SpringBoot整合Junit
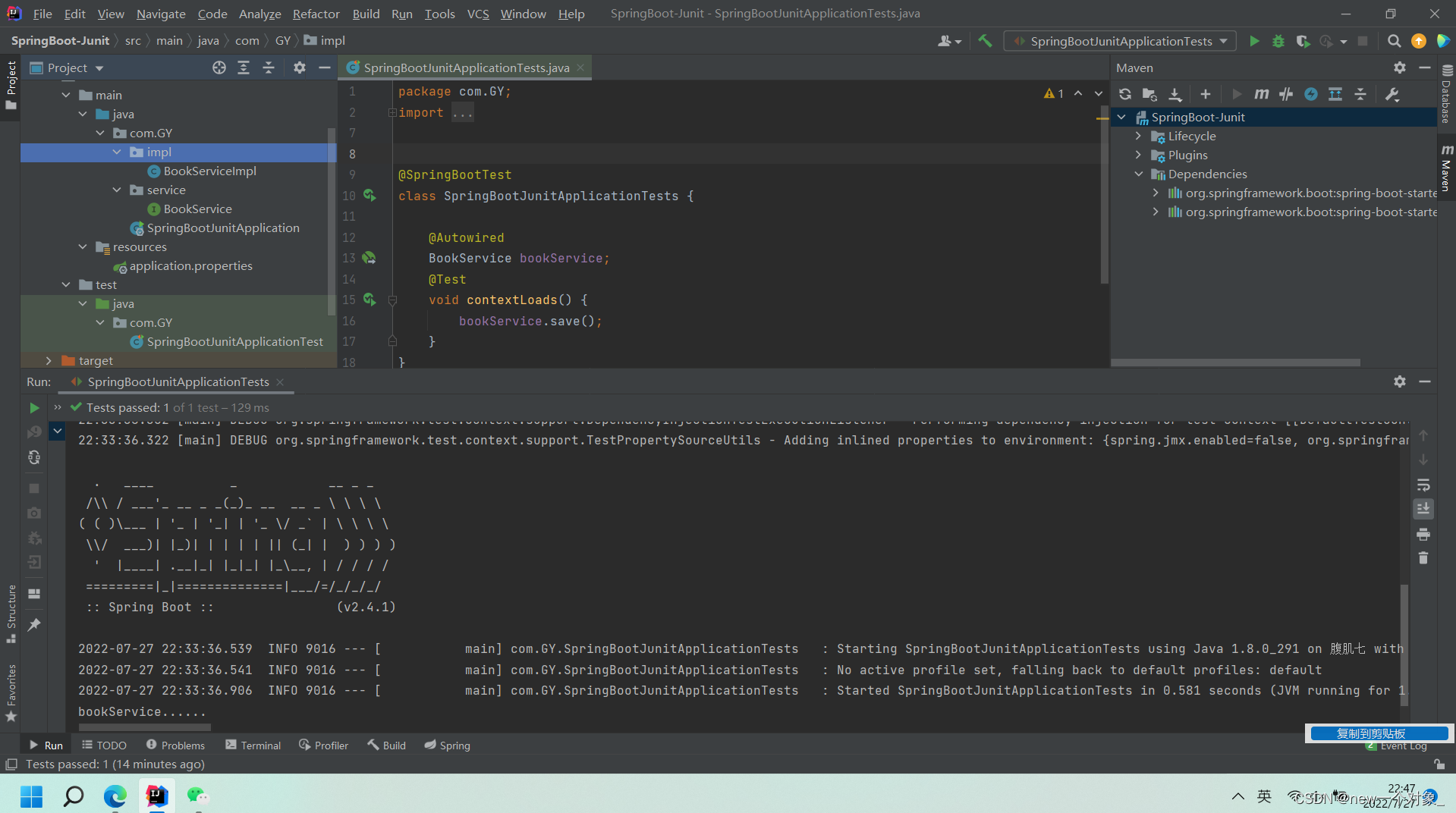
SpringBoot整合MyBatis
? 新建模块,选择Spring初始化,并配置模块相关基础信息 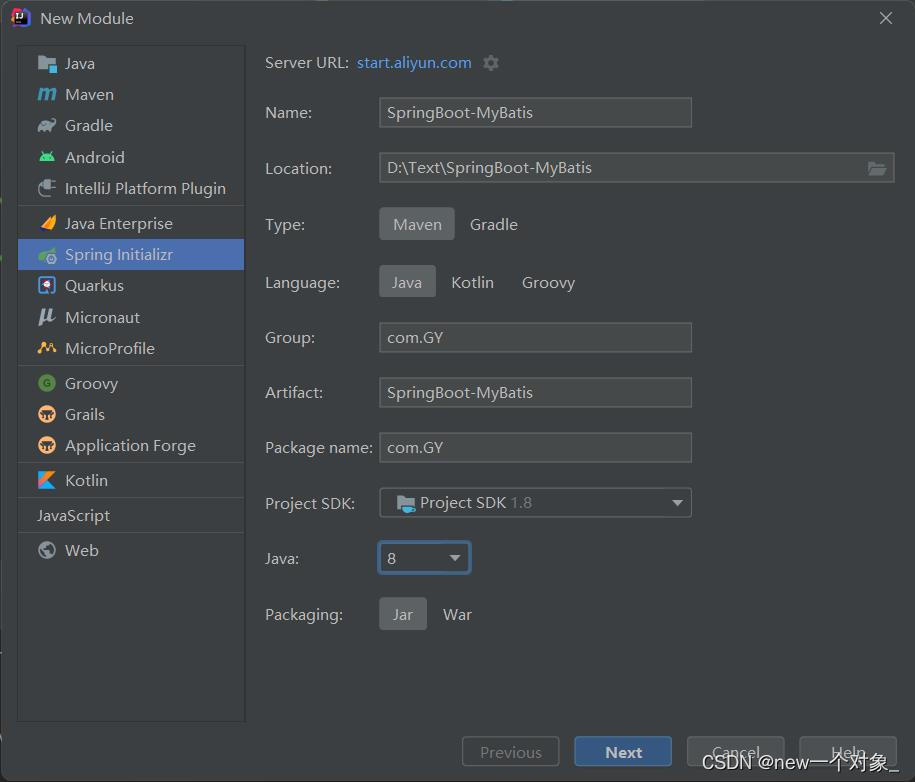
? 选择当前模块需要使用的技术集(MyBatis、MySQL) 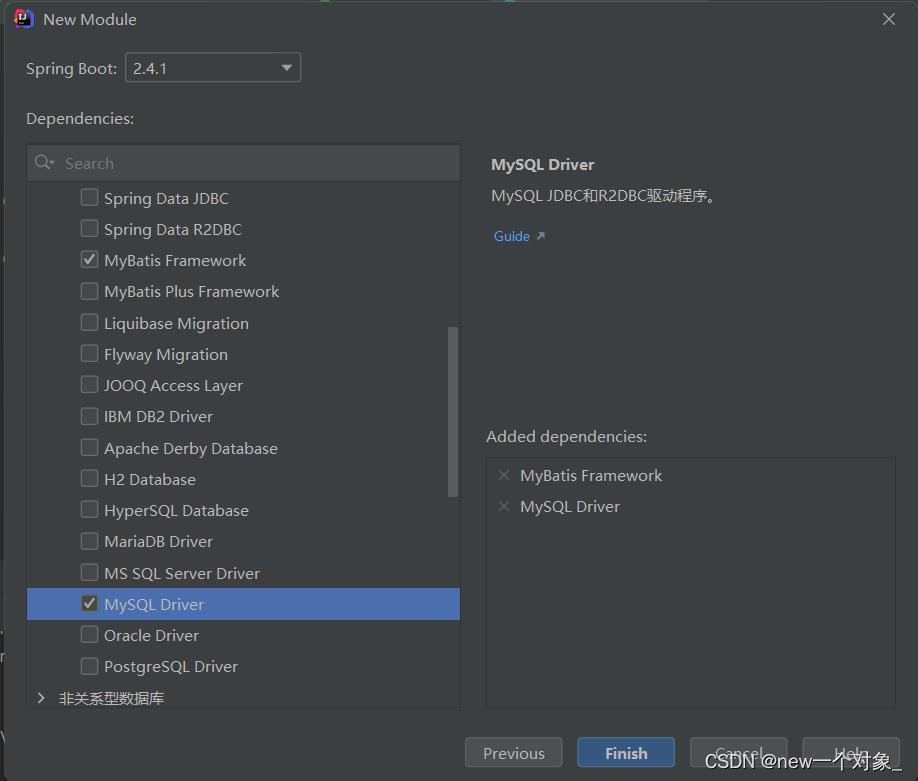
? 创建代理类(数据层接口)
package com.GY.dao;
import com.GY.domain.User;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
@Mapper
public interface UserDao {
@Select("select * from user where id=#{id}")
public User getID(Integer id);
}
? 设置数据源参数
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://127.0.0.1:3306/test?serverTimezone=UTC
username: root
password: 123456
此时我们测试dao接口(发现有大量报错信息) 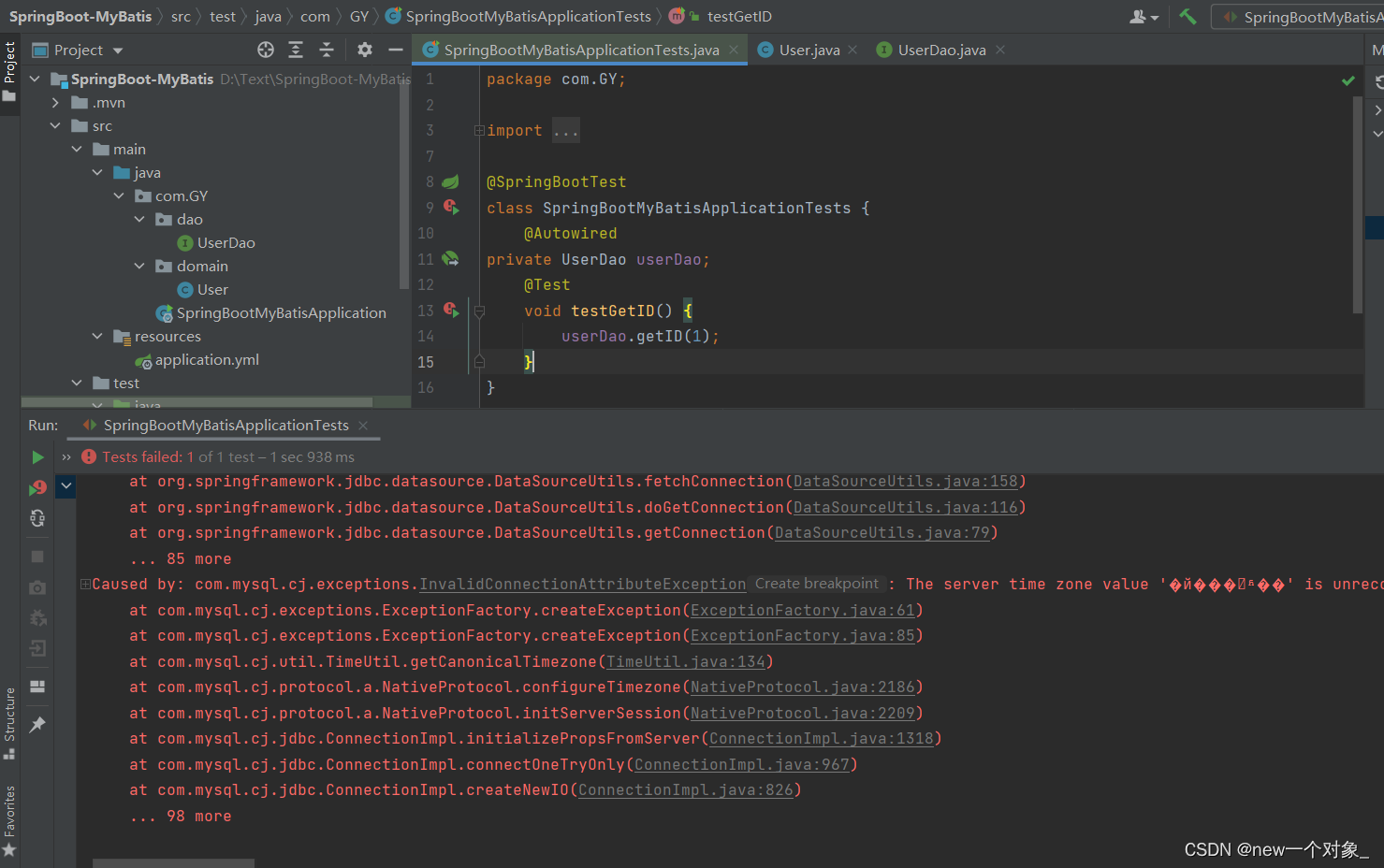
