springboot 使用grpc
springboot 集成GRPC
工程结构:
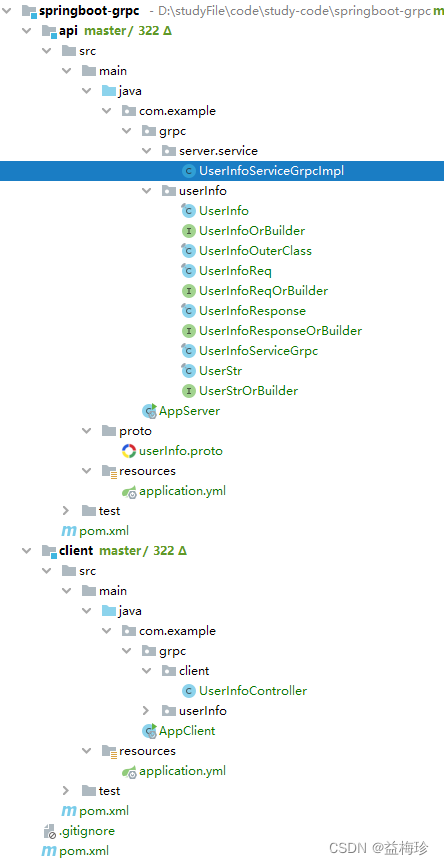
父级pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<packaging>pom</packaging>
<modules>
<module>api</module>
<module>client</module>
</modules>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.2</version>
<relativePath/>
</parent>
<groupId>com.example</groupId>
<artifactId>springboot-grpc</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-grpc</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>net.devh</groupId>
<artifactId>grpc-client-spring-boot-starter</artifactId>
<version>2.10.1.RELEASE</version>
</dependency>
<dependency>
<groupId>net.devh</groupId>
<artifactId>grpc-server-spring-boot-starter</artifactId>
<version>2.10.1.RELEASE</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
服务端(api)
proto
syntax = "proto3";
option java_multiple_files = true;
option java_package = "com.example.grpc.userInfo";
service UserInfoService {
rpc queryUserInfo(UserInfoReq) returns (UserInfoResponse) {}
rpc queryUserInfo2(UserInfoReq) returns (UserInfoResponse) {}
rpc queryUserInfo3(UserStr) returns (UserStr) {}
}
message UserStr{
string str = 1;
}
message UserInfoReq {
string name = 1;
int64 id = 2;
}
message UserInfoResponse {
int32 code = 1;
string msg = 2;
bool success = 3;
message Data {
UserInfo userInfo = 1;
}
Data data = 4;
}
message UserInfo {
int64 id = 1;
string name = 2;
string sex = 3;
string addr = 4;
}
UserInfoServiceGrpcImpl
package com.example.grpc.server.service;
import com.example.grpc.userInfo.*;
import io.grpc.stub.StreamObserver;
import net.devh.boot.grpc.server.service.GrpcService;
@GrpcService
public class UserInfoServiceGrpcImpl extends UserInfoServiceGrpc.UserInfoServiceImplBase {
@Override
public void queryUserInfo(UserInfoReq request, StreamObserver<UserInfoResponse> responseObserver) {
System.out.println("-------sever-----");
UserInfoResponse.Builder userInfoResp = UserInfoResponse.newBuilder();
userInfoResp.setCode(0).setMsg("success").setSuccess(true);
UserInfo.Builder userInfo = UserInfo.newBuilder();
userInfo.setId(request.getId());
userInfo.setName(request.getName());
userInfoResp.setData(UserInfoResponse.Data.newBuilder().setUserInfo(userInfo));
responseObserver.onNext(userInfoResp.build());
responseObserver.onCompleted();
}
@Override
public void queryUserInfo2(UserInfoReq request, StreamObserver<UserInfoResponse> responseObserver) {
super.queryUserInfo2(request, responseObserver);
}
@Override
public void queryUserInfo3(UserStr request, StreamObserver<UserStr> responseObserver) {
System.out.println("queryUserInfo3 =======> " + request.getStr());
responseObserver.onNext(UserStr.newBuilder().setStr("msg : success" + request.getStr()).build());
responseObserver.onCompleted();
}
}
AppServer
@SpringBootApplication
public class AppServer {
public static void main(String[] args) {
SpringApplication.run(AppServer.class, args);
System.out.println("server Hello World!");
}
}
application.yml
grpc:
server:
port: 8888
spring:
application:
name: grpc-server
server:
port: 8890
server的pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>springboot-grpc</artifactId>
<groupId>com.example</groupId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>api</artifactId>
<name>api</name>
