目录
IOC控制反转设计思路
Spring IoC思路整合Mybatis项目(例子)
文件结构:
配置依赖:?pom.xml
主要代码:
Spring核心容器(ApplicationContext):
ApplicationContext的主要实现类:
使用XML配置bean:
XML文件标签目录:
注解方式配置:
用于注解的三种方式:
注解所使用的标签:
用于创建对象
用于注入数据
用于其他设置
引入数据库后配置文件变化:
IOC控制反转设计思路
定义:是一种依赖倒置原则的设计思想,将代码里需要实现的对像创建,对象之间的依赖反转给容器去实现。
实现方式:通过xml文件,注解等方式,配置类与类之间的依赖关系,完成对像创建和依赖注入。实现IOC的主要设计模式是工厂模式。
优点:
集中管理,实现类的可配置和易管理。
降低类与类之间的耦合度
Spring IoC思路整合Mybatis项目(例子)
文件结构:
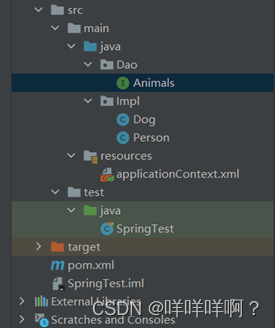
配置依赖:?pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SpringLearning</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.9.RELEASE</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.9.RELEASE</version>
<scope>compile</scope>
</dependency>
</dependencies>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
</project>
主要代码:
Spring整合的程序使用了SpringIOC思想,将所有的资源,都作为一个bean引入Spring容器中,代为创建对象。
Animals:
public interface Animals {
//两个实现类中的方法
String showAnimal();
void Hit();
}
Dog:
public class Dog implements Animals {
private String animals;
private Animals hit;
public String getAnimals() {
return animals;
}
public void setAnimals(String animals) {
this.animals = animals;
}
public Animals getHit() {
return hit;
}
public void setHit(Animals hit) {
this.hit = hit;
}
@Override
public String showAnimal() {
return null;
}
public String showName()
{
return animals;
}
@Override
public void Hit() {
System.out.println("Dog hit"+showName());
}
}
Person:
public class Person implements Animals {
private String animals;
private Animals hit;
public String getAnimals() {
return animals;
}
public void setAnimals(String animals) {
this.animals = animals;
}
public Animals getHit() {
return hit;
}
public void setHit(Animals hit) {
this.hit = hit;
}
@Override
public String showAnimal() {
return null;
}
public String showName()
{
return animals;
}
@Override
public void Hit() {
System.out.println("people hit"+showName());
}
}
applicationContext.xml:放在resource里面的Spring配置文件,里面放bean
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/beans ">
<bean id="Dog" class="Impl.Dog"></bean>//将Dog作为bean导入Spring中国
<bean id="Person" class="Impl.Person"></bean>
</beans>
SpringTest:测试文件:
public class SpringTest {
@Test
public void testAnimals()
{
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
Person per = app.getBean("Person",Person.class);
System.out.println(per);
}
}
Spring核心容器(ApplicationContext):
Spring为我们提供了两种核心容器,分别为BeanFactory和ApplicationContext.
