本文主要记录spring-boot-starter-data-jpa 的详细使用。 在做一些小型无并发的项目时,说实话第一个想到的就是Jpa,一个Entity走天下。
1.Spring Jpa的使用
基于spring-boot-starter-parent2.6.8
1.1 引入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
1.2 yaml配置
spring:
jpa:
hibernate:
ddl-auto: update
dialect: org.hibernate.dialect.MySQL8Dialect
open-in-view: false
show-sql: true
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://192.168.0.100:3306/a_channel?serverTimezone=Asia/Shanghai&characterEncoding=utf8&useSSL=false
username: root
password: 123456
hikari:
minimum-idle: 5
maximum-pool-size: 30
connection-timeout: 5000
idle-timeout: 60000
max-lifetime: 120000
validation-timeout: 60000
connection-test-query: SELECT 1
1.3 BaseEntity通用
@Getter
@Setter
@MappedSuperclass
@EntityListeners(AuditingEntityListener.class)
public abstract class BaseEntity extends SignVO implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
public Long id;
@CreatedDate
@Column(name = "create_time", updatable = false,
columnDefinition = "DATETIME(6) NULL DEFAULT CURRENT_TIMESTAMP(6) COMMENT '创建时间'")
@JSONField(format = "yyyy-MM-dd HH:mm:ss")
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
private Date createTime;
@LastModifiedDate
@Column(name = "update_time",
columnDefinition = "DATETIME(6) NULL DEFAULT CURRENT_TIMESTAMP(6) ON UPDATE CURRENT_TIMESTAMP(6) COMMENT '更新时间'")
@JSONField(format = "yyyy-MM-dd HH:mm:ss")
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
private Date updateTime;
@Override
public String toString() {
return JSONObject.toJSONString(this);
}
}
通用Entity要注意类上的注解,数据库自增策略要注意
1.4 entity类
@Entity
@Data
@Table(name = "t_agent",
uniqueConstraints = {
@UniqueConstraint(name = "channelCodetype", columnNames = {"channelCode", "type"})},
indexes = {@Index(columnList = "channelCode"), @Index(columnList = "name")})
public class Agent extends BaseEntity {
String name;
String channelCode;
Integer type;
@Column(unique = true)
String sign;
@Lob
String info;
}
1.5 Repository接口
import com.example.demo.entity.Agent;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.JpaSpecificationExecutor;
public interface AgentRepository extends JpaRepository<Agent, Long>, JpaSpecificationExecutor<Agent> {
Agent findByChannelCode(String key);
}
1.6 插入&&更新数据
jpa插入或者更新
@Resource
AgentRepository agentRepository;
Agent agent = new Agent();
agent.setName("test");
agent.setChannelCode("test1");
agent.setSign("1234xxx11123");
agent.setType(1);
agent.setInfo("info info");
agentRepository.save(agent);
更新与插入唯一的区别就是更新多了个主键id 的字段
1.7 查询数据
- 普通查询
Agent agent1 = agentRepository.findById(1l).orElse(null);
Agent agent1 = agentRepository.findByByChannelCode("test").orElse(null);
List<Agent> agents = agentRepository.findAll((root, query, cb) -> {
List<Predicate> predicates = new ArrayList<>();
predicates.add(cb.equal(root.get("type"), 2));
return cb.and(predicates.toArray(new Predicate[0]));
});
List<Agent> agents = agentRepository.findAll((root, query, cb) -> {
List<Predicate> predicates = new ArrayList<>();
predicates.add(cb.equal(root.get("type"), 2));
Expression<Integer> function = cb.function("FIND_IN_SET", Integer.class,
cb.literal(vo.ids()), root.get("appIds"));
predicates.add(cb.greaterThan(function, 0));
return cb.and(predicates.toArray(new Predicate[0]));
}, PageRequest.of(0,10, Sort.by(Sort.Direction.DESC,"id")));
需要注意字段一定要一一对应,分页和排序均可单独使用
1.8 Jpa注解使用
插入、更新、删除如果使用注解必须加@Transactional和@Modifying
@Transactional
@Modifying
@Query(value = "UPDATE t_agent set type=:#{#p.type} WHERE channel_code=:#{#p.channelCode}", nativeQuery = true)
void update(@Param("p") Agent agent);
@Transactional
@Modifying
@Query(value = "UPDATE agent set type=2 WHERE id in(:ids)", nativeQuery = true)
void updateAll(@Param("ids") List<Long> ids);
@Query(value = "SELECT * from t_agent where channelCode=?1 and type=?2 ", nativeQuery = true)
void select(String channelCode,Integer type);
mysql中的判空可以用if,list判空可以用coalesce(:#{#t.tagIds},null),与if的用法一致
2.Springboot集成Jpa和Mybatis
- Jpa的每次save都会先查询一遍,如果插入比较多的服务建议引入mybatis
- 真的不建议使用外键,虽然方便查询,但不管是查询还是操作都比较麻烦,Jpa还存在连接不能被回收的问题,Mybatis高端用法中有完美的替代
- 尽量不要使用注解sql,避免调试和取参困难
引入依赖
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.4.2</version>
</dependency>
于是便有了简单查询更新插入用Jpa,复杂查询用Mybatis,用起来简直方便多了
2.1 yaml配置
所有的orm框架都是基于应用层操作去封装的,而连接DataSource则是由不同的连接池实现,这里有的是默认的hikari连接池,如果想配置JPA和Mybatis只需要配置即可
spring:
jpa:
hibernate:
ddl-auto: update
dialect: org.hibernate.dialect.MySQL8Dialect
open-in-view: false
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://192.168.0.100:3306/d_file?serverTimezone=Asia/Shanghai&characterEncoding=utf8&useSSL=false
username: root
password: 123456
hikari:
minimum-idle: 5
maximum-pool-size: 30
connection-timeout: 5000
idle-timeout: 60000
max-lifetime: 120000
validation-timeout: 60000
connection-test-query: SELECT 1
mybatis:
mapperLocations: classpath:mapper/**.xml
executorType: SIMPLE
configuration:
map-underscore-to-camel-case: true
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
2.2 mapper接口记得添加@Mapper 注解
@Mapper
public interface FileMapper {}
2.3 mapper xml目录
要和配置对应,不然会报找不到boundsql 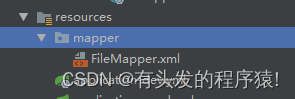
直接注解使用,相当于两个orm框架都共用连接池。只需要关心orm框架的配置,不用再去考虑连接池.
Jpa最实用的还是创建表与更新,性能上确实令人唏嘘
以上就是本章的全部内容了。
上一篇:SpringCloud第五话 – Gateway实现负载均衡、熔断、限流 下一篇:Jpa第二话 – Jpa多数据源集成
盛年不重来,一日难再晨
|