1.SpringBoot Starter机制
1.1 什么是Starter机制
SpringBoot中的starter是一种非常重要的机制,能够抛弃以前繁杂的配置,将其统一集成进starter,应用者只需要在maven中引入starter依赖,SpringBoot就能自动扫描到要加载的信息并启动相应的默认配置。starter让我们摆脱了各种依赖库的处理,需要配置各种信息的困扰。SpringBoot会自动通过classpath路径下的类发现需要的Bean,并注册进IOC容器SpringBoot提供了针对日常企业应用研发各种场景的spring-boot-starter依赖模块。所有这些依赖模块都遵循着约定成俗的默认配置,并允许我们调整这些配置,即遵循“约定大于配置”的理念。比如我们在springboot里面要引入redis,那么我们需要在pom中引入以下内容:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
这其实就是一个starter。简而言之,starter就是一个外部的项目,我们需要使用它的时候就可以在当前springboot项目中引入它。
1.2 为什么要定义Starter
在我们的日常开发工作中,经常会有一些独立于业务之外的配置模块,我们经常将其放到一个特定的包下,然后如果另一个工程需要复用这块功能的时候,需要将代码硬拷贝到另一个工程,重新集成一遍,麻烦至极。如果我们将这些可独立于业务代码之外的功配置模块封装成一个个starter,复用的时候只需要将其在pom中引用依赖即可,再由SpringBoot为我们完成自动装配,就非常轻松了。
2.自定义Starter
2.1 自定义Starter命名规则
SpringBoot提供的starter以 spring-boot-starter-xxx 的方式命名的。 官方建议自定义的starter使用 xxx-spring-boot-starter 命名规则。以区分SpringBoot生态提供的starter。
2.2 自定义Starter实现
1.自定义Starter (1)新建maven jar工程,工程名为custom-spring-boot-starter,导入依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure</artifactId>
<version>2.2.9.RELEASE</version>
</dependency>
</dependencies>
(2)编写自定义Bean: CustomSimpleBean
@EnableConfigurationProperties(CustomSimpleBean.class)
@ConfigurationProperties(prefix = "custom")
public class CustomSimpleBean {
private int id;
private String name;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "CustomSimpleBean{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
(3)编写配置类CustomAutoConfiguration
@Configuration
public class CustomAutoConfiguration {
static {
System.out.println("CustomAutoConfiguration init....");
}
@Bean
public CustomSimpleBean customSimpleBean(){
return new CustomSimpleBean();
}
}
(4)resources下创建/META-INF/spring.factories 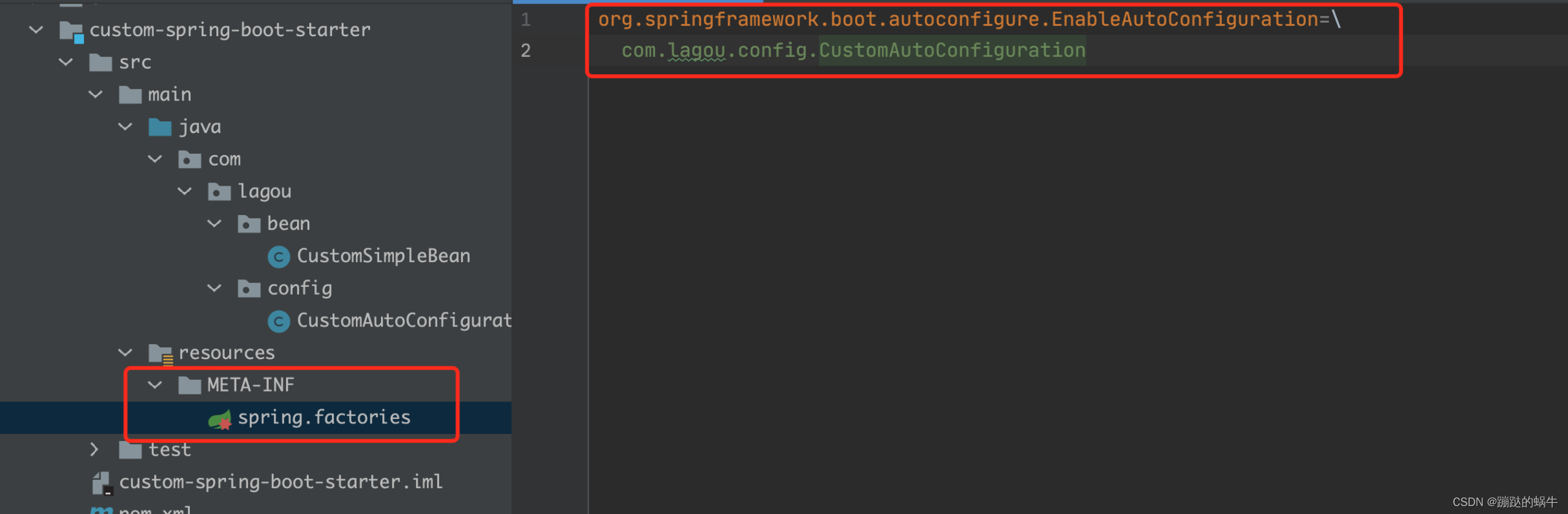
上面这句话的意思就是SpringBoot启动的时候会去加载我们的simpleBean到IOC容器中。这其实是一种变形的SPI机制。 2.使用Starter (1)导入自定义Starter的依赖
<dependency>
<groupId>com.lagou</groupId>
<artifactId>custom-spring-boot-starter</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
(2)配置属性
custom.id=1
custom.name=Custom starter
(3)自定义测试类进行测试
@RunWith(SpringRunner.class)
@SpringBootTest
public class CustomStarterTest {
@Autowired
private CustomSimpleBean customSimpleBean;