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<extensions>
<extension>
<groupId>kr.motd.maven</groupId>
<artifactId>os-maven-plugin</artifactId>
<version>1.6.2</version>
</extension>
</extensions>
<plugins>
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<configuration>
<protocArtifact>com.google.protobuf:protoc:3.12.0:exe:${os.detected.classifier}</protocArtifact>
<pluginId>grpc-java</pluginId>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:1.34.1:exe:${os.detected.classifier}</pluginArtifact>
<outputDirectory>${project.basedir}/src/main/java</outputDirectory>
<clearOutputDirectory>false</clearOutputDirectory>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>build-helper-maven-plugin</artifactId>
<version>3.0.0</version>
<executions>
<execution>
<id>add-source</id>
<phase>generate-sources</phase>
<goals>
<goal>add-source</goal>
</goals>
<configuration>
<sources>
<source>${project.basedir}/src/main/gen</source>
<source>${project.basedir}/src/main/java</source>
</sources>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
客户端(client)
AppClient
UserInfoController
package com.example.grpc.client;
import com.example.grpc.userInfo.UserInfoServiceGrpc;
import com.example.grpc.userInfo.UserStr;
import net.devh.boot.grpc.client.inject.GrpcClient;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.ExecutionException;
@RestController
@RequestMapping("/userInfo")
public class UserInfoController {
@GrpcClient("grpc-server")
UserInfoServiceGrpc.UserInfoServiceFutureStub userInfoServiceStub;
@RequestMapping(value="/query/{id}")
public String queryUser(@PathVariable Integer id, String str) {
String userResp = null;
try {
System.out.println("-------sever-----");
userResp = userInfoServiceStub.queryUserInfo3(UserStr.newBuilder().setStr(str).build()).get().getStr();
} catch (InterruptedException e) {
} catch (ExecutionException e) {
}
return userResp;
}
}
@SpringBootApplication
public class AppClient {
public static void main(String[] args) {
SpringApplication.run(AppClient.class, args);
System.out.println("client Hello World!");
}
}
application.yml
grpc:
client:
grpc-server:
address: static://localhost:8888
enableKeepAlive: true
keepAliveWithoutCalls: true
negotiationType: plaintext
spring:
application:
name: grpc-client
server:
port: 8891
client 的pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.example</groupId>
<artifactId>springboot-grpc</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<groupId>com.example</groupId>
<artifactId>client</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>client</name>
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<extensions>
<extension>
<groupId>kr.motd.maven</groupId>
<artifactId>os-maven-plugin</artifactId>
<version>1.6.2</version>
</extension>
</extensions>
</build>
</project>
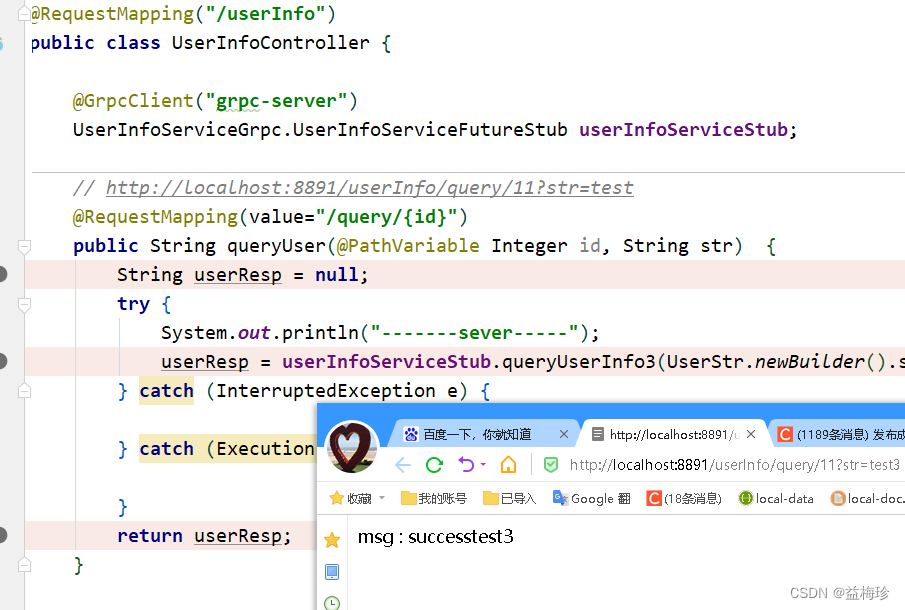
提示
编译服务端自动生成userInfo的文件代码,客户端可以继承服务端的pom或直接将服务端生成的userInfo复制一份倒客户端即可。
案例二:
pom文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<groupId>com.yymt</groupId>
<artifactId>zaxq-tag-community-property</artifactId>
<version>1.0.0</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>zaxq-tag</artifactId>
<packaging>jar</packaging>
<description>zaxq-tag</description>
<properties>