ApplicationContext是BeanFactory接口的一个子接口,它也可以作为Spring的上下文,所谓Spring容器使用非常方便。
ApplicationContext除了提供BeanFactory的全部功能外,还增加如下功能。
1、ApplicationContext适用于单例对象,在构造核心容器时,立即加载并创建对象,而BeanFactory适用于多例对象,采取延迟加载方式,当要用到Id对象时才创建容器对象。
2、继承MessageSource接口,因此提供国际化支持,
3、资源访问,如访问URL和文件。
4、支持事件机制
ApplicationContext的主要实现类:
ClassPathXmlApplicationContext:配置文件放在类路径下,resource目录下
例如:
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
FileSystemXmlApplicationContxt:配置文件放在有限读取的任何地方(要先在文件夹中创建一个配置文件)
ApplicationContext file = new FileSystemXmlApplicationContext("D:\\ApplicationContext.xml");
AnnotationConfigApplicationContext:读取注解创建容器。(在src中创建一个配置类MyConfig)
如:
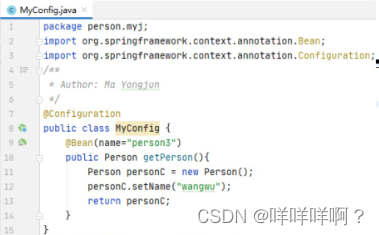
?再加载容器:
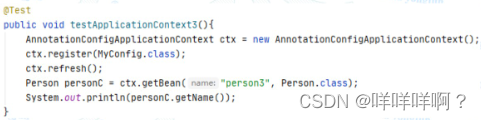
使用XML配置bean:
将Spring看作一个大工厂,Spring容器中的Bean对象就是该工厂的产品,而配置文件的作用就是生产和管理bean对象,配置方式:XML文件(或Properties文件),注解方式配置。
XML文件标签目录:
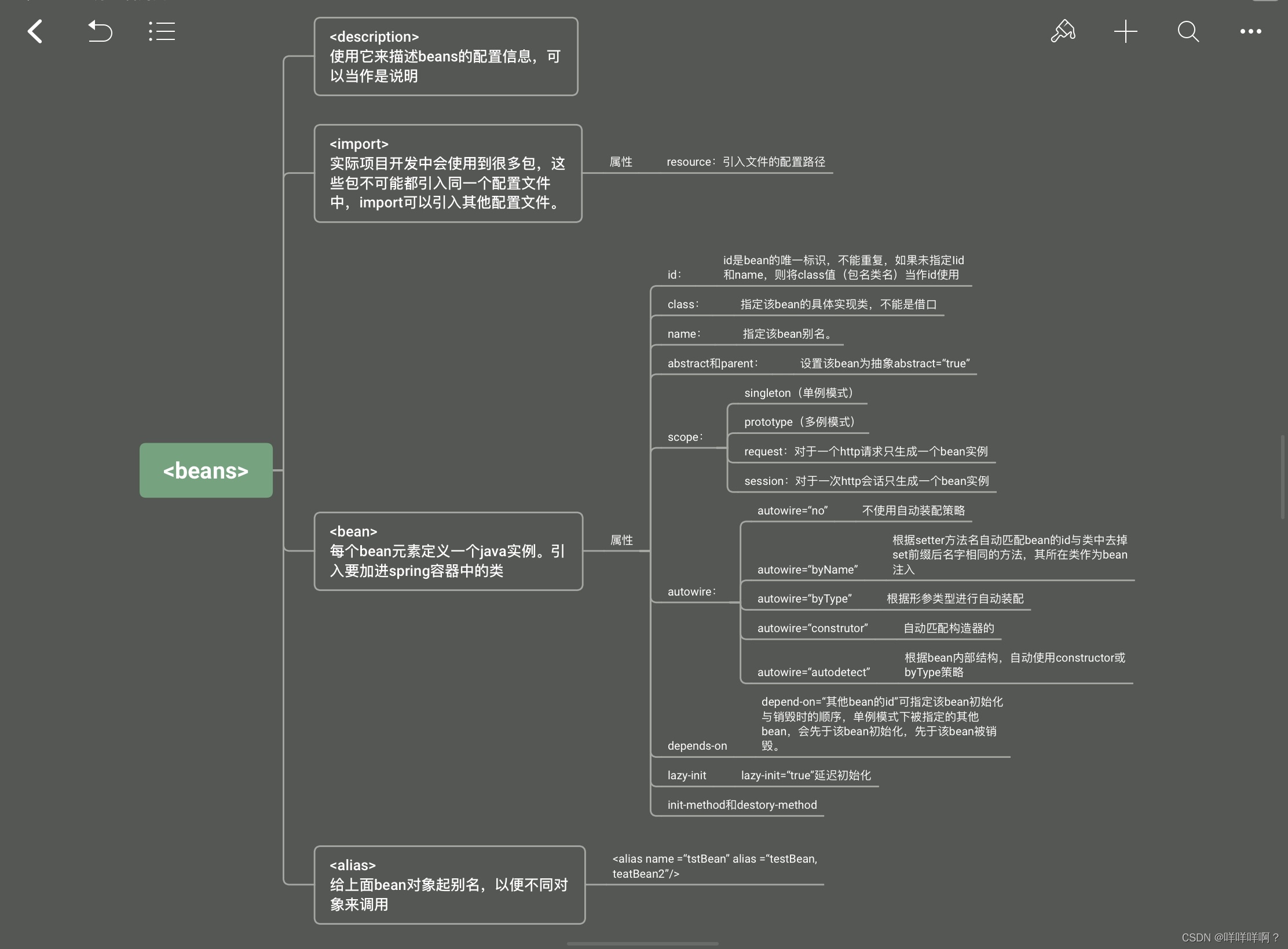
注解方式配置:
用于注解的三种方式:
注解所使用的标签:
? ? ? ? @Component(所有对象创建都可以用这个)
? ? ? ? @Controller:标注一个控制类,用于表现层
? ? ? ? @Service:标注一个业务类,用于业务层
? ? ? ? @Repository:标注一个DAO类,用在持久层接口
(下面三个与@Component作用与属性都一样,只是分三层更清晰)
? ? ? ? @Value:注入基本数据类型和字串
@value("zhangsan")
private String name;
????????@Resource:用于数据注入,默认自动按照名称注入,它等价于@Autowired+@Qualifier,其属性为name。
@Resource(name="steelAxe")
private Axe axe;
? ? ? ? @Autowired:自动按照类型注入
@Autowired//在引入的类上备注这个,它能自动去找继承了Axe的类中的st属性
private Axe st;
? ? ? ? @Qualifier:再按照类中的注入基础上再按照注解名称参数匹配
@Autowired
@Qualifier("stoneAxe")//如果下面的axe名字没有在相应类有相同的名字,则靠这个配置,其中的值就是要匹配的类中的属性名。
private Axe axe;
????????@Primary:在@Qualifier配置找不到时,才会去相应类中找被@Primary标注的类。标注在上
@Component
@Primary//这是@Qualifier 配置后没有用后需要配置的
public class StoneAxe implement Axe{}
? ? ? ? @Lookup:如果要在Bean A中插入Bean B,@lookup能在运行时通过getBean(String beanName)来获取最新的bean B。可用于将多例模式的类,插入到单例模式中。
@Component