报错信息大致如下 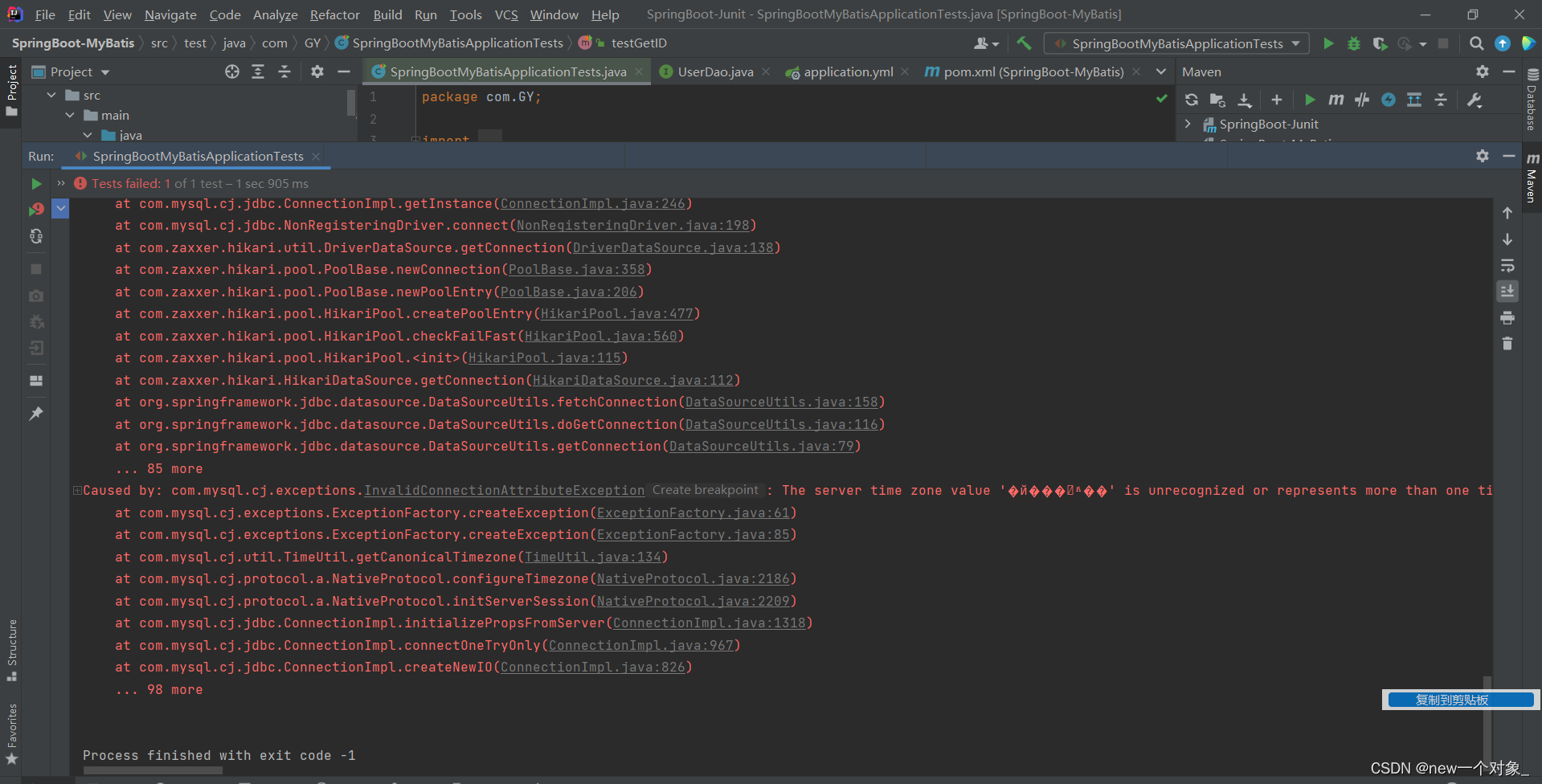
查阅后发现我们需要设置下时区,这里不是 springboot + mybatis 的问题,而是为了使MySQL JDBC驱动的版本与UTC时区配合使用( 全球标准时间),必须在连接字符串中明确指定serverTimezone (指定时区) UTC代表的是全球标准时间 ,但是我们使用的时间是北京时区也就是东八区,领先UTC八个小时。
serverTimezone=GMT%2B8
serverTimezone=Asia/Shangha
🚸🚸注意事项:SpringBoot版本低于2.4.3(不含),Mysql 驱动版本大于8.0时,需要在url连接串中配置时区 url: jdbc:mysql://127.0.0.1:3306/test?serverTimezone=UTC
? 修改application.yml中的配置信息 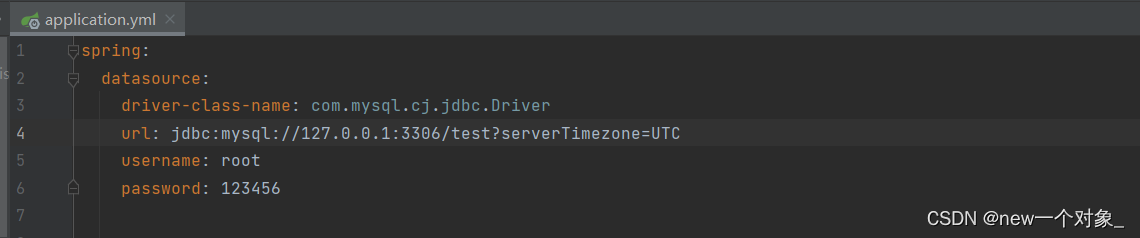
? 查询成功 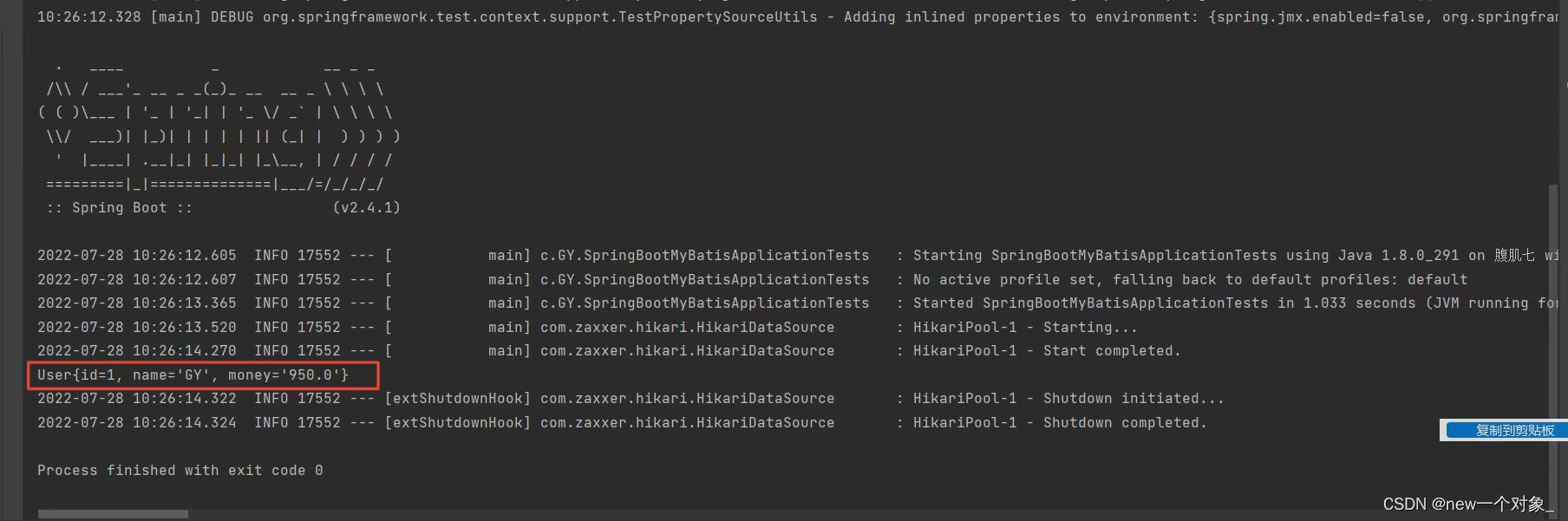
基于SpringBoot实现ssm整合 ? 选择当前模块需要使用的技术集(Spring Web、MyBatis、MySQL) 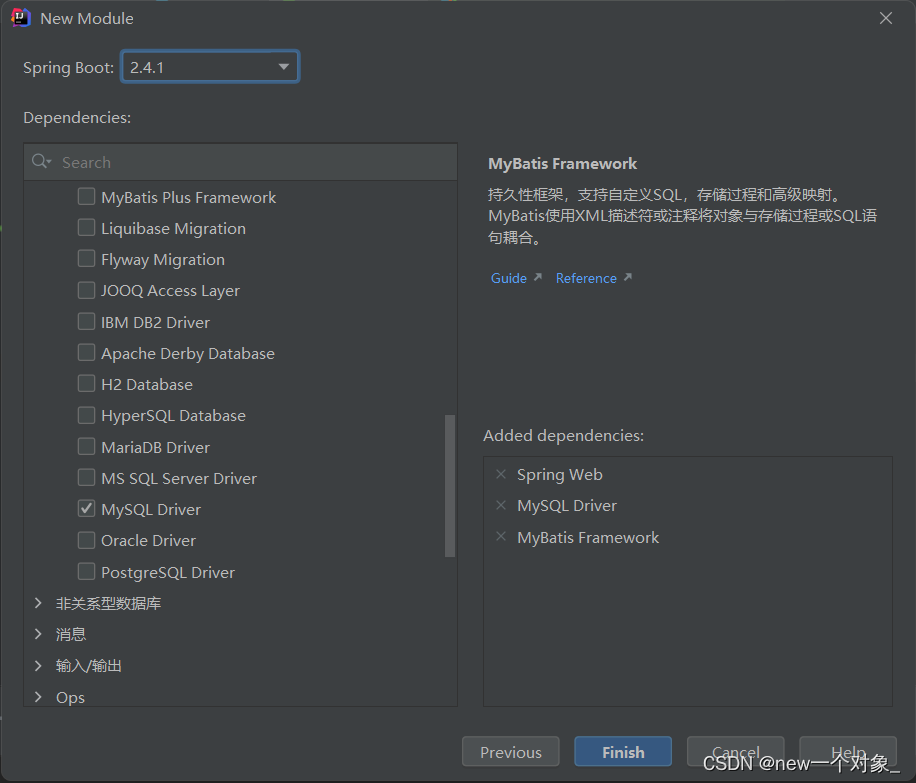