<grpc.version>1.29.0</grpc.version>
<protobuf.version>3.17.3</protobuf.version>
</properties>
<dependencies>
<dependency>
<groupId>com.yymt</groupId>
<artifactId>zaxq-tag-common</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
<version>2.1.2.RELEASE</version>
</dependency>
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.11</version>
</dependency>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpcore</artifactId>
<version>4.4.13</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
<dependency>
<groupId>org.dom4j</groupId>
<artifactId>dom4j</artifactId>
<version>2.1.3</version>
</dependency>
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>${protobuf.version}</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>${grpc.version}</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>${grpc.version}</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-okhttp</artifactId>
<version>${grpc.version}</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty</artifactId>
<version>${grpc.version}</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.0.0</version>
</dependency>
</dependencies>
<build>
<finalName>${project.artifactId}</finalName>
<extensions>
</extensions>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<skipTests>true</skipTests>
</configuration>
</plugin>
<plugin>
<groupId>com.spotify</groupId>
<artifactId>docker-maven-plugin</artifactId>
<version>0.4.14</version>
<configuration>
<imageName>renren/api</imageName>
<dockerDirectory>${project.basedir}</dockerDirectory>
<resources>
<resource>
<targetPath>/</targetPath>
<directory>${project.build.directory}</directory>
<include>${project.build.finalName}.jar</include>
</resource>
</resources>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-antrun-plugin</artifactId>
<executions>
<execution>
<id>delete</id>
<phase>clean</phase>
<configuration>
<target>
<delete file="../bin/${project.artifactId}.${project.packaging}"/>
</target>
</configuration>
<goals>
<goal>run</goal>
</goals>
</execution>
<execution>
<id>copy</id>
<phase>package</phase>
<configuration>
<target>
<copy file="target/${project.build.finalName}.${project.packaging}"
tofile="../../bin/${project.artifactId}.${project.packaging}"/>
</target>
</configuration>
<goals>
<goal>run</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
VideoFile.proto
syntax = "proto3";
option csharp_namespace = "NVR.GrpcService";
service VideoFile{
rpc DownloadByTime(DowFileRequest) returns(DowFileReply);
rpc GetDownloadPos(GetDownloadPosRequest) returns(GetDownloadPosReply);
rpc StopDownload(GetDownloadPosRequest) returns(GetDownloadPosReply);
rpc DownloadByName(DowFileByNameRequest) returns(DowFileReply);
rpc SetDeleteFileTask(DelFileRequest) returns(DelFileReply);
}
message DowFileRequest{
//设备Ip
string deviceIp = 1;
//设备端口,可空默认8000
int32 devicePort = 2;
//设备用户名,可空
string userName = 3;
//设备密码,可空
string password = 4;
//开始时间(yyyy-MM-dd HH:mm:ss)
string beginTime = 5;
//结束时间(yyyy-MM-dd HH:mm:ss)
string endTime = 6;
//设备通道ID
string channelID = 7;
//平台用户名
string platUserName = 8;
//平台用户Id
string platUserId = 9;
//文件名称(按文件下载服务方法的参数)
string fileName = 10;
}
message DowFileByNameRequest{
//设备Ip
string deviceIp = 1;
//设备端口,可空默认8000
int32 devicePort = 2;
//设备用户名,可空
string userName = 3;