@Scope("prototype")//表示多例模式
public class Prototypebean{}
@Component
public class SingletonBean{//单例模式
@lookup
public Prototypebean getPrototypebean(){return new Prototypebean();}
}
? ? ? ? @Scope:用于指定bean作用范围,取值singleton、prototype、request、session等
@Component
@Scope("singleton")//单例模式
public class person{}
? ? ? ? @DependsOn:设置依赖关系,指定后,会在将指定的类先初始化,在初始化当前类
@Component
@DependsOn("dog")//会在dog类初始化后,再初始化person类
public class person{}
? ? ? ? @Lazy:延迟初始化,@Lazy(true),设定后指定当Spring容器初始化时,不会预初始化当前类
? ? ? ? @PostConstruct:指定初始化方法
? ? ? ? @PreDestory:指定销毁方法
@Component
public class Car{
@PostConstruct
public void init() {System.out,println("执行初始化方法init");}
@PreDestroy
public void close() {System.out,println("执行销毁前方法close");}
}
引入数据库后配置文件变化:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd">
<!-- 其中一种引入datasource写法 -->
<!-- 注解包扫描 -->
<context:component-scan base-package="com.mojingyi.service">
</context:component-scan>
<!-- 引入DB配置文件 -->
<context:property-placeholder location="classpath:jdbc.properties"/>
<!-- 配置数据源 -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="${jdbc.driver}"></property>
<property name="url" value="${jdbc.url}"></property>
<property name="username" value="${jdbc.name}"></property>
<property name="password" value="${jdbc.password}"></property>
</bean>
<!-- 创建SqlSessionFactory -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
<property name="mapperLocations" value="classpath:com/mojingyi/dao/*DaoImpl.xml"></property>
</bean>
<!-- 扫描Mapper文件并生成dao对象 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"></property>
<property name="basePackage" value="com.mojingyi.dao"></property>
</bean>
<!-- 配置事务管理器 -->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"></property>
</bean>
<!-- 事务管理器注解 -->
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
|