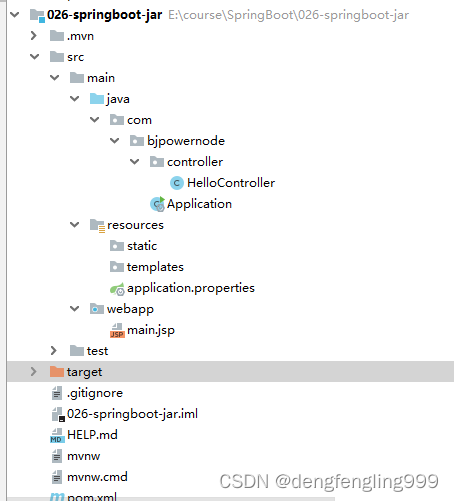
pom.xml:加入依赖,插件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.1</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.bjpowernode</groupId>
<artifactId>026-springboot-jar</artifactId>
<version>0.0.1-SNAPSHOT</version>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!--tomcat处理jsp的依赖-->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<!--resources插件,把jsp编译到META-INF/resources-->
<resources>
<resource>
<directory>src/main/webapp</directory>
<targetPath>META-INF/resources</targetPath>
<includes>
<include>**/*.*</include>
</includes>
</resource>
<!--如果使用了mybatis,而且mapper文件放在src/main/java目录下-->
<resource>
<directory>src/main/java</directory>
<!--这里没有写targetPath默认编译到Class目录下-->
<includes>
<include>**/*.xml</include>
</includes>
</resource>
<!--把src/main/resources下面所有的文件,都包含到class目录-->
<resource>
<directory>src\main\resources</directory>
<includes>
<include>**/*.*</include>
</includes>
</resource>
</resources>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
springboot配置文件:application.properties:
#设置端口号、上下文访问路径
server.port=9002
server.servlet.context-path=/myboot
#设置视图解析器
spring.mvc.view.prefix=/
spring.mvc.view.suffix=.jsp
创建mian.jsp:
<%--
Created by IntelliJ IDEA.
User: DELL
Date: 2022/7/16
Time: 12:25
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://" + request.getServerName() + ":" + request.getServerPort() + path + "/";
%>
<html>
<head>
<title>Title</title>
<base href="<%=basePath%>">
</head>
<body>
main.jsp用来显示数据 ${data}
</body>
</html>
创建控制器:HelloController:
package com.bjpowernode.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class HelloController {
@RequestMapping("/hello")
public ModelAndView hello(){
ModelAndView mv =new ModelAndView();
mv.addObject("data","SpringBoot打jar包");
mv.setViewName("main");//转发到main.jsp
return mv;
}
}
主启动类:Application:启动项目
package com.bjpowernode;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
测试运行:
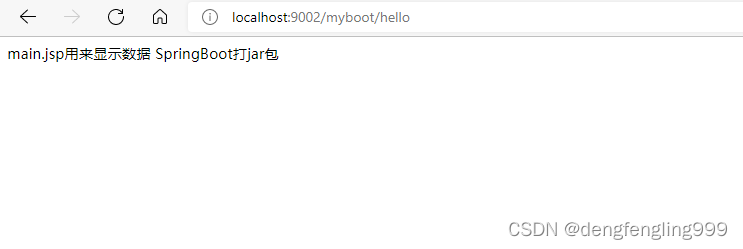
打jar包:
需要在pom.xml中指定打包名称,和Maven插件:maven-plugin的版本号
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.1</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.bjpowernode</groupId>
<artifactId>026-springboot-jar</artifactId>
<version>0.0.1-SNAPSHOT</version>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!--tomcat处理jsp的依赖-->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<!--指定打包名称-->
<finalName>myboot</finalName>
<!--resources插件,把jsp编译到META-INF/resources-->
<resources>
<resource>
<directory>src/main/webapp</directory>
<targetPath>META-INF/resources</targetPath>
<includes>
<include>**/*.*</include>
</includes>
</resource>
<!--如果使用了mybatis,而且mapper文件放在src/main/java目录下-->
<resource>
<directory>src/main/java</directory>
<!--这里没有写targetPath默认编译到Class目录下-->
<includes>
<include>**/*.xml</include>
</includes>
</resource>
<!--把src/main/resources下面所有的文件,都包含到class目录-->
<resource>
<directory>src\main\resources</directory>
<includes>
<include>**/*.*</include>
</includes>
</resource>
</resources>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<!--打包jar包,有jsp文件时,必须指定maven-plugin插件的版本是1.4.2.RELEASE-->
<version>1.4.2.RELEASE</version>
</plugin>
</plugins>
</build>
</project>
先clean,在package:?
?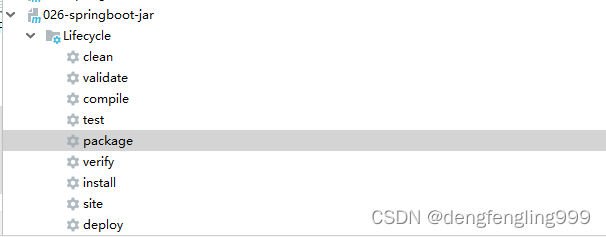
?打包成功:
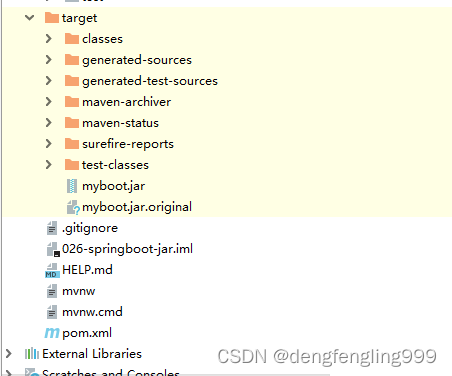
打开jar包所在的目录,输入cmd,以命令行的形式运行springboot的应用:
?输入命令
?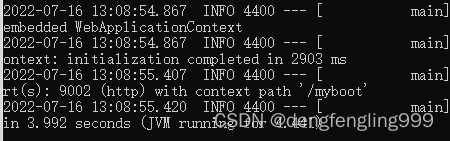
可以看到端口号是9002 ,地址myboot:在浏览器运行
?