包层次结构 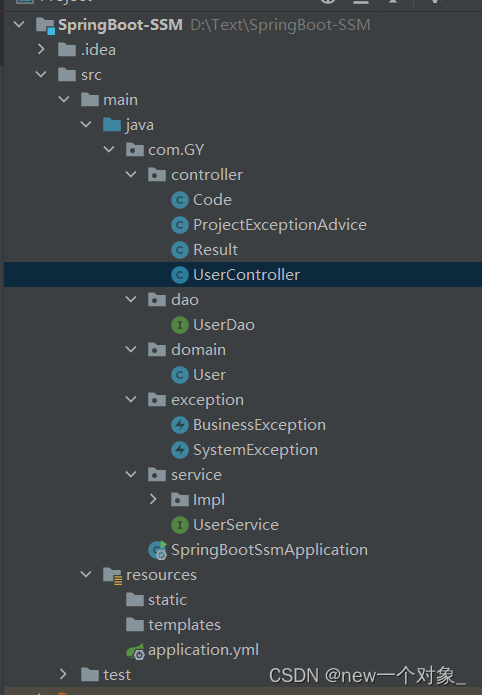
基于REST风格的控制器类
package com.GY.controller;
import com.GY.domain.User;
import com.GY.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@PostMapping
public Result save(@RequestBody User user){
boolean flag=userService.save(user);
return new Result(flag?Code.SAVE_OK:Code.SAVE_ERR,flag) ;
}
@DeleteMapping("/{id}")
public Result delete(@PathVariable Integer id){
boolean flag=userService.delete(id);
return new Result(flag?Code.DELETE_OK:Code.DELETE_ERR,flag) ;
}
@PutMapping
public Result update(@RequestBody User user){
boolean flag= userService.update(user);
