SpringCloud快速学习(4)——Hystrix
个人的动力节点视频学习笔记 视频地址:https://www.bilibili.com/video/BV1f94y1U7AB
介绍
这个Hystrix使用起来挺像异常处理的。
? Hystrix,熔断器,也叫断路器!(正常情况下 断路器是关的 只有出了问题才打开)用来保护微服务不雪崩的方法。思想和我们上面画的拦截器一样。 Hystrix 是 Netflix 公司开源的一个项目,它提供了熔断器功能,能够阻止分布式系统中出现 联动故障。Hystrix 是通过隔离服务的问点阻止联动故障的,并提供了故障的解决方案,从 而提高了整个分布式系统的弹性。
?
服务雪崩
基本介绍
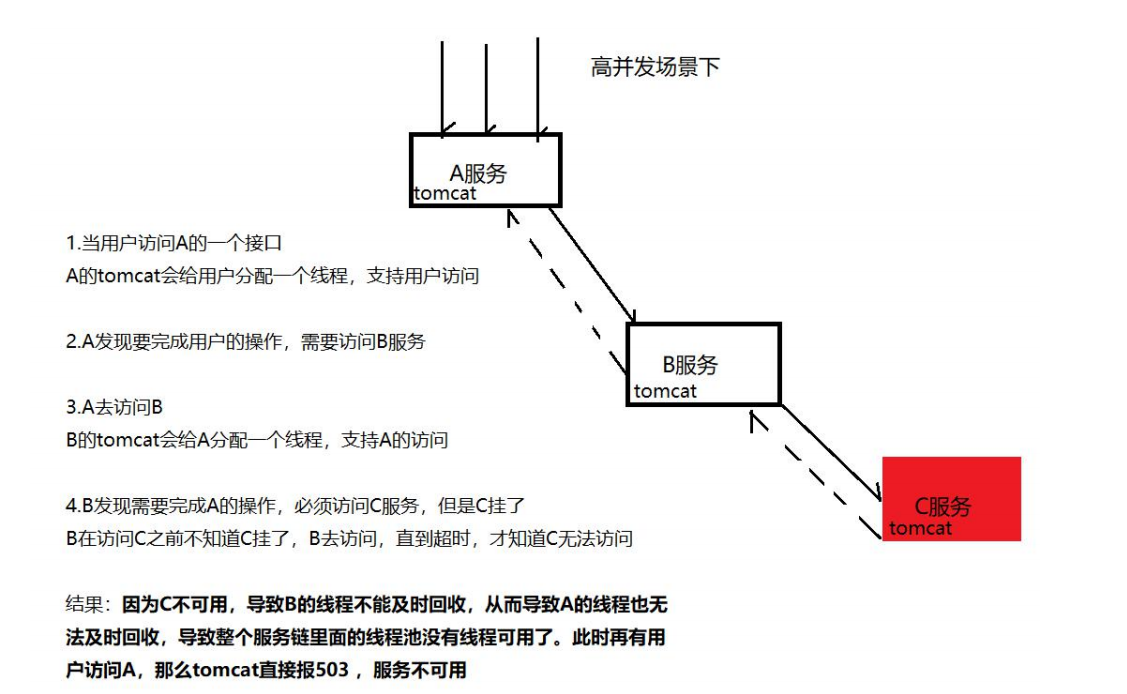
服务雪崩的本质:线程没有及时回收。
不管是调用成功还是失败,只要线程可以及时回收,就可以解决服务雪崩
怎么解决
将服务间的调用超时时长改小,这样就可以让线程及时回收,保证服务可用
优点:非常简单,也可以有效的解决服务雪崩
缺点:不够灵活,有的服务需要更长的时间去处理(写库,整理数据)
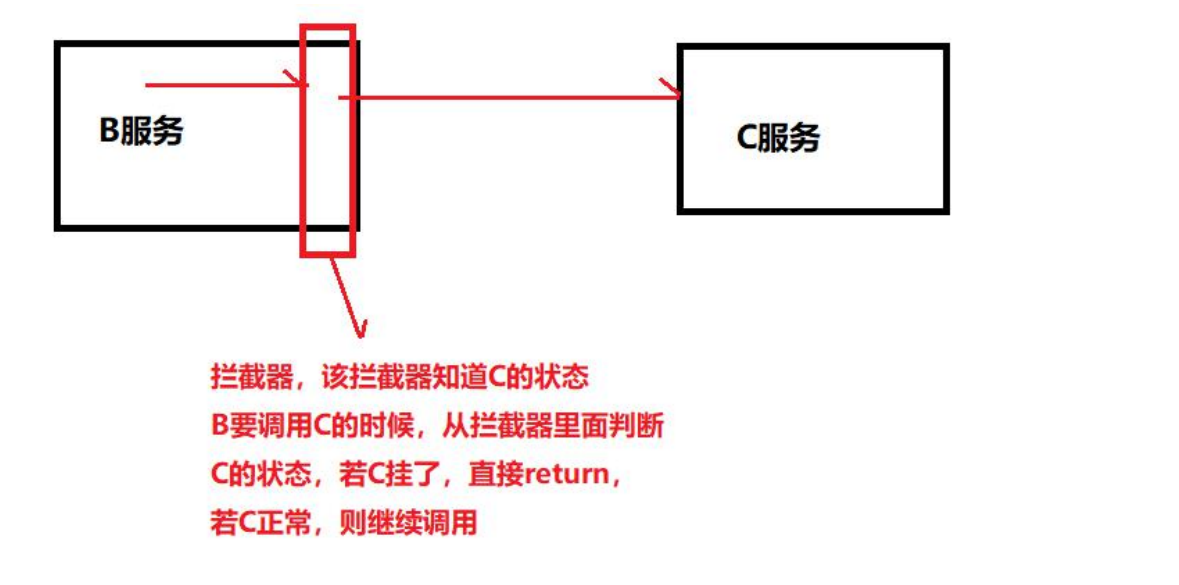
快速入门
基本结构
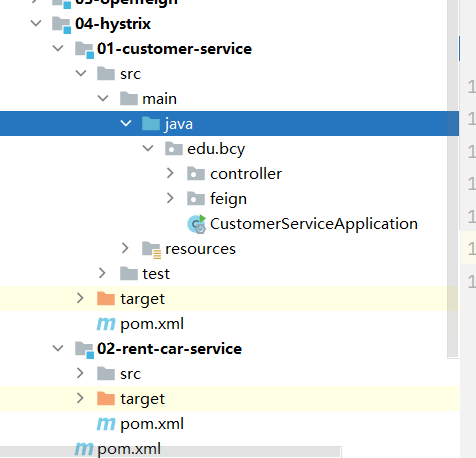
02-rent-car-service 生产者
pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>04-hystrix</artifactId>
<groupId>edu.bcy</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>02-rent-car-service</artifactId>
<properties>
<java.version>1.8</java.version>
<spring-cloud.version>Hoxton.SR12</spring-cloud.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
yml
server:
port: 8080
spring:
application:
name: rent-car-service
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka
instance:
hostname: localhost
instance-id: ${eureka.instance.hostname}:${spring.application.name}:${server.port}
RentCarController 对外Controller
@RestController
public class RentCarController {
@GetMapping("rent")
public String rent() {
return "租车成功";
}
}
01-customer-service
业务接口
@FeignClient(value = "rent-car-service",fallback = CustomerRentFeignHystrix.class)
public interface CustomerRentFeign {
@GetMapping("rent")
public String rent();
}
业务实现
@Component
public class CustomerRentFeignHystrix implements CustomerRentFeign {
@Override
public String rent() {
return "我是备胎";
}
}
CustomerController 对外Controller
import edu.bcy.feign.CustomerRentFeign;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class CustomerController {
@Qualifier("edu.bcy.feign.CustomerRentFeign")
@Autowired
private CustomerRentFeign customerRentFeign;
@GetMapping("customerRent")
public String CustomerRent(){
System.out.println("客户来租车了");
String rent = customerRentFeign.rent();
return rent;
}
}
结果
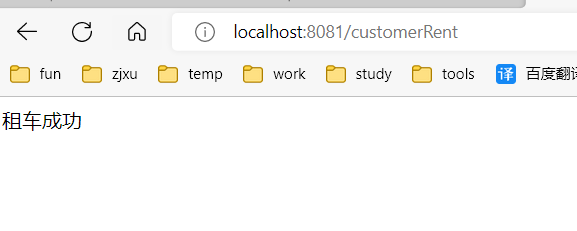
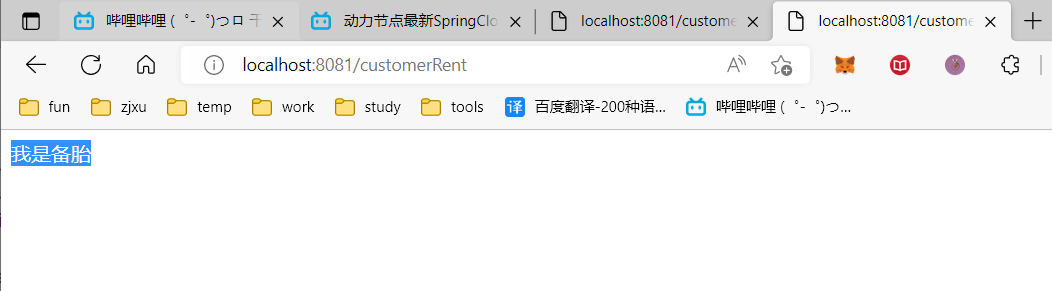
Hystrix模拟
@Component
@Aspect
public class FishAspect {
public static Map<String, Fish> fishMap = new HashMap<>();
static {
fishMap.put("order-service", new Fish());
}
Random random = new Random();
@Around(value = "@annotation(com.powernode.anno.MyFish)")
public Object fishAround(ProceedingJoinPoint joinPoint) {
Object result = null;
Fish fish = fishMap.get("order-service");
FishStatus status = fish.getStatus();
switch (status) {
case CLOSE:
try {