//设备密码,可空
string password = 4;
//设备通道ID
string channelID = 5;
//平台用户名
string platUserName = 6;
//平台用户Id
string platUserId = 7;
//文件名称(按文件下载服务方法的参数)
string fileName = 8;
}
message GetDownloadPosRequest{
//设备Ip
string deviceIp = 1;
//平台用户名
string platUserName = 2;
//平台用户Id
string platUserId = 3;
//文件名称
string fileName = 4;
//下载句柄
int32 downHandle = 6;
}
message DelFileRequest{
//是否启动
bool isRun = 1;
//删除周期(小时)
float periodHour = 2;
}
message DowFileReply{
//返回代码(-1失败 1成功)
int32 code = 1;
//返回信息
string message = 2;
//文件名称
string fileName = 4;
//文件路径
string filePath = 5;
//下载句柄
int32 downHandle = 6;
}
message GetDownloadPosReply{
//返回代码(-1失败 1成功)
int32 code = 1;
//返回信息
string message = 2;
//下载进度(0~100)
int32 pos = 3;
}
message DelFileReply{
//返回代码(-1失败 1成功)
int32 code = 1;
//返回信息
string message = 2;
//是否启动
bool isRun = 3;
//删除周期
float periodHour = 4;
}
VideoFileSdkClient
package com.yymt.tag.global;
import com.yymt.common.exception.RRException;
import com.yymt.common.zaxq.bo.CmsDeviceBo;
import com.yymt.common.zaxq.bo.IpcNvrDeviceBriefBo;
import com.yymt.common.zaxq.service.IpcRecordService;
import com.yymt.tag.vo.DelFileReplyVo;
import com.yymt.tag.vo.DowFileReplyVo;
import com.yymt.tag.vo.GetDownloadPosReplyVo;
import io.grpc.Channel;
import io.grpc.ManagedChannelBuilder;
import org.apache.commons.lang3.tuple.Pair;
import org.jetbrains.annotations.NotNull;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import videorecord.VideoFileGrpc;
import videorecord.VideoFileOuterClass;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.*;
@Component
public class VideoFileSdkClient {
private final Logger logger = LoggerFactory.getLogger(getClass());
@Value("${spring.jackson.date-format:yyyy-MM-dd HH:mm:ss}")
private String dateTimeFormat;
@Value("${data.grpc-video-file-host-root:}")
private String videoFileHostRoot;
private final Map<String, Pair<VideoFileGrpc.VideoFileBlockingStub, VideoFileGrpc.VideoFileStub>>
stubMap = new HashMap<>(16);
private Pair<VideoFileGrpc.VideoFileBlockingStub, VideoFileGrpc.VideoFileStub> defaultStubs;
@Autowired
private IpcRecordService ipcRecordService;
@Autowired
public VideoFileSdkClient(@Value("${data.grpc-video-record-host}") String host
, @Value("${data.grpc-video-record-port}") int port) {
ManagedChannelBuilder<?> channelBuilder = ManagedChannelBuilder.forAddress(host, port).usePlaintext();
Channel channel = channelBuilder.build();
VideoFileGrpc.VideoFileBlockingStub blockingStub = VideoFileGrpc.newBlockingStub(channel);
VideoFileGrpc.VideoFileStub asyncStub = VideoFileGrpc.newStub(channel);
String key = host + ":" + port + ":" + false;
Pair<VideoFileGrpc.VideoFileBlockingStub, VideoFileGrpc.VideoFileStub> pair
= Pair.of(blockingStub, asyncStub);
stubMap.put(key, pair);
defaultStubs = pair;
}
private Pair<VideoFileGrpc.VideoFileBlockingStub, VideoFileGrpc.VideoFileStub>
buildStub(String host, int port, boolean useTls) {
ManagedChannelBuilder<?> channelBuilder;
if (useTls) {
channelBuilder = ManagedChannelBuilder.forAddress(host, port).useTransportSecurity();
} else {
channelBuilder = ManagedChannelBuilder.forAddress(host, port).usePlaintext();
}
Channel channel = channelBuilder.build();
VideoFileGrpc.VideoFileBlockingStub blockingStub = VideoFileGrpc.newBlockingStub(channel);