return new Result(flag?Code.UPDATE_OK:Code.UPDATE_ERR,flag) ;
}
@GetMapping("/{id}")
public Result getById(@PathVariable Integer id){
User user=userService.getById(id);
System.out.println(user);
Integer code=user!=null?Code.GET_OK:Code.GET_ERR;
String msg=user!=null?"":"数据查询失败,请重试";
return new Result(code,user,msg);
}
@GetMapping
public Result getAll(){
List<User> list=userService.getAll();
Integer code=list!=null?Code.GET_OK:Code.GET_ERR;
String msg=list!=null?"":"数据查询失败,请重试";
return new Result(code,list,msg);
}
}
码值类 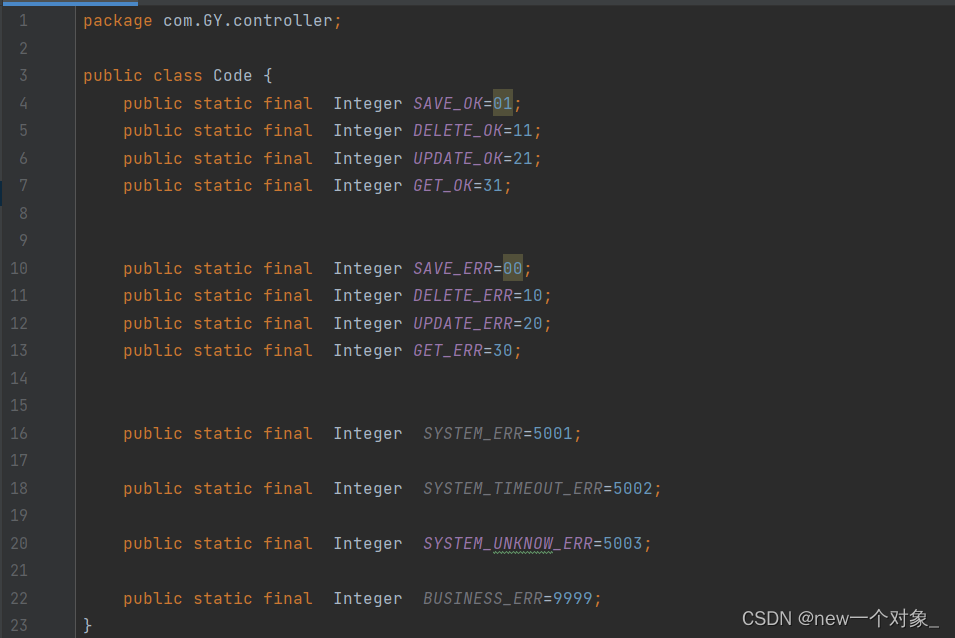
基于REST风格的控制器类
package com.GY.controller;
