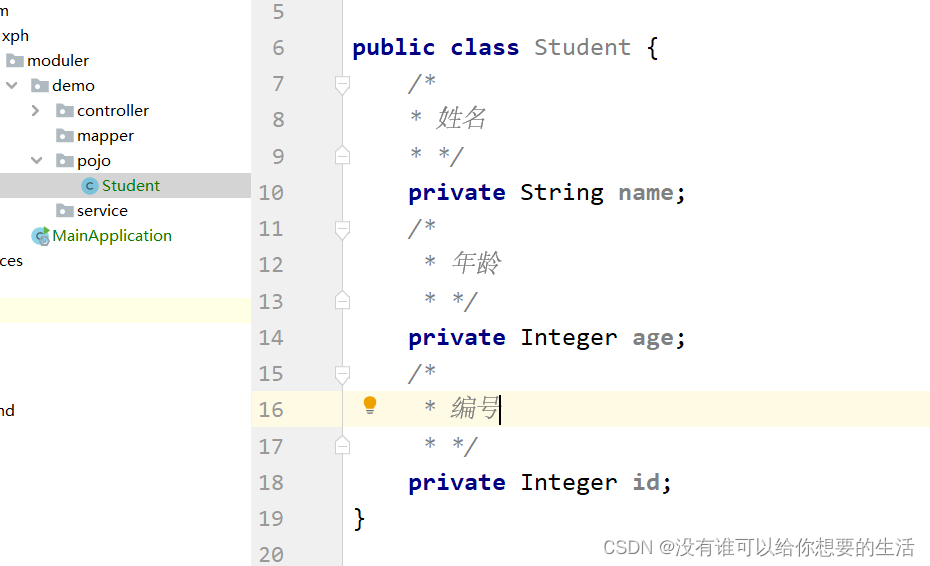
package com.xph.moduler.demo.pojo;
import com.baomidou.mybatisplus.annotation.TableName;
import java.security.PrivateKey;
import java.sql.PreparedStatement;
@TableName("student")
public class Student {
private String name;
private Integer age;
private Integer id;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
}
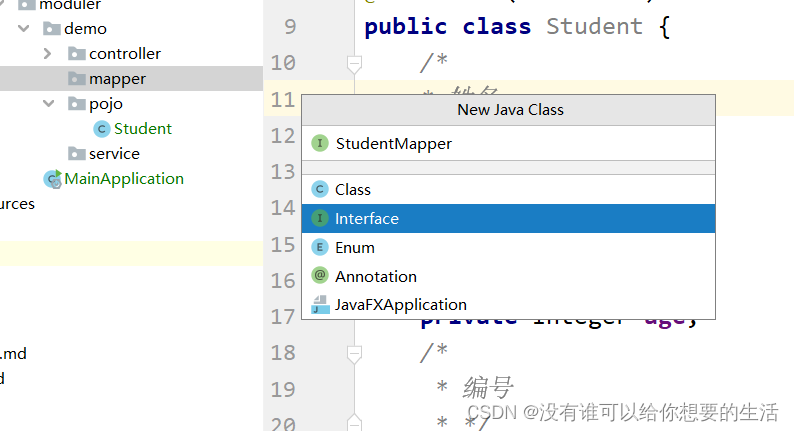
package com.xph.moduler.demo.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.xph.moduler.demo.pojo.Student;
@Mapper
public interface StudentMapper extends BaseMapper<Student> {
}
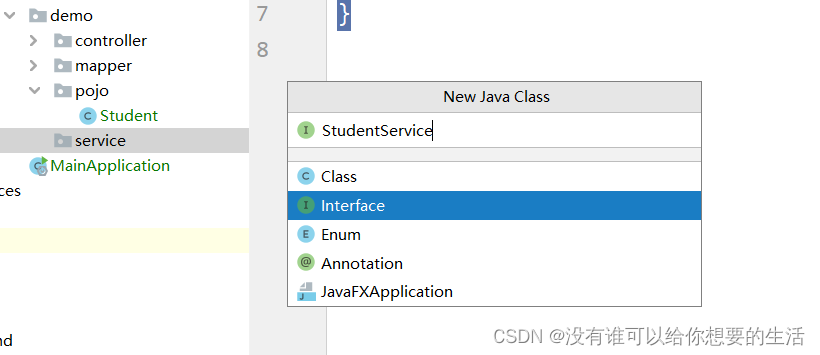
package com.xph.moduler.demo.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.xph.moduler.demo.pojo.Student;
public interface StudentService extends IService<Student> {
}
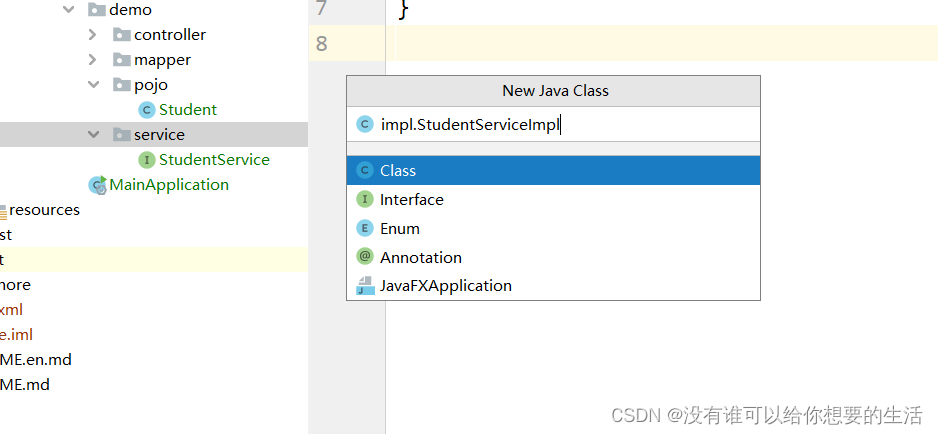
package com.xph.moduler.demo.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.xph.moduler.demo.mapper.StudentMapper;
import com.xph.moduler.demo.pojo.Student;
import com.xph.moduler.demo.service.StudentService;
import org.springframework.stereotype.Service;
@Service
public class StudentServiceImpl extends ServiceImpl<StudentMapper, Student> implements StudentService {
}
-
编写Controller package com.xph.moduler.demo.controller;
import com.xph.moduler.demo.pojo.Student;
import com.xph.moduler.demo.service.StudentService;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import java.util.List;
@RestController
public class demoController {
@Resource
private StudentService studentService;
@RequestMapping("/student")
public List<Student> getStudentList() {
return studentService.list();
}
@RequestMapping("/hello")
public String hello() {
return "Hello World";
}
}
-
输入http://localhost:8080/student
import java.util.List;
@RestController
public class demoController {
@Resource
private StudentService studentService;
@RequestMapping("/student")
public List<Student> getStudentList() {
return studentService.list();
}
@RequestMapping("/hello")
public String hello() {
return "Hello World";
}
}
|