?
?可以写一个脚本在Linux服务器上运行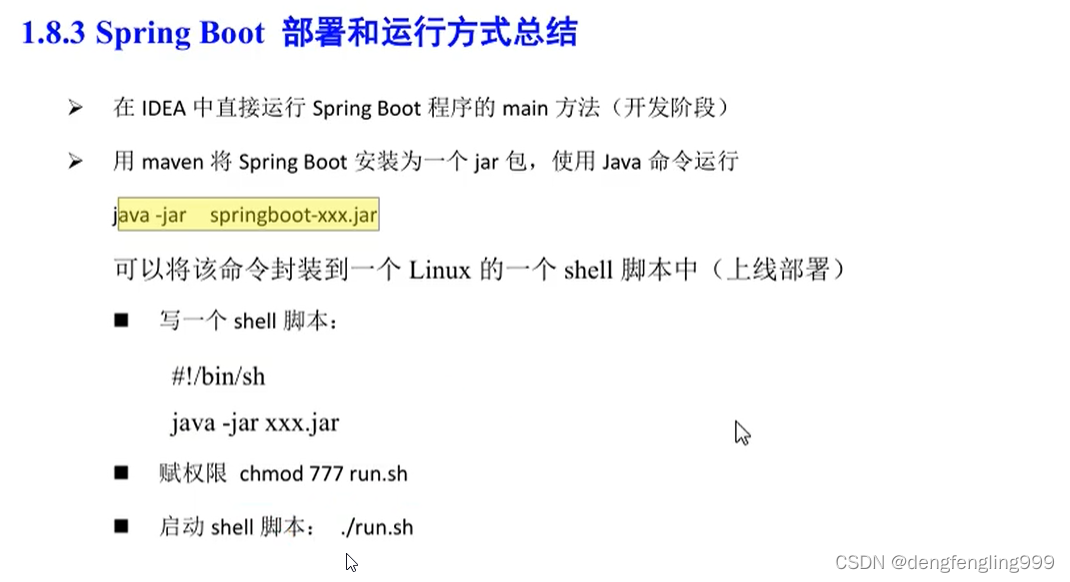
?
?
总结:
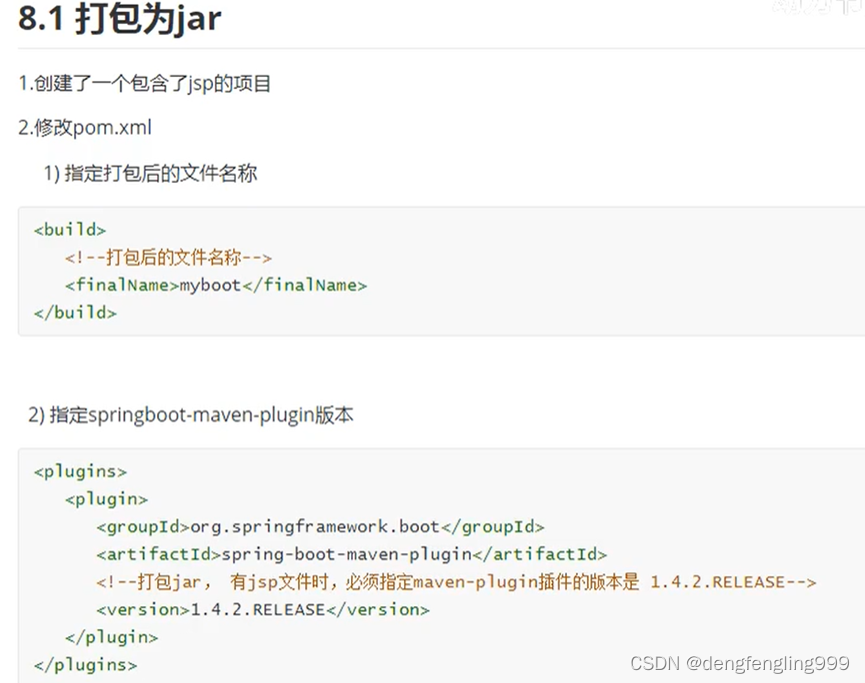
?
?
?
?
?
war和jar区别:
打包成war包:
需要一个服务器,有服务器才能执行,服务器启动的时候需要占用资源,而且还占用端口号,项目是放到服务器里面取的,这种方式是服务器占用比较多,启动tomcat,再启动应用才行,好处是能够充分应用这个服务器的能力,他们是独立的?,能利用tomcat做一些请求和操作
打包成jar包:
比较小巧一点,不依赖服务器,用起来比较简单和方便,不需要做过多的配置,jar包里面有内嵌的tomcat,但是内置的比不上独立的服务器,在某些功能上呢比独立的服务器要弱
|