一、搭建IDEA的Spring Boot的开发环境
在IDEA中进行 Spring Initializr 创建。 软件包命名为:com.example.restservice
参考链接:IDEA创建一个Spring Boot入门项目
二、构建 RESTful Web 服务
2.1 在文件夹中创建 src/main/java/com/example/restservice/Greeting.java
package com.example.restservice;
public class Greeting {
private final long id;
private final String content;
public Greeting(long id, String content) {
this.id = id;
this.content = content;
}
public long getId() {
return id;
}
public String getContent() {
return content;
}
}
2.2 创建资源控制器: 在 Spring boot 中创建 Restful Web 服务,使用控制器来处理 HTTP 请求, 需要用到 @RestController 注解。
在文件夹中创建 src/main/java/com/example/restservice/GreetingController.java
package com.example.restservice;
import java.util.concurrent.atomic.AtomicLong;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GreetingController {
private static final String template = "Hello, %s!";
private final AtomicLong counter = new AtomicLong();
@GetMapping("/greeting")
public Greeting greeting(@RequestParam(value = "name", defaultValue = "World") String name) {
return new Greeting(counter.incrementAndGet(), String.format(template, name));
}
}
@RestController (1)带有此注释的类型被视为控制器。
@GetMapping (1)@GetMapping 注释确保 HTTP GET 请求 /greeting 映射到 greeting()方法。 (2)即,确保访问 /greeting 的 HTTP GET请求 由 greeting() 函数处理。
有其他 HTTP 动词的伴随注释(例如 @PostMappingPOST )。还有一个 @RequestMapping 它们都源自的注释,并且可以用作同义词(例如 @RequestMapping(method=GET) )。
@RequestParam (1)用于接收 HTTP GET请求 传过来的 name 值,默认值为 “World”。
2.3 IDEA中项目目录结构 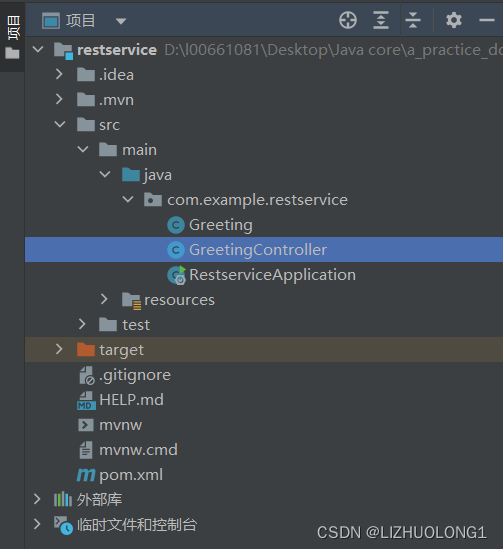
三、测试服务
3.1 启动服务; 3.2 访问URL: (1)http://localhost:8080/greeting
{"id":1,"content":"Hello, World!"}
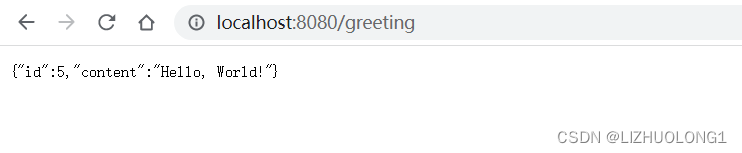 (2)http://localhost:8080/greeting?name=User
{"id":3,"content":"Hello, User!"}

它将以 JSON 形式进行响应。
官方教程链接:https://spring.io/guides/gs/rest-service/
四、附加
端口我们也可以自己指定,放到应用配置文件 application.properties 中即可。
# 默认的 8080 修改为 9090
server.port=9090
|