VideoFileGrpc.VideoFileStub asyncStub = VideoFileGrpc.newStub(channel);
String key = host + ":" + port + ":" + useTls;
Pair<VideoFileGrpc.VideoFileBlockingStub, VideoFileGrpc.VideoFileStub> pair = Pair.of(blockingStub, asyncStub);
stubMap.put(key, pair);
return pair;
}
public VideoFileOuterClass.DowFileReply downloadByTime(VideoFileOuterClass.DowFileRequest request) {
VideoFileGrpc.VideoFileBlockingStub blockingStub = defaultStubs.getLeft();
return blockingStub.downloadByTime(request);
}
public VideoFileOuterClass.GetDownloadPosReply getDownloadPos(VideoFileOuterClass.GetDownloadPosRequest request) {
VideoFileGrpc.VideoFileBlockingStub blockingStub = defaultStubs.getLeft();
return blockingStub.getDownloadPos(request);
}
public VideoFileOuterClass.GetDownloadPosReply stopDownload(VideoFileOuterClass.GetDownloadPosRequest request) {
VideoFileGrpc.VideoFileBlockingStub blockingStub = defaultStubs.getLeft();
return blockingStub.stopDownload(request);
}
public VideoFileOuterClass.DowFileReply downloadByName(VideoFileOuterClass.DowFileByNameRequest request) {
VideoFileGrpc.VideoFileBlockingStub blockingStub = defaultStubs.getLeft();
return blockingStub.downloadByName(request);
}
public VideoFileOuterClass.DelFileReply setDeleteFileTask(VideoFileOuterClass.DelFileRequest request) {
VideoFileGrpc.VideoFileBlockingStub blockingStub = defaultStubs.getLeft();
return blockingStub.setDeleteFileTask(request);
}
public DowFileReplyVo downloadByTimeGeneral(
String deviceId, LocalDateTime beginTime, LocalDateTime endTime,
String platUserName, String platUserId) {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(dateTimeFormat);
String beginTimeStr = formatter.format(beginTime);
String endTimeStr = formatter.format(endTime);
IpcNvrDeviceBriefBo ipcNvr = getIpcNvrDeviceBriefBo(deviceId);
String deviceIp = ipcNvr.getNvrRemoteIp();
String userName = ipcNvr.getNvrUserName();
String password = ipcNvr.getNvrPassword();
String channelID = String.valueOf(ipcNvr.getIpcChannelNo());
VideoFileOuterClass.DowFileRequest.Builder dowFileRequestBuilder
= VideoFileOuterClass.DowFileRequest.newBuilder();
VideoFileOuterClass.DowFileRequest dowFileRequest = dowFileRequestBuilder
.setDeviceIp(deviceIp)
.setUserName(userName)
.setPassword(password)
.setBeginTime(beginTimeStr)
.setEndTime(endTimeStr)
.setChannelID(channelID)
.setPlatUserName(platUserName)
.setPlatUserId(platUserId)
.build();
VideoFileOuterClass.DowFileReply dowFileReply = downloadByTime(dowFileRequest);
return new DowFileReplyVo(dowFileReply, videoFileHostRoot);
}
public GetDownloadPosReplyVo getDownloadPosGeneral(
String deviceId, String fileName, int downHandle,
String platUserName, String platUserId) {
IpcNvrDeviceBriefBo ipcNvr = getIpcNvrDeviceBriefBo(deviceId);
String deviceIp = ipcNvr.getNvrRemoteIp();
VideoFileOuterClass.GetDownloadPosRequest.Builder getDownloadPosRequestBuilder
= VideoFileOuterClass.GetDownloadPosRequest.newBuilder();
VideoFileOuterClass.GetDownloadPosRequest getDownloadPosRequest = getDownloadPosRequestBuilder
.setDeviceIp(deviceIp)
.setPlatUserName(platUserName)
.setPlatUserId(platUserId)
.setFileName(fileName)
.setDownHandle(downHandle)
.build();
