版本:elasticsearch7.17,jdk8
父工程依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.3.12.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<version>2.3.12.RELEASE</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.meng</groupId>
<artifactId>service</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.16</version>
<scope>compile</scope>
</dependency>
</dependencies>
子工程依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<version>${spring-boot.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
<version>${spring-boot.version}</version>
</dependency>
</dependencies>
application.yml(新版springboot2.3.12使用此配置,本文基于此版本)
spring:
elasticsearch:
rest:
uris: 192.168.233.134:9200
application.yml(旧版springboot2.1.6使用此配置)
spring:
data:
elasticsearch:
cluster-nodes: 192.168.233.134:9300
启动类
@SpringBootApplication
public class EsApplication {
public static void main(String[] args) {
SpringApplication.run(EsApplication.class , args);
}
}
实体类(es7中创建索引方式好像变了,这里行不通了)
@Data
@Document(indexName = "goods" , shards = 3 , replicas = 0)
public class Goods {
@Id
private Integer id;
@Field(type = FieldType.Text , analyzer = "ik_max_word")
private String name;
@Field(type = FieldType.Keyword)
private String category;
@Field(type = FieldType.Keyword)
private String images;
@Field(type = FieldType.Integer)
private Integer price;
}
Spring Data通过注解来声明字段的映射属性,有下面的三个注解:
-
@Document 作用在类,标记实体类为文档对象,一般有四个属性 indexName:对应索引库名称
type:对应在索引库中的类型
shards:分片数量,默认5
replicas:副本数量,默认1
-
@Id 作用在成员变量,标记一个字段作为id主键 -
@Field 作用在成员变量,标记为文档的字段,并指定字段映射属性: type:字段类型,取值是枚举:FieldType
index:是否索引,布尔类型,默认是true
store:是否存储,布尔类型,默认是false
analyzer:分词器名称:ik_max_word
Repository
public interface EsRepository extends ElasticsearchRepository<User, Integer> {
}
ElasticsearchTemplate、ElasticsearchRepository、ElasticsearchRestTemplate区别
ElasticsearchRepository可以做Elasticsearch的相关数据的增删改查,用法和普通的接口是一样的,这样就能统一ElasticSearch和普通的JPA操作,获得和操作mysql一样的代码体验。同时也可以看到ElasticsearchRepository的功能是比较少的,简单查询够用,但复杂查询就稍微显得力不从心了。 ElasticsearchRepository继承自PagingAndSortingRepository,PagingAndSortingRepository又继承CrudRepository ElasticsearchRepository中的方法基本都过时了 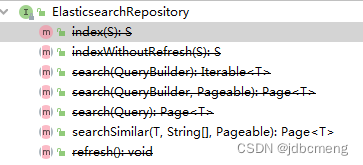 PagingAndSortingRepository中的两个方法,排序和分页
@NoRepositoryBean
public interface PagingAndSortingRepository<T, ID> extends CrudRepository<T, ID> {
Iterable<T> findAll(Sort sort);
Page<T> findAll(Pageable pageable);
}
CrudRepository中的方法就是简单的增删查改 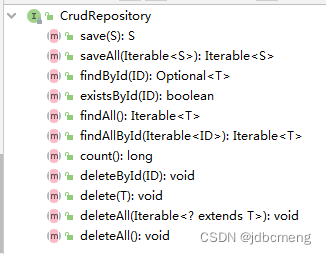 详见es中ElasticsearchRepository 的原理和使用
ElasticsearchTemplate 则提供了更多的方法,同时也包括分页之类的,他其实就是一个封装好的ElasticSearch Util功能类,通过直接连接client来完成数据的操作 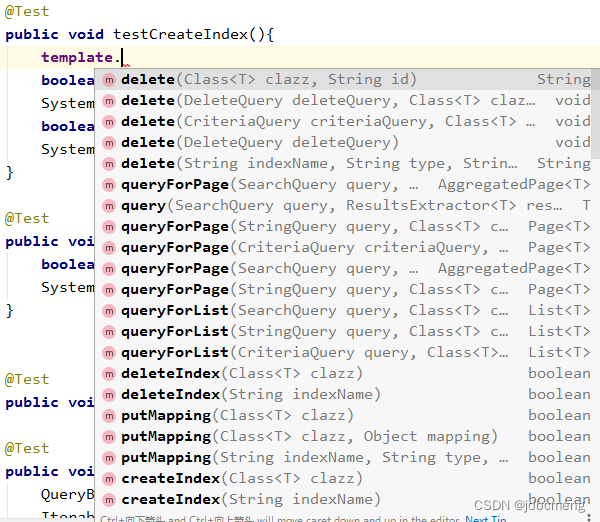 详见es中ElasticSearchTemplate类的实现原理及使用
ElasticsearchRestTemplate 在新版的SpringBoot项目中,在这个包下,推荐使用的是ElasticsearchRestTemplate这个类(ElasticsearchTemplate不推荐使用了),和之前的用法有些不同。 本文使用springboot版本:2.3.12 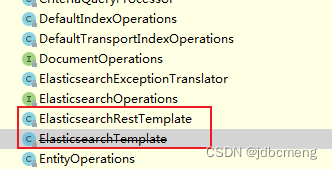
参考:ElasticsearchRestTemplate使用
创建索引
@Test
public void testIndex(){
boolean goods = template.indexOps(Goods.class).create();
boolean goods1 = template.createIndex(User.class);
System.out.println("goods = " + goods);
}
结果 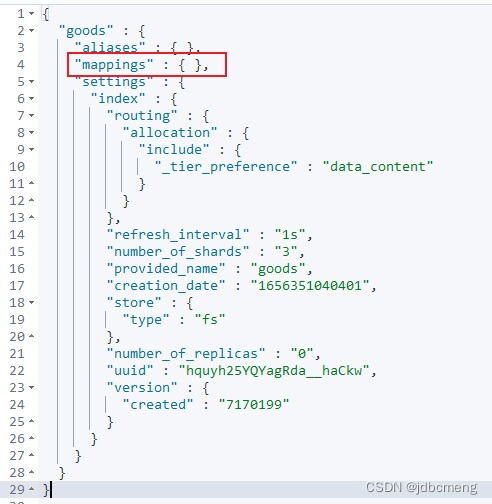 现在新版的API创建的索引没有mapping了,或者用其他的方法设置mapping,有一种引入外部json 的方式创建mapping,个人感觉挺好,适合项目中使用,方便查找和维护,可以参考这里es7创建索引
|