import com.GY.domain.User;
import com.GY.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@PostMapping
public Result save(@RequestBody User user){
boolean flag=userService.save(user);
return new Result(flag?Code.SAVE_OK:Code.SAVE_ERR,flag) ;
}
@DeleteMapping("/{id}")
public Result delete(@PathVariable Integer id){
boolean flag=userService.delete(id);
return new Result(flag?Code.DELETE_OK:Code.DELETE_ERR,flag) ;
}
@PutMapping
public Result update(@RequestBody User user){
boolean flag= userService.update(user);
return new Result(flag?Code.UPDATE_OK:Code.UPDATE_ERR,flag) ;
}
@GetMapping("/{id}")
public Result getById(@PathVariable Integer id){
User user=userService.getById(id);
System.out.println(user);
Integer code=user!=null?Code.GET_OK:Code.GET_ERR;
String msg=user!=null?"":"数据查询失败,请重试";
return new Result(code,user,msg);
}
@GetMapping
public Result getAll(){
List<User> list=userService.getAll();
Integer code=list!=null?Code.GET_OK:Code.GET_ERR;
String msg=list!=null?"":"数据查询失败,请重试";
return new Result(code,list,msg);
}
}
系统异常
package com.GY.exception;
public class SystemException extends RuntimeException{
private Integer code;
public Integer getCode() {
return code;
}
public SystemException(Integer code, String message) {
super(message);