@Test
public void customSimpleBeanTest(){
System.out.println(customSimpleBean);
}
}
运行结果: 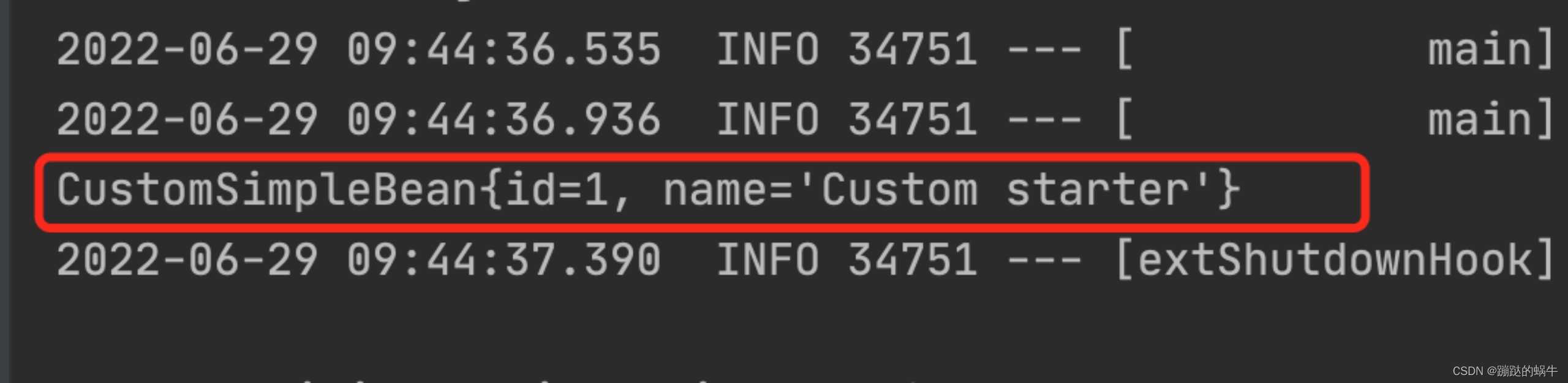
但此处还有一个问题,如果有一天我们不想要启动工程的时候自动装配SimpleBean呢?你可能会想,那就去pom中把依赖注释掉,的确,这是一种方案,但为免有点Low。下面说到热插拔技术。
2.3 热插拔技术
我们经常会在启动类Application上面加@EnableXXX注解,其实这个@Enablexxx注解就是一种热拔插技术,加了这个注解就可以启动对应的starter,当不需要对应的starter的时候只需要把这个注解注释掉就行,这就显得很优雅了。 (1) 新增标记类ConfigMarker
public class ConfigMarker {
}
(2) 新增EnableRegisterServer注解
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Import({ConfigMarker.class})
public @interface EnableRegisterServer {
}
(3) 改造 CustomAutoConfiguration 新增条件注解 @ConditionalOnBean(ConfigMarker.class) ,@ConditionalOnBean 这个是条件注解,前面的意思代表只有当期上下文中含有 ConfigMarker对象,被标注的类才会被实例化。
@Configuration
@ConditionalOnBean(ConfigMarker.class)
public class CustomAutoConfiguration {
static {
System.out.println("CustomAutoConfiguration init....");
}
@Bean
public CustomSimpleBean customSimpleBean(){
return new CustomSimpleBean();
}
}
(4) 在启动类上新增@EnableImRegisterServer注解
@SpringBootApplication
@EnableRegisterServer
public class SpringBootMyTestApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootMyTestApplication.class, args);
}
}
到此热插拔就实现好了,当你加了 @EnableImRegisterServer 的时候启动zdy工程就会自动装配CustomSimpleBean,反之则不装配。原理也很简单,当加了 @EnableImRegisterServer 注解的时候,由于这个注解使用了@Import({ConfigMarker.class}) ,所以会导致Spring去加载 ConfigMarker 到上下文中,而又因为条件注解 @ConditionalOnBean(ConfigMarker.class) 的存在,所以CustomAutoConfiguration 类就会被实例化。
附上条件注解的的讲解 @ConditionalOnBean:仅仅在当前上下文中存在某个对象时,才会实例化一个Bean。@ConditionalOnClass:某个class位于类路径上,才会实例化一个Bean。@ConditionalOnExpression:当表达式为true的时候,才会实例化一个Bean。基于SpEL表达式的条件判断。 @ConditionalOnMissingBean:仅仅在当前上下文中不存在某个对象时,才会实例化一个Bean。 @ConditionalOnMissingClass:某个class类路径上不存在的时候,才会实例化一个Bean。@ConditionalOnNotWebApplication:不是web应用,才会实例化一个Bean。@ConditionalOnWebApplication:当项目是一个Web项目时进行实例化。@ConditionalOnNotWebApplication:当项目不是一个Web项目时进行实例化。@ConditionalOnProperty:当指定的属性有指定的值时进行实例化。 @ConditionalOnJava:当JVM版本为指定的版本范围时触发实例化。@ConditionalOnResource:当类路径下有指定的资源时触发实例化。 @ConditionalOnJndi:在JNDI存在的条件下触发实例化。 @ConditionalOnSingleCandidate:当指定的Bean在容器中只有一个,或者有多个但是指定了首选的Bean时触发实例化
|