VideoFileOuterClass.GetDownloadPosReply getDownloadPosReply = getDownloadPos(getDownloadPosRequest);
return new GetDownloadPosReplyVo(getDownloadPosReply);
}
public GetDownloadPosReplyVo stopDownloadGeneral(
String deviceId, String fileName, int downHandle,
String platUserName, String platUserId) {
IpcNvrDeviceBriefBo ipcNvr = getIpcNvrDeviceBriefBo(deviceId);
String deviceIp = ipcNvr.getNvrRemoteIp();
VideoFileOuterClass.GetDownloadPosRequest.Builder getDownloadPosRequestBuilder
= VideoFileOuterClass.GetDownloadPosRequest.newBuilder();
VideoFileOuterClass.GetDownloadPosRequest getDownloadPosRequest = getDownloadPosRequestBuilder
.setDeviceIp(deviceIp)
.setFileName(fileName)
.setDownHandle(downHandle)
.setPlatUserName(platUserName)
.setPlatUserId(platUserId)
.build();
VideoFileOuterClass.GetDownloadPosReply getDownloadPosReply = stopDownload(getDownloadPosRequest);
return new GetDownloadPosReplyVo(getDownloadPosReply);
}
public DowFileReplyVo downloadByNameGeneral(
String deviceId, String fileName,
String platUserName, String platUserId) {
IpcNvrDeviceBriefBo ipcNvr = getIpcNvrDeviceBriefBo(deviceId);
String deviceIp = ipcNvr.getNvrRemoteIp();
String userName = ipcNvr.getNvrUserName();
String password = ipcNvr.getNvrPassword();
String channelID = String.valueOf(ipcNvr.getIpcChannelNo());
VideoFileOuterClass.DowFileByNameRequest.Builder dowFileByNameRequestBuilder
= VideoFileOuterClass.DowFileByNameRequest.newBuilder();
VideoFileOuterClass.DowFileByNameRequest dowFileByNameRequest = dowFileByNameRequestBuilder
.setDeviceIp(deviceIp)
.setUserName(userName)
.setPassword(password)
.setChannelID(channelID)
.setPlatUserName(platUserName)
.setPlatUserId(platUserId)
.setFileName(fileName)
.build();
VideoFileOuterClass.DowFileReply dowFileReply = downloadByName(dowFileByNameRequest);
return new DowFileReplyVo(dowFileReply, videoFileHostRoot);
}
public DelFileReplyVo setDeleteFileTaskGeneral(boolean isRun, float periodHour) {
VideoFileOuterClass.DelFileRequest.Builder delFileRequestBuilder
= VideoFileOuterClass.DelFileRequest.newBuilder();
VideoFileOuterClass.DelFileRequest delFileRequest = delFileRequestBuilder
.setIsRun(isRun)
.setPeriodHour(periodHour)
.build();
VideoFileOuterClass.DelFileReply delFileReply = setDeleteFileTask(delFileRequest);
return new DelFileReplyVo(delFileReply);
}
@NotNull
private IpcNvrDeviceBriefBo getIpcNvrDeviceBriefBo(String deviceId) {
List<CmsDeviceBo> cmsIpcDeviceBoList = ApplicationData.cmsIpcDeviceBoList;
if (cmsIpcDeviceBoList == null)
throw new RRException("全局IPC设备列表为空");
Optional<CmsDeviceBo> deviceOpt = cmsIpcDeviceBoList.stream()
.filter(d -> Objects.equals(d.getDeviceID(), deviceId)).findFirst();
if (!deviceOpt.isPresent())
throw new RRException("设备不存在,deviceId:" + deviceId);
CmsDeviceBo device = deviceOpt.get();
String nvrDeviceId = device.getNvrDeviceID();
List<CmsDeviceBo> cmsNvrDeviceBoList = ApplicationData.cmsNvrDeviceBoList;
if (cmsNvrDeviceBoList == null)
throw new RRException("全局NVR设备列表为空");
Optional<CmsDeviceBo> nvrOpt = cmsNvrDeviceBoList.stream()
.filter(d -> Objects.equals(nvrDeviceId, d.getDeviceID())).findFirst();
if (!nvrOpt.isPresent())
throw new RRException("该NVR不存在,nvrDeviceId:" + nvrDeviceId);
CmsDeviceBo nvr = nvrOpt.get();
return new IpcNvrDeviceBriefBo(device, nvr);
}
}
|