this.code = code;
}
public SystemException(Integer code,String message, Throwable cause) {
super(message, cause);
this.code = code;
}
}
业务异常
package com.GY.exception;
public class BusinessException extends RuntimeException{
private Integer code;
public Integer getCode() {
return code;
}
public BusinessException(Integer code, String message) {
super(message);
this.code = code;
}
public BusinessException(Integer code,String message, Throwable cause) {
super(message, cause);
this.code = code;
}
}
异常处理类
package com.GY.controller;
import com.GY.exception.BusinessException;
import com.GY.exception.SystemException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class ProjectExceptionAdvice {
@ExceptionHandler(SystemException.class)
public Result doSystemException(SystemException e){
return new Result(e.getCode(),null,e.getMessage());
}
@ExceptionHandler(BusinessException.class)
public Result doBusinessException(BusinessException e){
return new Result(e.getCode(),null,e.getMessage());
}
@ExceptionHandler(Exception.class)
public Result doException(Exception e){
return new Result(Code.SYSTEM_UNKNOW_ERR,null,"系统繁忙,请稍后重试");
}
}
返回结果集封装 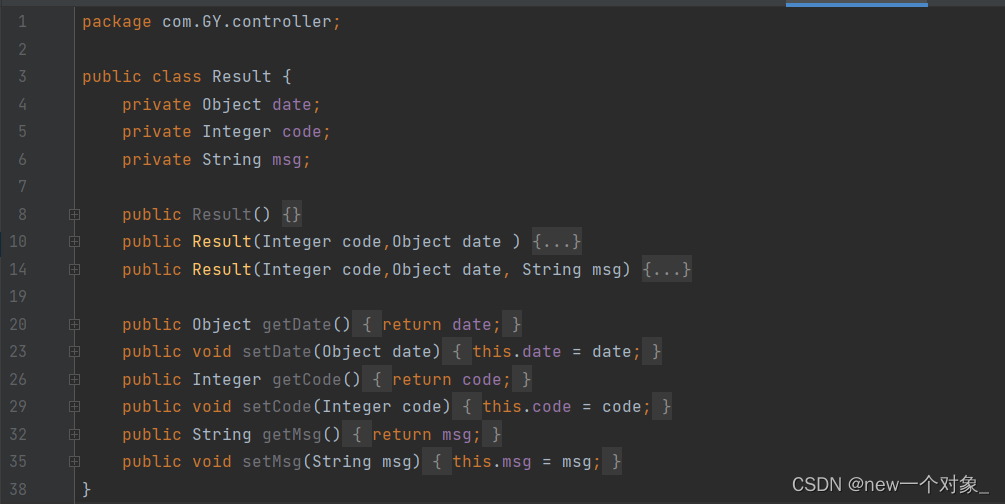
发送请求测试 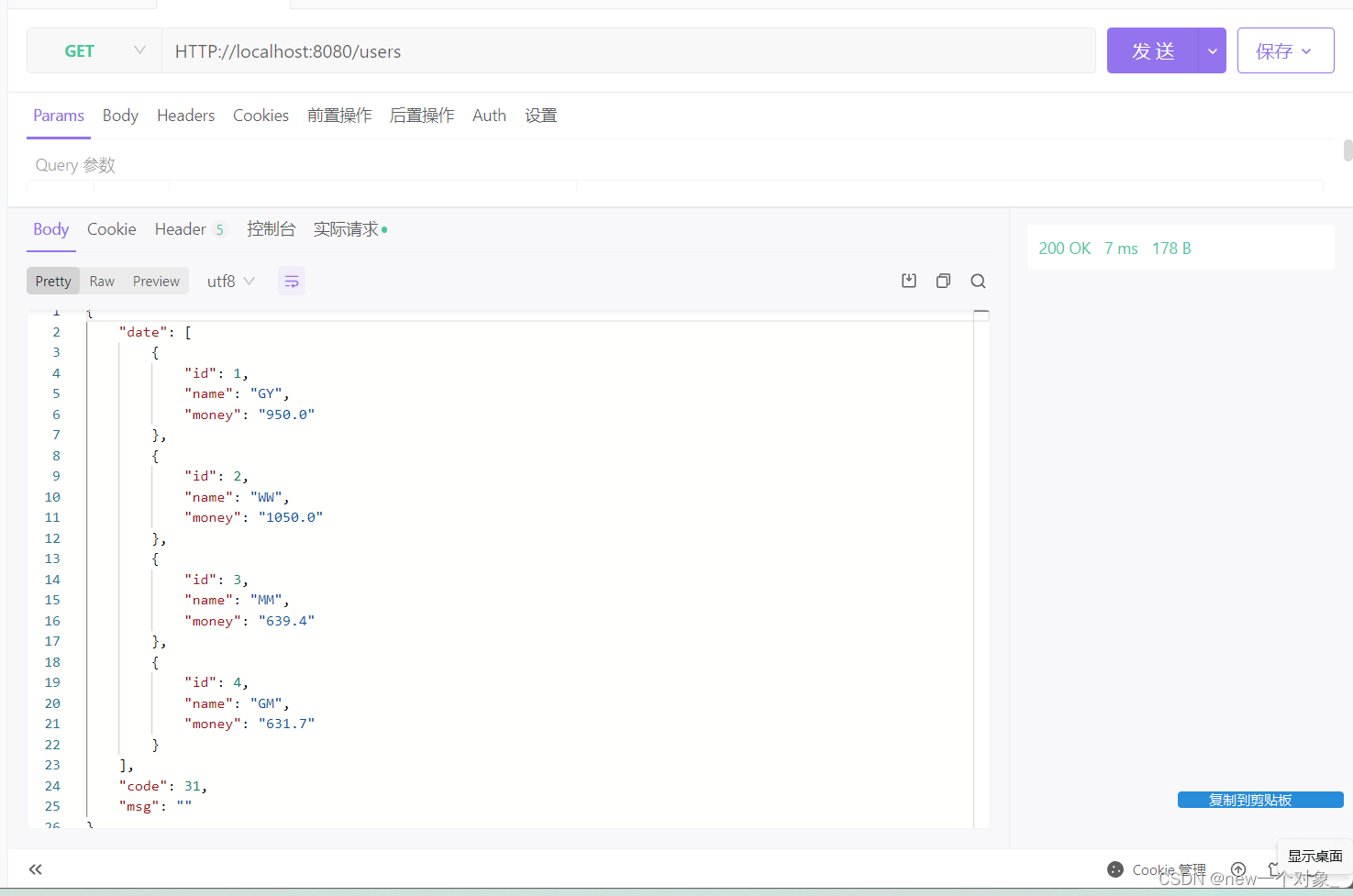
|