result = joinPoint.proceed();
return result;
} catch (Throwable throwable) {
fish.addFailCount();
return "我是备胎";
}
case OPEN:
return "我是备胎";
case HALF_OPEN:
int i = random.nextInt(5);
System.out.println(i);
if (i == 1) {
try {
result = joinPoint.proceed();
fish.setStatus(FishStatus.CLOSE);
synchronized (fish.getLock()) {
fish.getLock().notifyAll();
}
return result;
} catch (Throwable throwable) {
return "我是备胎";
}
}
default:
return "我是备胎";
}
}
}
常见架构分析
单体项目架构
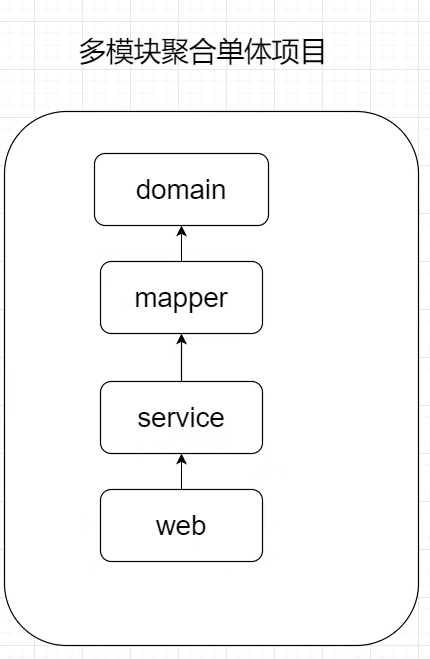
常见微服务架构
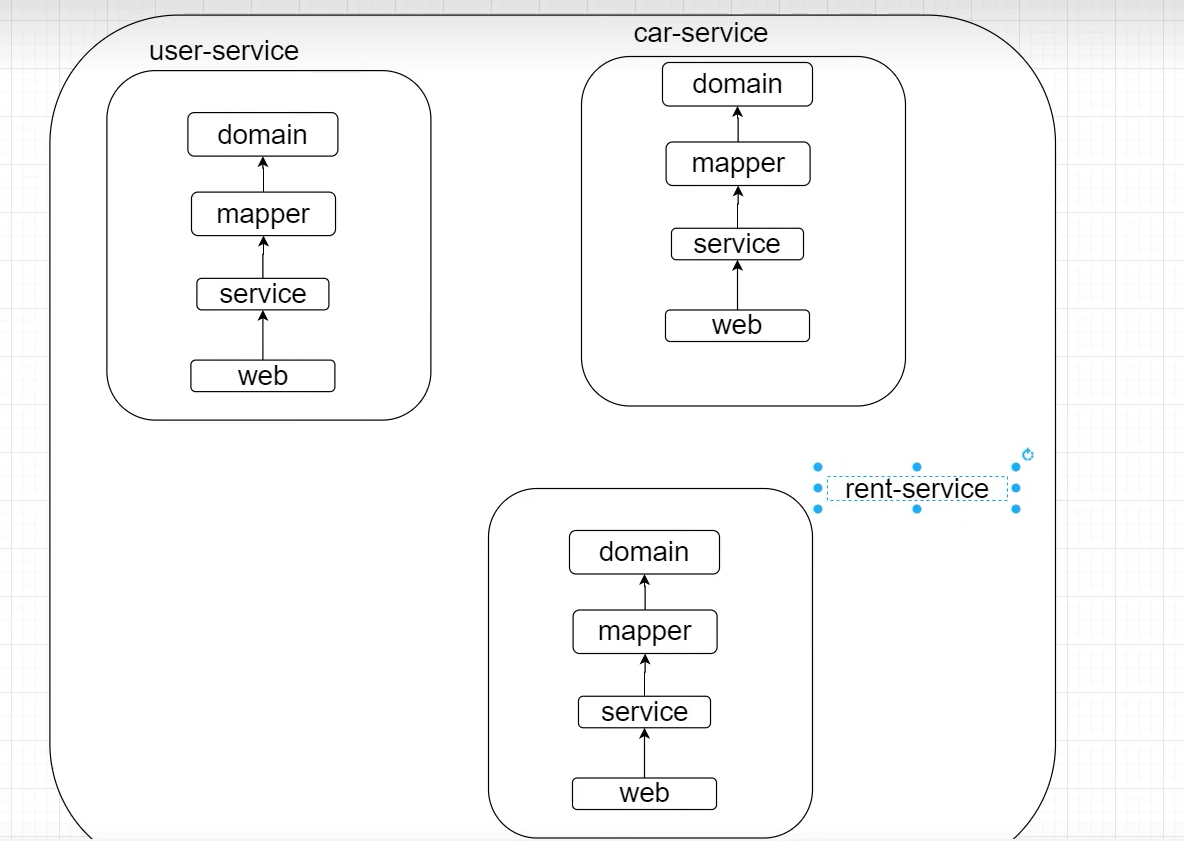
微服务模块统合架构
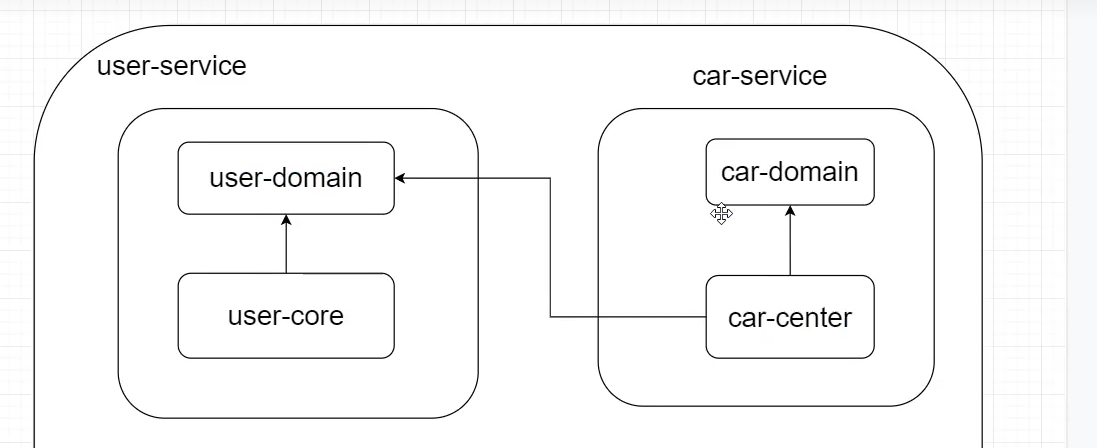
公共提取式架构
Feign项目架构
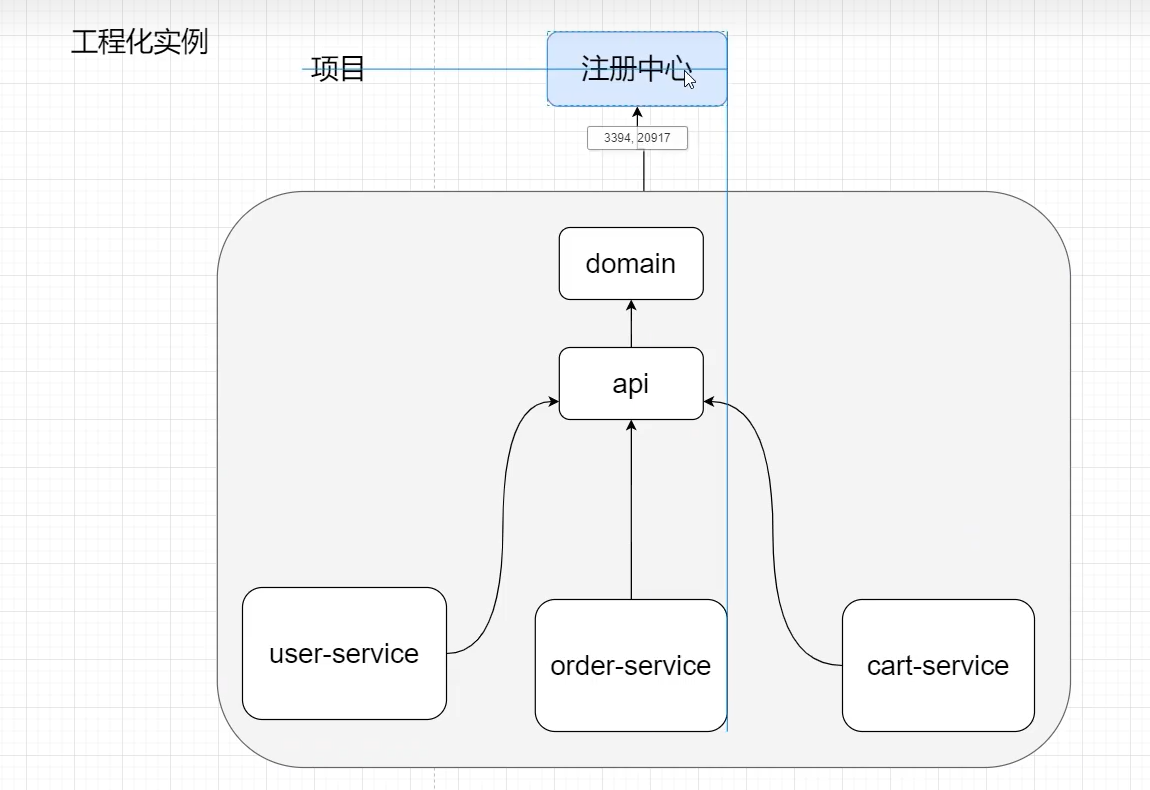
|